Kirigami::Platform::PlatformTheme
#include <Kirigami/PlatformTheme>
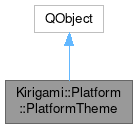
Public Types | |
enum | ColorGroup { Disabled = QPalette::Disabled , Active = QPalette::Active , Inactive = QPalette::Inactive , Normal = QPalette::Normal , ColorGroupCount } |
enum | ColorSet { View = 0 , Window , Button , Selection , Tooltip , Complementary , Header , ColorSetCount } |
![]() | |
typedef | QObjectList |
Signals | |
void | colorGroupChanged (Kirigami::Platform::PlatformTheme::ColorGroup colorGroup) |
void | colorsChanged () |
void | colorSetChanged (Kirigami::Platform::PlatformTheme::ColorSet colorSet) |
void | defaultFontChanged (const QFont &font) |
void | inheritChanged (bool inherit) |
void | paletteChanged (const QPalette &pal) |
void | smallFontChanged (const QFont &font) |
void | useAlternateBackgroundColorChanged (bool alternate) |
Static Public Member Functions | |
static PlatformTheme * | qmlAttachedProperties (QObject *object) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
bool | event (QEvent *event) override |
void | setActiveBackgroundColor (const QColor &color) |
void | setActiveTextColor (const QColor &color) |
void | setAlternateBackgroundColor (const QColor &color) |
void | setBackgroundColor (const QColor &color) |
void | setDefaultFont (const QFont &defaultFont) |
void | setDisabledTextColor (const QColor &color) |
void | setFocusColor (const QColor &color) |
void | setHighlightColor (const QColor &color) |
void | setHighlightedTextColor (const QColor &color) |
void | setHoverColor (const QColor &color) |
void | setLinkBackgroundColor (const QColor &color) |
void | setLinkColor (const QColor &color) |
void | setNegativeBackgroundColor (const QColor &color) |
void | setNegativeTextColor (const QColor &color) |
void | setNeutralBackgroundColor (const QColor &color) |
void | setNeutralTextColor (const QColor &color) |
void | setPositiveBackgroundColor (const QColor &color) |
void | setPositiveTextColor (const QColor &color) |
void | setSmallFont (const QFont &smallFont) |
void | setSupportsIconColoring (bool support) |
void | setTextColor (const QColor &color) |
void | setVisitedLinkBackgroundColor (const QColor &color) |
void | setVisitedLinkColor (const QColor &color) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class is the base for color management in Kirigami, different platforms can reimplement this class to integrate with system platform colors of a given platform.
Definition at line 33 of file platformtheme.h.
Member Enumeration Documentation
◆ ColorGroup
enum Kirigami::Platform::PlatformTheme::ColorGroup |
Definition at line 225 of file platformtheme.h.
◆ ColorSet
Enumerator | |
---|---|
View | Color set for item views, usually the lightest of all. |
Window | Default Color set for windows and "chrome" areas. |
Button | Color set used by buttons. |
Selection | Color set used by selected areas. |
Tooltip | Color set used by tooltips. |
Complementary | Color set meant to be complementary to Window: usually is a dark theme for light themes. |
Header | Color set to be used by heading areas of applications, such as toolbars. |
Definition at line 205 of file platformtheme.h.
Property Documentation
◆ activeBackgroundColor
|
readwrite |
Background for areas that are active or requesting attention.
Definition at line 137 of file platformtheme.h.
◆ activeTextColor
|
readwrite |
Foreground for areas that are active or requesting attention.
Definition at line 87 of file platformtheme.h.
◆ alternateBackgroundColor
|
readwrite |
The generic background color Alternate background; for example, for use in lists.
This color may be the same as BackgroundNormal, especially in sets other than View and Window.
Definition at line 126 of file platformtheme.h.
◆ backgroundColor
|
readwrite |
The generic background color.
Definition at line 118 of file platformtheme.h.
◆ colorGroup
|
readwrite |
This enumeration describes the color group used to generate the colors.
The enum value is based upon QPalette::ColorGroup and has the same values. It's redefined here in order to make it work with QML.
- Since
- 4.43
Definition at line 54 of file platformtheme.h.
◆ colorSet
|
readwrite |
This enumeration describes the color set for which a color is being selected.
Color sets define a color "environment", suitable for drawing all parts of a given region. Colors from different sets should not be combined.
Definition at line 46 of file platformtheme.h.
◆ defaultFont
|
read |
Definition at line 188 of file platformtheme.h.
◆ disabledTextColor
|
readwrite |
Foreground color for disabled areas, usually a mid-gray.
- Note
- Depending on the implementation, the color used for this property may not be based on the disabled palette. For example, for the Plasma implementation, "Inactive Text Color" of the active palette is used.
Definition at line 76 of file platformtheme.h.
◆ focusColor
|
readwrite |
A decoration color that indicates active focus.
Definition at line 174 of file platformtheme.h.
◆ frameContrast
|
read |
Definition at line 198 of file platformtheme.h.
◆ highlightColor
|
readwrite |
The background color for selected areas.
Definition at line 132 of file platformtheme.h.
◆ highlightedTextColor
|
readwrite |
Color for text that has been highlighted, often is a light color while normal text is dark.
Definition at line 81 of file platformtheme.h.
◆ hoverColor
|
readwrite |
A decoration color that indicates mouse hovering.
Definition at line 179 of file platformtheme.h.
◆ inherit
|
readwrite |
If true, the colorSet will be inherited from the colorset of a theme of one of the ancestor items default: true.
Definition at line 61 of file platformtheme.h.
◆ lightFrameContrast
|
read |
Definition at line 202 of file platformtheme.h.
◆ linkBackgroundColor
|
readwrite |
Background color for links.
Definition at line 143 of file platformtheme.h.
◆ linkColor
|
readwrite |
Color for links.
Definition at line 92 of file platformtheme.h.
◆ negativeBackgroundColor
|
readwrite |
Background color for negative areas, such as critical errors and destructive actions.
Definition at line 155 of file platformtheme.h.
◆ negativeTextColor
|
readwrite |
Foreground color for negative areas, such as critical error text.
Definition at line 102 of file platformtheme.h.
◆ neutralBackgroundColor
|
readwrite |
Background color for neutral areas, such as warnings (but not critical)
Definition at line 161 of file platformtheme.h.
◆ neutralTextColor
|
readwrite |
Foreground color for neutral areas, such as warning texts (but not critical)
Definition at line 107 of file platformtheme.h.
◆ palette
|
read |
Definition at line 194 of file platformtheme.h.
◆ positiveBackgroundColor
|
readwrite |
Background color for positive areas, such as success messages and trusted content.
Definition at line 167 of file platformtheme.h.
◆ positiveTextColor
|
readwrite |
Success messages, trusted content.
Definition at line 112 of file platformtheme.h.
◆ smallFont
|
read |
Definition at line 191 of file platformtheme.h.
◆ textColor
|
readwrite |
Color for normal foregrounds, usually text, but not limited to it, anything that should be painted with a clear contrast should use this color.
Definition at line 68 of file platformtheme.h.
◆ useAlternateBackgroundColor
|
readwrite |
Hint for item views to actually make use of the alternate background color feature.
Definition at line 184 of file platformtheme.h.
◆ visitedLinkBackgroundColor
|
readwrite |
Background color for visited links, usually a bit darker than linkBackgroundColor.
Definition at line 149 of file platformtheme.h.
◆ visitedLinkColor
|
readwrite |
Color for visited links, usually a bit darker than linkColor.
Definition at line 97 of file platformtheme.h.
Constructor & Destructor Documentation
◆ PlatformTheme()
|
explicit |
Definition at line 386 of file platformtheme.cpp.
◆ ~PlatformTheme()
|
override |
Definition at line 407 of file platformtheme.cpp.
Member Function Documentation
◆ activeBackgroundColor()
QColor Kirigami::Platform::PlatformTheme::activeBackgroundColor | ( | ) | const |
Definition at line 498 of file platformtheme.cpp.
◆ activeTextColor()
QColor Kirigami::Platform::PlatformTheme::activeTextColor | ( | ) | const |
Definition at line 493 of file platformtheme.cpp.
◆ alternateBackgroundColor()
QColor Kirigami::Platform::PlatformTheme::alternateBackgroundColor | ( | ) | const |
Definition at line 488 of file platformtheme.cpp.
◆ backgroundColor()
QColor Kirigami::Platform::PlatformTheme::backgroundColor | ( | ) | const |
Definition at line 483 of file platformtheme.cpp.
◆ colorGroup()
PlatformTheme::ColorGroup Kirigami::Platform::PlatformTheme::colorGroup | ( | ) | const |
Definition at line 441 of file platformtheme.cpp.
◆ colorSet()
PlatformTheme::ColorSet Kirigami::Platform::PlatformTheme::colorSet | ( | ) | const |
Definition at line 426 of file platformtheme.cpp.
◆ defaultFont()
QFont Kirigami::Platform::PlatformTheme::defaultFont | ( | ) | const |
Definition at line 664 of file platformtheme.cpp.
◆ disabledTextColor()
QColor Kirigami::Platform::PlatformTheme::disabledTextColor | ( | ) | const |
Definition at line 468 of file platformtheme.cpp.
◆ event()
|
overrideprotectedvirtual |
Reimplemented from QObject.
Definition at line 910 of file platformtheme.cpp.
◆ focusColor()
QColor Kirigami::Platform::PlatformTheme::focusColor | ( | ) | const |
Definition at line 553 of file platformtheme.cpp.
◆ frameContrast()
qreal Kirigami::Platform::PlatformTheme::frameContrast | ( | ) | const |
Definition at line 690 of file platformtheme.cpp.
◆ highlightColor()
QColor Kirigami::Platform::PlatformTheme::highlightColor | ( | ) | const |
Definition at line 473 of file platformtheme.cpp.
◆ highlightedTextColor()
QColor Kirigami::Platform::PlatformTheme::highlightedTextColor | ( | ) | const |
Definition at line 478 of file platformtheme.cpp.
◆ hoverColor()
QColor Kirigami::Platform::PlatformTheme::hoverColor | ( | ) | const |
Definition at line 558 of file platformtheme.cpp.
◆ iconFromTheme()
|
virtual |
Definition at line 836 of file platformtheme.cpp.
◆ inherit()
bool Kirigami::Platform::PlatformTheme::inherit | ( | ) | const |
Definition at line 446 of file platformtheme.cpp.
◆ lightFrameContrast()
qreal Kirigami::Platform::PlatformTheme::lightFrameContrast | ( | ) | const |
Definition at line 698 of file platformtheme.cpp.
◆ linkBackgroundColor()
QColor Kirigami::Platform::PlatformTheme::linkBackgroundColor | ( | ) | const |
Definition at line 508 of file platformtheme.cpp.
◆ linkColor()
QColor Kirigami::Platform::PlatformTheme::linkColor | ( | ) | const |
Definition at line 503 of file platformtheme.cpp.
◆ negativeBackgroundColor()
QColor Kirigami::Platform::PlatformTheme::negativeBackgroundColor | ( | ) | const |
Definition at line 528 of file platformtheme.cpp.
◆ negativeTextColor()
QColor Kirigami::Platform::PlatformTheme::negativeTextColor | ( | ) | const |
Definition at line 523 of file platformtheme.cpp.
◆ neutralBackgroundColor()
QColor Kirigami::Platform::PlatformTheme::neutralBackgroundColor | ( | ) | const |
Definition at line 538 of file platformtheme.cpp.
◆ neutralTextColor()
QColor Kirigami::Platform::PlatformTheme::neutralTextColor | ( | ) | const |
Definition at line 533 of file platformtheme.cpp.
◆ palette()
QPalette Kirigami::Platform::PlatformTheme::palette | ( | ) | const |
Definition at line 821 of file platformtheme.cpp.
◆ positiveBackgroundColor()
QColor Kirigami::Platform::PlatformTheme::positiveBackgroundColor | ( | ) | const |
Definition at line 548 of file platformtheme.cpp.
◆ positiveTextColor()
QColor Kirigami::Platform::PlatformTheme::positiveTextColor | ( | ) | const |
Definition at line 543 of file platformtheme.cpp.
◆ qmlAttachedProperties()
|
static |
Definition at line 853 of file platformtheme.cpp.
◆ setActiveBackgroundColor()
|
protected |
Definition at line 599 of file platformtheme.cpp.
◆ setActiveTextColor()
|
protected |
Definition at line 594 of file platformtheme.cpp.
◆ setAlternateBackgroundColor()
|
protected |
Definition at line 579 of file platformtheme.cpp.
◆ setBackgroundColor()
|
protected |
Definition at line 574 of file platformtheme.cpp.
◆ setColorGroup()
void Kirigami::Platform::PlatformTheme::setColorGroup | ( | PlatformTheme::ColorGroup | colorGroup | ) |
Definition at line 431 of file platformtheme.cpp.
◆ setColorSet()
void Kirigami::Platform::PlatformTheme::setColorSet | ( | PlatformTheme::ColorSet | colorSet | ) |
Definition at line 416 of file platformtheme.cpp.
◆ setCustomActiveBackgroundColor()
void Kirigami::Platform::PlatformTheme::setCustomActiveBackgroundColor | ( | const QColor & | color = QColor() | ) |
Definition at line 741 of file platformtheme.cpp.
◆ setCustomActiveTextColor()
Definition at line 736 of file platformtheme.cpp.
◆ setCustomAlternateBackgroundColor()
void Kirigami::Platform::PlatformTheme::setCustomAlternateBackgroundColor | ( | const QColor & | color = QColor() | ) |
Definition at line 721 of file platformtheme.cpp.
◆ setCustomBackgroundColor()
Definition at line 716 of file platformtheme.cpp.
◆ setCustomDisabledTextColor()
void Kirigami::Platform::PlatformTheme::setCustomDisabledTextColor | ( | const QColor & | color = QColor() | ) |
Definition at line 711 of file platformtheme.cpp.
◆ setCustomFocusColor()
Definition at line 801 of file platformtheme.cpp.
◆ setCustomHighlightColor()
Definition at line 726 of file platformtheme.cpp.
◆ setCustomHighlightedTextColor()
void Kirigami::Platform::PlatformTheme::setCustomHighlightedTextColor | ( | const QColor & | color = QColor() | ) |
Definition at line 731 of file platformtheme.cpp.
◆ setCustomHoverColor()
Definition at line 796 of file platformtheme.cpp.
◆ setCustomLinkBackgroundColor()
void Kirigami::Platform::PlatformTheme::setCustomLinkBackgroundColor | ( | const QColor & | color = QColor() | ) |
Definition at line 751 of file platformtheme.cpp.
◆ setCustomLinkColor()
Definition at line 746 of file platformtheme.cpp.
◆ setCustomNegativeBackgroundColor()
void Kirigami::Platform::PlatformTheme::setCustomNegativeBackgroundColor | ( | const QColor & | color = QColor() | ) |
Definition at line 771 of file platformtheme.cpp.
◆ setCustomNegativeTextColor()
void Kirigami::Platform::PlatformTheme::setCustomNegativeTextColor | ( | const QColor & | color = QColor() | ) |
Definition at line 766 of file platformtheme.cpp.
◆ setCustomNeutralBackgroundColor()
void Kirigami::Platform::PlatformTheme::setCustomNeutralBackgroundColor | ( | const QColor & | color = QColor() | ) |
Definition at line 781 of file platformtheme.cpp.
◆ setCustomNeutralTextColor()
void Kirigami::Platform::PlatformTheme::setCustomNeutralTextColor | ( | const QColor & | color = QColor() | ) |
Definition at line 776 of file platformtheme.cpp.
◆ setCustomPositiveBackgroundColor()
void Kirigami::Platform::PlatformTheme::setCustomPositiveBackgroundColor | ( | const QColor & | color = QColor() | ) |
Definition at line 791 of file platformtheme.cpp.
◆ setCustomPositiveTextColor()
void Kirigami::Platform::PlatformTheme::setCustomPositiveTextColor | ( | const QColor & | color = QColor() | ) |
Definition at line 786 of file platformtheme.cpp.
◆ setCustomTextColor()
Definition at line 706 of file platformtheme.cpp.
◆ setCustomVisitedLinkBackgroundColor()
void Kirigami::Platform::PlatformTheme::setCustomVisitedLinkBackgroundColor | ( | const QColor & | color = QColor() | ) |
Definition at line 761 of file platformtheme.cpp.
◆ setCustomVisitedLinkColor()
void Kirigami::Platform::PlatformTheme::setCustomVisitedLinkColor | ( | const QColor & | color = QColor() | ) |
Definition at line 756 of file platformtheme.cpp.
◆ setDefaultFont()
|
protected |
Definition at line 669 of file platformtheme.cpp.
◆ setDisabledTextColor()
|
protected |
Definition at line 569 of file platformtheme.cpp.
◆ setFocusColor()
|
protected |
Definition at line 659 of file platformtheme.cpp.
◆ setHighlightColor()
|
protected |
Definition at line 584 of file platformtheme.cpp.
◆ setHighlightedTextColor()
|
protected |
Definition at line 589 of file platformtheme.cpp.
◆ setHoverColor()
|
protected |
Definition at line 654 of file platformtheme.cpp.
◆ setInherit()
void Kirigami::Platform::PlatformTheme::setInherit | ( | bool | inherit | ) |
Definition at line 451 of file platformtheme.cpp.
◆ setLinkBackgroundColor()
|
protected |
Definition at line 609 of file platformtheme.cpp.
◆ setLinkColor()
|
protected |
Definition at line 604 of file platformtheme.cpp.
◆ setNegativeBackgroundColor()
|
protected |
Definition at line 629 of file platformtheme.cpp.
◆ setNegativeTextColor()
|
protected |
Definition at line 624 of file platformtheme.cpp.
◆ setNeutralBackgroundColor()
|
protected |
Definition at line 639 of file platformtheme.cpp.
◆ setNeutralTextColor()
|
protected |
Definition at line 634 of file platformtheme.cpp.
◆ setPositiveBackgroundColor()
|
protected |
Definition at line 649 of file platformtheme.cpp.
◆ setPositiveTextColor()
|
protected |
Definition at line 644 of file platformtheme.cpp.
◆ setSmallFont()
|
protected |
Definition at line 682 of file platformtheme.cpp.
◆ setSupportsIconColoring()
|
protected |
Definition at line 848 of file platformtheme.cpp.
◆ setTextColor()
|
protected |
Definition at line 564 of file platformtheme.cpp.
◆ setUseAlternateBackgroundColor()
void Kirigami::Platform::PlatformTheme::setUseAlternateBackgroundColor | ( | bool | alternate | ) |
Definition at line 811 of file platformtheme.cpp.
◆ setVisitedLinkBackgroundColor()
|
protected |
Definition at line 619 of file platformtheme.cpp.
◆ setVisitedLinkColor()
|
protected |
Definition at line 614 of file platformtheme.cpp.
◆ smallFont()
QFont Kirigami::Platform::PlatformTheme::smallFont | ( | ) | const |
Definition at line 677 of file platformtheme.cpp.
◆ supportsIconColoring()
bool Kirigami::Platform::PlatformTheme::supportsIconColoring | ( | ) | const |
Definition at line 843 of file platformtheme.cpp.
◆ textColor()
QColor Kirigami::Platform::PlatformTheme::textColor | ( | ) | const |
Definition at line 463 of file platformtheme.cpp.
◆ useAlternateBackgroundColor()
bool Kirigami::Platform::PlatformTheme::useAlternateBackgroundColor | ( | ) | const |
Definition at line 806 of file platformtheme.cpp.
◆ visitedLinkBackgroundColor()
QColor Kirigami::Platform::PlatformTheme::visitedLinkBackgroundColor | ( | ) | const |
Definition at line 518 of file platformtheme.cpp.
◆ visitedLinkColor()
QColor Kirigami::Platform::PlatformTheme::visitedLinkColor | ( | ) | const |
Definition at line 513 of file platformtheme.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 16:56:52 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.