KParts::NavigationExtension
#include <KParts/NavigationExtension>
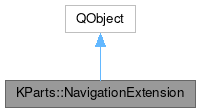
Public Types | |
typedef QMap< QString, QList< QAction * > > | ActionGroupMap |
typedef QMap< QByteArray, int > | ActionNumberMap |
typedef QMap< QByteArray, QByteArray > | ActionSlotMap |
enum | PopupFlag { DefaultPopupItems = 0x0000 , ShowBookmark = 0x0008 , ShowCreateDirectory = 0x0010 , ShowTextSelectionItems = 0x0020 , NoDeletion = 0x0040 , IsLink = 0x0080 , ShowUrlOperations = 0x0100 , ShowProperties = 0x200 } |
typedef QFlags< PopupFlag > | PopupFlags |
Properties | |
bool | urlDropHandling |
![]() | |
objectName | |
Public Member Functions | |
NavigationExtension (KParts::ReadOnlyPart *parent) | |
QString | actionText (const char *name) const |
bool | isActionEnabled (const char *name) const |
bool | isURLDropHandlingEnabled () const |
void | pasteRequest () |
virtual void | restoreState (QDataStream &stream) |
virtual void | saveState (QDataStream &stream) |
void | setURLDropHandlingEnabled (bool enable) |
virtual int | xOffset () |
virtual int | yOffset () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static ActionSlotMap * | actionSlotMap () |
static NavigationExtension * | childObject (QObject *obj) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
An extension to KParts::ReadOnlyPart, which allows a better integration of parts with browsers (in particular Konqueror).
Remember that ReadOnlyPart only has openUrl(QUrl) and a few arguments() but not much more. For full-fledged browsing, we need much more than that, including enabling/disabling of standard actions (print, copy, paste...), allowing parts to save and restore their data into the back/forward history, allowing parts to control the location bar URL, to requests URLs to be opened by the hosting browser, etc.
The part developer needs to define its own class derived from BrowserExtension, to implement the virtual methods [and the standard-actions slots, see below].
The way to associate the BrowserExtension with the part is to simply create the BrowserExtension as a child of the part (in QObject's terms). The hosting application will look for it automatically.
Another aspect of the browser integration is that a set of standard actions are provided by the browser, but implemented by the part (for the actions it supports).
The following standard actions are defined by the host of the view:
[selection-dependent actions]
cut
: Copy selected items to clipboard and store 'not cut' in clipboard.copy
: Copy selected items to clipboard and store 'cut' in clipboard.paste
: Paste clipboard into view URL.pasteTo(const QUrl &)
: Paste clipboard into given URL.searchProvider
: Lookup selected text at default search provider
[normal actions]
- None anymore.
The view defines a slot with the name of the action in order to implement the action. The browser will detect the slot automatically and connect its action to it when appropriate (i.e. when the view is active).
The selection-dependent actions are disabled by default and the view should enable them when the selection changes, emitting enableAction().
The normal actions do not depend on the selection.
A special case is the configuration slots, not connected to any action directly.
[configuration slot]
reparseConfiguration
: Re-read configuration and apply it.disableScrolling:
no scrollbars
Definition at line 87 of file navigationextension.h.
Member Typedef Documentation
◆ ActionGroupMap
typedef QMap<QString, QList<QAction *> > KParts::NavigationExtension::ActionGroupMap |
Associates a list of actions with a predefined name known by the host's popupmenu: "editactions" for actions related text editing, "linkactions" for actions related to hyperlinks, "partactions" for any other actions provided by the part.
Definition at line 239 of file navigationextension.h.
◆ ActionNumberMap
typedef QMap<QByteArray, int> KParts::NavigationExtension::ActionNumberMap |
Definition at line 410 of file navigationextension.h.
◆ ActionSlotMap
Definition at line 189 of file navigationextension.h.
◆ PopupFlags
Stores a combination of PopupFlag values.
Definition at line 121 of file navigationextension.h.
Member Enumeration Documentation
◆ PopupFlag
Set of flags passed via the popupMenu signal, to ask for some items in the popup menu.
- See also
- PopupFlags
Definition at line 105 of file navigationextension.h.
Property Documentation
◆ urlDropHandling
|
readwrite |
Definition at line 90 of file navigationextension.h.
Constructor & Destructor Documentation
◆ NavigationExtension()
|
explicit |
Constructor.
- Parameters
-
parent The KParts::ReadOnlyPart that this extension ... "extends" :)
Definition at line 95 of file navigationextension.cpp.
◆ ~NavigationExtension()
|
override |
Definition at line 121 of file navigationextension.cpp.
Member Function Documentation
◆ actionSlotMap()
|
static |
Returns a pointer to the static map containing the action names as keys and corresponding SLOT()'ified method names as data entries.
The map is created if it doesn't exist yet.
This is very useful for the host component, when connecting the own signals with the extension's slots. Basically you iterate over the map, check if the extension implements the slot and connect to the slot using the data value of your map iterator. Checking if the extension implements a certain slot can be done like this:
(note that actionName
is the iterator's key value if already iterating over the action slot map, returned by this method)
Connecting to the slot can be done like this:
(where "mapIterator" is your ActionSlotMap iterator)
Definition at line 267 of file navigationextension.cpp.
◆ actionText()
QString NavigationExtension::actionText | ( | const char * | name | ) | const |
- Returns
- the text of an action, if it was set explicitly by the part. When the setActionText signal is emitted, the browserextension stores the text of the action internally, so that it's possible to query later for the text of the action, using this method.
Definition at line 257 of file navigationextension.cpp.
◆ addWebSideBar
Ask the hosting application to add a new HTML (aka Mozilla/Netscape) SideBar entry.
◆ childObject()
|
static |
Queries obj
for a child object which inherits from this BrowserExtension class.
Convenience method.
Definition at line 275 of file navigationextension.cpp.
◆ createNewWindow
|
signal |
Asks the hosting browser to open a new window for the given url
and return a reference to the content part.
◆ enableAction
|
signal |
Enables or disable a standard action held by the browser.
See class documentation for the list of standard actions.
◆ isActionEnabled()
bool NavigationExtension::isActionEnabled | ( | const char * | name | ) | const |
- Returns
- the status (enabled/disabled) of an action. When the enableAction signal is emitted, the browserextension stores the status of the action internally, so that it's possible to query later for the status of the action, using this method.
Definition at line 240 of file navigationextension.cpp.
◆ isURLDropHandlingEnabled()
bool NavigationExtension::isURLDropHandlingEnabled | ( | ) | const |
Returns whether url drop handling is enabled.
See setURLDropHandlingEnabled for more information about this property.
Definition at line 157 of file navigationextension.cpp.
◆ itemsRemoved
|
signal |
Inform the host about items that have been removed.
◆ loadingProgress
|
signal |
Since the part emits the jobid in the started() signal, progress information is automatically displayed.
However, if you don't use a KIO::Job in the part, you can use loadingProgress() and speedProgress() to display progress information.
◆ mouseOverInfo
|
signal |
Inform the hosting application that the user moved the mouse over an item.
Used when the mouse is on an URL.
◆ moveTopLevelWidget
|
signal |
Ask the hosting application to move the top level widget.
◆ openUrlNotify
|
signal |
Tells the hosting browser that the part opened a new URL (which can be queried via KParts::Part::url().
This helps the browser to update/create an entry in the history. The part may not emit this signal together with openUrlRequest(). Emit openUrlRequest() if you want the browser to handle a URL the user asked to open (from within your part/document). This signal however is useful if you want to handle URLs all yourself internally, while still telling the hosting browser about new opened URLs, in order to provide a proper history functionality to the user. An example of usage is a html rendering component which wants to emit this signal when a child frame document changed its URL. Conclusion: you probably want to use openUrlRequest() instead.
◆ openUrlRequest
|
signal |
Asks the host (browser) to open url
.
To set a reload, the x and y offsets, the service type etc., fill in the appropriate fields in the args
structure. Hosts should not connect to this signal but to openUrlRequestDelayed().
◆ openUrlRequestDelayed
|
signal |
This signal is emitted when openUrlRequest() is called, after a 0-seconds timer.
This allows the caller to terminate what it's doing first, before (usually) being destroyed. Parts should never use this signal, hosts should only connect to this signal.
◆ pasteRequest()
void NavigationExtension::pasteRequest | ( | ) |
Asks the hosting browser to perform a paste (using openUrlRequestDelayed())
Definition at line 167 of file navigationextension.cpp.
◆ popupMenu [1/2]
|
signal |
Emit this to make the browser show a standard popup menu for the files items
.
- Parameters
-
global global coordinates where the popup should be shown items list of file items which the popup applies to args OpenUrlArguments, mostly for metadata here flags enables/disables certain builtin actions in the popupmenu actionGroups named groups of actions which should be inserted into the popup, see ActionGroupMap
◆ popupMenu [2/2]
|
signal |
Emit this to make the browser show a standard popup menu for the given url
.
Give as much information about this URL as possible, like args.mimeType
and the file type mode
- Parameters
-
global global coordinates where the popup should be shown url the URL this popup applies to mode the file type of the url (S_IFREG, S_IFDIR...) args OpenUrlArguments, set the mimetype of the URL using setMimeType() flags enables/disables certain builtin actions in the popupmenu actionGroups named groups of actions which should be inserted into the popup, see ActionGroupMap
◆ requestFocus
|
signal |
Ask the hosting application to focus part
.
◆ resizeTopLevelWidget
|
signal |
Ask the hosting application to resize the top level widget.
◆ restoreState()
|
virtual |
Used by the browser to restore the view in the state it was when we left it.
If you saved additional properties, reimplement it but don't forget to call the parent method (probably first).
Definition at line 142 of file navigationextension.cpp.
◆ saveState()
|
virtual |
Used by the browser to save the current state of the view (in order to restore it if going back in navigation).
If you want to save additional properties, reimplement it but don't forget to call the parent method (probably first).
Definition at line 136 of file navigationextension.cpp.
◆ selectionInfo
|
signal |
Inform the hosting application about the current selection.
Used when a set of files/URLs is selected (with full information about those URLs, including size, permissions etc.)
◆ setActionText
|
signal |
Change the text of a standard action held by the browser.
This can be used to change "Paste" into "Paste Image" for instance.
See class documentation for the list of standard actions.
◆ setIconUrl
|
signal |
Sets the URL of an icon for the currently displayed page.
◆ setLocationBarUrl
|
signal |
Updates the URL shown in the browser's location bar to url
.
◆ setPageSecurity
|
signal |
Tell the host (browser) about security state of current page enum PageSecurity { NotCrypted, Encrypted, Mixed };.
◆ setURLDropHandlingEnabled()
void NavigationExtension::setURLDropHandlingEnabled | ( | bool | enable | ) |
Enables or disables url drop handling.
URL drop handling is a property describing whether the hosting shell component is allowed to install an event filter on the part's widget, to listen for URI drop events. Set it to true if you are exporting a BrowserExtension implementation and do not provide any special URI drop handling. If set to false you can be sure to receive all those URI drop events unfiltered. Also note that the implementation as of Konqueror installs the event filter only on the part's widget itself, not on child widgets.
Definition at line 162 of file navigationextension.cpp.
◆ speedProgress
|
signal |
- See also
- loadingProgress
◆ xOffset()
|
virtual |
Returns the current x offset.
For a scrollview, implement this using contentsX().
Definition at line 126 of file navigationextension.cpp.
◆ yOffset()
|
virtual |
Returns the current y offset.
For a scrollview, implement this using contentsY().
Definition at line 131 of file navigationextension.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:36 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.