KParts::ReadOnlyPart
#include <KParts/ReadOnlyPart>
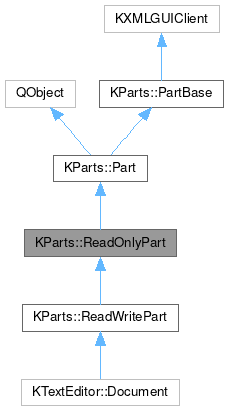
Properties | |
QUrl | url |
![]() | |
objectName | |
Signals | |
void | canceled (const QString &errMsg) |
void | completed () |
void | completedWithPendingAction () |
void | started (KIO::Job *job) |
void | urlChanged (const QUrl &url) |
![]() | |
void | setStatusBarText (const QString &text) |
void | setWindowCaption (const QString &caption) |
Public Slots | |
virtual bool | openUrl (const QUrl &url) |
Public Member Functions | |
ReadOnlyPart (QObject *parent=nullptr, const KPluginMetaData &data={}) | |
~ReadOnlyPart () override | |
OpenUrlArguments | arguments () const |
bool | closeStream () |
virtual bool | closeUrl () |
bool | isProgressInfoEnabled () const |
NavigationExtension * | navigationExtension () const |
bool | openStream (const QString &mimeType, const QUrl &url) |
void | setArguments (const OpenUrlArguments &arguments) |
void | setProgressInfoEnabled (bool show) |
QUrl | url () const |
bool | writeStream (const QByteArray &data) |
![]() | |
Part (QObject *parent=nullptr, const KPluginMetaData &data={}) | |
~Part () override | |
virtual Part * | hitTest (QWidget *widget, const QPoint &globalPos) |
PartManager * | manager () const |
KPluginMetaData | metaData () const |
void | setAutoDeletePart (bool autoDeletePart) |
void | setAutoDeleteWidget (bool autoDeleteWidget) |
virtual void | setManager (PartManager *manager) |
virtual QWidget * | widget () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
PartBase () | |
~PartBase () override | |
QObject * | partObject () const |
void | setPartObject (QObject *object) |
![]() | |
KXMLGUIClient (KXMLGUIClient *parent) | |
virtual QAction * | action (const QDomElement &element) const |
QAction * | action (const QString &name) const |
virtual KActionCollection * | actionCollection () const |
QList< KXMLGUIClient * > | childClients () |
KXMLGUIBuilder * | clientBuilder () const |
virtual QString | componentName () const |
virtual QDomDocument | domDocument () const |
KXMLGUIFactory * | factory () const |
void | insertChildClient (KXMLGUIClient *child) |
KXMLGUIClient * | parentClient () const |
void | plugActionList (const QString &name, const QList< QAction * > &actionList) |
void | reloadXML () |
void | removeChildClient (KXMLGUIClient *child) |
void | replaceXMLFile (const QString &xmlfile, const QString &localxmlfile, bool merge=false) |
void | setClientBuilder (KXMLGUIBuilder *builder) |
void | setFactory (KXMLGUIFactory *factory) |
void | setXMLGUIBuildDocument (const QDomDocument &doc) |
void | unplugActionList (const QString &name) |
virtual QString | xmlFile () const |
QDomDocument | xmlguiBuildDocument () const |
Protected Member Functions | |
KPARTS_NO_EXPORT | ReadOnlyPart (ReadOnlyPartPrivate &dd, QObject *parent) |
void | abortLoad () |
virtual bool | doCloseStream () |
virtual bool | doOpenStream (const QString &mimeType) |
virtual bool | doWriteStream (const QByteArray &data) |
void | guiActivateEvent (GUIActivateEvent *event) override |
QString | localFilePath () const |
virtual bool | openFile () |
void | setLocalFilePath (const QString &localFilePath) |
void | setUrl (const QUrl &url) |
![]() | |
KPARTS_NO_EXPORT | Part (PartPrivate &dd, QObject *parent) |
void | customEvent (QEvent *event) override |
QWidget * | hostContainer (const QString &containerName) |
virtual void | partActivateEvent (PartActivateEvent *event) |
virtual void | setWidget (QWidget *widget) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
KPARTS_NO_EXPORT | PartBase (PartBasePrivate &dd) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentName (const QString &componentName, const QString &componentDisplayName) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QString | findVersionNumber (const QString &xml) |
![]() | |
typedef | QObjectList |
![]() | |
void | slotWidgetDestroyed () |
![]() | |
static QString | standardsXmlFileLocation () |
![]() | |
std::unique_ptr< PartBasePrivate > const | d_ptr |
Detailed Description
Base class for any "viewer" part.
This class takes care of network transparency for you, in the simplest way (downloading to a temporary file, then letting the part load from the temporary file). To use the built-in network transparency, you only need to implement openFile(), not openUrl().
To implement network transparency differently (e.g. for progressive loading, like a web browser does for instance), or to prevent network transparency (but why would you do that?), you can override openUrl().
An application using KParts can use the signals to show feedback while the URL is being loaded.
ReadOnlyPart handles the window caption by setting it to the current URL (set in openUrl(), and each time the part is activated). If you want another caption, set it in openFile() and, if the part might ever be used with a part manager, in guiActivateEvent().
Definition at line 51 of file readonlypart.h.
Property Documentation
◆ url
|
read |
Definition at line 55 of file readonlypart.h.
Constructor & Destructor Documentation
◆ ReadOnlyPart() [1/2]
|
explicit |
Constructor.
See also Part for the setXXX methods to call.
Definition at line 29 of file readonlypart.cpp.
◆ ~ReadOnlyPart()
|
override |
Destructor.
Definition at line 39 of file readonlypart.cpp.
◆ ReadOnlyPart() [2/2]
|
protected |
Definition at line 34 of file readonlypart.cpp.
Member Function Documentation
◆ abortLoad()
|
protected |
Definition at line 200 of file readonlypart.cpp.
◆ arguments()
OpenUrlArguments KParts::ReadOnlyPart::arguments | ( | ) | const |
- Returns
- the arguments that were used to open this URL.
Definition at line 335 of file readonlypart.cpp.
◆ canceled
|
signal |
Emit this if loading is canceled by the user or by an error.
- Parameters
-
errMsg the error message, empty if the user canceled the loading voluntarily.
◆ closeStream()
bool ReadOnlyPart::closeStream | ( | ) |
Terminate the sending of data to the part.
With some data types (text, html...) closeStream might never actually be called, in the case of continuous streams, for instance plain text or HTML data.
Definition at line 318 of file readonlypart.cpp.
◆ closeUrl()
|
virtual |
Called when closing the current URL (for example, a document), for instance when switching to another URL (note that openUrl() calls it automatically in this case).
If the current URL is not fully loaded yet, aborts loading. Deletes the temporary file used when the URL is remote. Resets the current url() to QUrl().
- Returns
- always true, but the return value exists for reimplementations
Reimplemented in KParts::ReadWritePart.
Definition at line 216 of file readonlypart.cpp.
◆ completed
|
signal |
Emit this when you have completed loading data.
Hosting applications will want to know when the process of loading the data is finished, so that they can access the data when everything is loaded.
◆ completedWithPendingAction
|
signal |
This signal is similar to the KParts::ReadOnlyPart::completed()
signal except it is only emitted if there is still a pending action to be executed on a delayed timer.
An example of this is the meta-refresh tags on web pages used to reload/redirect after a certain period of time. This signal is useful if you want to give the user the ability to cancel such pending actions.
- Since
- 5.81
◆ doCloseStream()
|
inlineprotectedvirtual |
This is called by closeStream(), to indicate that all the data has been sent.
Parts should ensure that all of the data is displayed at this point.
- Returns
- whether the data could be displayed correctly.
Definition at line 201 of file readonlypart.h.
◆ doOpenStream()
|
inlineprotectedvirtual |
Called by openStream to initiate sending of data.
Parts which implement progress loading should check the mimeType
parameter, and return true if they can accept a data stream of that type.
Definition at line 180 of file readonlypart.h.
◆ doWriteStream()
|
inlineprotectedvirtual |
Receive some data from the hosting application.
In this method the part should attempt to display the data progressively. With some data types (text, html...) closeStream might never actually be called, in the case of continuous streams. This can't happen with e.g. images.
Definition at line 191 of file readonlypart.h.
◆ guiActivateEvent()
|
overrideprotectedvirtual |
Reimplemented from Part, so that the window caption is set to the current URL (decoded) when the part is activated.
This is the usual behavior in 99% of applications. Reimplement if you don't like it - test for event->activated()!
- Note
- This is done with GUIActivateEvent and not with PartActivateEvent because it's handled by the main window (which gets the event after the PartActivateEvent events have been sent).
Reimplemented from KParts::Part.
Definition at line 286 of file readonlypart.cpp.
◆ isProgressInfoEnabled()
bool ReadOnlyPart::isProgressInfoEnabled | ( | ) | const |
Returns whether the part shows the progress info dialog used by the internal KIO job.
Definition at line 86 of file readonlypart.cpp.
◆ localFilePath()
|
protected |
Returns the local file path associated with this part.
- Note
- The result will only be valid if openUrl() or setLocalFilePath() has previously been called.
Definition at line 65 of file readonlypart.cpp.
◆ navigationExtension()
NavigationExtension * ReadOnlyPart::navigationExtension | ( | ) | const |
This convenience method returns the NavigationExtension for this part, or nullptr
if there isn't one.
Definition at line 323 of file readonlypart.cpp.
◆ openFile()
|
protectedvirtual |
If the part uses the standard implementation of openUrl(), it must reimplement this to open the local file.
The default implementation simply returns false.
If this method returns true the part emits completed(), otherwise it emits canceled().
- See also
- completed(), canceled()
Definition at line 134 of file readonlypart.cpp.
◆ openStream()
Initiate sending data to this part.
This is an alternative to openUrl(), which allows the user of the part to load the data itself, and send it progressively to the part.
- Parameters
-
mimeType the type of data that is going to be sent to this part. url the URL representing this data. Although not directly used, every ReadOnlyPart has a URL (see url()), so this simply sets it.
- Returns
- true if the part supports progressive loading and accepts data, false otherwise.
Definition at line 300 of file readonlypart.cpp.
◆ openUrl
|
virtualslot |
Only reimplement this if you don't want the network transparency support to download from the URL into a temporary file (when the URL isn't local).
Otherwise, reimplement openFile() only.
If you reimplement it, don't forget to set the caption, usually with
and also, if the URL refers to a local file, resolve it to a local path and call setLocalFilePath().
Definition at line 93 of file readonlypart.cpp.
◆ setArguments()
void KParts::ReadOnlyPart::setArguments | ( | const OpenUrlArguments & | arguments | ) |
Sets the arguments to use for the next openUrl() call.
Definition at line 328 of file readonlypart.cpp.
◆ setLocalFilePath()
|
protected |
Sets the local file path associated with this part.
Definition at line 72 of file readonlypart.cpp.
◆ setProgressInfoEnabled()
void ReadOnlyPart::setProgressInfoEnabled | ( | bool | show | ) |
Call this to turn off the progress info dialog used by the internal KIO job.
Use this if you provide another way of displaying progress info (e.g. a statusbar), using the signals emitted by this class, and/or those emitted by the job given by started().
Definition at line 79 of file readonlypart.cpp.
◆ setUrl()
|
protected |
Sets the URL associated with this part.
Definition at line 53 of file readonlypart.cpp.
◆ started
|
signal |
The part emits this when starting to load data.
If using a KIO::Job, it provides the job
so that progress information can be shown. Otherwise, job
is nullptr
.
◆ url()
QUrl ReadOnlyPart::url | ( | ) | const |
Returns the URL currently opened in (or being opened by) this part.
- Note
- The URL is not cleared if openUrl() fails to load the URL. Call closeUrl() if you need to explicitly reset it.
- Returns
- The current URL.
Definition at line 46 of file readonlypart.cpp.
◆ urlChanged
|
signal |
Emitted by the part when url() changes.
- Since
- 4.10
◆ writeStream()
bool ReadOnlyPart::writeStream | ( | const QByteArray & | data | ) |
Send some data to the part.
openStream must have been called previously, and must have returned true.
- Returns
- true if the data was accepted by the part. If false is returned, the application should stop sending data, and doesn't have to call closeStream.
Definition at line 313 of file readonlypart.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Apr 27 2024 22:09:40 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.