KTextEditor::DocumentPrivate
#include <katedocument.h>
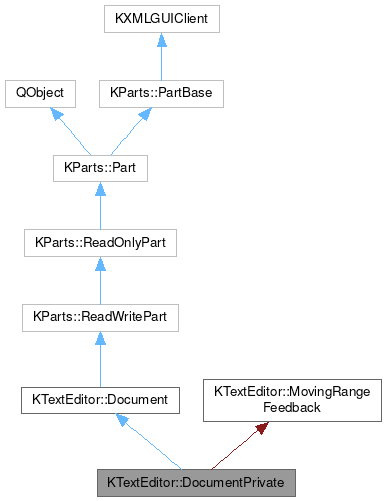
Public Types | |
enum | CommentType { UnComment = -1 , ToggleComment = 0 , Comment = 1 } |
enum | EditingPositionKind { Previous , Next } |
enum | NewLineIndent { Indent , NoIndent } |
enum | NewLinePos { Normal , Above , Below } |
typedef QList< QPair< int, int > > | OffsetList |
enum | TextTransform { Uppercase , Lowercase , Capitalize } |
![]() | |
enum | ModifiedOnDiskReason { OnDiskUnmodified = 0 , OnDiskModified = 1 , OnDiskCreated = 2 , OnDiskDeleted = 3 } |
enum | MarkTypes { markType01 = 0x1 , markType02 = 0x2 , markType03 = 0x4 , markType04 = 0x8 , markType05 = 0x10 , markType06 = 0x20 , markType07 = 0x40 , markType08 = 0x80 , markType09 = 0x100 , markType10 = 0x200 , markType11 = 0x400 , markType12 = 0x800 , markType13 = 0x1000 , markType14 = 0x2000 , markType15 = 0x4000 , markType16 = 0x8000 , markType17 = 0x10000 , markType18 = 0x20000 , markType19 = 0x40000 , markType20 = 0x80000 , markType21 = 0x100000 , markType22 = 0x200000 , markType23 = 0x400000 , markType24 = 0x800000 , markType25 = 0x1000000 , markType26 = 0x2000000 , markType27 = 0x4000000 , markType28 = 0x8000000 , markType29 = 0x10000000 , markType30 = 0x20000000 , markType31 = 0x40000000 , markType32 = 0x80000000 , Bookmark = markType01 , BreakpointActive = markType02 , BreakpointReached = markType03 , BreakpointDisabled = markType04 , Execution = markType05 , Warning = markType06 , Error = markType07 , SearchMatch = markType32 } |
enum | MarkChangeAction { MarkAdded = 0 , MarkRemoved = 1 } |
Public Slots | |
void | addMark (int line, uint markType) override |
bool | clear () override |
void | clearDictionaryRanges () |
void | clearEditingPosStack () |
void | clearMark (int line) override |
void | clearMarks () override |
bool | documentReload () override |
bool | documentSave () override |
bool | documentSaveAs () override |
bool | documentSaveAsWithEncoding (const QString &encoding) |
void | documentSaveCopyAs () |
bool | handleMarkClick (int line) |
bool | handleMarkContextMenu (int line, QPoint position) |
bool | insertLine (int line, const QString &s) override |
bool | insertLines (int line, const QStringList &s) override |
bool | insertText (KTextEditor::Cursor position, const QString &s, bool block=false) override |
bool | insertText (KTextEditor::Cursor position, const QStringList &text, bool block=false) override |
void | messageDestroyed (KTextEditor::Message *message) |
void | onTheFlySpellCheckingEnabled (bool enable) |
void | openWithLineLengthLimitOverride () |
bool | print () override |
void | printPreview () override |
void | redo () |
void | refreshOnTheFlyCheck (KTextEditor::Range range=KTextEditor::Range::invalid()) |
bool | removeLine (int line) override |
void | removeMark (int line, uint markType) override |
bool | removeText (KTextEditor::Range range, bool block=false) override |
bool | replaceText (KTextEditor::Range r, const QStringList &l, bool b) override |
bool | replaceText (KTextEditor::Range range, const QString &s, bool block=false) override |
void | requestMarkTooltip (int line, QPoint position) |
bool | save () override |
void | saveEditingPositions (const KTextEditor::Cursor cursor) |
void | setDefaultDictionary (const QString &dict) |
void | setDictionary (const QString &dict, KTextEditor::Range range) |
void | setDictionary (const QString &dict, KTextEditor::Range range, bool blockmode) |
void | setEditableMarks (uint markMask) override |
void | setMark (int line, uint markType) override |
void | setMarkDescription (Document::MarkTypes, const QString &) override |
void | setMarkIcon (Document::MarkTypes markType, const QIcon &icon) override |
void | setPageUpDownMovesCursor (bool on) |
bool | setText (const QString &) override |
bool | setText (const QStringList &text) override |
void | setWordWrap (bool on) |
void | setWordWrapAt (uint col) |
virtual void | slotModifiedOnDisk (KTextEditor::View *v=nullptr) |
void | slotQueryClose_save (bool *handled, bool *abortClosing) |
void | tagLine (int line) |
void | tagLines (KTextEditor::LineRange lineRange) |
void | undo () |
![]() | |
virtual bool | save () |
void | setModified () |
bool | waitSaveComplete () |
Public Member Functions | |
DocumentPrivate (bool bSingleViewMode=false, bool bReadOnly=false, QWidget *parentWidget=nullptr, QObject *=nullptr) | |
DocumentPrivate (const KPluginMetaData &data, bool bSingleViewMode=false, bool bReadOnly=false, QWidget *parentWidget=nullptr, QObject *=nullptr) | |
KTextEditor::View * | activeView () const |
void | addView (KTextEditor::View *) |
void | align (KTextEditor::ViewPrivate *view, KTextEditor::Range range) |
void | alignOn (KTextEditor::Range range, const QString &pattern, bool blockwise) |
KTextEditor::AnnotationModel * | annotationModel () const override |
KToggleAction * | autoReloadToggleAction () |
void | backspace (KTextEditor::ViewPrivate *view) |
void | bomSetByUser () |
KateBuffer & | buffer () |
void | bufferHlChanged () |
QChar | characterAt (KTextEditor::Cursor position) const override |
QByteArray | checksum () const override |
void | clearMisspellingForWord (const QString &word) |
bool | closeUrl () override |
void | comment (KTextEditor::ViewPrivate *view, uint line, uint column, CommentType change) |
KateDocumentConfig * | config () |
KateDocumentConfig * | config () const |
QStringList | configKeys () const override |
QVariant | configValue (const QString &key) override |
bool | containsCharacterEncoding (KTextEditor::Range range) |
KTextEditor::View * | createView (QWidget *parent, KTextEditor::MainWindow *mainWindow=nullptr) override |
qsizetype | cursorToOffset (KTextEditor::Cursor c) const override |
QString | decodeCharacters (KTextEditor::Range range, KTextEditor::DocumentPrivate::OffsetList &decToEncOffsetList, KTextEditor::DocumentPrivate::OffsetList &encToDecOffsetList) |
QString | defaultDictionary () const |
KSyntaxHighlighting::Theme::TextStyle | defaultStyleAt (KTextEditor::Cursor position) const override |
KSyntaxHighlighting::Theme::TextStyle | defStyleNum (int line, int column) |
void | del (KTextEditor::ViewPrivate *view, const KTextEditor::Cursor) |
void | delayAutoReload () |
QString | dictionaryForMisspelledRange (KTextEditor::Range range) const |
QList< QPair< KTextEditor::MovingRange *, QString > > | dictionaryRanges () const |
void | discardDataRecovery () override |
KTextEditor::Cursor | documentEnd () const override |
QString | documentName () const override |
uint | editableMarks () const override |
bool | editEnd () |
bool | editInsertLine (int line, const QString &s, bool notify=true) |
bool | editInsertText (int line, int col, const QString &s, bool notify=true) |
bool | editMarkLineAutoWrapped (int line, bool autowrapped) |
bool | editRemoveLine (int line) |
bool | editRemoveLines (int from, int to) |
bool | editRemoveText (int line, int col, int len) |
bool | editStart () |
bool | editUnWrapLine (int line, bool removeLine=true, int length=0) |
bool | editWrapLine (int line, int col, bool newLine=true, bool *newLineAdded=nullptr, bool notify=true) |
QStringList | embeddedHighlightingModes () const override |
QString | encoding () const override |
QString | fileType () const |
KTextEditor::Range | findMatchingBracket (const KTextEditor::Cursor start, int maxLines) |
int | findTouchedLine (int startLine, bool down) |
int | fromVirtualColumn (const KTextEditor::Cursor) const |
int | fromVirtualColumn (int line, int column) const |
KateHighlighting * | highlight () const |
QString | highlightingMode () const override |
QString | highlightingModeAt (KTextEditor::Cursor position) override |
QStringList | highlightingModes () const override |
QString | highlightingModeSection (int index) const override |
void | indent (KTextEditor::Range range, int change) |
void | inputMethodEnd () |
void | inputMethodStart () |
void | insertTab (KTextEditor::ViewPrivate *view, const KTextEditor::Cursor) |
bool | isAutoReload () |
bool | isComment (int line, int column) |
bool | isDataRecoveryAvailable () const override |
bool | isEditingTransactionRunning () const override |
bool | isEditRunning () const |
bool | isLineModified (int line) const override |
bool | isLineSaved (int line) const override |
bool | isLineTouched (int line) const override |
bool | isModifiedOnDisc () |
bool | isOnTheFlySpellCheckingEnabled () const |
bool | isValidTextPosition (KTextEditor::Cursor cursor) const override |
void | joinLines (uint first, uint last) |
Kate::TextLine | kateTextLine (int i) |
KTextEditor::Cursor | lastEditingPosition (EditingPositionKind nextOrPrevious, KTextEditor::Cursor) |
int | lastLine () const |
qint64 | lastSavedRevision () const override |
QString | line (int line) const override |
int | lineLength (int line) const override |
int | lineLengthLimit () const |
int | lines () const override |
void | lockRevision (qint64 revision) override |
uint | mark (int line) override |
virtual QColor | markColor (Document::MarkTypes) const |
QString | markDescription (Document::MarkTypes) const override |
QIcon | markIcon (Document::MarkTypes markType) const override |
const QHash< int, KTextEditor::Mark * > & | marks () override |
QString | mimeType () override |
QString | mode () const override |
QStringList | modes () const override |
QString | modeSection (int index) const override |
bool | multiPaste (KTextEditor::ViewPrivate *view, const QStringList &texts) |
void | newLine (KTextEditor::ViewPrivate *view, NewLineIndent indent=NewLineIndent::Indent, NewLinePos newLinePos=Normal) |
KTextEditor::MovingCursor * | newMovingCursor (KTextEditor::Cursor position, KTextEditor::MovingCursor::InsertBehavior insertBehavior=KTextEditor::MovingCursor::MoveOnInsert) override |
KTextEditor::MovingRange * | newMovingRange (KTextEditor::Range range, KTextEditor::MovingRange::InsertBehaviors insertBehaviors=KTextEditor::MovingRange::DoNotExpand, KTextEditor::MovingRange::EmptyBehavior emptyBehavior=KTextEditor::MovingRange::AllowEmpty) override |
KTextEditor::Cursor | offsetToCursor (qsizetype offset) const override |
KateOnTheFlyChecker * | onTheFlySpellChecker () const |
bool | openFile () override |
bool | openUrl (const QUrl &url) override |
bool | ownedView (KTextEditor::ViewPrivate *) |
bool | pageUpDownMovesCursor () const |
void | paste (KTextEditor::ViewPrivate *view, const QString &text) |
Kate::TextLine | plainKateTextLine (int i) |
void | popEditState () |
bool | postMessage (KTextEditor::Message *message) override |
void | pushEditState () |
bool | queryClose () override |
KTextEditor::Range | rangeOnLine (KTextEditor::Range range, int line) const |
bool | readOnly () const |
void | readSessionConfig (const KConfigGroup &config, const QSet< QString > &flags=QSet< QString >()) override |
void | recoverData () override |
uint | redoCount () const |
void | rememberUserDidSetIndentationMode () |
void | removeAllTrailingSpaces () |
void | removeView (KTextEditor::View *) |
void | repaintViews (bool paintOnlyDirty=true) |
void | replaceCharactersByEncoding (KTextEditor::Range range) |
qint64 | revision () const override |
bool | saveAs (const QUrl &url) override |
bool | saveFile () override |
QList< KTextEditor::Range > | searchText (KTextEditor::Range range, const QString &pattern, const KTextEditor::SearchOptions options) const |
void | setActiveTemplateHandler (KateTemplateHandler *handler) |
void | setActiveView (KTextEditor::View *) |
void | setAnnotationModel (KTextEditor::AnnotationModel *model) override |
void | setConfigValue (const QString &key, const QVariant &value) override |
void | setDontChangeHlOnSave () |
bool | setEncoding (const QString &e) override |
bool | setHighlightingMode (const QString &name) override |
bool | setMode (const QString &name) override |
void | setModified (bool m) override |
void | setModifiedOnDisk (ModifiedOnDiskReason reason) override |
void | setModifiedOnDiskWarning (bool on) override |
void | setReadWrite (bool rw=true) override |
void | setUndoMergeAllEdits (bool merge) |
virtual void | setVariable (const QString &name, const QString &value) |
bool | singleViewMode () const |
QUrl | startUrlForFileDialog () |
Kate::SwapFile * | swapFile () |
void | swapTextRanges (KTextEditor::Range firstWord, KTextEditor::Range secondWord) |
QString | text () const override |
QString | text (KTextEditor::Range range, bool blockwise=false) const override |
QStringList | textLines (KTextEditor::Range range, bool block=false) const override |
qsizetype | totalCharacters () const override |
int | toVirtualColumn (const KTextEditor::Cursor) const |
int | toVirtualColumn (int line, int column) const |
void | transform (KTextEditor::ViewPrivate *view, KTextEditor::Cursor, TextTransform) |
void | transformCursor (int &line, int &column, KTextEditor::MovingCursor::InsertBehavior insertBehavior, qint64 fromRevision, qint64 toRevision=-1) override |
void | transformCursor (KTextEditor::Cursor &cursor, KTextEditor::MovingCursor::InsertBehavior insertBehavior, qint64 fromRevision, qint64 toRevision=-1) override |
void | transformRange (KTextEditor::Range &range, KTextEditor::MovingRange::InsertBehaviors insertBehaviors, KTextEditor::MovingRange::EmptyBehavior emptyBehavior, qint64 fromRevision, qint64 toRevision=-1) override |
void | transpose (const KTextEditor::Cursor) |
void | typeChars (KTextEditor::ViewPrivate *view, QString chars) |
uint | undoCount () const |
KateUndoManager * | undoManager () |
void | unlockRevision (qint64 revision) override |
void | updateConfig () |
bool | updateFileType (const QString &newType, bool user=false) |
void | userSetEncodingForNextReload () |
virtual QString | variable (const QString &name) const |
QList< KTextEditor::View * > | views () const override |
QWidget * | widget () override |
QString | wordAt (KTextEditor::Cursor cursor) const override |
KTextEditor::Range | wordRangeAt (KTextEditor::Cursor cursor) const override |
bool | wordWrap () const |
uint | wordWrapAt () const |
bool | wrapParagraph (int first, int last) |
bool | wrapText (int startLine, int endLine) |
void | writeSessionConfig (KConfigGroup &config, const QSet< QString > &flags=QSet< QString >()) override |
![]() | |
~Document () override | |
virtual bool | print ()=0 |
virtual void | printPreview ()=0 |
virtual void | setMark (int line, uint markType)=0 |
virtual void | clearMark (int line)=0 |
virtual void | addMark (int line, uint markType)=0 |
virtual void | removeMark (int line, uint markType)=0 |
virtual void | clearMarks ()=0 |
virtual void | setMarkDescription (MarkTypes mark, const QString &text)=0 |
virtual void | setEditableMarks (uint markMask)=0 |
virtual void | setMarkIcon (MarkTypes markType, const QIcon &icon)=0 |
virtual bool | documentReload ()=0 |
virtual bool | documentSave ()=0 |
virtual bool | documentSaveAs ()=0 |
bool | openingError () const |
Range | documentRange () const |
virtual bool | isEmpty () const |
Cursor | endOfLine (int line) const |
virtual bool | setText (const QString &text)=0 |
virtual bool | setText (const QStringList &text)=0 |
virtual bool | clear ()=0 |
virtual bool | insertText (KTextEditor::Cursor position, const QString &text, bool block=false)=0 |
virtual bool | insertText (KTextEditor::Cursor position, const QStringList &text, bool block=false)=0 |
virtual bool | replaceText (Range range, const QString &text, bool block=false) |
virtual bool | replaceText (Range range, const QStringList &text, bool block=false) |
virtual bool | removeText (Range range, bool block=false)=0 |
virtual bool | insertLine (int line, const QString &text)=0 |
virtual bool | insertLines (int line, const QStringList &text)=0 |
virtual bool | removeLine (int line)=0 |
QList< KTextEditor::Range > | searchText (KTextEditor::Range range, const QString &pattern, const SearchOptions options=Default) const |
![]() | |
ReadWritePart (QObject *parent=nullptr, const KPluginMetaData &data={}) | |
bool | closeUrl () override |
virtual bool | closeUrl (bool promptToSave) |
bool | isModified () const |
bool | isReadWrite () const |
![]() | |
ReadOnlyPart (QObject *parent=nullptr, const KPluginMetaData &data={}) | |
OpenUrlArguments | arguments () const |
bool | closeStream () |
bool | isProgressInfoEnabled () const |
NavigationExtension * | navigationExtension () const |
bool | openStream (const QString &mimeType, const QUrl &url) |
void | setArguments (const OpenUrlArguments &arguments) |
void | setProgressInfoEnabled (bool show) |
QUrl | url () const |
bool | writeStream (const QByteArray &data) |
![]() | |
Part (QObject *parent=nullptr, const KPluginMetaData &data={}) | |
virtual Part * | hitTest (QWidget *widget, const QPoint &globalPos) |
PartManager * | manager () const |
KPluginMetaData | metaData () const |
void | setAutoDeletePart (bool autoDeletePart) |
void | setAutoDeleteWidget (bool autoDeleteWidget) |
virtual void | setManager (PartManager *manager) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
void | setPartObject (QObject *object) |
![]() | |
KXMLGUIClient (KXMLGUIClient *parent) | |
virtual QAction * | action (const QDomElement &element) const |
QAction * | action (const QString &name) const |
virtual KActionCollection * | actionCollection () const |
QList< KXMLGUIClient * > | childClients () |
KXMLGUIBuilder * | clientBuilder () const |
virtual QString | componentName () const |
virtual QDomDocument | domDocument () const |
KXMLGUIFactory * | factory () const |
void | insertChildClient (KXMLGUIClient *child) |
KXMLGUIClient * | parentClient () const |
void | plugActionList (const QString &name, const QList< QAction * > &actionList) |
void | reloadXML () |
void | removeChildClient (KXMLGUIClient *child) |
void | replaceXMLFile (const QString &xmlfile, const QString &localxmlfile, bool merge=false) |
void | setClientBuilder (KXMLGUIBuilder *builder) |
void | setFactory (KXMLGUIFactory *factory) |
void | setXMLGUIBuildDocument (const QDomDocument &doc) |
void | unplugActionList (const QString &name) |
virtual QString | xmlFile () const |
QDomDocument | xmlguiBuildDocument () const |
Static Public Member Functions | |
static int | computePositionWrtOffsets (const OffsetList &offsetList, int pos) |
static int | reservedMarkersCount () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QString | findVersionNumber (const QString &xml) |
Protected Member Functions | |
void | deleteDictionaryRange (KTextEditor::MovingRange *movingRange) |
void | rangeEmpty (KTextEditor::MovingRange *movingRange) override |
void | rangeInvalid (KTextEditor::MovingRange *movingRange) override |
![]() | |
Document (DocumentPrivate *impl, const KPluginMetaData &data, QObject *parent) | |
![]() | |
virtual bool | saveToUrl () |
![]() | |
void | abortLoad () |
virtual bool | doCloseStream () |
virtual bool | doOpenStream (const QString &mimeType) |
virtual bool | doWriteStream (const QByteArray &data) |
void | guiActivateEvent (GUIActivateEvent *event) override |
QString | localFilePath () const |
void | setLocalFilePath (const QString &localFilePath) |
void | setUrl (const QUrl &url) |
![]() | |
void | customEvent (QEvent *event) override |
QWidget * | hostContainer (const QString &containerName) |
virtual void | partActivateEvent (PartActivateEvent *event) |
virtual void | setWidget (QWidget *widget) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentName (const QString &componentName, const QString &componentDisplayName) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
Protected Attributes | |
QString | m_defaultDictionary |
QList< QPair< KTextEditor::MovingRange *, QString > > | m_dictionaryRanges |
KateOnTheFlyChecker * | m_onTheFlyChecker = nullptr |
KateUndoManager *const | m_undoManager |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
typedef | QObjectList |
![]() | |
void | slotWidgetDestroyed () |
![]() | |
static QString | standardsXmlFileLocation () |
Detailed Description
Backend of KTextEditor::Document related public KTextEditor interfaces.
- Warning
- This file is private API and not part of the public KTextEditor interfaces.
Definition at line 67 of file katedocument.h.
Member Typedef Documentation
◆ OffsetList
typedef QList<QPair<int, int> > KTextEditor::DocumentPrivate::OffsetList |
Definition at line 1310 of file katedocument.h.
Member Enumeration Documentation
◆ CommentType
enum KTextEditor::DocumentPrivate::CommentType |
Definition at line 814 of file katedocument.h.
◆ EditingPositionKind
enum KTextEditor::DocumentPrivate::EditingPositionKind |
Definition at line 335 of file katedocument.h.
◆ NewLineIndent
enum KTextEditor::DocumentPrivate::NewLineIndent |
Definition at line 795 of file katedocument.h.
◆ NewLinePos
enum KTextEditor::DocumentPrivate::NewLinePos |
Definition at line 799 of file katedocument.h.
◆ TextTransform
enum KTextEditor::DocumentPrivate::TextTransform |
Definition at line 836 of file katedocument.h.
Constructor & Destructor Documentation
◆ DocumentPrivate() [1/2]
|
explicit |
Definition at line 164 of file katedocument.cpp.
◆ DocumentPrivate() [2/2]
|
inlineexplicit |
Definition at line 81 of file katedocument.h.
◆ ~DocumentPrivate()
|
override |
Definition at line 271 of file katedocument.cpp.
Member Function Documentation
◆ activeView()
|
inline |
Definition at line 132 of file katedocument.h.
◆ addMark
|
overrideslot |
Definition at line 2015 of file katedocument.cpp.
◆ addView()
void KTextEditor::DocumentPrivate::addView | ( | KTextEditor::View * | view | ) |
Definition at line 2949 of file katedocument.cpp.
◆ align()
void KTextEditor::DocumentPrivate::align | ( | KTextEditor::ViewPrivate * | view, |
KTextEditor::Range | range ) |
Definition at line 3699 of file katedocument.cpp.
◆ alignOn()
void KTextEditor::DocumentPrivate::alignOn | ( | KTextEditor::Range | range, |
const QString & | pattern, | ||
bool | blockwise ) |
Definition at line 3704 of file katedocument.cpp.
◆ annotationModel()
|
overridevirtual |
returns the currently set AnnotationModel or 0 if there's none set
- Returns
- the current AnnotationModel
Implements KTextEditor::Document.
Definition at line 5902 of file katedocument.cpp.
◆ autoReloadToggleAction()
|
inline |
Definition at line 723 of file katedocument.h.
◆ backspace()
void KTextEditor::DocumentPrivate::backspace | ( | KTextEditor::ViewPrivate * | view | ) |
Definition at line 3498 of file katedocument.cpp.
◆ bomSetByUser()
void KTextEditor::DocumentPrivate::bomSetByUser | ( | ) |
Set that the BOM marker is forced via the tool menu.
Definition at line 1866 of file katedocument.cpp.
◆ buffer()
|
inline |
Get access to buffer of this document.
Is needed to create cursors and ranges for example.
- Returns
- document buffer
Definition at line 1099 of file katedocument.h.
◆ bufferHlChanged()
void KTextEditor::DocumentPrivate::bufferHlChanged | ( | ) |
Definition at line 1850 of file katedocument.cpp.
◆ characterAt()
|
overridevirtual |
Get the character at text position cursor
.
- Parameters
-
position the location of the character to retrieve
- Returns
- the requested character, or QChar() for invalid cursors.
- See also
- setText()
Implements KTextEditor::Document.
Definition at line 483 of file katedocument.cpp.
◆ checksum()
|
overridevirtual |
Returns a git compatible sha1 checksum of this document on disk.
- Returns
- checksum for this document on disk
Implements KTextEditor::Document.
Definition at line 5542 of file katedocument.cpp.
◆ clear
|
overrideslot |
Definition at line 660 of file katedocument.cpp.
◆ clearDictionaryRanges
|
slot |
Definition at line 6145 of file katedocument.cpp.
◆ clearEditingPosStack
|
slot |
Removes all the elements in m_editingStack of the respective document.
Definition at line 373 of file katedocument.cpp.
◆ clearMark
|
overrideslot |
Definition at line 2000 of file katedocument.cpp.
◆ clearMarks
|
overrideslot |
work on a copy as deletions below might trigger the use of m_marks
Definition at line 2135 of file katedocument.cpp.
◆ clearMisspellingForWord()
void KTextEditor::DocumentPrivate::clearMisspellingForWord | ( | const QString & | word | ) |
Definition at line 6281 of file katedocument.cpp.
◆ closeUrl()
|
overridevirtual |
Reimplemented from KParts::ReadOnlyPart.
Definition at line 2776 of file katedocument.cpp.
◆ comment()
void KTextEditor::DocumentPrivate::comment | ( | KTextEditor::ViewPrivate * | view, |
uint | line, | ||
uint | column, | ||
CommentType | change ) |
Definition at line 4249 of file katedocument.cpp.
◆ computePositionWrtOffsets()
|
static |
Definition at line 6348 of file katedocument.cpp.
◆ config() [1/2]
|
inline |
Configuration.
Definition at line 1169 of file katedocument.h.
◆ config() [2/2]
|
inline |
Definition at line 1173 of file katedocument.h.
◆ configKeys()
|
overridevirtual |
Get a list of all available keys.
Implements KTextEditor::Document.
Definition at line 5762 of file katedocument.cpp.
◆ configValue()
Get a value for the key
.
Implements KTextEditor::Document.
Definition at line 5768 of file katedocument.cpp.
◆ containsCharacterEncoding()
bool KTextEditor::DocumentPrivate::containsCharacterEncoding | ( | KTextEditor::Range | range | ) |
Definition at line 6323 of file katedocument.cpp.
◆ createView()
|
overridevirtual |
Create a new view attached to parent
.
- Parameters
-
parent parent widget mainWindow the main window responsible for this view, if any
- Returns
- the new view
Implements KTextEditor::Document.
Definition at line 402 of file katedocument.cpp.
◆ cursorToOffset()
|
overridevirtual |
Retrives the offset for the given cursor position NOTE: It will return -1 if the cursor was invalid or out of bounds.
- Since
- 6.0
Implements KTextEditor::Document.
Definition at line 926 of file katedocument.cpp.
◆ decodeCharacters()
QString KTextEditor::DocumentPrivate::decodeCharacters | ( | KTextEditor::Range | range, |
KTextEditor::DocumentPrivate::OffsetList & | decToEncOffsetList, | ||
KTextEditor::DocumentPrivate::OffsetList & | encToDecOffsetList ) |
The first OffsetList is from decoded to encoded, and the second OffsetList from encoded to decoded.
Definition at line 6360 of file katedocument.cpp.
◆ defaultDictionary()
QString KTextEditor::DocumentPrivate::defaultDictionary | ( | ) | const |
Definition at line 6135 of file katedocument.cpp.
◆ defaultStyleAt()
|
overridevirtual |
Get the default style of the character located at position
.
If position
is not a valid text position, the default style KSyntaxHighlighting::Theme::TextStyle::Normal is returned.
- Note
- Further information about the colors of default styles depend on the currently chosen schema. Since each View may have a different color schema, the color information can be obtained through View::defaultStyleAttribute() and View::lineAttributes().
- Parameters
-
position text position
- Returns
- default style, see enum KSyntaxHighlighting::Theme::TextStyle
Implements KTextEditor::Document.
Definition at line 1791 of file katedocument.cpp.
◆ defStyleNum()
KSyntaxHighlighting::Theme::TextStyle KTextEditor::DocumentPrivate::defStyleNum | ( | int | line, |
int | column ) |
- Returns
-1
ifline
orcolumn
invalid, otherwise one of standard style attribute number
Definition at line 6469 of file katedocument.cpp.
◆ del()
void KTextEditor::DocumentPrivate::del | ( | KTextEditor::ViewPrivate * | view, |
const KTextEditor::Cursor | c ) |
Definition at line 3549 of file katedocument.cpp.
◆ delayAutoReload()
void KTextEditor::DocumentPrivate::delayAutoReload | ( | ) |
Definition at line 4777 of file katedocument.cpp.
◆ deleteDictionaryRange()
|
protected |
Definition at line 6305 of file katedocument.cpp.
◆ dictionaryForMisspelledRange()
QString KTextEditor::DocumentPrivate::dictionaryForMisspelledRange | ( | KTextEditor::Range | range | ) | const |
Definition at line 6272 of file katedocument.cpp.
◆ dictionaryRanges()
QList< QPair< KTextEditor::MovingRange *, QString > > KTextEditor::DocumentPrivate::dictionaryRanges | ( | ) | const |
Definition at line 6140 of file katedocument.cpp.
◆ discardDataRecovery()
|
overridevirtual |
If recover data is available, calling discardDataRecovery() will discard the recover data and the recover data is lost.
If isDataRecoveryAvailable() returns false, calling this function does nothing.
- See also
- isDataRecoveryAvailable(), recoverData()
Implements KTextEditor::Document.
Definition at line 2884 of file katedocument.cpp.
◆ documentEnd()
|
overridevirtual |
End position of the document.
- Returns
- The last column on the last line of the document
- See also
- all()
Implements KTextEditor::Document.
Definition at line 5782 of file katedocument.cpp.
◆ documentName()
|
inlineoverridevirtual |
Get this document's name.
The editor part should provide some meaningful name, like some unique "Untitled XYZ" for the document - without URL or basename for documents with url.
- Returns
- readable document name
Implements KTextEditor::Document.
Definition at line 953 of file katedocument.h.
◆ documentReload
|
overrideslot |
Reloads the current document from disk if possible.
Definition at line 4835 of file katedocument.cpp.
◆ documentSave
|
overrideslot |
Definition at line 4929 of file katedocument.cpp.
◆ documentSaveAs
|
overrideslot |
Definition at line 4938 of file katedocument.cpp.
◆ documentSaveAsWithEncoding
|
slot |
Definition at line 4948 of file katedocument.cpp.
◆ documentSaveCopyAs
|
slot |
Definition at line 4959 of file katedocument.cpp.
◆ editableMarks()
|
overridevirtual |
Get, which marks can be toggled by the user.
The returned value is a mark mask containing all editable marks combined with a logical OR.
- Returns
- mark mask containing all editable marks
- See also
- setEditableMarks()
Implements KTextEditor::Document.
Definition at line 2179 of file katedocument.cpp.
◆ editEnd()
bool KTextEditor::DocumentPrivate::editEnd | ( | ) |
◆ editInsertLine()
bool KTextEditor::DocumentPrivate::editInsertLine | ( | int | line, |
const QString & | s, | ||
bool | notify = true ) |
Insert a string at the given line.
- Parameters
-
line line number s string to insert
- Returns
- true on success
Definition at line 1517 of file katedocument.cpp.
◆ editInsertText()
bool KTextEditor::DocumentPrivate::editInsertText | ( | int | line, |
int | col, | ||
const QString & | s, | ||
bool | notify = true ) |
Add a string in the given line/column.
- Parameters
-
line line number col column s string to be inserted
- Returns
- true on success
Definition at line 1257 of file katedocument.cpp.
◆ editLineUnWrapped
|
signal |
Emitted each time text from nextLine
was upwrapped onto line
.
◆ editLineWrapped
|
signal |
Emitted when text from line
was wrapped at position pos onto line nextLine
.
◆ editMarkLineAutoWrapped()
bool KTextEditor::DocumentPrivate::editMarkLineAutoWrapped | ( | int | line, |
bool | autowrapped ) |
Mark line
as autowrapped
.
This is necessary if static word warp is enabled, because we have to know whether to insert a new line or add the wrapped words to the following line.
- Parameters
-
line line number autowrapped autowrapped?
- Returns
- true on success
Definition at line 1353 of file katedocument.cpp.
◆ editRemoveLine()
bool KTextEditor::DocumentPrivate::editRemoveLine | ( | int | line | ) |
Remove a line.
- Parameters
-
line line number
- Returns
- true on success
Definition at line 1590 of file katedocument.cpp.
◆ editRemoveLines()
bool KTextEditor::DocumentPrivate::editRemoveLines | ( | int | from, |
int | to ) |
Definition at line 1595 of file katedocument.cpp.
◆ editRemoveText()
bool KTextEditor::DocumentPrivate::editRemoveText | ( | int | line, |
int | col, | ||
int | len ) |
Remove a string in the given line/column.
- Parameters
-
line line number col column len length of text to be removed
- Returns
- true on success
Definition at line 1306 of file katedocument.cpp.
◆ editStart()
bool KTextEditor::DocumentPrivate::editStart | ( | ) |
Enclose editor actions with editStart()
and editEnd()
to group them.
Definition at line 971 of file katedocument.cpp.
◆ editUnWrapLine()
bool KTextEditor::DocumentPrivate::editUnWrapLine | ( | int | line, |
bool | removeLine = true, | ||
int | length = 0 ) |
Unwrap line
.
If removeLine
is true, we force to join the lines. If removeLine
is true, length
is ignored (eg not needed).
- Parameters
-
line line number removeLine if true, force to remove the next line
- Returns
- true on success
Definition at line 1451 of file katedocument.cpp.
◆ editWrapLine()
bool KTextEditor::DocumentPrivate::editWrapLine | ( | int | line, |
int | col, | ||
bool | newLine = true, | ||
bool * | newLineAdded = nullptr, | ||
bool | notify = true ) |
Wrap line
.
If newLine
is true, ignore the textline's flag KateTextLine::flagAutoWrapped and force a new line. Whether a new line was needed/added you can grab with newLineAdded
.
- Parameters
-
line line number col column newLine if true, force a new line newLineAdded return value is true, if new line was added (may be 0)
- Returns
- true on success
Definition at line 1379 of file katedocument.cpp.
◆ embeddedHighlightingModes()
|
overridevirtual |
Get all available highlighting modes for the current document.
Each document can be highlighted using an arbitrary number of highlighting contexts. This method will return the names for each of the used modes.
Example: The "PHP (HTML)" mode includes the highlighting for PHP, HTML, CSS and JavaScript.
- Returns
- Returns a list of embedded highlighting modes for the current Document.
Implements KTextEditor::Document.
Definition at line 6450 of file katedocument.cpp.
◆ encoding()
|
overridevirtual |
Get the current chosen encoding.
The return value is an empty string, if the document uses the default encoding of the editor and no own special encoding.
- Returns
- current encoding of the document
- See also
- setEncoding()
Implements KTextEditor::Document.
Definition at line 5031 of file katedocument.cpp.
◆ fileType()
|
inline |
Definition at line 1089 of file katedocument.h.
◆ findMatchingBracket()
KTextEditor::Range KTextEditor::DocumentPrivate::findMatchingBracket | ( | const KTextEditor::Cursor | start, |
int | maxLines ) |
Definition at line 4477 of file katedocument.cpp.
◆ findTouchedLine()
int KTextEditor::DocumentPrivate::findTouchedLine | ( | int | startLine, |
bool | down ) |
Find the next modified/saved line, starting at startLine
.
If down
is true, the search is performed downwards, otherwise upwards.
- Returns
- the touched line in the requested search direction, or -1 if not found
Definition at line 6501 of file katedocument.cpp.
◆ fromVirtualColumn() [1/2]
int KTextEditor::DocumentPrivate::fromVirtualColumn | ( | const KTextEditor::Cursor | cursor | ) | const |
Definition at line 3010 of file katedocument.cpp.
◆ fromVirtualColumn() [2/2]
int KTextEditor::DocumentPrivate::fromVirtualColumn | ( | int | line, |
int | column ) const |
Definition at line 3004 of file katedocument.cpp.
◆ handleMarkClick
|
slot |
Returns true if the click on the mark should not be further processed.
Definition at line 2109 of file katedocument.cpp.
◆ handleMarkContextMenu
|
slot |
Returns true if the context-menu event should not further be processed.
Definition at line 2122 of file katedocument.cpp.
◆ highlight()
KateHighlighting * KTextEditor::DocumentPrivate::highlight | ( | ) | const |
Definition at line 5797 of file katedocument.cpp.
◆ highlightingMode()
|
overridevirtual |
Return the name of the currently used mode.
- Returns
- name of the used mode
Implements KTextEditor::Document.
Definition at line 1824 of file katedocument.cpp.
◆ highlightingModeAt()
|
overridevirtual |
Get the highlight mode used at a given position in the document.
Retrieve the name of the applied highlight mode at a given position
in the current document.
Calling this might trigger re-highlighting up to the given line. Therefore this is not const.
- See also
- highlightingModes()
Implements KTextEditor::Document.
Definition at line 6455 of file katedocument.cpp.
◆ highlightingModes()
|
overridevirtual |
Return a list of the names of all possible modes.
- Returns
- list of mode names
Implements KTextEditor::Document.
Definition at line 1829 of file katedocument.cpp.
◆ highlightingModeSection()
|
overridevirtual |
Returns the name of the section for a highlight given its index
in the highlight list (as returned by highlightModes()).
You can use this function to build a tree of the highlight names, organized in sections.
- Parameters
-
index in the highlight list for which to find the section name.
Implements KTextEditor::Document.
Definition at line 1840 of file katedocument.cpp.
◆ indent()
void KTextEditor::DocumentPrivate::indent | ( | KTextEditor::Range | range, |
int | change ) |
Definition at line 3688 of file katedocument.cpp.
◆ inputMethodEnd()
void KTextEditor::DocumentPrivate::inputMethodEnd | ( | ) |
Definition at line 1075 of file katedocument.cpp.
◆ inputMethodStart()
void KTextEditor::DocumentPrivate::inputMethodStart | ( | ) |
Definition at line 1070 of file katedocument.cpp.
◆ insertLine
|
overrideslot |
Definition at line 863 of file katedocument.cpp.
◆ insertLines
|
overrideslot |
Definition at line 876 of file katedocument.cpp.
◆ insertTab()
void KTextEditor::DocumentPrivate::insertTab | ( | KTextEditor::ViewPrivate * | view, |
const KTextEditor::Cursor | ) |
Definition at line 3741 of file katedocument.cpp.
◆ insertText [1/2]
|
overrideslot |
Definition at line 682 of file katedocument.cpp.
◆ insertText [2/2]
|
overrideslot |
Definition at line 786 of file katedocument.cpp.
◆ isAutoReload()
bool KTextEditor::DocumentPrivate::isAutoReload | ( | ) |
Definition at line 4772 of file katedocument.cpp.
◆ isComment()
bool KTextEditor::DocumentPrivate::isComment | ( | int | line, |
int | column ) |
Definition at line 6496 of file katedocument.cpp.
◆ isDataRecoveryAvailable()
|
overridevirtual |
Returns whether a recovery is available for the current document.
- See also
- recoverData(), discardDataRecovery()
Implements KTextEditor::Document.
Definition at line 2872 of file katedocument.cpp.
◆ isEditingTransactionRunning()
|
overridevirtual |
Check whether an editing transaction is currently running.
- See also
- EditingTransaction
Implements KTextEditor::Document.
Definition at line 432 of file katedocument.cpp.
◆ isEditRunning()
bool KTextEditor::DocumentPrivate::isEditRunning | ( | ) | const |
Definition at line 5813 of file katedocument.cpp.
◆ isLineModified()
|
overridevirtual |
Check whether line
currently contains unsaved data.
If line
contains unsaved data, true is returned, otherwise false. When the user saves the file, a modified line turns into a saved line. In this case isLineModified() returns false and in its stead isLineSaved() returns true.
- Parameters
-
line line to query
- See also
- isLineSaved(), isLineTouched()
- Since
- 5.0
Implements KTextEditor::Document.
Definition at line 936 of file katedocument.cpp.
◆ isLineSaved()
|
overridevirtual |
Check whether line
currently contains only saved text.
Saved text in this case implies that a line was touched at some point by the user and then then changes were either undone or the user saved the file.
In case line
was touched and currently contains only saved data, true is returned, otherwise false.
- Parameters
-
line line to query
- See also
- isLineModified(), isLineTouched()
- Since
- 5.0
Implements KTextEditor::Document.
Definition at line 946 of file katedocument.cpp.
◆ isLineTouched()
|
overridevirtual |
Check whether line
was touched since the file was opened.
This equals the statement isLineModified() || isLineSaved().
- Parameters
-
line line to query
- See also
- isLineModified(), isLineSaved()
- Since
- 5.0
Implements KTextEditor::Document.
Definition at line 956 of file katedocument.cpp.
◆ isModifiedOnDisc()
|
inline |
- Returns
- whether the document is modified on disk since last saved
Definition at line 968 of file katedocument.h.
◆ isOnTheFlySpellCheckingEnabled()
bool KTextEditor::DocumentPrivate::isOnTheFlySpellCheckingEnabled | ( | ) | const |
Definition at line 6267 of file katedocument.cpp.
◆ isValidTextPosition()
|
overridevirtual |
Get whether cursor
is a valid text position.
A cursor position at (line, column) is valid, if
- line >= 0 and line < lines() holds, and
- column >= 0 and column <= lineLength(column).
The text position cursor
is also invalid if it is inside a Unicode surrogate. Therefore, use this function when iterating over the characters of a line.
- Parameters
-
cursor cursor position to check for validity
- Returns
- true, if
cursor
is a valid text position, otherwisefalse
- Since
- 5.0
Implements KTextEditor::Document.
Definition at line 519 of file katedocument.cpp.
◆ joinLines()
void KTextEditor::DocumentPrivate::joinLines | ( | uint | first, |
uint | last ) |
Unwrap a range of lines.
Definition at line 4413 of file katedocument.cpp.
◆ kateTextLine()
Kate::TextLine KTextEditor::DocumentPrivate::kateTextLine | ( | int | i | ) |
Same as plainKateTextLine(), except that it is made sure the line is highlighted.
Definition at line 5802 of file katedocument.cpp.
◆ lastEditingPosition()
KTextEditor::Cursor KTextEditor::DocumentPrivate::lastEditingPosition | ( | EditingPositionKind | nextOrPrevious, |
KTextEditor::Cursor | currentCursor ) |
Returns the next or previous position cursor in this document from the stack depending on the argument passed.
- Returns
- cursor invalid if m_editingStack empty
Definition at line 356 of file katedocument.cpp.
◆ lastLine()
|
inline |
gets the last line number (lines() - 1)
Definition at line 760 of file katedocument.h.
◆ lastSavedRevision()
|
overridevirtual |
Last revision the buffer got successful saved.
- Returns
- last revision buffer got saved, -1 if none
Implements KTextEditor::Document.
Definition at line 5848 of file katedocument.cpp.
◆ line()
|
overridevirtual |
Get a single text line.
- Parameters
-
line the wanted line
- Returns
- the requested line, or "" for invalid line numbers
- See also
- text(), lineLength()
Implements KTextEditor::Document.
Definition at line 580 of file katedocument.cpp.
◆ lineLength()
|
overridevirtual |
Get the length of a given line in characters.
- Parameters
-
line line to get length from
- Returns
- the number of characters in the line or -1 if the line was invalid
- See also
- line()
Implements KTextEditor::Document.
Definition at line 921 of file katedocument.cpp.
◆ lineLengthLimit()
int KTextEditor::DocumentPrivate::lineLengthLimit | ( | ) | const |
reads the line length limit from config, if it is not overridden
Definition at line 2289 of file katedocument.cpp.
◆ lines()
|
overridevirtual |
Get the count of lines of the document.
- Returns
- the current number of lines in the document
- See also
- length()
Implements KTextEditor::Document.
Definition at line 916 of file katedocument.cpp.
◆ lockRevision()
|
overridevirtual |
Lock a revision, this will keep it around until released again.
But all revisions will always be cleared on buffer clear() (and therefor load())
- Parameters
-
revision revision to lock
Implements KTextEditor::Document.
Definition at line 5853 of file katedocument.cpp.
◆ mark()
|
overridevirtual |
Get all marks set on the line
.
- Parameters
-
line requested line
- Returns
- a uint representing of the marks set in
line
concatenated by logical OR
- See also
- addMark(), removeMark()
Implements KTextEditor::Document.
Definition at line 1984 of file katedocument.cpp.
◆ markColor()
|
virtual |
Definition at line 2159 of file katedocument.cpp.
◆ markDescription()
|
overridevirtual |
Get the mark's
description to text.
- Parameters
-
mark mark to set the description
- Returns
- text of the given
mark
or QString(), if the entry does not exist
- See also
- setMarkDescription(), setMarkPixmap()
Implements KTextEditor::Document.
Definition at line 2169 of file katedocument.cpp.
◆ markIcon()
|
overridevirtual |
Get the mark's
icon.
- Parameters
-
markType mark type. If the icon does not exist the resulting is null (check with QIcon::isNull()).
- See also
- setMarkDescription()
Implements KTextEditor::Document.
Definition at line 2190 of file katedocument.cpp.
◆ marks()
|
overridevirtual |
Get a hash holding all marks in the document.
The hash key for a mark is its line.
- Returns
- a hash holding all marks in the document
KF6 TODO: Change Mark* to Mark. No need for pointer here.
Implements KTextEditor::Document.
Definition at line 2093 of file katedocument.cpp.
◆ messageDestroyed
|
slot |
Definition at line 6572 of file katedocument.cpp.
◆ mimeType()
|
overridevirtual |
Tries to detect mime-type based on file name and content of buffer.
- Returns
- the name of the mimetype for the document.
Implements KTextEditor::Document.
Definition at line 2208 of file katedocument.cpp.
◆ mode()
|
overridevirtual |
Return the name of the currently used mode.
- Returns
- name of the used mode
Implements KTextEditor::Document.
Definition at line 1796 of file katedocument.cpp.
◆ modes()
|
overridevirtual |
Return a list of the names of all possible modes.
- Returns
- list of mode names
Implements KTextEditor::Document.
Definition at line 1801 of file katedocument.cpp.
◆ modeSection()
|
overridevirtual |
Returns the name of the section for a mode given its index
in the highlight list (as returned by modes()).
You can use this function to build a tree of the mode names, organized in sections.
- Parameters
-
index index in the highlight list for which to find the section name.
Implements KTextEditor::Document.
Definition at line 1845 of file katedocument.cpp.
◆ multiPaste()
bool KTextEditor::DocumentPrivate::multiPaste | ( | KTextEditor::ViewPrivate * | view, |
const QStringList & | texts ) |
Definition at line 3572 of file katedocument.cpp.
◆ newLine()
void KTextEditor::DocumentPrivate::newLine | ( | KTextEditor::ViewPrivate * | view, |
NewLineIndent | indent = NewLineIndent::Indent, | ||
NewLinePos | newLinePos = Normal ) |
Definition at line 3278 of file katedocument.cpp.
◆ newMovingCursor()
|
overridevirtual |
Create a new moving cursor for this document.
- Parameters
-
position position of the moving cursor to create insertBehavior insertion behavior
- Returns
- new moving cursor for the document
Implements KTextEditor::Document.
Definition at line 5831 of file katedocument.cpp.
◆ newMovingRange()
|
overridevirtual |
Create a new moving range for this document.
- Parameters
-
range range of the moving range to create insertBehaviors insertion behaviors emptyBehavior behavior on becoming empty
- Returns
- new moving range for the document
Implements KTextEditor::Document.
Definition at line 5836 of file katedocument.cpp.
◆ offsetToCursor()
|
overridevirtual |
Retrives the cursor position for given offset NOTE: It will return an invalid cursor(-1, -1) if offset is invalid.
- Since
- 6.0
Implements KTextEditor::Document.
Definition at line 931 of file katedocument.cpp.
◆ onTheFlySpellChecker()
|
inline |
Definition at line 1287 of file katedocument.h.
◆ onTheFlySpellCheckingEnabled
|
slot |
Definition at line 6248 of file katedocument.cpp.
◆ openFile()
|
overridevirtual |
open the file obtained by the kparts framework the framework abstracts the loading of remote files
- Returns
- success
Reimplemented from KParts::ReadOnlyPart.
Definition at line 2295 of file katedocument.cpp.
◆ openUrl()
|
overridevirtual |
Reimplemented from KParts::ReadOnlyPart.
Definition at line 2765 of file katedocument.cpp.
◆ openWithLineLengthLimitOverride
|
slot |
Definition at line 2268 of file katedocument.cpp.
◆ ownedView()
bool KTextEditor::DocumentPrivate::ownedView | ( | KTextEditor::ViewPrivate * | view | ) |
Definition at line 2987 of file katedocument.cpp.
◆ pageUpDownMovesCursor()
bool KTextEditor::DocumentPrivate::pageUpDownMovesCursor | ( | ) | const |
Definition at line 5020 of file katedocument.cpp.
◆ paste()
void KTextEditor::DocumentPrivate::paste | ( | KTextEditor::ViewPrivate * | view, |
const QString & | text ) |
Definition at line 3607 of file katedocument.cpp.
◆ plainKateTextLine()
Kate::TextLine KTextEditor::DocumentPrivate::plainKateTextLine | ( | int | i | ) |
Return line lineno
.
Highlighting of returned line might be out-dated, which may be sufficient for pure text manipulation functions, like search/replace. If you require highlighting to be up to date, call ensureHighlighted prior to this method.
Definition at line 5808 of file katedocument.cpp.
◆ popEditState()
void KTextEditor::DocumentPrivate::popEditState | ( | ) |
Definition at line 1053 of file katedocument.cpp.
◆ postMessage()
|
overridevirtual |
Post message
to the Document and its Views.
If multiple Messages are posted, the one with the highest priority is shown first.
Usually, you can simply forget the pointer, as the Message is deleted automatically, once it is processed or the document gets closed.
If the Document does not have a View yet, the Message is queued and shown, once a View for the Document is created.
- Parameters
-
message the message to show
- Returns
- true, if
message
was posted. false, if message == 0.
Implements KTextEditor::Document.
Definition at line 6524 of file katedocument.cpp.
|
overrideslot |
Definition at line 2196 of file katedocument.cpp.
◆ printPreview
|
overrideslot |
Definition at line 2201 of file katedocument.cpp.
◆ pushEditState()
void KTextEditor::DocumentPrivate::pushEditState | ( | ) |
Definition at line 1048 of file katedocument.cpp.
◆ queryClose()
|
overridevirtual |
Reimplemented from KParts::ReadWritePart.
Definition at line 5909 of file katedocument.cpp.
◆ rangeEmpty()
|
overrideprotectedvirtual |
The range is now empty (ie.
the start and end cursors are the same). If the range has invalidateIfEmpty set, this will never be emitted, but instead rangeInvalid is triggered. You may delete the range inside this method, but don't alter the range here (for example by using setRange).
- Parameters
-
range pointer to the range which generated the notification.
Reimplemented from KTextEditor::MovingRangeFeedback.
Definition at line 6300 of file katedocument.cpp.
◆ rangeInvalid()
|
overrideprotectedvirtual |
The range is now invalid (ie.
the start and end cursors are invalid). You may delete the range inside this method, but don't alter the range here (for example by using setRange).
- Parameters
-
range pointer to the range which generated the notification.
Reimplemented from KTextEditor::MovingRangeFeedback.
Definition at line 6295 of file katedocument.cpp.
◆ rangeOnLine()
KTextEditor::Range KTextEditor::DocumentPrivate::rangeOnLine | ( | KTextEditor::Range | range, |
int | line ) const |
Definition at line 423 of file katedocument.cpp.
◆ readOnly()
|
inline |
Definition at line 107 of file katedocument.h.
◆ readSessionConfig()
|
overridevirtual |
Read session settings from the given config
.
Known flags: "SkipUrl" => don't save/restore the file "SkipMode" => don't save/restore the mode "SkipHighlighting" => don't save/restore the highlighting "SkipEncoding" => don't save/restore the encoding
- Parameters
-
config read the session settings from this KConfigGroup flags additional flags
- See also
- writeSessionConfig()
Implements KTextEditor::Document.
Definition at line 1873 of file katedocument.cpp.
◆ recoverData()
|
overridevirtual |
If recover data is available, calling recoverData() will trigger the recovery of the data.
If isDataRecoveryAvailable() returns false, calling this function does nothing.
Implements KTextEditor::Document.
Definition at line 2877 of file katedocument.cpp.
◆ redo
|
slot |
Definition at line 1708 of file katedocument.cpp.
◆ redoCount()
uint KTextEditor::DocumentPrivate::redoCount | ( | ) | const |
Definition at line 1690 of file katedocument.cpp.
◆ refreshOnTheFlyCheck
|
slot |
Definition at line 6288 of file katedocument.cpp.
◆ rememberUserDidSetIndentationMode()
|
inline |
set indentation mode by user this will remember that a user did set it and will avoid reset on save
Definition at line 1108 of file katedocument.h.
◆ removeAllTrailingSpaces()
void KTextEditor::DocumentPrivate::removeAllTrailingSpaces | ( | ) |
This function doesn't check for config and is available for use all the time via an action.
Definition at line 5661 of file katedocument.cpp.
◆ removeLine
|
overrideslot |
Definition at line 894 of file katedocument.cpp.
◆ removeMark
|
overrideslot |
Definition at line 2055 of file katedocument.cpp.
◆ removeText
|
overrideslot |
Definition at line 796 of file katedocument.cpp.
◆ removeView()
void KTextEditor::DocumentPrivate::removeView | ( | KTextEditor::View * | view | ) |
removes the view from the list of views.
The view is not deleted. That's your job. Or, easier, just delete the view in the first place. It will remove itself. TODO: this could be converted to a private slot connected to the view's destroyed() signal. It is not currently called anywhere except from the KTextEditor::ViewPrivate destructor.
Definition at line 2968 of file katedocument.cpp.
◆ repaintViews()
void KTextEditor::DocumentPrivate::repaintViews | ( | bool | paintOnlyDirty = true | ) |
Definition at line 4463 of file katedocument.cpp.
◆ replaceCharactersByEncoding()
void KTextEditor::DocumentPrivate::replaceCharactersByEncoding | ( | KTextEditor::Range | range | ) |
Definition at line 6418 of file katedocument.cpp.
◆ replaceText [1/2]
|
inlineoverrideslot |
Definition at line 160 of file katedocument.h.
◆ replaceText [2/2]
|
overrideslot |
Definition at line 5787 of file katedocument.cpp.
◆ requestMarkTooltip
|
slot |
Definition at line 2098 of file katedocument.cpp.
◆ revision()
|
overridevirtual |
Current revision.
- Returns
- current revision
Implements KTextEditor::Document.
Definition at line 5843 of file katedocument.cpp.
◆ save
|
overrideslot |
Definition at line 6066 of file katedocument.cpp.
◆ saveAs()
|
overridevirtual |
Reimplemented from KParts::ReadWritePart.
Definition at line 6088 of file katedocument.cpp.
◆ saveEditingPositions
|
slot |
Saves the editing positions into the stack.
If the consecutive editings happens in the same line, then remove the previous and add the new one with updated column no.
Definition at line 319 of file katedocument.cpp.
◆ saveFile()
|
overridevirtual |
save the file obtained by the kparts framework the framework abstracts the uploading of remote files
- Returns
- success
Implements KParts::ReadWritePart.
Definition at line 2429 of file katedocument.cpp.
◆ searchText()
QList< KTextEditor::Range > KTextEditor::DocumentPrivate::searchText | ( | KTextEditor::Range | range, |
const QString & | pattern, | ||
const KTextEditor::SearchOptions | options ) const |
Definition at line 1724 of file katedocument.cpp.
◆ setActiveTemplateHandler()
void KTextEditor::DocumentPrivate::setActiveTemplateHandler | ( | KateTemplateHandler * | handler | ) |
Definition at line 6516 of file katedocument.cpp.
◆ setActiveView()
void KTextEditor::DocumentPrivate::setActiveView | ( | KTextEditor::View * | view | ) |
Definition at line 2978 of file katedocument.cpp.
◆ setAnnotationModel()
|
overridevirtual |
Sets a new AnnotationModel for this document to provide annotation information for each line.
- Parameters
-
model the new AnnotationModel
Implements KTextEditor::Document.
Definition at line 5895 of file katedocument.cpp.
◆ setConfigValue()
|
overridevirtual |
Set a the key's
value to value
.
Implements KTextEditor::Document.
Definition at line 5774 of file katedocument.cpp.
◆ setDefaultDictionary
|
slot |
Definition at line 6233 of file katedocument.cpp.
◆ setDictionary [1/2]
|
slot |
Definition at line 6170 of file katedocument.cpp.
◆ setDictionary [2/2]
|
slot |
Definition at line 6157 of file katedocument.cpp.
◆ setDontChangeHlOnSave()
void KTextEditor::DocumentPrivate::setDontChangeHlOnSave | ( | ) |
allow to mark, that we changed hl on user wish and should not reset it atm used for the user visible menu to select highlightings
Definition at line 1861 of file katedocument.cpp.
◆ setEditableMarks
|
overrideslot |
Definition at line 2174 of file katedocument.cpp.
◆ setEncoding()
|
overridevirtual |
Set the encoding for this document.
This encoding will be used while loading and saving files, it will not affect the already existing content of the document, e.g. if the file has already been opened without the correct encoding, this will not fix it, you would for example need to trigger a reload for this.
- Parameters
-
encoding new encoding for the document, the name must be accepted by QStringDecoder/QStringEncoder, if an empty encoding name is given, the part should fallback to its own default encoding, e.g. the system encoding or the global user settings
- Returns
- true on success, or false, if the encoding could not be set.
- See also
- encoding()
Implements KTextEditor::Document.
Definition at line 5026 of file katedocument.cpp.
◆ setHighlightingMode()
|
overridevirtual |
Set the current mode of the document by giving its name.
- Parameters
-
name name of the mode to use for this document
- Returns
- true on success, otherwise false
Implements KTextEditor::Document.
Definition at line 1814 of file katedocument.cpp.
◆ setMark
|
overrideslot |
Definition at line 1994 of file katedocument.cpp.
◆ setMarkDescription
|
overrideslot |
Definition at line 2154 of file katedocument.cpp.
◆ setMarkIcon
|
overrideslot |
Definition at line 2185 of file katedocument.cpp.
◆ setMode()
|
overridevirtual |
Set the current mode of the document by giving its name.
- Parameters
-
name name of the mode to use for this document
- Returns
- true on success, otherwise false
Implements KTextEditor::Document.
Definition at line 1786 of file katedocument.cpp.
◆ setModified()
|
overridevirtual |
Reimplemented from KParts::ReadWritePart.
Definition at line 2908 of file katedocument.cpp.
◆ setModifiedOnDisk()
|
overridevirtual |
Set the document's modified-on-disk state to reason
.
KTextEditor implementations should emit the signal modifiedOnDisk() along with the reason. When the document is in a clean state again the reason should be ModifiedOnDiskReason::OnDiskUnmodified.
- Parameters
-
reason the modified-on-disk reason.
- See also
- ModifiedOnDiskReason, modifiedOnDisk()
Implements KTextEditor::Document.
Definition at line 4816 of file katedocument.cpp.
◆ setModifiedOnDiskWarning()
|
overridevirtual |
Control, whether the editor should show a warning dialog whenever a file was modified on disk.
If on
is true the editor will show warning dialogs.
- Parameters
-
on controls, whether the editor should show a warning dialog for files modified on disk
Implements KTextEditor::Document.
Definition at line 4830 of file katedocument.cpp.
◆ setPageUpDownMovesCursor
|
slot |
Definition at line 5015 of file katedocument.cpp.
◆ setReadWrite()
|
overridevirtual |
Reimplemented from KParts::ReadWritePart.
Definition at line 2891 of file katedocument.cpp.
◆ setText [1/2]
|
overrideslot |
Definition at line 586 of file katedocument.cpp.
◆ setText [2/2]
|
overrideslot |
Definition at line 623 of file katedocument.cpp.
◆ setUndoMergeAllEdits()
void KTextEditor::DocumentPrivate::setUndoMergeAllEdits | ( | bool | merge | ) |
Definition at line 5818 of file katedocument.cpp.
◆ setVariable()
|
virtual |
Set the variable name
to value
.
Setting and changing a variable has immediate effect on the Document. For instance, setting the variable indent-mode to cstyle will immediately cause the Document to load the C Style indenter.
// TODO KF6: expose in KTextEditor::Document?
- Parameters
-
name the variable name value the value to be set
- See also
- variable()
Definition at line 5450 of file katedocument.cpp.
◆ setWordWrap
|
slot |
Definition at line 4995 of file katedocument.cpp.
◆ setWordWrapAt
|
slot |
Definition at line 5005 of file katedocument.cpp.
◆ singleViewMode()
|
inline |
Definition at line 111 of file katedocument.h.
◆ slotModifiedOnDisk
|
virtualslot |
Ask the user what to do, if the file has been modified on disk.
Reimplemented from KTextEditor::Document.
Definition at line 4682 of file katedocument.cpp.
◆ slotQueryClose_save
|
slot |
Definition at line 5741 of file katedocument.cpp.
◆ startUrlForFileDialog()
QUrl KTextEditor::DocumentPrivate::startUrlForFileDialog | ( | ) |
Definition at line 6110 of file katedocument.cpp.
◆ swapFile()
Kate::SwapFile * KTextEditor::DocumentPrivate::swapFile | ( | ) |
Definition at line 6460 of file katedocument.cpp.
◆ swapTextRanges()
void KTextEditor::DocumentPrivate::swapTextRanges | ( | KTextEditor::Range | firstWord, |
KTextEditor::Range | secondWord ) |
Definition at line 3406 of file katedocument.cpp.
◆ tagLine
|
slot |
Definition at line 4458 of file katedocument.cpp.
◆ tagLines
|
slot |
Definition at line 4451 of file katedocument.cpp.
◆ text() [1/2]
|
overridevirtual |
Get the document content.
- Returns
- the complete document content
- See also
- setText()
Implements KTextEditor::Document.
Definition at line 437 of file katedocument.cpp.
◆ text() [2/2]
|
overridevirtual |
Get the document content within the given range
.
- Parameters
-
range the range of text to retrieve block Set this to true to receive text in a visual block, rather than everything inside range
.
- Returns
- the requested text part, or QString() for invalid ranges.
- See also
- setText()
Implements KTextEditor::Document.
Definition at line 442 of file katedocument.cpp.
◆ textInsertedRange
|
signal |
The document
emits this signal whenever text was inserted.
The insertion occurred at range.start(), and new text now occupies up to range.end().
- Parameters
-
document document which emitted this signal range range that the newly inserted text occupies
- See also
- insertText(), insertLine()
◆ textLines()
|
overridevirtual |
Get the document content within the given range
.
- Parameters
-
range the range of text to retrieve block Set this to true to receive text in a visual block, rather than everything inside range
.
- Returns
- the requested text lines, or QStringList() for invalid ranges. no end of line termination is included.
- See also
- setText()
Implements KTextEditor::Document.
Definition at line 541 of file katedocument.cpp.
◆ textRemoved
|
signal |
The document
emits this signal whenever range
was removed, i.e.
text was removed.
- Parameters
-
document document which emitted this signal range range that the removed text previously occupied oldText the text that has been removed
- See also
- removeText(), removeLine(), clear()
◆ totalCharacters()
|
overridevirtual |
Get the count of characters in the document.
A TAB character counts as only one character.
- Returns
- the number of characters in the document
- See also
- lines()
Implements KTextEditor::Document.
Definition at line 907 of file katedocument.cpp.
◆ toVirtualColumn() [1/2]
int KTextEditor::DocumentPrivate::toVirtualColumn | ( | const KTextEditor::Cursor | cursor | ) | const |
Definition at line 2999 of file katedocument.cpp.
◆ toVirtualColumn() [2/2]
int KTextEditor::DocumentPrivate::toVirtualColumn | ( | int | line, |
int | column ) const |
Definition at line 2993 of file katedocument.cpp.
◆ transform()
void KTextEditor::DocumentPrivate::transform | ( | KTextEditor::ViewPrivate * | view, |
KTextEditor::Cursor | c, | ||
TextTransform | t ) |
Handling uppercase, lowercase and capitalize for the view.
If there is a selection, that is transformed, otherwise for uppercase or lowercase the character right of the cursor is transformed, for capitalize the word under the cursor is transformed.
Definition at line 4383 of file katedocument.cpp.
◆ transformCursor() [1/2]
|
overridevirtual |
Transform a cursor from one revision to an other.
- Parameters
-
line line number of the cursor to transform column column number of the cursor to transform insertBehavior behavior of this cursor on insert of text at its position fromRevision from this revision we want to transform toRevision to this revision we want to transform, default of -1 is current revision
Implements KTextEditor::Document.
Definition at line 5863 of file katedocument.cpp.
◆ transformCursor() [2/2]
|
overridevirtual |
Transform a cursor from one revision to an other.
- Parameters
-
cursor cursor to transform insertBehavior behavior of this cursor on insert of text at its position fromRevision from this revision we want to transform toRevision to this revision we want to transform, default of -1 is current revision
Implements KTextEditor::Document.
Definition at line 5872 of file katedocument.cpp.
◆ transformRange()
|
overridevirtual |
Transform a range from one revision to an other.
- Parameters
-
range range to transform insertBehaviors behavior of this range on insert of text at its position emptyBehavior behavior on becoming empty fromRevision from this revision we want to transform toRevision to this revision we want to transform, default of -1 is current revision
Implements KTextEditor::Document.
Definition at line 5883 of file katedocument.cpp.
◆ transpose()
void KTextEditor::DocumentPrivate::transpose | ( | const KTextEditor::Cursor | cursor | ) |
Definition at line 3373 of file katedocument.cpp.
◆ typeChars()
void KTextEditor::DocumentPrivate::typeChars | ( | KTextEditor::ViewPrivate * | view, |
QString | chars ) |
Type chars in a view.
Characters are filtered in KateViewInternal::isAcceptableInput() before calling typeChars.
- Parameters
-
view view that received the input chars characters to type
Definition at line 3045 of file katedocument.cpp.
◆ undo
|
slot |
Definition at line 1695 of file katedocument.cpp.
◆ undoCount()
uint KTextEditor::DocumentPrivate::undoCount | ( | ) | const |
Definition at line 1685 of file katedocument.cpp.
◆ undoManager()
|
inline |
Definition at line 378 of file katedocument.h.
◆ unlockRevision()
|
overridevirtual |
Release a revision.
- Parameters
-
revision revision to release
Implements KTextEditor::Document.
Definition at line 5858 of file katedocument.cpp.
◆ updateConfig()
void KTextEditor::DocumentPrivate::updateConfig | ( | ) |
Definition at line 5036 of file katedocument.cpp.
◆ updateFileType()
bool KTextEditor::DocumentPrivate::updateFileType | ( | const QString & | newType, |
bool | user = false ) |
- Returns
- false if
newType
is an invalid mode.
Definition at line 5676 of file katedocument.cpp.
◆ userSetEncodingForNextReload()
|
inline |
User did set encoding for next reload => enforce it!
Definition at line 1116 of file katedocument.h.
◆ variable()
Returns the value for the variable name
.
If the Document does not have a variable called name
, an empty QString() is returned.
// TODO KF6: expose in KTextEditor::Document?
- Parameters
-
name variable to query
- Returns
- value of the variable
name
- See also
- setVariable()
Definition at line 5441 of file katedocument.cpp.
◆ views()
|
inlineoverridevirtual |
Returns the views pre-casted to KTextEditor::Views.
Implements KTextEditor::Document.
Definition at line 127 of file katedocument.h.
◆ widget()
|
overridevirtual |
- Returns
- The widget defined by this part, set by setWidget().
Reimplemented from KParts::Part.
Definition at line 380 of file katedocument.cpp.
◆ wordAt()
|
overridevirtual |
Get the word at the text position cursor
.
The returned word is defined by the word boundaries to the left and right starting at cursor
. The algorithm takes highlighting information into account, e.g. a dash ('-') in C++ is interpreted as word boundary, whereas e.g. CSS allows identifiers with dash ('-').
If cursor
is not a valid text position or if there is no word under the requested position cursor
, an empty string is returned.
- Parameters
-
cursor requested cursor position for the word
- Returns
- the word under the cursor or an empty string if there is no word.
- See also
- wordRangeAt(), characterAt()
Implements KTextEditor::Document.
Definition at line 489 of file katedocument.cpp.
◆ wordRangeAt()
|
overridevirtual |
Get the text range for the word located under the text position cursor
.
The returned word is defined by the word boundaries to the left and right starting at cursor
. The algorithm takes highlighting information into account, e.g. a dash ('-') in C++ is interpreted as word boundary, whereas e.g. CSS allows identifiers with dash ('-').
If cursor
is not a valid text position or if there is no word under the requested position cursor
, an invalid text range is returned. If the text range is valid, it is always on a single line.
- Parameters
-
cursor requested cursor position for the word
- Returns
- the Range spanning the word under the cursor or an invalid range if there is no word.
Implements KTextEditor::Document.
Definition at line 494 of file katedocument.cpp.
◆ wordWrap()
bool KTextEditor::DocumentPrivate::wordWrap | ( | ) | const |
Definition at line 5000 of file katedocument.cpp.
◆ wordWrapAt()
unsigned int KTextEditor::DocumentPrivate::wordWrapAt | ( | ) | const |
Definition at line 5010 of file katedocument.cpp.
◆ wrapParagraph()
bool KTextEditor::DocumentPrivate::wrapParagraph | ( | int | first, |
int | last ) |
Wrap lines touched by the selection with respect of existing paragraphs.
To do so will the paragraph prior to the wrap joined as one single line which cause an almost perfect wrapped paragraph as long as there are no unneeded spaces exist or some formatting like this comment block. Without any selection the current line is wrapped. Empty lines around each paragraph are untouched.
- Parameters
-
first line to begin wrapping last line to stop wrapping
- Returns
- true on success
Definition at line 1196 of file katedocument.cpp.
◆ wrapText()
bool KTextEditor::DocumentPrivate::wrapText | ( | int | startLine, |
int | endLine ) |
Warp a line.
- Parameters
-
startLine line to begin wrapping endLine line to stop wrapping
- Returns
- true on success
Definition at line 1080 of file katedocument.cpp.
◆ writeSessionConfig()
|
overridevirtual |
Write session settings to the config
.
See readSessionConfig() for more details.
- Parameters
-
config write the session settings to this KConfigGroup flags additional flags
- See also
- readSessionConfig()
Implements KTextEditor::Document.
Definition at line 1927 of file katedocument.cpp.
Member Data Documentation
◆ m_defaultDictionary
|
protected |
Definition at line 1325 of file katedocument.h.
◆ m_dictionaryRanges
|
protected |
Definition at line 1326 of file katedocument.h.
◆ m_onTheFlyChecker
|
protected |
Definition at line 1324 of file katedocument.h.
◆ m_undoManager
|
protected |
Definition at line 384 of file katedocument.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:16:21 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.