KTextEditor::Message
#include <KTextEditor/Message>
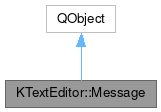
Public Types | |
enum | AutoHideMode { Immediate = 0 , AfterUserInteraction } |
enum | MessagePosition { AboveView = 0 , BelowView , TopInView , BottomInView , CenterInView } |
enum | MessageType { Positive = 0 , Information , Warning , Error } |
Signals | |
void | closed (KTextEditor::Message *message) |
void | iconChanged (const QIcon &icon) |
void | textChanged (const QString &text) |
Public Slots | |
void | setIcon (const QIcon &icon) |
void | setText (const QString &richtext) |
Public Member Functions | |
Message (const QString &richtext, MessageType type=Message::Information) | |
~Message () override | |
QList< QAction * > | actions () const |
void | addAction (QAction *action, bool closeOnTrigger=true) |
int | autoHide () const |
KTextEditor::Message::AutoHideMode | autoHideMode () const |
KTextEditor::Document * | document () const |
QIcon | icon () const |
MessageType | messageType () const |
MessagePosition | position () const |
int | priority () const |
void | setAutoHide (int delay=0) |
void | setAutoHideMode (KTextEditor::Message::AutoHideMode mode) |
void | setDocument (KTextEditor::Document *document) |
void | setPosition (MessagePosition position) |
void | setPriority (int priority) |
void | setView (KTextEditor::View *view) |
void | setWordWrap (bool wordWrap) |
QString | text () const |
KTextEditor::View * | view () const |
bool | wordWrap () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class holds a Message to display in Views.
Introduction
The Message class holds the data used to display interactive message widgets in the editor. Use the Document::postMessage() to post a message as follows:
A Message is deleted automatically if the Message gets closed, meaning that you usually can forget the pointer. If you really need to delete a message before the user processed it, always guard it with a QPointer!
Message Creation and Deletion
To create a new Message, use code like this:
Although discouraged in general, the text of the Message can be changed on the fly when it is already visible with setText().
Once you posted the Message through Document::postMessage(), the lifetime depends on the user interaction. The Message gets automatically deleted either if the user clicks a closing action in the message, or for instance if the document is reloaded.
If you posted a message but want to remove it yourself again, just delete the message. But beware of the following warning!
- Warning
- Always use QPointer<Message> to guard the message pointer from getting invalid, if you need to access the Message after you posted it.
Positioning
By default, the Message appears right above of the View. However, if desired, the position can be changed through setPosition(). For instance, the search-and-replace code in Kate Part shows the number of performed replacements in a message floating in the view. For further information, have a look at the enum MessagePosition.
Autohiding Messages
Messages can be shown for only a short amount of time by using the autohide feature. With setAutoHide() a timeout in milliseconds can be set after which the Message is automatically hidden. Further, use setAutoHideMode() to either trigger the autohide timer as soon as the widget is shown (AutoHideMode::Immediate), or only after user interaction with the view (AutoHideMode::AfterUserInteraction).
The default autohide mode is set to AutoHideMode::AfterUserInteraction. This way, it is unlikely the user misses a notification.
- Since
- 4.11
Member Enumeration Documentation
◆ AutoHideMode
The AutoHideMode determines when to trigger the autoHide timer.
- See also
- setAutoHide(), autoHide()
Enumerator | |
---|---|
Immediate | auto-hide is triggered as soon as the message is shown |
AfterUserInteraction | auto-hide is triggered only after the user interacted with the view |
◆ MessagePosition
Message position used to place the message either above or below of the KTextEditor::View.
◆ MessageType
Message types used as visual indicator.
The message types match exactly the behavior of KMessageWidget::MessageType. For simple notifications either use Positive or Information.
Enumerator | |
---|---|
Positive | positive information message |
Information | information message type |
Warning | warning message type |
Error | error message type |
Constructor & Destructor Documentation
◆ Message()
KTextEditor::Message::Message | ( | const QString & | richtext, |
MessageType | type = Message::Information ) |
Constructor for new messages.
- Parameters
-
type the message type, e.g. MessageType::Information richtext text to be displayed
Definition at line 30 of file messageinterface.cpp.
◆ ~Message()
|
override |
Destructor.
Definition at line 37 of file messageinterface.cpp.
Member Function Documentation
◆ actions()
Accessor to all actions, mainly used in the internal implementation to add the actions into the gui.
- See also
- addAction()
Definition at line 85 of file messageinterface.cpp.
◆ addAction()
void KTextEditor::Message::addAction | ( | QAction * | action, |
bool | closeOnTrigger = true ) |
Adds an action to the message.
By default (closeOnTrigger
= true), the action closes the message displayed in all Views. If closeOnTrigger
is false, the message is stays open.
The actions will be displayed in the order you added the actions.
To connect to an action, use the following code:
- Parameters
-
action action to be added closeOnTrigger when triggered, the message widget is closed
- Warning
- The added actions are deleted automatically. So do not delete the added actions yourself.
Definition at line 73 of file messageinterface.cpp.
◆ autoHide()
int KTextEditor::Message::autoHide | ( | ) | const |
Returns the auto hide time in milliseconds.
Please refer to setAutoHide() for an explanation of the return value.
- See also
- setAutoHide(), autoHideMode()
Definition at line 95 of file messageinterface.cpp.
◆ autoHideMode()
KTextEditor::Message::AutoHideMode KTextEditor::Message::autoHideMode | ( | ) | const |
Get the Message's auto hide mode.
The default mode is set to AutoHideMode::AfterUserInteraction.
- See also
- setAutoHideMode(), autoHide()
Definition at line 105 of file messageinterface.cpp.
◆ closed
|
signal |
This signal is emitted before the message
is deleted.
Afterwards, this pointer is invalid.
Use the function document() to access the associated Document.
- Parameters
-
message the closed/processed message
◆ document()
KTextEditor::Document * KTextEditor::Message::document | ( | ) | const |
Returns the document pointer this message was posted in.
This pointer is 0 as long as the message was not posted.
Definition at line 145 of file messageinterface.cpp.
◆ icon()
QIcon KTextEditor::Message::icon | ( | ) | const |
Returns the icon of this message.
If the message has no icon set, a null icon is returned.
- See also
- setIcon()
Definition at line 63 of file messageinterface.cpp.
◆ iconChanged
|
signal |
◆ messageType()
Message::MessageType KTextEditor::Message::messageType | ( | ) | const |
Returns the message type set in the constructor.
Definition at line 68 of file messageinterface.cpp.
◆ position()
Message::MessagePosition KTextEditor::Message::position | ( | ) | const |
Returns the message position of this message.
- See also
- setPosition(), MessagePosition
Definition at line 155 of file messageinterface.cpp.
◆ priority()
int KTextEditor::Message::priority | ( | ) | const |
Returns the priority of the message.
- See also
- setPriority()
Definition at line 125 of file messageinterface.cpp.
◆ setAutoHide()
void KTextEditor::Message::setAutoHide | ( | int | delay = 0 | ) |
Set the auto hide time to delay
milliseconds.
If delay
< 0, auto hide is disabled. If delay
= 0, auto hide is enabled and set to a sane default value of several seconds.
By default, auto hide is disabled.
- See also
- autoHide(), setAutoHideMode()
Definition at line 90 of file messageinterface.cpp.
◆ setAutoHideMode()
void KTextEditor::Message::setAutoHideMode | ( | KTextEditor::Message::AutoHideMode | mode | ) |
Sets the auto hide mode to mode
.
The default mode is set to AutoHideMode::AfterUserInteraction.
- Parameters
-
mode auto hide mode
- See also
- autoHideMode(), setAutoHide()
Definition at line 100 of file messageinterface.cpp.
◆ setDocument()
void KTextEditor::Message::setDocument | ( | KTextEditor::Document * | document | ) |
Set the document pointer to document
.
This is called by the implementation, as soon as you post a message through Document::postMessage(), so that you do not have to call this yourself.
- See also
- document()
Definition at line 140 of file messageinterface.cpp.
◆ setIcon
|
slot |
Add an optional icon
for this notification which will be shown next to the message text.
If the message was already sent through Document::postMessage(), the displayed icon changes on the fly.
- Note
- Change the icon on the fly with care, since changing the text may resize the notification widget, which may result in a distracting user experience.
- Parameters
-
icon the icon to be displayed
- See also
- iconChanged()
Definition at line 57 of file messageinterface.cpp.
◆ setPosition()
void KTextEditor::Message::setPosition | ( | Message::MessagePosition | position | ) |
Sets the position of the message to position
.
By default, the position is set to MessagePosition::AboveView.
- See also
- MessagePosition
Definition at line 150 of file messageinterface.cpp.
◆ setPriority()
void KTextEditor::Message::setPriority | ( | int | priority | ) |
Set the priority of this message to priority
.
Messages with higher priority are shown first. The default priority is 0.
- See also
- priority()
Definition at line 120 of file messageinterface.cpp.
◆ setText
|
slot |
Sets the notification contents to richtext
.
If the message was already sent through Document::postMessage(), the displayed text changes on the fly.
- Note
- Change text on the fly with care, since changing the text may resize the notification widget, which may result in a distracting user experience.
- Parameters
-
richtext new notification text (rich text supported)
- See also
- textChanged()
Definition at line 49 of file messageinterface.cpp.
◆ setView()
void KTextEditor::Message::setView | ( | KTextEditor::View * | view | ) |
Set the associated view of the message.
If view
is 0, the message is shown in all Views of the Document. If view
is given, i.e. non-zero, the message is shown only in this view.
- Parameters
-
view the associated view the message should be displayed in
Definition at line 130 of file messageinterface.cpp.
◆ setWordWrap()
void KTextEditor::Message::setWordWrap | ( | bool | wordWrap | ) |
Enabled word wrap according to wordWrap
.
By default, auto wrap is disabled.
Word wrap is enabled automatically, if the Message's width is larger than the parent widget's width to avoid breaking the gui layout.
- See also
- wordWrap()
Definition at line 110 of file messageinterface.cpp.
◆ text()
QString KTextEditor::Message::text | ( | ) | const |
Returns the text set in the constructor.
Definition at line 44 of file messageinterface.cpp.
◆ textChanged
|
signal |
◆ view()
KTextEditor::View * KTextEditor::Message::view | ( | ) | const |
This function returns the view you set by setView().
If setView() was not called, the return value is 0.
Definition at line 135 of file messageinterface.cpp.
◆ wordWrap()
bool KTextEditor::Message::wordWrap | ( | ) | const |
Check, whether word wrap is enabled or not.
- See also
- setWordWrap()
Definition at line 115 of file messageinterface.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:16:21 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.