KTextEditor::View
#include <KTextEditor/View>
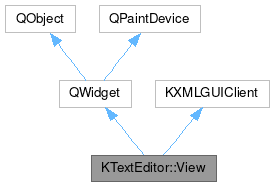
Public Types | |
enum | InputMode { NormalInputMode = 0 , ViInputMode = 1 } |
enum | LineType { RealLine = 0 , VisibleLine = 1 } |
enum | ViewMode { NormalModeInsert = 0 , NormalModeOverwrite = 1 , ViModeNormal = 10 , ViModeInsert = 11 , ViModeVisual = 12 , ViModeVisualLine = 13 , ViModeVisualBlock = 14 , ViModeReplace = 15 } |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Public Member Functions | |
~View () override | |
virtual void | abortCompletion ()=0 |
virtual KTextEditor::AbstractAnnotationItemDelegate * | annotationItemDelegate () const =0 |
virtual AnnotationModel * | annotationModel () const =0 |
virtual bool | blockSelection () const =0 |
virtual QList< CodeCompletionModel * > | codeCompletionModels () const =0 |
virtual QStringList | configKeys () const =0 |
virtual QVariant | configValue (const QString &key)=0 |
virtual QMenu * | contextMenu () const =0 |
virtual KTextEditor::Cursor | coordinatesToCursor (const QPoint &coord) const =0 |
virtual Cursor | cursorPosition () const =0 |
virtual QPoint | cursorPositionCoordinates () const =0 |
QList< KTextEditor::Cursor > | cursorPositions () const |
virtual Cursor | cursorPositionVirtual () const =0 |
virtual QPoint | cursorToCoordinate (KTextEditor::Cursor cursor) const =0 |
virtual QMenu * | defaultContextMenu (QMenu *menu=nullptr) const =0 |
virtual QExplicitlySharedDataPointer< KTextEditor::Attribute > | defaultStyleAttribute (KSyntaxHighlighting::Theme::TextStyle defaultStyle) const =0 |
virtual Document * | document () const =0 |
int | firstDisplayedLine (LineType lineType=RealLine) const |
virtual void | forceCompletion ()=0 |
virtual QScrollBar * | horizontalScrollBar () const =0 |
bool | insertTemplate (KTextEditor::Cursor insertPosition, const QString &templateString, const QString &script=QString()) |
virtual bool | insertText (const QString &text) |
virtual bool | isAnnotationBorderVisible () const =0 |
virtual bool | isAutomaticInvocationEnabled () const =0 |
virtual bool | isCompletionActive () const =0 |
bool | isStatusBarEnabled () const |
int | lastDisplayedLine (LineType lineType=RealLine) const |
virtual QList< KTextEditor::AttributeBlock > | lineAttributes (int line)=0 |
virtual KTextEditor::MainWindow * | mainWindow () const =0 |
KTextEditor::Cursor | maxScrollPosition () const |
virtual bool | mouseTrackingEnabled () const =0 |
virtual bool | print ()=0 |
virtual void | printPreview ()=0 |
virtual void | readSessionConfig (const KConfigGroup &config, const QSet< QString > &flags=QSet< QString >())=0 |
virtual void | registerCompletionModel (CodeCompletionModel *model)=0 |
virtual void | registerInlineNoteProvider (KTextEditor::InlineNoteProvider *provider)=0 |
virtual void | registerTextHintProvider (KTextEditor::TextHintProvider *provider)=0 |
virtual bool | removeSelection ()=0 |
virtual bool | removeSelectionText ()=0 |
virtual bool | selection () const =0 |
virtual Range | selectionRange () const =0 |
QList< KTextEditor::Range > | selectionRanges () const |
virtual QString | selectionText () const =0 |
virtual void | setAnnotationBorderVisible (bool visible)=0 |
virtual void | setAnnotationItemDelegate (KTextEditor::AbstractAnnotationItemDelegate *delegate)=0 |
virtual void | setAnnotationModel (AnnotationModel *model)=0 |
virtual void | setAnnotationUniformItemSizes (bool uniformItemSizes)=0 |
virtual void | setAutomaticInvocationEnabled (bool enabled=true)=0 |
virtual bool | setBlockSelection (bool on)=0 |
virtual void | setConfigValue (const QString &key, const QVariant &value)=0 |
virtual void | setContextMenu (QMenu *menu)=0 |
virtual bool | setCursorPosition (Cursor position)=0 |
void | setCursorPositions (const QList< KTextEditor::Cursor > &positions) |
void | setHorizontalScrollPosition (int x) |
virtual bool | setMouseTrackingEnabled (bool enable)=0 |
void | setScrollPosition (KTextEditor::Cursor cursor) |
virtual bool | setSelection (Range range)=0 |
void | setSelections (const QList< KTextEditor::Range > &ranges) |
void | setStatusBarEnabled (bool enable) |
virtual void | setTextHintDelay (int delay)=0 |
virtual void | setViewInputMode (InputMode inputMode)=0 |
virtual void | startCompletion (const Range &word, const QList< CodeCompletionModel * > &models=QList< CodeCompletionModel * >(), KTextEditor::CodeCompletionModel::InvocationType invocationType=KTextEditor::CodeCompletionModel::ManualInvocation)=0 |
virtual void | startCompletion (Range word, CodeCompletionModel *model)=0 |
QRect | textAreaRect () const |
virtual int | textHintDelay () const =0 |
KSyntaxHighlighting::Theme | theme () const |
virtual bool | uniformAnnotationItemSizes () const =0 |
virtual void | unregisterCompletionModel (CodeCompletionModel *model)=0 |
virtual void | unregisterInlineNoteProvider (KTextEditor::InlineNoteProvider *provider)=0 |
virtual void | unregisterTextHintProvider (KTextEditor::TextHintProvider *provider)=0 |
virtual QScrollBar * | verticalScrollBar () const =0 |
virtual InputMode | viewInputMode () const =0 |
virtual QString | viewInputModeHuman () const =0 |
virtual ViewMode | viewMode () const =0 |
virtual QString | viewModeHuman () const =0 |
virtual void | writeSessionConfig (KConfigGroup &config, const QSet< QString > &flags=QSet< QString >())=0 |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
virtual QSize | sizeHint () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
![]() | |
KXMLGUIClient (KXMLGUIClient *parent) | |
virtual QAction * | action (const QDomElement &element) const |
QAction * | action (const QString &name) const |
virtual KActionCollection * | actionCollection () const |
QList< KXMLGUIClient * > | childClients () |
KXMLGUIBuilder * | clientBuilder () const |
virtual QString | componentName () const |
virtual QDomDocument | domDocument () const |
KXMLGUIFactory * | factory () const |
void | insertChildClient (KXMLGUIClient *child) |
KXMLGUIClient * | parentClient () const |
void | plugActionList (const QString &name, const QList< QAction * > &actionList) |
void | reloadXML () |
void | removeChildClient (KXMLGUIClient *child) |
void | replaceXMLFile (const QString &xmlfile, const QString &localxmlfile, bool merge=false) |
void | setClientBuilder (KXMLGUIBuilder *builder) |
void | setFactory (KXMLGUIFactory *factory) |
void | setXMLGUIBuildDocument (const QDomDocument &doc) |
void | unplugActionList (const QString &name) |
virtual QString | xmlFile () const |
QDomDocument | xmlguiBuildDocument () const |
Protected Member Functions | |
View (ViewPrivate *impl, QWidget *parent) | |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
virtual void | closeEvent (QCloseEvent *event) |
virtual void | contextMenuEvent (QContextMenuEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual bool | event (QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentName (const QString &componentName, const QString &componentDisplayName) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
Detailed Description
A text widget with KXMLGUIClient that represents a Document.
Topics:
- Introduction
- Merging the View's GUI
- Text Selection
- Cursor Positions
- Mouse Tracking
- Input/View Modes
- View Config
- Annotation Interface
- Inline Notes
- Introduction
- Completion Interface
Introduction
The View class represents a single view of a KTextEditor::Document, get the document on which the view operates with document(). A view provides both the graphical representation of the text and the KXMLGUIClient for the actions. The view itself does not provide text manipulation, use the methods from the Document instead. The only method to insert text is insertText(), which inserts the given text at the current cursor position and emits the signal textInserted().
Usually a view is created by using Document::createView(). Furthermore a view can have a context menu. Set it with setContextMenu() and get it with contextMenu().
Merging the View's GUI
A View is derived from the class KXMLGUIClient, so its GUI elements (like menu entries and toolbar items) can be merged into the application's GUI (or into a KXMLGUIFactory) by calling
You can add only one view as client, so if you have several views, you first have to remove the current view, and then add the new one, like this
Text Selection
As the view is a graphical text editor it provides normal and block text selection. You can check with selection() whether a selection exists. removeSelection() will remove the selection without removing the text, whereas removeSelectionText() also removes both, the selection and the selected text. Use selectionText() to get the selected text and setSelection() to specify the selected text range. The signal selectionChanged() is emitted whenever the selection changed.
Cursor Positions
A view has one Cursor which represents a line/column tuple. Two different kinds of cursor positions are supported: first is the real cursor position where a tab character only counts one character. Second is the virtual cursor position, where a tab character counts as many spaces as defined. Get the real position with cursorPosition() and the virtual position with cursorPositionVirtual(). Set the real cursor position with setCursorPosition(). The signal cursorPositionChanged() is emitted whenever the cursor position changed.
Screen coordinates of the current text cursor position in pixels are obtained through cursorPositionCoordinates(). Further conversion of screen pixel coordinates and text cursor positions are provided by cursorToCoordinate() and coordinatesToCursor().
Mouse Tracking
It is possible to get notified via the signal mousePositionChanged() for mouse move events, if mouseTrackingEnabled() returns true. Mouse tracking can be turned on/off by calling setMouseTrackingEnabled(). If an editor implementation does not support mouse tracking, mouseTrackingEnabled() will always return false.
Input/View Modes
A view supports several input modes. Common input modes are NormalInputMode and ViInputMode. Which input modes the editor supports depends on the implementation. The getter viewInputMode() returns enum \InputMode representing the current mode.
Input modes can have their own view modes. In case of default NormalInputMode those are NormalModeInsert and NormalModeOverwrite. You can use viewMode() getter to obtain those.
For viewMode() and viewInputMode() there are also variants with Human suffix, which returns the human readable representation (i18n) usable for displaying in user interface.
Whenever the input/view mode changes the signals viewInputModeChanged()/viewModeChanged() are emitted.
View Config
Config provides methods to access and modify the low level config information for a given View KTextEditor::View has support for the following keys:
- line-numbers [bool], show/hide line numbers
- icon-bar [bool], show/hide icon bar
- folding-bar [bool], show/hide the folding bar
- folding-preview [bool], enable/disable folding preview when mouse hovers on folded region
- dynamic-word-wrap [bool], enable/disable dynamic word wrap
- background-color [QColor], read/set the default background color
- selection-color [QColor], read/set the default color for selections
- search-highlight-color [QColor], read/set the background color for search
- replace-highlight-color [QColor], read/set the background color for replaces
- default-mark-type [uint], read/set the default mark type
- allow-mark-menu [bool], enable/disable the menu shown when right clicking on the left gutter. When disabled, click on the gutter will always set or clear the mark of default type.
- icon-border-color [QColor] read/set the icon border color (on the left, with the line numbers)
- folding-marker-color [QColor] read/set folding marker colors (in the icon border)
- line-number-color [QColor] read/set line number colors (in the icon border)
- current-line-number-color [QColor] read/set current line number color (in the icon border)
- modification-markers [bool] read/set whether the modification markers are shown
- word-count [bool] enable/disable the counting of words and characters in the statusbar
- line-count [bool] show/hide the total number of lines in the status bar (
- Since
- 5.66)
- scrollbar-minimap [bool] enable/disable scrollbar minimap
- scrollbar-preview [bool] enable/disable scrollbar text preview on hover
- font [QFont] change the font
- theme [QString] change the theme
- word-completion-minimal-word-length [int] minimal word length to trigger word completion
- enter-to-insert-completion [bool] enable/disable whether pressing enter inserts completion
You can retrieve the value of a config key using configValue() and set the value for a config key using setConfigValue().
Annotation Interface
The Annotation Interface allows to do these things:
- (1) show/hide the annotation border along with the possibility to add actions into its context menu.
- (2) set a separate AnnotationModel for the View: Note that this interface inherits the AnnotationInterface.
- (3) set a custom AbstractAnnotationItemDelegate for the View.
For a more detailed explanation about whether you want to set a custom delegate for rendering the annotations, read the detailed documentation about the AbstractAnnotationItemDelegate.
Inline Notes
The inline notes interface provides a way to render arbitrary things in the text. The text layout of the line is adapted to create space for the note. Possible applications include showing a name of a function parameter in a function call or rendering a square with a color preview next to CSS color property.
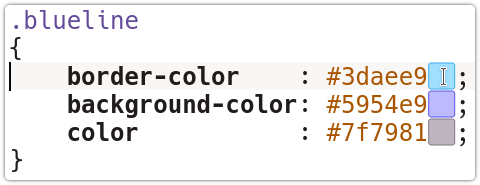
To register as inline note provider, call registerInlineNoteProvider() with an instance that inherits InlineNoteProvider. Finally, make sure you remove your inline note provider by calling unregisterInlineNoteProvider().
Introduction
The text hint interface provides a way to show tool tips for text located under the mouse. Possible applications include showing a value of a variable when debugging an application, or showing a complete path of an include directive.
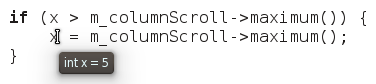
To register as text hint provider, call registerTextHintProvider() with an instance that inherits TextHintProvider. Finally, make sure you remove your text hint provider by calling unregisterTextHintProvider().
Text hints are shown after the user hovers with the mouse for a delay of textHintDelay() milliseconds over the same word. To change the delay, call setTextHintDelay().
Completion Interface
The Completion Interface is designed to provide code completion functionality for a KTextEditor::View. This interface provides the basic mechanisms to display a list of completions, update this list according to user input, and allow the user to select a completion.
Essentially, this provides an item view for the available completions. In order to use this interface, you will need to implement a CodeCompletionModel that generates the relevant completions given the current input.
More information about interfaces for the view can be found in View Extension Interfaces.
- See also
- KTextEditor::Document, KXMLGUIClient
Member Enumeration Documentation
◆ InputMode
◆ LineType
◆ ViewMode
Constructor & Destructor Documentation
◆ View()
|
protected |
Constructor.
Create a view attached to the widget parent
.
Pass it the internal implementation to store a d-pointer.
- Parameters
-
impl d-pointer to use parent parent widget
- See also
- Document::createView()
Definition at line 222 of file ktexteditor.cpp.
◆ ~View()
|
overridedefault |
Virtual destructor.
Member Function Documentation
◆ abortCompletion()
|
pure virtual |
Abort the currently displayed code completion without executing any currently selected completion.
This is safe, even when the completion box is not currently active.
- See also
- isCompletionActive()
◆ annotationActivated
|
signal |
This signal is emitted when an entry on the annotation border was activated, for example by clicking or double-clicking it.
This follows the KDE wide setting for activation via click or double-clcik
- Parameters
-
view the view to which the activated border belongs to line the document line that the activated position belongs to
◆ annotationBorderVisibilityChanged
|
signal |
This signal is emitted when the annotation border is shown or hidden.
- Parameters
-
view the view to which the border belongs to visible the current visibility state
◆ annotationContextMenuAboutToShow
|
signal |
This signal is emitted before a context menu is shown on the annotation border for the given line and view.
- Note
- Kate Part implementation detail: In Kate Part, the menu has an entry to hide the annotation border.
- Parameters
-
view the view that the annotation border belongs to menu the context menu that will be shown line the annotated line for which the context menu is shown
◆ annotationItemDelegate()
|
pure virtual |
Returns the currently used AbstractAnnotationItemDelegate.
- Returns
- the current AbstractAnnotationItemDelegate
- Since
- 6.0
◆ annotationModel()
|
pure virtual |
returns the currently set AnnotationModel or 0 if there's none set
- Returns
- the current AnnotationModel
◆ blockSelection()
|
pure virtual |
Get the status of the selection mode.
true indicates that block selection mode is on. If this is true, selections applied via the SelectionInterface are handled as block selections and the Copy&Paste functions work on rectangular blocks of text rather than normal.
- Returns
- true, if block selection mode is enabled, otherwise false
- See also
- setBlockSelection()
◆ codeCompletionModels()
|
pure virtual |
Obtain the list of registered code completion models.
- Returns
- a list of a models that are currently registered
◆ configChanged
|
signal |
This signal is emitted whenever the current view configuration is changed.
- Parameters
-
view the view which's config has changed
- Since
- 5.79
◆ configKeys()
|
pure virtual |
View Config.
Get a list of all available keys.
◆ configValue()
Get a value for the key
.
◆ contextMenu()
|
pure virtual |
Get the context menu for this view.
The return value can be NULL if no context menu object was set and kxmlgui is not initialized yet. If there is no user set menu, the kxmlgui menu is returned. Do not delete this menu, if if it is the xmlgui menu.
- Returns
- context menu object
- See also
- setContextMenu()
◆ contextMenuAboutToShow
|
signal |
Signal which is emitted immediately prior to showing the current context menu.
◆ coordinatesToCursor()
|
pure virtual |
Get the text-cursor in the document from the screen coordinates, relative to the view widget.
To map a cursor to pixel coordinates (the reverse transformation) use cursorToCoordinate().
- Parameters
-
coord coordinates relative to the view widget
- Returns
- cursor in the View, that points onto the character under the given coordinate. May be KTextEditor::Cursor::invalid().
◆ cursorPosition()
|
pure virtual |
Get the view's current cursor position.
A TAB character is handled as only one character.
- Returns
- current cursor position
- See also
- setCursorPosition()
◆ cursorPositionChanged
|
signal |
This signal is emitted whenever the view's
cursor position changed.
- Parameters
-
view view which emitted the signal newPosition new position of the cursor (Kate will pass the real cursor position, not the virtual)
- See also
- cursorPosition(), cursorPositionVirtual()
◆ cursorPositionCoordinates()
|
pure virtual |
Get the screen coordinates (x, y) of the cursor position in pixels.
The returned coordinates are relative to the View such that (0, 0) represents tht top-left corner of the View.
If global screen coordinates are required, e.g. for showing a QToolTip, convert the view coordinates to global screen coordinates as follows:
- Returns
- cursor screen coordinates
◆ cursorPositions()
QList< KTextEditor::Cursor > View::cursorPositions | ( | ) | const |
Get the view's current cursor positions.
A TAB character is handled as only one character.
The returned vector contains the primary cursor as first element.
- Since
- 5.95
- Returns
- all currently existing cursors
Definition at line 189 of file ktexteditor.cpp.
◆ cursorPositionVirtual()
|
pure virtual |
Get the current virtual cursor position, virtual means the tabulator character (TAB) counts multiple characters, as configured by the user (e.g.
one TAB is 8 spaces). The virtual cursor position provides access to the user visible values of the current cursor position.
- Returns
- virtual cursor position
- See also
- cursorPosition()
◆ cursorToCoordinate()
|
pure virtual |
Get the screen coordinates (x, y) of the supplied cursor relative to the view widget in pixels.
Thus, (0, 0) represents the top left hand of the view widget.
To map pixel coordinates to a Cursor position (the reverse transformation) use coordinatesToCursor().
- Parameters
-
cursor cursor to determine coordinate for.
- Returns
- cursor screen coordinates relative to the view widget
◆ defaultContextMenu()
Populate menu with default text editor actions.
If menu is null, a menu will be created with the view as its parent.
- Note
- to use this menu, you will next need to call setContextMenu(), as this does not assign the new context menu.
- Warning
- This contains only basic options from the editor component (katepart). Plugins are
not
merged/integrated into it! If you want to be a better citizen and take full advantage of KTextEditor plugins do something like:KXMLGUIClient* client = view;// search parent XmlGuiClientwhile (client->parentClient()) {client = client->parentClient();}if (client->factory()) {for (QWidget *w : menuContainers) {if (w->objectName() == "ktexteditor_popup") {// do something with the menu (ie adding an onshow handler)break;}}}KXMLGUIClient * parentClient() constKXMLGUIClient()KXMLGUIFactory * factory() const - or simply use the aboutToShow, aboutToHide signals !!!!!
- Parameters
-
menu the menu to be populated, or null to create a new menu.
- Returns
- the menu, whether created or passed initially
◆ defaultStyleAttribute()
|
pure virtual |
Returns the attribute for the default style defaultStyle
.
- Parameters
-
defaultStyle default style to get the attribute for
- See also
- KTextEditor::Attribute
◆ displayRangeChanged
|
signal |
This signal is emitted whenever the displayed range changes.
- Since
- 6.8
◆ document()
|
pure virtual |
Get the view's document, that means the view is a view of the returned document.
- Returns
- the view's document
◆ firstDisplayedLine()
Get the first displayed line in the view.
- Note
- If code is folded, many hundred lines can be between firstDisplayedLine() and lastDisplayedLine().
- Parameters
-
lineType if RealLine (the default), it returns the real line number accounting for folded regions. In that case it walks over all folded regions O(n) for n == number of folded ranges
- Returns
- the first displayed line
- See also
- lastDisplayedLine()
- Since
- 5.33
Definition at line 398 of file ktexteditor.cpp.
◆ focusIn
|
signal |
This signal is emitted whenever the view
gets the focus.
- Parameters
-
view view which gets focus
- See also
- focusOut()
◆ focusOut
|
signal |
This signal is emitted whenever the view
loses the focus.
- Parameters
-
view view which lost focus
- See also
- focusIn()
◆ forceCompletion()
|
pure virtual |
Force execution of the currently selected completion, and hide the code completion box.
◆ horizontalScrollBar()
|
pure virtual |
- Returns
- The horizontal scrollbar of this view
- Since
- 6.0
◆ horizontalScrollPositionChanged
|
signal |
This signal should be emitted whenever the view
is scrolled horizontally.
- Parameters
-
view view which emitted the signal
◆ insertTemplate()
bool View::insertTemplate | ( | KTextEditor::Cursor | insertPosition, |
const QString & | templateString, | ||
const QString & | script = QString() ) |
Insert a template into the document.
The template can have editable fields which can be filled by the user. You can create editable fields with ${fieldname}; multiple fields with the same name will have their contents synchronized automatically, and only the first one is editable in this case. Fields can have a default value specified by writing ${fieldname=default}. Note that ‘default’ is a JavaScript expression and strings need to be quoted. You can also provide a piece of JavaScript for more complex logic. To create a field which provides text based on a JS function call and the values of the other, editable fields, use the ${func()} syntax. func() must be a callable object defined in script
. You can pass arguments to the function by just writing any constant expression or a field name.
- Parameters
-
insertPosition where to insert the template templateString template to insert using the above syntax script script with functions which can be used in templateScript
- Returns
- true on success, false if insertion failed (e.g. read-only mode)
Definition at line 174 of file ktexteditor.cpp.
◆ insertText()
|
virtual |
This is a convenience function which inserts text
at the view's current cursor position.
You do not necessarily need to reimplement it, except you want to do some special things.
- Parameters
-
text Text to be inserted
- Returns
- true on success of insertion, otherwise false
- See also
- textInserted()
Definition at line 148 of file ktexteditor.cpp.
◆ isAnnotationBorderVisible()
|
pure virtual |
Checks whether the View's annotation border is visible.
◆ isAutomaticInvocationEnabled()
|
pure virtual |
Determine the status of automatic code completion invocation.
◆ isCompletionActive()
|
pure virtual |
Completion.
Query whether the code completion box is currently displayed.
◆ isStatusBarEnabled()
bool View::isStatusBarEnabled | ( | ) | const |
Is the status bar enabled?
- Returns
- status bar enabled?
Definition at line 157 of file ktexteditor.cpp.
◆ lastDisplayedLine()
Get the last displayed line in the view.
- Note
- If code is folded, many hundred lines can be between firstDisplayedLine() and lastDisplayedLine().
- Parameters
-
lineType if RealLine (the default), it returns the real line number accounting for folded regions. In that case it walks over all folded regions O(n) for n == number of folded ranges
- Returns
- the last displayed line
- See also
- firstDisplayedLine()
- Since
- 5.33
Definition at line 403 of file ktexteditor.cpp.
◆ lineAttributes()
|
pure virtual |
Get the list of AttributeBlocks for a given line
in the document.
- Returns
- list of AttributeBlocks for given
line
.
◆ mainWindow()
|
pure virtual |
Get the view's main window, if any.
- Returns
- the view's main window, will always return at least some non-nullptr dummy interface
◆ maxScrollPosition()
KTextEditor::Cursor View::maxScrollPosition | ( | ) | const |
Get the cursor corresponding to the maximum position the view can vertically scroll to.
- Returns
- cursor position of the maximum vertical scroll position.
- Since
- 5.33
Definition at line 393 of file ktexteditor.cpp.
◆ mousePositionChanged
|
signal |
This signal is emitted whenever the position of the mouse changes over this view.
If the mouse moves off the view, an invalid cursor position should be emitted, i.e. Cursor::invalid().
- Note
- If mouseTrackingEnabled() returns false, this signal is never emitted.
- Parameters
-
view view which emitted the signal newPosition new position of the mouse or Cursor::invalid(), if the mouse moved out of the view
.
- See also
- mouseTrackingEnabled()
◆ mouseTrackingEnabled()
|
pure virtual |
Check, whether mouse tracking is enabled.
Mouse tracking is required to have the signal mousePositionChanged() emitted.
- Returns
- true, if mouse tracking is enabled, otherwise false
◆ print()
|
pure virtual |
Print the document.
This should result in showing the print dialog.
- Returns
- true if document was printed
◆ printPreview()
|
pure virtual |
Shows the print preview dialog/.
◆ readSessionConfig()
|
pure virtual |
Read session settings from the given config
.
Known flags: none atm
- Parameters
-
config read the session settings from this KConfigGroup flags additional flags
- See also
- writeSessionConfig()
◆ registerCompletionModel()
|
pure virtual |
Register a new code completion model
.
- Parameters
-
model new completion model
- See also
- unregisterCompletionModel()
◆ registerInlineNoteProvider()
|
pure virtual |
Inline Note.
Register the inline note provider provider
.
Whenever a line is painted, the provider
will be queried for notes that should be painted in it. When the provider is about to be destroyed, make sure to call unregisterInlineNoteProvider() to avoid a dangling pointer.
- Parameters
-
provider inline note provider
◆ registerTextHintProvider()
|
pure virtual |
Text Hint.
Register the text hint provider provider
.
Whenever the user hovers over text, provider
will be asked for a text hint. When the provider is about to be destroyed, make sure to call unregisterTextHintProvider() to avoid a dangling pointer.
- Parameters
-
provider text hint provider
◆ removeSelection()
|
pure virtual |
Remove the view's current selection, without deleting the selected text.
- Returns
- true on success, otherwise false
- See also
- removeSelectionText()
◆ removeSelectionText()
|
pure virtual |
Remove the view's current selection including the selected text.
- Returns
- true on success, otherwise false
- See also
- removeSelection()
◆ selection()
|
pure virtual |
Query the view whether it has selected text, i.e.
whether a selection exists.
- Returns
- true if a text selection exists, otherwise false
- See also
- setSelection(), selectionRange()
◆ selectionChanged
|
signal |
This signal is emitted whenever the view's
selection changes.
- Note
- If the mode switches from block selection to normal selection or vice versa this signal should also be emitted.
- Parameters
-
view view in which the selection changed
- See also
- selection(), selectionRange(), selectionText()
◆ selectionRange()
|
pure virtual |
Get the range occupied by the current selection.
- Returns
- selection range, valid only if a selection currently exists.
- See also
- setSelection()
◆ selectionRanges()
QList< KTextEditor::Range > View::selectionRanges | ( | ) | const |
Get the ranges occupied by the current selections.
- Returns
- selection ranges, valid only if a selection currently exists.
- See also
- setSelections()
- Since
- 5.95
Definition at line 199 of file ktexteditor.cpp.
◆ selectionText()
|
pure virtual |
◆ setAnnotationBorderVisible()
|
pure virtual |
This function can be used to show or hide the annotation border The annotation border is hidden by default.
- Parameters
-
visible if true the annotation border is shown, otherwise hidden
◆ setAnnotationItemDelegate()
|
pure virtual |
Sets the AbstractAnnotationItemDelegate for this view and the model to provide custom rendering of annotation information for each line.
Ownership is not transferred.
- Parameters
-
delegate the new AbstractAnnotationItemDelegate, or nullptr
to reset to the default delegate
- Since
- 6.0
◆ setAnnotationModel()
|
pure virtual |
View Annotation Interface.
Sets a new AnnotationModel for this document to provide annotation information for each line.
- Parameters
-
model the new AnnotationModel
◆ setAnnotationUniformItemSizes()
|
pure virtual |
This function can be used to declare whether it is known that the annotation items rendered by the set delegate all have the same size.
This enables the view to do some optimizations for performance purposes.
By default the value of this property is false
.
- Parameters
-
uniformItemSizes if true
the annotation items are considered to all have the same size
- Since
- 6.0
◆ setAutomaticInvocationEnabled()
|
pure virtual |
Enable or disable automatic code completion invocation.
◆ setBlockSelection()
|
pure virtual |
Set block selection mode to state on
.
- Parameters
-
on if true, block selection mode is turned on, otherwise off
- Returns
- true on success, otherwise false
- See also
- blockSelection()
◆ setConfigValue()
|
pure virtual |
Set a the key's
value to value
.
◆ setContextMenu()
|
pure virtual |
Set a context menu for this view to menu
.
- Note
- any previously assigned menu is not deleted. If you are finished with the previous menu, you may delete it.
- Warning
- Use this with care! Plugin xml gui clients are not merged into this menu!
- !!!!!! DON'T USE THIS FUNCTION, UNLESS YOU ARE SURE YOU DON'T WANT PLUGINS TO WORK !!!!!!
- Parameters
-
menu new context menu object for this view
- See also
- contextMenu()
◆ setCursorPosition()
|
pure virtual |
Set the view's new cursor to position
.
A TAB character is handled as only on character.
- Parameters
-
position new cursor position
- Returns
- true on success, otherwise false
- See also
- cursorPosition()
◆ setCursorPositions()
void View::setCursorPositions | ( | const QList< KTextEditor::Cursor > & | positions | ) |
Set the view's new cursors to positions
.
A TAB character is handled as only on character.
This allows to create multiple cursors in this view.
The first passed position will be used for the primary cursor just like if you would call setCursorPosition.
- Parameters
-
positions new cursor positions
- See also
- cursorPositions()
- Since
- 5.95
Definition at line 184 of file ktexteditor.cpp.
◆ setHorizontalScrollPosition()
void View::setHorizontalScrollPosition | ( | int | x | ) |
Horizontally scroll view to position.
- Parameters
-
x the pixel position to scroll to.
- Since
- 5.33
Definition at line 388 of file ktexteditor.cpp.
◆ setMouseTrackingEnabled()
|
pure virtual |
Try to enable or disable mouse tracking according to enable
.
The return value contains the state of mouse tracking after the request. Mouse tracking is required to have the mousePositionChanged() signal emitted.
- Note
- Implementation Notes: An implementation is not forced to support this, and should always return false if it does not have support.
- Parameters
-
enable if true, try to enable mouse tracking, otherwise disable it.
- Returns
- the current state of mouse tracking
◆ setScrollPosition()
void View::setScrollPosition | ( | KTextEditor::Cursor | cursor | ) |
Scroll view to cursor.
- Parameters
-
cursor the cursor position to scroll to.
- Since
- 5.33
Definition at line 383 of file ktexteditor.cpp.
◆ setSelection()
|
pure virtual |
Set the view's selection to the range selection
.
The old selection will be discarded.
- Parameters
-
range the range of the new selection
- Returns
- true on success, otherwise false (e.g. when the cursor range is invalid)
- See also
- selectionRange(), selection()
◆ setSelections()
void View::setSelections | ( | const QList< KTextEditor::Range > & | ranges | ) |
Set the view's selection to the range selection
.
The old selection will be discarded.
- Parameters
-
ranges the ranges of the new selections
- See also
- selectionRanges(), selection()
- Since
- 5.95
Definition at line 194 of file ktexteditor.cpp.
◆ setStatusBarEnabled()
void View::setStatusBarEnabled | ( | bool | enable | ) |
Show/hide the status bar of the view.
Per default, the status bar is enabled.
- Parameters
-
enable should the status bar be enabled?
Definition at line 163 of file ktexteditor.cpp.
◆ setTextHintDelay()
|
pure virtual |
Set the text hint delay to delay
milliseconds.
The delay specifies the time the user needs to hover over the text before the tool tip is shown. Therefore, delay
should not be too large, a value of 500 milliseconds is recommended and set by default.
If delay
is <= 0, the default delay will be set.
- Parameters
-
delay tool tip delay in milliseconds
◆ setViewInputMode()
|
pure virtual |
Set the view's new input mode.
- Parameters
-
inputMode new InputMode value
- See also
- viewInputMode()
- Since
- 5.54
◆ startCompletion() [1/2]
|
pure virtual |
Invoke code completion over a given range, with specific models and invocation type.
- Parameters
-
models list of models to start. If this is an empty list, all registered models are started.
◆ startCompletion() [2/2]
|
pure virtual |
Invoke code completion over a given range, with a specific model.
◆ statusBarEnabledChanged
|
signal |
This signal is emitted whenever the status bar of view
is toggled.
- Parameters
-
enabled Whether the status bar is currently enabled or not
◆ textAreaRect()
QRect View::textAreaRect | ( | ) | const |
Get the view's text area rectangle excluding border, scrollbars, etc.
- Returns
- the view's text area rectangle
- Since
- 5.33
Definition at line 408 of file ktexteditor.cpp.
◆ textHintDelay()
|
pure virtual |
Get the text hint delay in milliseconds.
By default, the text hint delay is set to 500 milliseconds. It can be changed by calling setTextHintDelay()
.
◆ textInserted
|
signal |
This signal is emitted from view
whenever the users inserts text
at position
, that means the user typed/pasted text.
- Parameters
-
view view in which the text was inserted position position where the text was inserted text the text the user has typed into the editor
- See also
- insertText()
◆ theme()
KSyntaxHighlighting::Theme View::theme | ( | ) | const |
Get the current active theme of this view.
Might change during runtime, configChanged() will be emitted in that cases.
- Returns
- current active theme
- Since
- 5.79
Definition at line 179 of file ktexteditor.cpp.
◆ uniformAnnotationItemSizes()
|
pure virtual |
Checks whether the annotation items all have the same size.
- Since
- 6.0
◆ unregisterCompletionModel()
|
pure virtual |
Unregister a code completion model
.
- Parameters
-
model the model that should be unregistered
- See also
- registerCompletionModel()
◆ unregisterInlineNoteProvider()
|
pure virtual |
Unregister the inline note provider provider
.
- Parameters
-
provider inline note provider to unregister
◆ unregisterTextHintProvider()
|
pure virtual |
Unregister the text hint provider provider
.
- Parameters
-
provider text hint provider to unregister
◆ verticalScrollBar()
|
pure virtual |
- Returns
- The vertical scrollbar of this view
- Since
- 6.0
◆ verticalScrollPositionChanged
|
signal |
This signal should be emitted whenever the view
is scrolled vertically.
- Parameters
-
view view which emitted the signal newPos the new scroll position
◆ viewInputMode()
|
pure virtual |
Get the view's current input mode.
The current mode can be \NormalInputMode and \ViInputMode. For human translated version
- See also
- viewInputModeHuman.
- Returns
- the current input mode of this view
- See also
- viewInputModeChanged()
◆ viewInputModeChanged
|
signal |
This signal is emitted whenever the view's
input mode
changes.
- Parameters
-
view view which changed its input mode mode new input mode
- See also
- viewInputMode()
◆ viewInputModeHuman()
|
pure virtual |
Get the view's current input mode in human readable form.
The string should be translated (i18n). For id like version
- See also
- viewInputMode
- Returns
- the current input mode of this view in human readable form
◆ viewMode()
|
pure virtual |
Get the current view mode/state.
This can be used to detect the view's current mode. For example \NormalInputMode, \ViInputMode or whatever other input modes are supported.
- See also
- viewModeHuman() for translated version.
- Returns
- current view mode/state
- See also
- viewModeChanged()
◆ viewModeChanged
|
signal |
This signal is emitted whenever the view mode of view
changes.
- Parameters
-
view the view which changed its mode mode new view mode
- See also
- viewMode()
◆ viewModeHuman()
|
pure virtual |
Get the current view mode state.
This can be used to visually indicate the view's current mode, for example INSERT mode, OVERWRITE mode or COMMAND mode - or whatever other edit modes are supported. The string should be translated (i18n), as this is a user aimed representation of the view state, which should be shown in the GUI, for example in the status bar. This string may be rich-text.
- Returns
- Human-readable version of the view mode state
- See also
- viewModeChanged()
◆ writeSessionConfig()
|
pure virtual |
Write session settings to the config
.
See readSessionConfig() for more details.
- Parameters
-
config write the session settings to this KConfigGroup flags additional flags
- See also
- readSessionConfig()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:16:21 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.