KTextEditor::Plugin
#include <KTextEditor/Plugin>
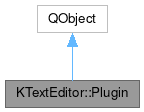
Public Member Functions | |
Plugin (QObject *parent) | |
~Plugin () override | |
virtual ConfigPage * | configPage (int number, QWidget *parent) |
virtual int | configPages () const |
virtual QObject * | createView (KTextEditor::MainWindow *mainWindow)=0 |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
KTextEditor Plugin interface.
Topics:
Introduction
The Plugin class provides methods to create loadable plugins for the KTextEditor framework. The Plugin class itself a function createView() that is called for each MainWindow. In createView(), the plugin is responsible to create tool views through MainWindow::createToolView(), hook itself into the menus and toolbars through KXMLGuiClient, and attach itself to Views or Documents.
Plugin Config Pages
If your plugin needs config pages, override the functions configPages() and configPage() in your plugin as follows:
The host application will call configPage() for each config page.
Session Management
As an extension a Plugin can implement the SessionConfigInterface. This interface provides functions to read and write session related settings. If you have session dependent data additionally derive your Plugin from this interface and implement the session related functions, for example:
Constructor & Destructor Documentation
◆ Plugin()
Plugin::Plugin | ( | QObject * | parent | ) |
Constructor.
Create a new application plugin.
- Parameters
-
parent parent object
Definition at line 231 of file ktexteditor.cpp.
◆ ~Plugin()
|
overridedefault |
Virtual destructor.
Member Function Documentation
◆ configPage()
|
virtual |
Get the config page with the number
, config pages from 0 to configPages()-1 are available if configPages() > 0.
- Parameters
-
number index of config page parent parent widget for config page
- Returns
- created config page or NULL, if the number is out of bounds, default implementation returns NULL
- See also
- configPages()
Definition at line 244 of file ktexteditor.cpp.
◆ configPages()
|
virtual |
Get the number of available config pages.
If a number < 1 is returned, it does not support config pages.
- Returns
- number of config pages, default implementation says 0
- See also
- configPage()
Definition at line 239 of file ktexteditor.cpp.
◆ createView()
|
pure virtual |
Create a new View for this plugin for the given KTextEditor::MainWindow.
This may be called arbitrary often by the application to create as much views as MainWindows exist. The application will take care to delete a view whenever a MainWindow is closed, so you do not need to handle deletion of the view yourself in the plugin.
- Note
- Session configuration: The host application will try to cast the returned QObject with
qobject_cast
into the SessionConfigInterface. This way, not only your Plugin, but also each Plugin view can have session related settings.
- Parameters
-
mainWindow the MainWindow for which a view should be created
- Returns
- the new created view or NULL
- See also
- SessionConfigInterface
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:11:28 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.