KTextEditor::MainWindow
#include <KTextEditor/MainWindow>
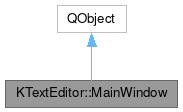
Public Types | |
enum | ToolViewPosition { Left = 0 , Right = 1 , Top = 2 , Bottom = 3 } |
Signals | |
void | pluginViewCreated (const QString &name, QObject *pluginView) |
void | pluginViewDeleted (const QString &name, QObject *pluginView) |
void | unhandledShortcutOverride (QEvent *e) |
void | viewChanged (KTextEditor::View *view) |
void | viewCreated (KTextEditor::View *view) |
void | widgetAdded (QWidget *widget) |
void | widgetRemoved (QWidget *widget) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class allows the application that embeds the KTextEditor component to allow it to access parts of its main window.
For example the component can get a place to show view bar widgets (e.g. search&replace, goto line, ...). This is useful to e.g. have one place inside the window to show such stuff even if the application allows the user to have multiple split views available per window.
The application must pass a pointer to the MainWindow object to the createView method on view creation and ensure that this main window stays valid for the complete lifetime of the view.
It must not reimplement this class but construct an instance and pass a pointer to a QObject that has the required slots to receive the requests.
Definition at line 46 of file mainwindow.h.
Member Enumeration Documentation
◆ ToolViewPosition
Toolview position.
A toolview can only be at one side at a time.
Enumerator | |
---|---|
Left | Left side. |
Right | Right side. |
Top | Top side. |
Bottom | Bottom side. |
Definition at line 218 of file mainwindow.h.
Constructor & Destructor Documentation
◆ MainWindow()
KTextEditor::MainWindow::MainWindow | ( | QObject * | parent | ) |
Construct an MainWindow wrapper object.
The passed parent is both the parent of this QObject and the receiver of all interface calls via invokeMethod.
- Parameters
-
parent object the calls are relayed to
Definition at line 19 of file mainwindow.cpp.
◆ ~MainWindow()
|
override |
Virtual Destructor.
Definition at line 25 of file mainwindow.cpp.
Member Function Documentation
◆ activateView()
KTextEditor::View * KTextEditor::MainWindow::activateView | ( | KTextEditor::Document * | document | ) |
Activate the view with the corresponding document
.
If none exist for this document, create one
- Parameters
-
document the document
- Returns
- activated view of this document, return nullptr if not possible
Definition at line 61 of file mainwindow.cpp.
◆ activateWidget()
void KTextEditor::MainWindow::activateWidget | ( | QWidget * | widget | ) |
activate widget
.
If the widget is not present in the window, it will be added to the window
- Parameters
-
widget the widget to activate
- Since
- 6.0
Definition at line 250 of file mainwindow.cpp.
◆ activeView()
KTextEditor::View * KTextEditor::MainWindow::activeView | ( | ) |
Access the active view.
- Returns
- active view, nullptr if not available
Definition at line 53 of file mainwindow.cpp.
◆ activeWidget()
QWidget * KTextEditor::MainWindow::activeWidget | ( | ) |
returns the currently active widget.
It can be a non-KTextEditorView widget or a KTextEditor::View
- Since
- 6.0
Definition at line 242 of file mainwindow.cpp.
◆ addWidget()
bool KTextEditor::MainWindow::addWidget | ( | QWidget * | widget | ) |
Add a widget to the main window.
This is useful to show non-KTextEditorView widgets in the main window. The host application should try to manage this like some KTextEditor::View (e.g. as a tab) and provide the means to close it.
- Parameters
-
widget widget to add
- Returns
- success, if false, the plugin needs to take care to show the widget itself, otherwise the main window will take ownership of the widget
- Since
- 5.98
Definition at line 218 of file mainwindow.cpp.
◆ addWidgetToViewBar()
void KTextEditor::MainWindow::addWidgetToViewBar | ( | KTextEditor::View * | view, |
QWidget * | bar ) |
Add a widget to the view bar.
- Parameters
-
view view for which the view bar is used bar bar widget, shall have the viewBarParent() as parent widget
Definition at line 130 of file mainwindow.cpp.
◆ closeSplitView()
bool KTextEditor::MainWindow::closeSplitView | ( | KTextEditor::View * | view | ) |
Close the split view that contains the given view.
- Parameters
-
view the view.
- Returns
- true if the split view was closed.
Definition at line 95 of file mainwindow.cpp.
◆ closeView()
bool KTextEditor::MainWindow::closeView | ( | KTextEditor::View * | view | ) |
Close selected view.
- Parameters
-
view the view
- Returns
- true if view was closed
Definition at line 81 of file mainwindow.cpp.
◆ createToolView()
QWidget * KTextEditor::MainWindow::createToolView | ( | KTextEditor::Plugin * | plugin, |
const QString & | identifier, | ||
KTextEditor::MainWindow::ToolViewPosition | pos, | ||
const QIcon & | icon, | ||
const QString & | text ) |
Create a new toolview with unique identifier
at side pos
with icon
and caption text
.
Use the returned widget to embedd your widgets.
- Parameters
-
plugin which owns this tool view identifier unique identifier for this toolview pos position for the toolview, if we are in session restore, this is only a preference icon icon to use in the sidebar for the toolview text translated text (i18n()) to use in addition to icon
- Returns
- created toolview on success, otherwise NULL
Definition at line 148 of file mainwindow.cpp.
◆ createViewBar()
QWidget * KTextEditor::MainWindow::createViewBar | ( | KTextEditor::View * | view | ) |
Try to create a view bar for the given view.
- Parameters
-
view view for which we want an view bar
- Returns
- suitable widget that can host view bars widgets or nullptr
Definition at line 116 of file mainwindow.cpp.
◆ deleteViewBar()
void KTextEditor::MainWindow::deleteViewBar | ( | KTextEditor::View * | view | ) |
Delete the view bar for the given view.
- Parameters
-
view view for which we want an view bar
Definition at line 124 of file mainwindow.cpp.
◆ guiFactory()
KXMLGUIFactory * KTextEditor::MainWindow::guiFactory | ( | ) |
Accessor to the XMLGUIFactory.
- Returns
- the mainwindow's KXMLGUIFactory, nullptr if not available
Definition at line 37 of file mainwindow.cpp.
◆ hideToolView()
bool KTextEditor::MainWindow::hideToolView | ( | QWidget * | widget | ) |
Hide the toolview widget
.
- Parameters
-
widget the toolview to hide, where the widget was constructed by createToolView().
- Returns
- true on success, otherwise false
Definition at line 189 of file mainwindow.cpp.
◆ hideViewBar()
void KTextEditor::MainWindow::hideViewBar | ( | KTextEditor::View * | view | ) |
Hide the view bar for the given view.
- Parameters
-
view view for which the view bar is used
Definition at line 142 of file mainwindow.cpp.
◆ moveToolView()
bool KTextEditor::MainWindow::moveToolView | ( | QWidget * | widget, |
KTextEditor::MainWindow::ToolViewPosition | pos ) |
Move the toolview widget
to position pos
.
- Parameters
-
widget the toolview to move, where the widget was constructed by createToolView(). pos new position to move widget to
- Returns
- true on success, otherwise false
Definition at line 168 of file mainwindow.cpp.
◆ openUrl()
KTextEditor::View * KTextEditor::MainWindow::openUrl | ( | const QUrl & | url, |
const QString & | encoding = QString() ) |
Open the document url
with the given encoding
.
- Parameters
-
url the document's url encoding the preferred encoding. If encoding is QString() the encoding will be guessed or the default encoding will be used.
- Returns
- a pointer to the created view for the new document, if a document with this url is already existing, its view will be activated, return nullptr if not possible
Definition at line 73 of file mainwindow.cpp.
◆ pluginView()
Get a plugin view for the plugin with with identifier name
.
- Parameters
-
name the plugin's name
- Returns
- pointer to the plugin view if a plugin with
name
is loaded and has a view for this mainwindow, otherwise NULL
Definition at line 210 of file mainwindow.cpp.
◆ pluginViewCreated
|
signal |
This signal is emitted when the view of some Plugin is created for this main window.
- Parameters
-
name name of plugin pluginView the new plugin view
◆ pluginViewDeleted
|
signal |
This signal is emitted when the view of some Plugin got deleted.
- Warning
- Do not access the data referenced by the pointer, it is already invalid. Use the pointer only to remove mappings in hash or maps
- Parameters
-
name name of plugin pluginView the deleted plugin view
◆ removeWidget()
bool KTextEditor::MainWindow::removeWidget | ( | QWidget * | widget | ) |
remove this widget
from this mainwindow.
The widget will be deleted afterwards
- Parameters
-
widget the widget to be removed
- Returns
- true on success
- Since
- 6.0
Definition at line 226 of file mainwindow.cpp.
◆ showMessage()
bool KTextEditor::MainWindow::showMessage | ( | const QVariantMap & | message | ) |
Display a message to the user.
The host application might show this inside a dedicated output view.
- Parameters
-
message incoming message we shall handle
- Returns
- true, if the host application was able to handle the message, else false
- Since
- 5.98
details of message format:
message text, will be trimmed before output
message["text"] = i18n("your cool message")
the text will be split in lines, all lines beside the first can be collapsed away
message type, we support at the moment
message["type"] = "Error" message["type"] = "Warning" message["type"] = "Info" message["type"] = "Log"
this is take from https://microsoft.github.io/language-server-protocol/specification#window_showMessage MessageType of LSP
will lead to appropriate icons/... in the output view
a message should have some category, like Git, LSP, ....
message["category"] = i18n(...)
will be used to allow the user to filter for
one can additionally provide a categoryIcon
message["categoryIcon"] = QIcon(...)
the categoryIcon icon QVariant must contain a QIcon, nothing else!
A string token can be passed to allow to replace messages already send out with new ones. That is useful for e.g. progress output
message["token"] = "yourmessagetoken"
Definition at line 256 of file mainwindow.cpp.
◆ showPluginConfigPage()
bool KTextEditor::MainWindow::showPluginConfigPage | ( | KTextEditor::Plugin * | plugin, |
int | page ) |
Shows the plugin's
config page.
The page
specifies which config page will be shown, see KTextEditor::Plugin::configPages().
- Returns
- true on success, otherwise false
- Since
- 5.63
Definition at line 197 of file mainwindow.cpp.
◆ showToolView()
bool KTextEditor::MainWindow::showToolView | ( | QWidget * | widget | ) |
Show the toolview widget
.
- Parameters
-
widget the toolview to show, where the widget was constructed by createToolView().
- Returns
- true on success, otherwise false
Definition at line 181 of file mainwindow.cpp.
◆ showViewBar()
void KTextEditor::MainWindow::showViewBar | ( | KTextEditor::View * | view | ) |
Show the view bar for the given view.
- Parameters
-
view view for which the view bar is used
Definition at line 136 of file mainwindow.cpp.
◆ splitView()
void KTextEditor::MainWindow::splitView | ( | Qt::Orientation | orientation | ) |
Split current view space according to orientation
.
- Parameters
-
orientation in which line split the view
Definition at line 89 of file mainwindow.cpp.
◆ unhandledShortcutOverride
|
signal |
This signal is emitted for every unhandled ShortcutOverride in the window.
- Parameters
-
e responsible event
◆ viewChanged
|
signal |
This signal is emitted whenever the active view changes.
- Parameters
-
view new active view
◆ viewCreated
|
signal |
This signal is emitted whenever a new view is created.
- Parameters
-
view view that was created
◆ views()
QList< KTextEditor::View * > KTextEditor::MainWindow::views | ( | ) |
Get a list of all views for this main window.
It is beneficial if the list is sorted by most recently used, as the library will e.g. try to use the most recent used url() by walking over this list for save and other such things.
- Returns
- all views, might be empty!
Definition at line 45 of file mainwindow.cpp.
◆ viewsInSameSplitView()
bool KTextEditor::MainWindow::viewsInSameSplitView | ( | KTextEditor::View * | view1, |
KTextEditor::View * | view2 ) |
- Returns
true
if the given viewsview1
andview2
share the same split view, false otherwise.
Definition at line 103 of file mainwindow.cpp.
◆ widgetAdded
|
signal |
The widget
was added to this window.
- Parameters
-
widget the widget that got added
- Since
- 6.0
◆ widgetRemoved
|
signal |
The widget
was removed from this window.
- Parameters
-
widget the widget that got removed
- Since
- 6.0
◆ widgets()
QWidgetList KTextEditor::MainWindow::widgets | ( | ) |
returns the list of non-KTextEditorView widgets in this main window.
- See also
- addWidget
- Since
- 6.0
Definition at line 234 of file mainwindow.cpp.
◆ window()
QWidget * KTextEditor::MainWindow::window | ( | ) |
Get the toplevel widget.
- Returns
- the real main window widget, nullptr if not available
Definition at line 29 of file mainwindow.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:22 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.