KTextEditor::CodeCompletionModel
#include <KTextEditor/CodeCompletionModel>
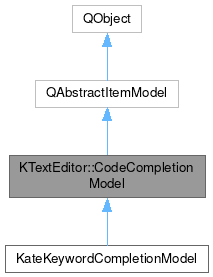
Public Types | |
enum | Columns { Prefix = 0 , Icon , Scope , Name , Arguments , Postfix } |
typedef QFlags< CompletionProperty > | CompletionProperties |
enum | CompletionProperty { NoProperty = 0x0 , FirstProperty = 0x1 , Public = 0x1 , Protected = 0x2 , Private = 0x4 , Static = 0x8 , Const = 0x10 , Namespace = 0x20 , Class = 0x40 , Struct = 0x80 , Union = 0x100 , Function = 0x200 , Variable = 0x400 , Enum = 0x800 , Template = 0x1000 , TypeAlias = 0x2000 , Virtual = 0x4000 , Override = 0x8000 , Inline = 0x10000 , Friend = 0x20000 , Signal = 0x40000 , Slot = 0x80000 , LocalScope = 0x100000 , NamespaceScope = 0x200000 , GlobalScope = 0x400000 , LastProperty = GlobalScope } |
enum | ExtraItemDataRoles { CompletionRole = Qt::UserRole , ScopeIndex , MatchQuality , SetMatchContext , HighlightingMethod , CustomHighlight , InheritanceDepth , IsExpandable , ExpandingWidget , ItemSelected , ArgumentHintDepth , BestMatchesCount , AccessibilityNext , AccessibilityPrevious , AccessibilityAccept , GroupRole , UnimportantItemRole , LastExtraItemDataRole } |
enum | HighlightMethod { NoHighlighting = 0x0 , InternalHighlighting = 0x1 , CustomHighlighting = 0x2 } |
typedef QFlags< HighlightMethod > | HighlightMethods |
enum | InvocationType { AutomaticInvocation , UserInvocation , ManualInvocation } |
![]() | |
enum | CheckIndexOption |
enum | LayoutChangeHint |
Signals | |
void | hasGroupsChanged (KTextEditor::CodeCompletionModel *model, bool hasGroups) |
void | waitForReset () |
Public Member Functions | |
CodeCompletionModel (QObject *parent) | |
int | columnCount (const QModelIndex &parent=QModelIndex()) const override |
virtual void | completionInvoked (KTextEditor::View *view, const KTextEditor::Range &range, InvocationType invocationType) |
virtual void | executeCompletionItem (KTextEditor::View *view, const Range &word, const QModelIndex &index) const |
bool | hasGroups () const |
QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const override |
QMap< int, QVariant > | itemData (const QModelIndex &index) const override |
QModelIndex | parent (const QModelIndex &index) const override |
int | rowCount (const QModelIndex &parent=QModelIndex()) const override |
void | setRowCount (int rowCount) |
![]() | |
QAbstractItemModel (QObject *parent) | |
virtual QModelIndex | buddy (const QModelIndex &index) const const |
virtual bool | canDropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const const |
virtual bool | canFetchMore (const QModelIndex &parent) const const |
bool | checkIndex (const QModelIndex &index, CheckIndexOptions options) const const |
virtual bool | clearItemData (const QModelIndex &index) |
void | columnsAboutToBeInserted (const QModelIndex &parent, int first, int last) |
void | columnsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | columnsInserted (const QModelIndex &parent, int first, int last) |
void | columnsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsRemoved (const QModelIndex &parent, int first, int last) |
virtual QVariant | data (const QModelIndex &index, int role) const const=0 |
void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight, const QList< int > &roles) |
virtual bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) |
virtual void | fetchMore (const QModelIndex &parent) |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const const |
virtual bool | hasChildren (const QModelIndex &parent) const const |
bool | hasIndex (int row, int column, const QModelIndex &parent) const const |
virtual QVariant | headerData (int section, Qt::Orientation orientation, int role) const const |
void | headerDataChanged (Qt::Orientation orientation, int first, int last) |
bool | insertColumn (int column, const QModelIndex &parent) |
virtual bool | insertColumns (int column, int count, const QModelIndex &parent) |
bool | insertRow (int row, const QModelIndex &parent) |
virtual bool | insertRows (int row, int count, const QModelIndex &parent) |
void | layoutAboutToBeChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | layoutChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
virtual QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits, Qt::MatchFlags flags) const const |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const const |
virtual QStringList | mimeTypes () const const |
void | modelAboutToBeReset () |
void | modelReset () |
bool | moveColumn (const QModelIndex &sourceParent, int sourceColumn, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveColumns (const QModelIndex &sourceParent, int sourceColumn, int count, const QModelIndex &destinationParent, int destinationChild) |
bool | moveRow (const QModelIndex &sourceParent, int sourceRow, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveRows (const QModelIndex &sourceParent, int sourceRow, int count, const QModelIndex &destinationParent, int destinationChild) |
virtual void | multiData (const QModelIndex &index, QModelRoleDataSpan roleDataSpan) const const |
bool | removeColumn (int column, const QModelIndex &parent) |
virtual bool | removeColumns (int column, int count, const QModelIndex &parent) |
bool | removeRow (int row, const QModelIndex &parent) |
virtual bool | removeRows (int row, int count, const QModelIndex &parent) |
virtual void | revert () |
virtual QHash< int, QByteArray > | roleNames () const const |
void | rowsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | rowsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | rowsInserted (const QModelIndex &parent, int first, int last) |
void | rowsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsRemoved (const QModelIndex &parent, int first, int last) |
virtual bool | setData (const QModelIndex &index, const QVariant &value, int role) |
virtual bool | setHeaderData (int section, Qt::Orientation orientation, const QVariant &value, int role) |
virtual bool | setItemData (const QModelIndex &index, const QMap< int, QVariant > &roles) |
virtual QModelIndex | sibling (int row, int column, const QModelIndex &index) const const |
virtual void | sort (int column, Qt::SortOrder order) |
virtual QSize | span (const QModelIndex &index) const const |
virtual bool | submit () |
virtual Qt::DropActions | supportedDragActions () const const |
virtual Qt::DropActions | supportedDropActions () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Attributes | |
static const int | ColumnCount = Postfix + 1 |
Protected Member Functions | |
void | setHasGroups (bool hasGroups) |
![]() | |
void | beginInsertColumns (const QModelIndex &parent, int first, int last) |
void | beginInsertRows (const QModelIndex &parent, int first, int last) |
bool | beginMoveColumns (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
bool | beginMoveRows (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
void | beginRemoveColumns (const QModelIndex &parent, int first, int last) |
void | beginRemoveRows (const QModelIndex &parent, int first, int last) |
void | beginResetModel () |
void | changePersistentIndex (const QModelIndex &from, const QModelIndex &to) |
void | changePersistentIndexList (const QModelIndexList &from, const QModelIndexList &to) |
QModelIndex | createIndex (int row, int column, const void *ptr) const const |
QModelIndex | createIndex (int row, int column, quintptr id) const const |
void | endInsertColumns () |
void | endInsertRows () |
void | endMoveColumns () |
void | endMoveRows () |
void | endRemoveColumns () |
void | endRemoveRows () |
void | endResetModel () |
QModelIndexList | persistentIndexList () const const |
virtual void | resetInternalData () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | CheckIndexOptions |
DoNotUseParent | |
HorizontalSortHint | |
IndexIsValid | |
NoLayoutChangeHint | |
NoOption | |
ParentIsInvalid | |
VerticalSortHint | |
![]() | |
typedef | QObjectList |
Detailed Description
An item model for providing code completion, and meta information for enhanced presentation.
Introduction
The CodeCompletionModel is the actual workhorse to provide code completions in a KTextEditor::View. It is meant to be used in conjunction with the View. The CodeCompletionModel is not meant to be used as is. Rather you need to implement a subclass of CodeCompletionModel to actually generate completions appropriate for your type of Document.
Implementing a CodeCompletionModel
The CodeCompletionModel is a QAbstractItemModel, and can be subclassed in the same way. It provides default implementations of several members, however, so in most cases (if your completions are essentially a non-hierarchical, flat list of matches) you will only need to overload few virtual functions.
Implementing a CodeCompletionModel for a flat list
For the simple case of a flat list of completions, you will need to:
- Implement completionInvoked() to actually generate/update the list of completion matches
- implement itemData() (or QAbstractItemModel::data()) to return the information that should be displayed for each match.
- use setRowCount() to reflect the number of matches.
Columns and roles
- Todo
- document the meaning and usage of the columns and roles used by the Completion Interface
Using the new CodeCompletionModel
To start using your KTextEditor::View Completion Interface.
ControllerInterface to get more control
To have more control over code completion implement CodeCompletionModelControllerInterface in your CodeCompletionModel.
Definition at line 67 of file codecompletionmodel.h.
Member Typedef Documentation
◆ CompletionProperties
Stores a combination of CompletionProperty values.
Definition at line 130 of file codecompletionmodel.h.
◆ HighlightMethods
Stores a combination of HighlightMethod values.
Definition at line 139 of file codecompletionmodel.h.
Member Enumeration Documentation
◆ Columns
Enumerator | |
---|---|
Icon | Icon representing the type of completion. We have a separate icon field so that names remain aligned where only some completions have icons, and so that they can be rearranged by the user. |
Definition at line 75 of file codecompletionmodel.h.
◆ CompletionProperty
- See also
- CompletionProperties
Definition at line 89 of file codecompletionmodel.h.
◆ ExtraItemDataRoles
Meta information is passed through extra {Qt::ItemDataRole}s.
This information should be returned when requested on the Name column.
Enumerator | |
---|---|
CompletionRole | The model should return a set of CompletionProperties. |
ScopeIndex | The model should return an index to the scope -1 represents no scope.
|
MatchQuality | If requested, your model should try to determine whether the completion in question is a suitable match for the context (ie. is accessible, exported, + returns the data type required). The returned data should ideally be matched against the argument-hint context set earlier by SetMatchContext. Return an integer value that should be positive if the completion is suitable, and zero if the completion is not suitable. The value should be between 0 an 10, where 10 means perfect match. Return QVariant::Invalid if you are unable to determine this. |
SetMatchContext | Is requested before MatchQuality(..) is requested. The item on which this is requested is an argument-hint item(
Feel free to ignore this, but ideally you should return QVariant::Invalid to make clear that your model does not support this. |
HighlightingMethod | Define which highlighting method will be used:
|
CustomHighlight | Allows an item to provide custom highlighting. Return a QList<QVariant> in the following format:
Repeat this triplet as many times as required, however each column must be >= the previous, and startColumn != endColumn. |
InheritanceDepth | Returns the inheritance depth of the completion. For example, a completion which comes from the base class would have depth 0, one from a parent class would have depth 1, one from that class' parent 2, etc. you can use this to symbolize the general distance of a completion-item from a user. It will be used for sorting. |
IsExpandable | This allows items in the completion-list to be expandable. If a model returns an QVariant bool value that evaluates to true, the completion-widget will draw a handle to expand the item, and will also make that action accessible through keyboard. |
ExpandingWidget | After a model returned true for a row on IsExpandable, the row may be expanded by the user. When this happens, ExpandingWidget is requested. The model may return two types of values: QWidget*: If the model returns a QVariant of type QWidget*, the code-completion takes over the given widget and embeds it into the completion-list under the completion-item. The widget will be automatically deleted at some point. The completion-widget will use the height of the widget as a hint for its preferred size, but it will resize the widget at will. QString: If the mode returns a QVariant of type QString, it will create a small html-widget showing the given html-code, and embed it into the completion-list under the completion-item.
|
ItemSelected | Whenever an item is selected, this will be requested from the underlying model. It may be used as a simple notification that the item was selected. Above that, the model may return a QString, which then should then contain html-code. A html-widget will then be displayed as a one- or two-liner under the currently selected item(it will be partially expanded) |
ArgumentHintDepth | Is this completion-item an argument-hint? The model should return an integral positive number if the item is an argument-hint, and QVariant() or 0 if it is not one. The returned depth-integer is important for sorting and matching. Example: "otherFunction(function1(function2(" all functions named function2 should have ArgumentHintDepth 1, all functions found for function1 should have ArgumentHintDepth 2, and all functions named otherFunction should have ArgumentHintDepth 3 Later, a completed item may then be matched with the first argument of function2, the return-type of function2 with the first argument-type of function1, and the return-type of function1 with the argument-type of otherFunction. If the model returns a positive value on this role for a row, the content will be treated specially:
Example: You are matching a function with signature "void test(int param1, int param2)", and you are matching the first argument. The model should then return: Prefix: "void test(" Name: "int param1" Suffix: ", int param2)" If you don't use the highlighting, matching, etc. you can also return the columns in the usual way. |
BestMatchesCount | This will be requested for each item to ask whether it should be included in computing a best-matches list. If you return a valid positive integer n here, the n best matches will be listed at top of the completion-list separately. This is expensive because all items of the whole completion-list will be tested for their matching-quality, with each of the level 1 argument-hints. For that reason the end-user should be able to disable this feature. |
AccessibilityNext | The following three enumeration-values are only used on expanded completion-list items that contain an expanding-widget(.
You can use them to allow the user to interact with the widget by keyboard. AccessibilityNext will be requested on an item if it is expanded, contains an expanding-widget, and the user triggers a special navigation short-cut to go to navigate to the next position within the expanding-widget(if applicable). Return QVariant(true) if the input was used. |
AccessibilityPrevious | AccessibilityPrevious will be requested on an item if it is expanded, contains an expanding-widget, and the user triggers a special navigation short-cut to go to navigate to the previous position within the expanding-widget(if applicable). Return QVariant(true) if the input was used. |
AccessibilityAccept | AccessibilityAccept will be requested on an item if it is expanded, contains an expanding-widget, and the user triggers a special shortcut to trigger the action associated with the position within the expanding-widget the user has navigated to using AccessibilityNext and AccessibilityPrevious. This should return QVariant(true) if an action was triggered, else QVariant(false) or QVariant(). |
GroupRole | Using this Role, it is possible to greatly optimize the time needed to process very long completion-lists. In the completion-list, the items are usually ordered by some properties like argument-hint depth, inheritance-depth and attributes. Kate usually has to query the completion-models for these values for each item in the completion-list in order to extract the argument-hints and correctly sort the completion-list. However, with a very long completion-list, only a very small fraction of the items is actually visible. By using a tree structure you can give the items in a grouped order to kate, so it does not need to look at each item and query data in order to initially show the completion-list. This is how it works:
The recommended grouping order is: Argument-hint depth, inheritance depth, attributes. This role can also be used to define completely custom groups, bypassing the editors builtin grouping:
|
UnimportantItemRole | Return a nonzero value here to enforce sorting the item at the end of the list. |
Definition at line 143 of file codecompletionmodel.h.
◆ HighlightMethod
- See also
- HighlightMethods
Definition at line 133 of file codecompletionmodel.h.
◆ InvocationType
enum KTextEditor::CodeCompletionModel::InvocationType |
Definition at line 364 of file codecompletionmodel.h.
Constructor & Destructor Documentation
◆ CodeCompletionModel()
|
explicit |
Definition at line 25 of file codecompletionmodel.cpp.
◆ ~CodeCompletionModel()
|
override |
Definition at line 31 of file codecompletionmodel.cpp.
Member Function Documentation
◆ columnCount()
|
overridevirtual |
Reimplemented from QAbstractItemModel::columnCount().
The default implementation returns ColumnCount for all indices.
Implements QAbstractItemModel.
Definition at line 36 of file codecompletionmodel.cpp.
◆ completionInvoked()
|
virtual |
This function is responsible to generating / updating the list of current completions.
The default implementation does nothing.
When implementing this function, remember to call setRowCount() (or implement rowCount()), and to generate the appropriate change notifications (for instance by calling QAbstractItemModel::reset()).
- Parameters
-
view The view to generate completions for range The range of text to generate completions for invocationType How the code completion was triggered
Reimplemented in KateKeywordCompletionModel.
Definition at line 83 of file codecompletionmodel.cpp.
◆ executeCompletionItem()
|
virtual |
This function is responsible for inserting a selected completion into the view.
The default implementation replaces the text that the completions were based on with the Qt::DisplayRole of the Name column of the given match.
- Parameters
-
view the view to insert the completion into word the Range that the completions are based on (what the user entered so far) index identifies the completion match to insert
Definition at line 90 of file codecompletionmodel.cpp.
◆ hasGroups()
bool CodeCompletionModel::hasGroups | ( | ) | const |
This function returns true if the model needs grouping, otherwise false.
The default is false if not changed via setHasGroups().
Definition at line 95 of file codecompletionmodel.cpp.
◆ hasGroupsChanged
|
signal |
Internal.
◆ index()
|
overridevirtual |
Reimplemented from QAbstractItemModel::index().
The default implementation returns a standard QModelIndex as long as the row and column are valid.
Implements QAbstractItemModel.
Reimplemented in KateKeywordCompletionModel.
Definition at line 41 of file codecompletionmodel.cpp.
◆ itemData()
|
overridevirtual |
Reimplemented from QAbstractItemModel::itemData().
The default implementation returns a map with the QAbstractItemModel::data() for all roles that are used by the CodeCompletionInterface. You will need to reimplement either this function or QAbstractItemModel::data() in your CodeCompletionModel.
Reimplemented from QAbstractItemModel.
Definition at line 50 of file codecompletionmodel.cpp.
◆ parent()
|
overridevirtual |
Reimplemented from QAbstractItemModel::parent().
The default implementation returns an invalid QModelIndex for all items. This is appropriate for non-hierarchical / flat lists of completions.
Implements QAbstractItemModel.
Reimplemented in KateKeywordCompletionModel.
Definition at line 64 of file codecompletionmodel.cpp.
◆ rowCount()
|
overridevirtual |
Reimplemented from QAbstractItemModel::rowCount().
The default implementation returns the value set by setRowCount() for invalid (toplevel) indices, and 0 for all other indices. This is appropriate for non-hierarchical / flat lists of completions
Implements QAbstractItemModel.
Reimplemented in KateKeywordCompletionModel.
Definition at line 74 of file codecompletionmodel.cpp.
◆ setHasGroups()
|
protected |
Definition at line 100 of file codecompletionmodel.cpp.
◆ setRowCount()
void CodeCompletionModel::setRowCount | ( | int | rowCount | ) |
Definition at line 69 of file codecompletionmodel.cpp.
◆ waitForReset
|
signal |
Emit this if the code-completion for this model was invoked, some time is needed in order to get the data, and the model is reset once the data is available.
This only has an effect if emitted from within completionInvoked(..).
This prevents the code-completion list from showing until this model is reset, so there is no annoying flashing in the user-interface resulting from other models supplying their data earlier.
- Note
- The implementation may choose to show the completion-list anyway after some timeout
- Warning
- If you emit this, you must also reset the model at some point, else the code-completion will be completely broken to the user. Consider that there may always be additional completion-models apart from yours.
- Since
- 4.3
Member Data Documentation
◆ ColumnCount
|
static |
Definition at line 86 of file codecompletionmodel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:55:40 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.