KTextEditor::CodeCompletionModelControllerInterface
#include <KTextEditor/CodeCompletionModelControllerInterface>
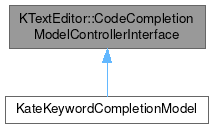
Public Types | |
enum | MatchReaction { None = 0 , HideListIfAutomaticInvocation = 1 , ForExtension = 0xffff } |
Public Member Functions | |
virtual void | aborted (View *view) |
virtual Range | completionRange (View *view, const Cursor &position) |
virtual QString | filterString (View *view, const Range &range, const Cursor &position) |
virtual MatchReaction | matchingItem (const QModelIndex &matched) |
virtual bool | shouldAbortCompletion (View *view, const Range &range, const QString ¤tCompletion) |
virtual bool | shouldExecute (const QModelIndex &selected, QChar inserted) |
virtual bool | shouldHideItemsWithEqualNames () const |
virtual bool | shouldStartCompletion (View *view, const QString &insertedText, bool userInsertion, const Cursor &position) |
virtual Range | updateCompletionRange (View *view, const Range &range) |
Detailed Description
Controller interface for a CodeCompletionModel.
The CodeCompletionModelControllerInterface gives an CodeCompletionModel better control over the completion.
By implementing methods defined in this interface you can:
- control when automatic completion should start (shouldStartCompletion())
- define a custom completion range (that will be replaced when the completion is executed) (completionRange())
- dynamically modify the completion range during completion (updateCompletionRange())
- specify the string used for filtering the completion (filterString())
- control when completion should stop (shouldAbortCompletion())
When the interface is not implemented, or no methods are overridden the default behaviour is used, which will be correct in many situations.
Implementing the Interface
To use this class implement it in your CodeCompletionModel.
- See also
- CodeCompletionModel
Definition at line 60 of file codecompletionmodelcontrollerinterface.h.
Member Enumeration Documentation
◆ MatchReaction
Enumerator | |
---|---|
ForExtension | If this is returned, the completion-list is hidden if it was invoked automatically. |
Definition at line 156 of file codecompletionmodelcontrollerinterface.h.
Constructor & Destructor Documentation
◆ CodeCompletionModelControllerInterface()
KTextEditor::CodeCompletionModelControllerInterface::CodeCompletionModelControllerInterface | ( | ) |
Definition at line 18 of file codecompletionmodelcontrollerinterface.cpp.
Member Function Documentation
◆ aborted()
|
virtual |
Notification that completion for this model has been aborted.
- Parameters
-
view The view in which the completion for this model was aborted
Definition at line 102 of file codecompletionmodelcontrollerinterface.cpp.
◆ completionRange()
|
virtual |
This function returns the completion range that will be used for the current completion.
This range will be used for filtering the completion list and will get replaced when executing the completion
The default implementation will work for most languages that don't have special chars in identifiers. Since 5.83 the default implementation takes into account the wordCompletionRemoveTail configuration option, if that option is enabled the whole word the cursor is inside is replaced with the completion, however if it's disabled only the text on the left of the cursor will be replaced with the completion.
- Parameters
-
view The view to generate completions for position Current cursor position
- Returns
- the completion range
Reimplemented in KateKeywordCompletionModel.
Definition at line 40 of file codecompletionmodelcontrollerinterface.cpp.
◆ filterString()
|
virtual |
This function returns the filter-text used for the current completion.
Can return an empty string to disable filtering.
The default implementation will return the text from range
start to the cursor position
.
- Parameters
-
view The view to generate completions for range The completion range position Current cursor position
- Returns
- the string used for filtering the completion list
Definition at line 86 of file codecompletionmodelcontrollerinterface.cpp.
◆ matchingItem()
|
virtual |
Called whenever an item in the completion-list perfectly matches the current filter text.
- Parameters
-
matched The index that is matched
- Returns
- Whether the completion-list should be hidden on this event. The default-implementation always returns HideListIfAutomaticInvocation
Reimplemented in KateKeywordCompletionModel.
Definition at line 114 of file codecompletionmodelcontrollerinterface.cpp.
◆ shouldAbortCompletion()
|
virtual |
This function decides if the completion should be aborted.
Called after every change to the range (eg. when user entered text)
The default implementation will return true when any special character was entered, or when the range is empty.
- Parameters
-
view The view to generate completions for range The completion range currentCompletion The text typed so far
- Returns
- true, if the completion should be aborted, otherwise false
Reimplemented in KateKeywordCompletionModel.
Definition at line 91 of file codecompletionmodelcontrollerinterface.cpp.
◆ shouldExecute()
|
virtual |
When an item within this model is currently selected in the completion-list, and the user inserted the given character, should the completion-item be executed?
This can be used to execute items from other inputs than the return-key. For example a function item could be executed by typing '(', or variable items by typing '.'.
- Parameters
-
selected The currently selected index inserted The character that was inserted by tue user
Definition at line 107 of file codecompletionmodelcontrollerinterface.cpp.
◆ shouldHideItemsWithEqualNames()
|
virtual |
When multiple completion models are used at the same time, it may happen that multiple models add items with the same name to the list.
This option allows to hide items from this completion model when another model with higher priority contains items with the same name.
- Returns
- Whether items of this completion model should be hidden if another completion model has items with the same name
Reimplemented in KateKeywordCompletionModel.
Definition at line 120 of file codecompletionmodelcontrollerinterface.cpp.
◆ shouldStartCompletion()
|
virtual |
This function decides if the automatic completion should be started when the user entered some text.
The default implementation will return true if the last character in insertedText
is a letter, a number, '.', '_' or '>'
- Parameters
-
view The view to generate completions for insertedText The text that was inserted by the user userInsertion Whether the text was inserted by the user using typing. If false, it may have been inserted for example by code-completion. position Current cursor position
- Returns
- true, if the completion should be started, otherwise false
Reimplemented in KateKeywordCompletionModel.
Definition at line 24 of file codecompletionmodelcontrollerinterface.cpp.
◆ updateCompletionRange()
|
virtual |
This function lets the CompletionModel dynamically modify the range.
Called after every change to the range (eg. when user entered text)
The default implementation does nothing.
- Parameters
-
view The view to generate completions for range Reference to the current range
- Returns
- the modified range
Definition at line 74 of file codecompletionmodelcontrollerinterface.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:22 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.