KXMLGUIClient
#include <KXMLGUIClient>
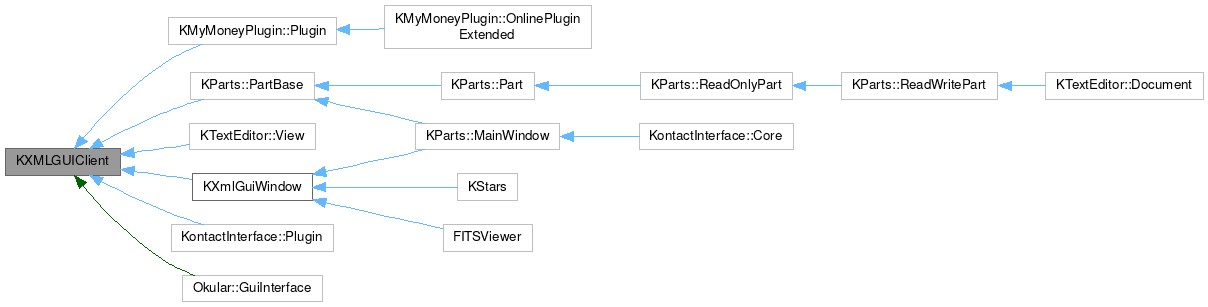
Public Types | |
enum | ReverseStateChange { StateNoReverse , StateReverse } |
Static Public Member Functions | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
static QString | findVersionNumber (const QString &xml) |
Protected Member Functions | |
void | loadStandardsXmlFile () |
virtual void | setComponentName (const QString &componentName, const QString &componentDisplayName) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Static Protected Member Functions | |
static QString | standardsXmlFileLocation () |
Detailed Description
A KXMLGUIClient can be used with KXMLGUIFactory to create a GUI from actions and an XML document, and can be dynamically merged with other KXMLGUIClients.
Definition at line 39 of file kxmlguiclient.h.
Member Enumeration Documentation
◆ ReverseStateChange
enum KXMLGUIClient::ReverseStateChange |
Definition at line 257 of file kxmlguiclient.h.
Constructor & Destructor Documentation
◆ KXMLGUIClient() [1/2]
KXMLGUIClient::KXMLGUIClient | ( | ) |
Constructs a KXMLGUIClient which can be used with a KXMLGUIFactory to create a GUI from actions and an XML document, and which can be dynamically merged with other KXMLGUIClients.
Definition at line 65 of file kxmlguiclient.cpp.
◆ KXMLGUIClient() [2/2]
|
explicit |
Constructs a KXMLGUIClient which can be used with a KXMLGUIFactory to create a GUI from actions and an XML document, and which can be dynamically merged with other KXMLGUIClients.
This constructor takes an additional parent
argument, which makes the client a child client of the parent.
Child clients are automatically added to the GUI if the parent is added.
Definition at line 70 of file kxmlguiclient.cpp.
◆ ~KXMLGUIClient()
|
virtual |
Destructs the KXMLGUIClient.
If the client was in a factory, the factory is NOT informed about the client being removed. This is a feature, it makes window destruction fast (the xmlgui is not updated for every client being deleted), but if you want to simply remove one client and to keep using the window, make sure to call factory->removeClient(client) before deleting the client.
Definition at line 78 of file kxmlguiclient.cpp.
Member Function Documentation
◆ action() [1/2]
|
virtual |
Retrieves an action for a given QDomElement.
The default implementation uses the "name" attribute to query the action object via the other action() method.
Definition at line 124 of file kxmlguiclient.cpp.
◆ action() [2/2]
Retrieves an action of the client by name.
If not found, it looks in its child clients. This method is provided for convenience, as it uses actionCollection() to get the action object.
- Since
- 6.0
Definition at line 101 of file kxmlguiclient.cpp.
◆ actionCollection()
|
virtual |
Retrieves the entire action collection for the GUI client.
Definition at line 115 of file kxmlguiclient.cpp.
◆ addStateActionDisabled()
Definition at line 715 of file kxmlguiclient.cpp.
◆ addStateActionEnabled()
Definition at line 705 of file kxmlguiclient.cpp.
◆ beginXMLPlug()
void KXMLGUIClient::beginXMLPlug | ( | QWidget * | w | ) |
Definition at line 756 of file kxmlguiclient.cpp.
◆ childClients()
QList< KXMLGUIClient * > KXMLGUIClient::childClients | ( | ) |
Retrieves a list of all child clients.
Definition at line 665 of file kxmlguiclient.cpp.
◆ clientBuilder()
KXMLGUIBuilder * KXMLGUIClient::clientBuilder | ( | ) | const |
Retrieves the client's GUI builder or nullptr if no client specific builder has been assigned via setClientBuilder()
Definition at line 675 of file kxmlguiclient.cpp.
◆ componentName()
|
virtual |
- Returns
- The component name for this GUI client.
Definition at line 129 of file kxmlguiclient.cpp.
◆ domDocument()
|
virtual |
- Returns
- The parsed XML in a QDomDocument, set by setXMLFile() or setXML(). This document describes the layout of the GUI.
Definition at line 134 of file kxmlguiclient.cpp.
◆ endXMLPlug()
void KXMLGUIClient::endXMLPlug | ( | ) |
Definition at line 764 of file kxmlguiclient.cpp.
◆ factory()
KXMLGUIFactory * KXMLGUIClient::factory | ( | ) | const |
Retrieves a pointer to the KXMLGUIFactory this client is associated with (will return nullptr if the client's GUI has not been built by a KXMLGUIFactory.
Definition at line 631 of file kxmlguiclient.cpp.
◆ findMostRecentXMLFile()
|
static |
Definition at line 698 of file kxmlguiclient.cpp.
◆ findVersionNumber()
Returns the version number of the given xml data (belonging to an xml rc file)
- Since
- 5.73
Definition at line 781 of file kxmlguiclient.cpp.
◆ getActionsToChangeForState()
KXMLGUIClient::StateChange KXMLGUIClient::getActionsToChangeForState | ( | const QString & | state | ) |
Definition at line 725 of file kxmlguiclient.cpp.
◆ insertChildClient()
void KXMLGUIClient::insertChildClient | ( | KXMLGUIClient * | child | ) |
Use this method to make a client a child client of another client.
Usually you don't need to call this method, as it is called automatically when using the second constructor, which takes a parent argument.
Definition at line 641 of file kxmlguiclient.cpp.
◆ loadStandardsXmlFile()
|
protected |
Load the ui_standards.rc file.
Usually followed by setXMLFile(xmlFile, true), for merging.
- Since
- 4.6
Definition at line 196 of file kxmlguiclient.cpp.
◆ localXMLFile()
|
virtual |
Definition at line 144 of file kxmlguiclient.cpp.
◆ parentClient()
KXMLGUIClient * KXMLGUIClient::parentClient | ( | ) | const |
KXMLGUIClients can form a simple child/parent object tree.
This method returns a pointer to the parent client or nullptr if it has no parent client assigned.
Definition at line 636 of file kxmlguiclient.cpp.
◆ plugActionList()
ActionLists are a way for XMLGUI to support dynamic lists of actions.
E.g. if you are writing a file manager, and there is a menu file whose contents depend on the mimetype of the file that is selected, then you can achieve this using ActionLists. It works as follows: In your xxxui.rc file ( the one that you set in setXMLFile() / pass to setupGUI() ), you put a tag <ActionList name="xxx">
.
Example:
This tag will get expanded to a list of actions. In the example above ( a file manager with a dynamic file menu ), you would call
every time a file is selected, unselected or ...
- Note
- You should not call KXmlGuiWindow::createGUI() after calling this function. In fact, that would remove the newly added actionlists again...
- Forgetting to call unplugActionList() before plugActionList() would leave the previous actions in the menu too..
- See also
- unplugActionList()
Definition at line 680 of file kxmlguiclient.cpp.
◆ prepareXMLUnplug()
void KXMLGUIClient::prepareXMLUnplug | ( | QWidget * | w | ) |
Definition at line 768 of file kxmlguiclient.cpp.
◆ reloadXML()
void KXMLGUIClient::reloadXML | ( | ) |
Forces this client to re-read its XML resource file.
This is intended to be used when you know that the resource file has changed and you will soon be rebuilding the GUI. This will only have an effect if the client is then removed and re-added to the factory.
This method is only for child clients, do not call it for a mainwindow! For a mainwindow, use loadStandardsXmlFile + setXmlFile(xmlFile()) instead.
Definition at line 161 of file kxmlguiclient.cpp.
◆ removeChildClient()
void KXMLGUIClient::removeChildClient | ( | KXMLGUIClient * | child | ) |
Removes the given child
from the client's children list.
Definition at line 650 of file kxmlguiclient.cpp.
◆ replaceXMLFile()
void KXMLGUIClient::replaceXMLFile | ( | const QString & | xmlfile, |
const QString & | localxmlfile, | ||
bool | merge = false ) |
Sets a new xmlFile() and localXMLFile().
The purpose of this public method is to allow non-inherited objects to replace the ui definition of an embedded client with a customized version. It corresponds to the usual calls to setXMLFile() and setLocalXMLFile().
- Parameters
-
xmlfile The xml file to use. Contrary to setXMLFile(), this must be an absolute file path. localxmlfile The local xml file to set. This should be the full path to a writeable file, usually using QStandardPaths::writableLocation. You can set this to QString(), but no user changes to shortcuts / toolbars will be possible in this case. merge Whether to merge with the global document
- Note
- If in any doubt whether you need this or not, use setXMLFile() and setLocalXMLFile(), instead of this function.
- Just like setXMLFile(), this function has to be called before the client is added to a KXMLGUIFactory in order to have an effect.
- See also
- setLocalXMLFile()
- Since
- 4.4
Definition at line 256 of file kxmlguiclient.cpp.
◆ setClientBuilder()
void KXMLGUIClient::setClientBuilder | ( | KXMLGUIBuilder * | builder | ) |
A client can have an own KXMLGUIBuilder.
Use this method to assign your builder instance to the client (so that the KXMLGUIFactory can use it when building the client's GUI)
Client specific guibuilders are useful if you want to create custom container widgets for your GUI.
Definition at line 670 of file kxmlguiclient.cpp.
◆ setComponentName()
|
protectedvirtual |
Sets the component name for this part.
Call this first in the inherited class constructor. (At least before setXMLFile().)
- Parameters
-
componentName the name of the directory where the XMLGUI files will be found componentDisplayName a user-visible name (e.g. for the toolbar editor)
Definition at line 172 of file kxmlguiclient.cpp.
◆ setDOMDocument()
|
protectedvirtual |
Sets the Document for the part, describing the layout of the GUI.
Call this in the Part-inherited class constructor if you don't call setXMLFile() or setXML().
- Warning
- Using this method is not recommended. Many code paths lead to reloading from the XML file on disk. And editing toolbars requires that the result is written to disk anyway, and loaded from there the next time.
For application-specific changes to a client's XML, it is a better idea to save the modified dom document to an app/default-client.xml and define a local-xml-file to something specific like app/local-client.xml, using replaceXMLFile(). See kdepimlibs/kontactinterface/plugin.cpp for an example.
Definition at line 319 of file kxmlguiclient.cpp.
◆ setFactory()
void KXMLGUIClient::setFactory | ( | KXMLGUIFactory * | factory | ) |
This method is called by the KXMLGUIFactory as soon as the client is added to the KXMLGUIFactory's GUI.
Definition at line 626 of file kxmlguiclient.cpp.
◆ setLocalXMLFile()
|
protectedvirtual |
Set the full path to the "local" xml file, the one used for saving toolbar and shortcut changes.
You normally don't need to call this, if you pass a simple filename to setXMLFile.
Definition at line 251 of file kxmlguiclient.cpp.
◆ setXML()
|
protectedvirtual |
Sets the XML for the part.
Call this in the Part-inherited class constructor if you don't call setXMLFile().
Definition at line 296 of file kxmlguiclient.cpp.
◆ setXMLFile()
|
protectedvirtual |
Sets the name of the rc file containing the XML for the part.
Call this in the inherited class constructor, for parts and plugins.
- Note
- For mainwindows, don't call this, pass the name of the xml file to KXmlGuiWindow::setupGUI() or KXmlGuiWindow::createGUI().
- Parameters
-
file Either an absolute path for the file, or simply the filename. See below for details. If you pass an absolute path here, make sure to also call setLocalXMLFile, otherwise toolbar editing won't work. merge Whether to merge with the global document. setXMLDoc Specify whether to call setXML. Default is true.
The preferred way to call this method is with a simple filename for the file
argument.
Since KF 5.1, the file will then be assumed to be installed in DATADIR/kxmlgui5/, under a directory named after the component name. You should use ${KDE_INSTALL_KXMLGUIDIR}/componentname in your CMakeLists.txt file, to install the .rc file(s).
Since KF 5.4, the file will then be assumed to be installed in a Qt resource in :/kxmlgui5/, under a directory named after the component name.
Definition at line 201 of file kxmlguiclient.cpp.
◆ setXMLGUIBuildDocument()
void KXMLGUIClient::setXMLGUIBuildDocument | ( | const QDomDocument & | doc | ) |
Definition at line 616 of file kxmlguiclient.cpp.
◆ standardsXmlFileLocation()
|
staticprotected |
Return the full path to the ui_standards.rc, might return a resource path.
- Returns
- full path to ui_standards.rc, always non-empty.
- Since
- 5.16
Definition at line 182 of file kxmlguiclient.cpp.
◆ stateChanged()
|
protectedvirtual |
Actions can collectively be assigned a "State".
To accomplish this the respective actions are tagged as <enable> or <disable> in a <State> </State> group of the XMLfile. During program execution the programmer can call stateChanged() to set actions to a defined state.
- Parameters
-
newstate Name of a State in the XMLfile. reverse If the flag reverse is set to StateReverse, the State is reversed. (actions to be enabled will be disabled and action to be disabled will be enabled) Default is reverse=false.
Definition at line 730 of file kxmlguiclient.cpp.
◆ unplugActionList()
void KXMLGUIClient::unplugActionList | ( | const QString & | name | ) |
Unplugs the action list name
from the XMLGUI.
Calling this removes the specified action list, i.e. this is the complement to plugActionList(). See plugActionList() for a more detailed example.
- See also
- plugActionList()
Definition at line 689 of file kxmlguiclient.cpp.
◆ virtual_hook()
|
protectedvirtual |
Reimplemented in KontactInterface::Plugin.
Definition at line 776 of file kxmlguiclient.cpp.
◆ xmlFile()
|
virtual |
This will return the name of the XML file as set by setXMLFile().
If setXML() is used directly, then this will return an empty string.
The filename that this returns is obvious for components as each component has exactly one XML file. In non-components, however, there are usually two: the global file and the local file. This function doesn't really care about that, though. It will always return the last XML file set. This, in almost all cases, will be the local XML file.
- Returns
- The name of the XML file or QString()
Definition at line 139 of file kxmlguiclient.cpp.
◆ xmlguiBuildDocument()
QDomDocument KXMLGUIClient::xmlguiBuildDocument | ( | ) | const |
Definition at line 621 of file kxmlguiclient.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 20 2024 11:54:58 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.