KXmlGuiWindow
#include <KXmlGuiWindow>
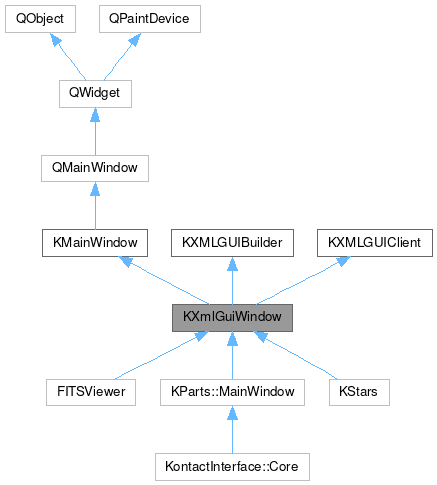
Public Types | |
enum | StandardWindowOption { ToolBar = 1 , Keys = 2 , StatusBar = 4 , Save = 8 , Create = 16 , Default = ToolBar | Keys | StatusBar | Save | Create } |
typedef QFlags< StandardWindowOption > | StandardWindowOptions |
![]() | |
enum | DockOption |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
![]() | |
enum | ReverseStateChange { StateNoReverse , StateReverse } |
Public Slots | |
virtual void | configureToolbars () |
bool | isToolBarVisible (const QString &name) |
void | setToolBarVisible (const QString &name, bool visible) |
virtual void | slotStateChanged (const QString &newstate) |
void | slotStateChanged (const QString &newstate, bool reverse) |
![]() | |
void | appHelpActivated () |
virtual void | setCaption (const QString &caption) |
virtual void | setCaption (const QString &caption, bool modified) |
virtual void | setPlainCaption (const QString &caption) |
void | setSettingsDirty () |
Public Member Functions | |
KXmlGuiWindow (QWidget *parent=nullptr, Qt::WindowFlags flags=Qt::WindowFlags()) | |
~KXmlGuiWindow () override | |
void | applyMainWindowSettings (const KConfigGroup &config) override |
void | createGUI (const QString &xmlfile=QString()) |
void | createStandardStatusBarAction () |
void | finalizeGUI (bool force) |
virtual void | finalizeGUI (KXMLGUIClient *client) |
virtual KXMLGUIFactory * | guiFactory () |
bool | isCommandBarEnabled () const |
bool | isHelpMenuEnabled () const |
bool | isStandardToolBarMenuEnabled () const |
void | setCommandBarEnabled (bool showCommandBar) |
void | setHelpMenuEnabled (bool showHelpMenu=true) |
void | setStandardToolBarMenuEnabled (bool showToolBarMenu) |
void | setupGUI (const QSize &defaultSize, StandardWindowOptions options=Default, const QString &xmlfile=QString()) |
void | setupGUI (StandardWindowOptions options=Default, const QString &xmlfile=QString()) |
void | setupToolbarMenuActions () |
QAction * | toolBarMenuAction () |
QStringList | toolBarNames () const |
![]() | |
KMainWindow (QWidget *parent=nullptr, Qt::WindowFlags flags=Qt::WindowFlags()) | |
~KMainWindow () override | |
KConfigGroup | autoSaveConfigGroup () const |
QString | autoSaveGroup () const |
bool | autoSaveSettings () const |
QString | dbusName () const |
bool | hasMenuBar () |
void | resetAutoSaveSettings () |
bool | restore (int numberOfInstances, bool show=true) |
void | saveMainWindowSettings (KConfigGroup &config) |
void | setAutoSaveSettings (const KConfigGroup &group, bool saveWindowSize=true) |
void | setAutoSaveSettings (const QString &groupName=QStringLiteral("MainWindow"), bool saveWindowSize=true) |
void | setStateConfigGroup (const QString &configGroup) |
KConfigGroup | stateConfigGroup () const |
KToolBar * | toolBar (const QString &name=QString()) |
QList< KToolBar * > | toolBars () const |
![]() | |
QMainWindow (QWidget *parent, Qt::WindowFlags flags) | |
void | addDockWidget (Qt::DockWidgetArea area, QDockWidget *dockwidget) |
void | addDockWidget (Qt::DockWidgetArea area, QDockWidget *dockwidget, Qt::Orientation orientation) |
QToolBar * | addToolBar (const QString &title) |
void | addToolBar (Qt::ToolBarArea area, QToolBar *toolbar) |
void | addToolBar (QToolBar *toolbar) |
void | addToolBarBreak (Qt::ToolBarArea area) |
QWidget * | centralWidget () const const |
Qt::DockWidgetArea | corner (Qt::Corner corner) const const |
virtual QMenu * | createPopupMenu () |
DockOptions | dockOptions () const const |
Qt::DockWidgetArea | dockWidgetArea (QDockWidget *dockwidget) const const |
bool | documentMode () const const |
QSize | iconSize () const const |
void | iconSizeChanged (const QSize &iconSize) |
void | insertToolBar (QToolBar *before, QToolBar *toolbar) |
void | insertToolBarBreak (QToolBar *before) |
bool | isAnimated () const const |
bool | isDockNestingEnabled () const const |
QMenuBar * | menuBar () const const |
QWidget * | menuWidget () const const |
void | removeDockWidget (QDockWidget *dockwidget) |
void | removeToolBar (QToolBar *toolbar) |
void | removeToolBarBreak (QToolBar *before) |
void | resizeDocks (const QList< QDockWidget * > &docks, const QList< int > &sizes, Qt::Orientation orientation) |
bool | restoreDockWidget (QDockWidget *dockwidget) |
bool | restoreState (const QByteArray &state, int version) |
QByteArray | saveState (int version) const const |
void | setAnimated (bool enabled) |
void | setCentralWidget (QWidget *widget) |
void | setCorner (Qt::Corner corner, Qt::DockWidgetArea area) |
void | setDockNestingEnabled (bool enabled) |
void | setDockOptions (DockOptions options) |
void | setDocumentMode (bool enabled) |
void | setIconSize (const QSize &iconSize) |
void | setMenuBar (QMenuBar *menuBar) |
void | setMenuWidget (QWidget *menuBar) |
void | setStatusBar (QStatusBar *statusbar) |
void | setTabPosition (Qt::DockWidgetAreas areas, QTabWidget::TabPosition tabPosition) |
void | setTabShape (QTabWidget::TabShape tabShape) |
void | setToolButtonStyle (Qt::ToolButtonStyle toolButtonStyle) |
void | setUnifiedTitleAndToolBarOnMac (bool set) |
void | splitDockWidget (QDockWidget *first, QDockWidget *second, Qt::Orientation orientation) |
QStatusBar * | statusBar () const const |
void | tabifiedDockWidgetActivated (QDockWidget *dockWidget) |
QList< QDockWidget * > | tabifiedDockWidgets (QDockWidget *dockwidget) const const |
void | tabifyDockWidget (QDockWidget *first, QDockWidget *second) |
QTabWidget::TabPosition | tabPosition (Qt::DockWidgetArea area) const const |
QTabWidget::TabShape | tabShape () const const |
QWidget * | takeCentralWidget () |
Qt::ToolBarArea | toolBarArea (const QToolBar *toolbar) const const |
bool | toolBarBreak (QToolBar *toolbar) const const |
Qt::ToolButtonStyle | toolButtonStyle () const const |
void | toolButtonStyleChanged (Qt::ToolButtonStyle toolButtonStyle) |
bool | unifiedTitleAndToolBarOnMac () const const |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
virtual QSize | minimumSizeHint () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
virtual void | setVisible (bool visible) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
virtual QSize | sizeHint () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
![]() | |
KXMLGUIBuilder (QWidget *widget) | |
KXMLGUIClient * | builderClient () const |
virtual QStringList | containerTags () const |
virtual QWidget * | createContainer (QWidget *parent, int index, const QDomElement &element, QAction *&containerAction) |
virtual QAction * | createCustomElement (QWidget *parent, int index, const QDomElement &element) |
virtual QStringList | customTags () const |
virtual void | removeContainer (QWidget *container, QWidget *parent, QDomElement &element, QAction *containerAction) |
void | setBuilderClient (KXMLGUIClient *client) |
QWidget * | widget () |
![]() | |
KXMLGUIClient () | |
KXMLGUIClient (KXMLGUIClient *parent) | |
virtual | ~KXMLGUIClient () |
virtual QAction * | action (const QDomElement &element) const |
QAction * | action (const QString &name) const |
virtual KActionCollection * | actionCollection () const |
void | addStateActionDisabled (const QString &state, const QString &action) |
void | addStateActionEnabled (const QString &state, const QString &action) |
void | beginXMLPlug (QWidget *) |
QList< KXMLGUIClient * > | childClients () |
KXMLGUIBuilder * | clientBuilder () const |
virtual QString | componentName () const |
virtual QDomDocument | domDocument () const |
void | endXMLPlug () |
KXMLGUIFactory * | factory () const |
StateChange | getActionsToChangeForState (const QString &state) |
void | insertChildClient (KXMLGUIClient *child) |
virtual QString | localXMLFile () const |
KXMLGUIClient * | parentClient () const |
void | plugActionList (const QString &name, const QList< QAction * > &actionList) |
void | prepareXMLUnplug (QWidget *) |
void | reloadXML () |
void | removeChildClient (KXMLGUIClient *child) |
void | replaceXMLFile (const QString &xmlfile, const QString &localxmlfile, bool merge=false) |
void | setClientBuilder (KXMLGUIBuilder *builder) |
void | setFactory (KXMLGUIFactory *factory) |
void | setXMLGUIBuildDocument (const QDomDocument &doc) |
void | unplugActionList (const QString &name) |
virtual QString | xmlFile () const |
QDomDocument | xmlguiBuildDocument () const |
Protected Slots | |
virtual void | saveNewToolbarConfig () |
![]() | |
void | saveAutoSaveSettings () |
Protected Member Functions | |
void | checkAmbiguousShortcuts () |
bool | event (QEvent *event) override |
![]() | |
KXMLGUI_NO_EXPORT | KMainWindow (KMainWindowPrivate &dd, QWidget *parent, Qt::WindowFlags f) |
void | closeEvent (QCloseEvent *) override |
void | keyPressEvent (QKeyEvent *keyEvent) override |
virtual bool | queryClose () |
virtual void | readGlobalProperties (KConfig *sessionConfig) |
virtual void | readProperties (const KConfigGroup &) |
bool | readPropertiesInternal (KConfig *, int) |
virtual void | saveGlobalProperties (KConfig *sessionConfig) |
virtual void | saveProperties (KConfigGroup &) |
void | savePropertiesInternal (KConfig *, int) |
bool | settingsDirty () const |
![]() | |
virtual void | contextMenuEvent (QContextMenuEvent *event) override |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showEvent (QShowEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
virtual void | virtual_hook (int id, void *data) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentName (const QString &componentName, const QString &componentDisplayName) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
static bool | canBeRestored (int numberOfInstances) |
static const QString | classNameOfToplevel (int instanceNumber) |
static QList< KMainWindow * > | memberList () |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
static QString | findVersionNumber (const QString &xml) |
![]() | |
AllowNestedDocks | |
AllowTabbedDocks | |
AnimatedDocks | |
typedef | DockOptions |
ForceTabbedDocks | |
GroupedDragging | |
VerticalTabs | |
![]() | |
DrawChildren | |
DrawWindowBackground | |
IgnoreMask | |
typedef | RenderFlags |
![]() | |
typedef | QObjectList |
![]() | |
PdmDepth | |
PdmDevicePixelRatio | |
PdmDevicePixelRatioScaled | |
PdmDpiX | |
PdmDpiY | |
PdmHeight | |
PdmHeightMM | |
PdmNumColors | |
PdmPhysicalDpiX | |
PdmPhysicalDpiY | |
PdmWidth | |
PdmWidthMM | |
![]() | |
static QString | standardsXmlFileLocation () |
![]() | |
std::unique_ptr< KMainWindowPrivate > const | d_ptr |
Detailed Description
KMainWindow with convenience functions and integration with XmlGui files.
This class includes several convenience <action>Enabled() functions to toggle the presence of functionality in your main window, including a KCommandBar instance.
The StandardWindowOptions enum can be used to pass additional options to describe the main window behavior/appearance. Use it in conjunction with setupGUI() to load an appnameui.rc file to manage the main window's actions.
setCommandBarEnabled() is set by default.
A minimal example can be created with QMainWindow::setCentralWidget() and setupGUI():
With this, a ready-made main window with menubar and statusbar is created, as well as two default menus, Settings and Help.
Management of QActions is made trivial in combination with KActionCollection and KStandardAction.
See https://develop.kde.org/docs/use/kxmlgui/ for a tutorial on how to create a simple text editor using KXmlGuiWindow.
See https://develop.kde.org/docs/use/session-managment for more information on session management.
Definition at line 87 of file kxmlguiwindow.h.
Member Typedef Documentation
◆ StandardWindowOptions
Stores a combination of StandardWindowOptions values.
Use these options for the first argument of setupGUI().
- See also
- setupGUI()
- StandardWindowOption
Definition at line 335 of file kxmlguiwindow.h.
Member Enumeration Documentation
◆ StandardWindowOption
Use these options for the first argument of setupGUI().
- See also
- setupGUI()
- StandardWindowOption
Enumerator | |
---|---|
ToolBar | Adds action(s) to show/hide the toolbar(s) and adds a menu action to configure the toolbar(s). |
Keys | Adds an action in the 'Settings' menu to open the configure keyboard shortcuts dialog. |
StatusBar | Adds an action to show/hide the statusbar in the 'Settings' menu. Note that setting this value will create a statusbar if one doesn't already exist.
|
Save | Autosaves (and loads) the toolbar/menubar/statusbar settings and window size using the default name. Like KMainWindow::setAutoSaveSettings(), enabling this causes the application to save state data upon close in a KConfig-managed configuration file. Typically you want to let the default window size be determined by the widgets' size hints. Make sure that setupGUI() is called after all the widgets are created (including QMainWindow::setCentralWidget()) so that the default size is managed properly. |
Create | Calls createGUI() once ToolBar, Keys and Statusbar have been taken care of.
@ StatusBar Adds an action to show/hide the statusbar in the 'Settings' menu. Definition kxmlguiwindow.h:287 @ Save Autosaves (and loads) the toolbar/menubar/statusbar settings and window size using the default name. Definition kxmlguiwindow.h:304 @ ToolBar Adds action(s) to show/hide the toolbar(s) and adds a menu action to configure the toolbar(s). Definition kxmlguiwindow.h:272 @ Keys Adds an action in the 'Settings' menu to open the configure keyboard shortcuts dialog. Definition kxmlguiwindow.h:278
|
Default | Sets all of the above options as true. |
Definition at line 264 of file kxmlguiwindow.h.
Property Documentation
◆ autoSaveGroup
|
read |
Definition at line 92 of file kxmlguiwindow.h.
◆ autoSaveSettings
|
read |
Definition at line 91 of file kxmlguiwindow.h.
◆ hasMenuBar
|
read |
Definition at line 90 of file kxmlguiwindow.h.
◆ standardToolBarMenuEnabled
|
readwrite |
Definition at line 93 of file kxmlguiwindow.h.
◆ toolBars
|
read |
Definition at line 94 of file kxmlguiwindow.h.
Constructor & Destructor Documentation
◆ KXmlGuiWindow()
|
explicit |
Construct a main window.
Note that by default a KXmlGuiWindow is created with the Qt::WA_DeleteOnClose attribute set, i.e. it is automatically destroyed when the window is closed. If you do not want this behavior, call:
KXmlGuiWindows must be created on the heap with 'new', like:
IMPORTANT: For session management and window management to work properly, all main windows in the application should have a different name. Otherwise, the base class KMainWindow will create a unique name, but it's recommended to explicitly pass a window name that will also describe the type of the window. If there can be several windows of the same type, append '#' (hash) to the name, and KMainWindow will replace it with numbers to make the names unique. For example, for a mail client which has one main window showing the mails and folders, and which can also have one or more windows for composing mails, the name for the folders window should be e.g. "mainwindow" and for the composer windows "composer#".
- Parameters
-
parent The widget parent. This is usually nullptr
, but it may also be the window group leader. In that case, the KXmlGuiWindow becomes a secondary window.flags Specify the window flags. The default is none.
- See also
- KMainWindow::KMainWindow
Definition at line 148 of file kxmlguiwindow.cpp.
◆ ~KXmlGuiWindow()
|
override |
Destructor.
Will also destroy the toolbars and menubar if needed.
Definition at line 245 of file kxmlguiwindow.cpp.
Member Function Documentation
◆ applyMainWindowSettings()
|
overridevirtual |
Read settings for statusbar, menubar and toolbar from their respective groups in the config file and apply them.
- Parameters
-
config Config group to read the settings from.
Reimplemented from KMainWindow.
Definition at line 513 of file kxmlguiwindow.cpp.
◆ checkAmbiguousShortcuts()
|
protected |
Checks if there are actions using the same shortcut.
This is called automatically from createGUI().
- Since
- 5.30
Definition at line 523 of file kxmlguiwindow.cpp.
◆ configureToolbars
|
virtualslot |
Show a standard configure toolbar dialog.
This slot can be connected directly to the action to configure the toolbar.
Definition at line 293 of file kxmlguiwindow.cpp.
◆ createGUI()
Generates the interface based on a local XML file.
This is the function that generates UI elements such as the main menu, toolbar (if any) and statusbar. This is called by setupGUI(Create) as well.
Typically, in a regular application, you would use setupGUI() instead, as it sets up the toolbar/shortcut edit actions, among other things.
If xmlfile
is an empty string, this method will try to construct a local XML filename like appnameui.rc where 'appname' is your app's name. Typically that app name is what KXMLGUIClient::componentName() returns. If that file does not exist, then the XML UI code will use only the global (standard) XML file for its layout purposes.
- Parameters
-
xmlfile The path (relative or absolute) to the local xmlfile
- See also
- setupGUI()
Definition at line 360 of file kxmlguiwindow.cpp.
◆ createStandardStatusBarAction()
void KXmlGuiWindow::createStandardStatusBarAction | ( | ) |
Creates a toggle under the 'Settings' menu to show/hide the statusbar.
Calling this method will create a statusbar if one doesn't already exist.
If an application maintains the action on its own (i.e. never calls this function), a connection needs to be made to let KMainWindow know when the hidden/shown status of the statusbar has changed. For example:
Otherwise the status might not be saved by KMainWindow.
- Note
- This function only makes sense before calling createGUI() or when using setupGUI() without StandardWindowOption::StatusBar.
- See also
- createGUI()
- setupGUI()
- StandardWindowOption
- KStandardAction::showStatusbar()
- setStandardToolBarMenuEnabled()
- QMainWindow::setStatusBar()
- QMainWindow::statusBar()
Definition at line 485 of file kxmlguiwindow.cpp.
◆ event()
|
overrideprotectedvirtual |
Reimplemented to catch QEvent::Polish in order to adjust the object name if needed, once all constructor code for the main window has run.
Also reimplemented to catch when a QDockWidget is added or removed.
Reimplemented from KMainWindow.
Definition at line 251 of file kxmlguiwindow.cpp.
◆ finalizeGUI() [1/2]
void KXmlGuiWindow::finalizeGUI | ( | bool | force | ) |
Definition at line 502 of file kxmlguiwindow.cpp.
◆ finalizeGUI() [2/2]
|
virtual |
Reimplemented from KXMLGUIBuilder.
Definition at line 73 of file kxmlguibuilder.cpp.
◆ guiFactory()
|
virtual |
Definition at line 281 of file kxmlguiwindow.cpp.
◆ isCommandBarEnabled()
bool KXmlGuiWindow::isCommandBarEnabled | ( | ) | const |
Returns whether a KCommandBar was set.
- Returns
true
by default,false
if setCommandBarEnabled(false) was set.
- Since
- 5.83
- See also
- setCommandBarEnabled()
Definition at line 594 of file kxmlguiwindow.cpp.
◆ isHelpMenuEnabled()
bool KXmlGuiWindow::isHelpMenuEnabled | ( | ) | const |
- Returns
true
if the help menu is enabled,false
if setHelpMenuEnabled(false) was set.
- See also
- setHelpMenuEnabled()
Definition at line 275 of file kxmlguiwindow.cpp.
◆ isStandardToolBarMenuEnabled()
bool KXmlGuiWindow::isStandardToolBarMenuEnabled | ( | ) | const |
Returns whether setStandardToolBarMenuEnabled() was set.
- Note
- This function only makes sense if createGUI() was used. This function returns true only if setStandardToolBarMenuEnabled() was set and will return false even if StandardWindowOption::ToolBar was used.
- Returns
true
if setStandardToolBarMenuEnabled() was set,false
otherwise.
Definition at line 479 of file kxmlguiwindow.cpp.
◆ isToolBarVisible
|
slot |
Checks the visual state of a given toolbar.
If an invalid name is given, the method will always return false.
This does not utilize KMainWindow::toolBar(), as that method would create a new toolbar in the case of an invalid name, which is not something that should be freely accessable via the dbus.
- Parameters
-
name The internal name of the toolbar.
- Returns
- whether the toolbar with the specified name is currently visible
- Since
- 6.10
Definition at line 213 of file kxmlguiwindow.cpp.
◆ saveNewToolbarConfig
|
protectedvirtualslot |
Rebuilds the GUI after KEditToolBar changes the toolbar layout.
- See also
- configureToolbars()
Definition at line 306 of file kxmlguiwindow.cpp.
◆ setCommandBarEnabled()
void KXmlGuiWindow::setCommandBarEnabled | ( | bool | showCommandBar | ) |
Enable a KCommandBar to list and quickly execute actions.
A KXmlGuiWindow by default automatically creates a KCommandBar, but it is inaccessible unless createGUI() or setupGUI(Create) is used.
It provides a HUD-like menu that lists all QActions in your application and can be activated via Ctrl+Atl+i or via an action in the 'Help' menu.
If you need more than a global set of QActions listed for your application, use KCommandBar directly instead.
- Parameters
-
showCommandBar Whether to show the command bar. true
by default.
- Since
- 5.83
- See also
- KCommandBar
- KCommandBar::setActions()
- isCommandBarEnabled()
Unset the shortcut
Definition at line 578 of file kxmlguiwindow.cpp.
◆ setHelpMenuEnabled()
void KXmlGuiWindow::setHelpMenuEnabled | ( | bool | showHelpMenu = true | ) |
Creates a standard help menu when calling createGUI() or setupGUI().
- Parameters
-
showHelpMenu Whether to create a Help Menu. true
by default.
- See also
- isHelpMenuEnabled()
Definition at line 269 of file kxmlguiwindow.cpp.
◆ setStandardToolBarMenuEnabled()
void KXmlGuiWindow::setStandardToolBarMenuEnabled | ( | bool | showToolBarMenu | ) |
Creates a toggle under the 'Settings' menu to show/hide the available toolbars.
The standard toolbar menu toggles the visibility of one or multiple toolbars.
If there is only one toolbar configured, a simple 'Show <toolbar name>' menu item is shown; if more than one toolbar is configured, a "Shown Toolbars" menu is created instead, with 'Show <toolbar1 name>', 'Show <toolbar2 name>' ... sub-menu actions.
If your application uses a non-default XmlGui resource file, then you can specify the exact position of the menu/menu item by adding a <Merge name="StandardToolBarMenuHandler" /> line to the settings menu section of your resource file ( usually appname.rc ).
- Parameters
-
showToolBarMenu Whether to show the standard toolbar menu. false
by default.
- Note
- This function only makes sense before calling createGUI(). Using setupGUI(ToolBar) overrides this function.
- See also
- createGUI()
- setupGUI()
- KToggleBarAction
- StandardWindowOption
- KMainWindow::toolBar()
- KMainWindow::toolBars()
- QMainWindow::addToolBar()
- QMainWindow::removeToolBar()
- createStandardStatusBarAction()
Definition at line 452 of file kxmlguiwindow.cpp.
◆ setToolBarVisible
|
slot |
Sets the visibility of a given toolbar.
If an invalid name is given, nothing happens.
- Parameters
-
name The internal name of the toolbar. visible The new state of the toolbar's visibility.
- Since
- 6.10
Definition at line 223 of file kxmlguiwindow.cpp.
◆ setupGUI() [1/2]
void KXmlGuiWindow::setupGUI | ( | const QSize & | defaultSize, |
StandardWindowOptions | options = Default, | ||
const QString & | xmlfile = QString() ) |
This is an overloaded function.
- Parameters
-
defaultSize A manually specified window size that overrides the saved size. options A combination of StandardWindowOptions to specify UI elements to be present in your application window. xmlfile The relative or absolute path to the local xmlfile.
- See also
- setupGUI()
Definition at line 322 of file kxmlguiwindow.cpp.
◆ setupGUI() [2/2]
void KXmlGuiWindow::setupGUI | ( | StandardWindowOptions | options = Default, |
const QString & | xmlfile = QString() ) |
Configures the current window and its actions in the typical KDE fashion.
You can specify which window options/features are going to be set up using options
, see the StandardWindowOptions enum for more details.
Use a bitwise OR (|) to select multiple enum choices for setupGUI() (except when using StandardWindowOption::Default).
Typically this function replaces createGUI(), but it is possible to call setupGUI(Create) together with helper functions such as setStandardToolBarMenuEnabled() and createStandardStatusBarAction().
- Warning
- To use createGUI() and setupGUI() for the same window, you must avoid using StandardWindowOption::Create. Prefer using only setupGUI().
- Note
- When StandardWindowOption::Save is used, this method will restore the state of the application window (toolbar, dockwindows positions ...etc), so you need to have added all your actions to your UI before calling this method.
- Parameters
-
options A combination of StandardWindowOptions to specify UI elements to be present in your application window. xmlfile The relative or absolute path to the local xmlfile. If this is an empty string, the code will look for a local XML file appnameui.rc, where 'appname' is the name of your app. See the note about the xmlfile argument in createGUI().
- See also
- StandardWindowOption
Definition at line 317 of file kxmlguiwindow.cpp.
◆ setupToolbarMenuActions()
void KXmlGuiWindow::setupToolbarMenuActions | ( | ) |
for KToolBar
Definition at line 205 of file kxmlguiwindow.cpp.
◆ slotStateChanged [1/2]
|
virtualslot |
Applies a state change.
Reimplement this to enable and disable actions as defined in the XmlGui rc file.
- Parameters
-
newstate The state change to be applied.
Definition at line 442 of file kxmlguiwindow.cpp.
◆ slotStateChanged [2/2]
|
slot |
Applies a state change.
Reimplement this to enable and disable actions as defined in the XmlGui rc file.
This function can "reverse" the state (disable the actions which should be enabled, and vice-versa) if specified.
- Parameters
-
newstate The state change to be applied. reverse Whether to reverse newstate
or not.
Definition at line 447 of file kxmlguiwindow.cpp.
◆ toolBarMenuAction()
QAction * KXmlGuiWindow::toolBarMenuAction | ( | ) |
- Returns
- A pointer to the main window's action responsible for the toolbar's menu.
Definition at line 195 of file kxmlguiwindow.cpp.
◆ toolBarNames()
QStringList KXmlGuiWindow::toolBarNames | ( | ) | const |
- Returns
- A list of all toolbars for this window in string form
- See also
- KMainWindow::toolBars()
- Since
- 6.10
Definition at line 233 of file kxmlguiwindow.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:44 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.