KTextEditor::MovingRange
#include <KTextEditor/MovingRange>
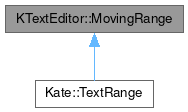
Public Types | |
enum | EmptyBehavior { AllowEmpty = 0x0 , InvalidateIfEmpty = 0x1 } |
enum | InsertBehavior { DoNotExpand = 0x0 , ExpandLeft = 0x1 , ExpandRight = 0x2 } |
typedef QFlags< InsertBehavior > | InsertBehaviors |
Public Member Functions | |
MovingRange (const MovingRange &)=delete | |
virtual | ~MovingRange () |
virtual const Attribute::Ptr & | attribute () const =0 |
virtual bool | attributeOnlyForViews () const =0 |
virtual Document * | document () const =0 |
virtual EmptyBehavior | emptyBehavior () const =0 |
virtual const MovingCursor & | end () const =0 |
virtual MovingRangeFeedback * | feedback () const =0 |
virtual InsertBehaviors | insertBehaviors () const =0 |
bool | isEmpty () const |
operator Range () const | |
MovingRange & | operator= (const MovingRange &)=delete |
virtual void | setAttribute (Attribute::Ptr attribute)=0 |
virtual void | setAttributeOnlyForViews (bool onlyForViews)=0 |
virtual void | setEmptyBehavior (EmptyBehavior emptyBehavior)=0 |
virtual void | setFeedback (MovingRangeFeedback *feedback)=0 |
virtual void | setInsertBehaviors (InsertBehaviors insertBehaviors)=0 |
void | setRange (Cursor start, Cursor end) |
virtual void | setRange (KTextEditor::Range range)=0 |
virtual void | setRange (KTextEditor::Range range, Attribute::Ptr attribute)=0 |
virtual void | setRange (KTextEditor::Range range, Attribute::Ptr attribute, qreal zDepth)=0 |
virtual void | setView (View *view)=0 |
virtual void | setZDepth (qreal zDepth)=0 |
virtual const MovingCursor & | start () const =0 |
LineRange | toLineRange () const Q_DECL_NOEXCEPT |
const Range | toRange () const |
virtual View * | view () const =0 |
virtual qreal | zDepth () const =0 |
Comparison | |
The following functions perform checks against this range in comparison to other lines, columns, cursors, and ranges. | |
bool | contains (const Range &range) const |
bool | contains (Cursor cursor) const |
bool | containsLine (int line) const |
bool | containsColumn (int column) const |
bool | overlaps (const Range &range) const |
bool | overlapsLine (int line) const |
bool | overlapsColumn (int column) const |
bool | onSingleLine () const |
int | numberOfLines () const Q_DECL_NOEXCEPT |
Protected Member Functions | |
MovingRange () | |
Detailed Description
A range that is bound to a specific Document, and maintains its position.
Introduction
A MovingRange is an extension of the basic Range class. It maintains its position in the document. As a result of this, MovingRanges may not be copied, as they need to maintain a connection to the associated Document.
Create a new MovingRange like this:
The ownership of the range is passed to the user. When finished with a MovingRange, simply delete it.
Editing Behavior
The insert behavior controls how the range reacts to characters inserted at the range boundaries, i.e. at the start of the range or the end of the range. Either the range boundary moves with text insertion, or it stays. Use setInsertBehaviors() and insertBehaviors() to set and query the current insert behavior.
When the start() and end() Cursor of a range equal, isEmpty() returns true. Further, the empty-behavior can be changed such that the start() and end() Cursors of MovingRanges that get empty are automatically set to (-1, -1). Use setEmptyBehavior() and emptyBehavior() to control the empty behavior.
- Warning
- MovingRanges may be set to (-1, -1, -1, -1) at any time, if the user reloads a document (F5)! Use a MovingRangeFeedback to get notified if you need to catch this case, and/or listen to the signal MovingInterface::aboutToInvalidateMovingInterfaceContent().
MovingRange Feedback
With setFeedback() a feedback instance can be associated with the moving range. The MovingRangeFeedback notifies about the following events:
- the text cursor (caret) entered the range,
- the text cursor (caret) left the range,
- the mouse cursor entered the range,
- the mouse cursor left the range,
- the range got empty, i.e. start() == end(),
- the range got invalid, i.e. start() == end() == (-1, -1).
If a feedback is not needed anymore, call setFeedback(0).
Working with Ranges
There are several convenience methods that make working with MovingRanges very simple. For instance, use isEmpty() to check if the start() Cursor equals the end() Cursor. Use contains(), containsLine() or containsColumn() to check whether the MovingRange contains a Range, a Cursor, a line or column. The same holds for overlaps(), overlapsLine() and overlapsColumn(). Besides onSingleLine() returns whether a MovingRange spans only one line.
For compatibility, a MovingRange can be explicitly converted to a simple Range by calling toRange(), or implicitly by the Range operator.
Arbitrary Highlighting
With setAttribute() highlighting Attributes can be assigned to a MovingRange. By default, this highlighting is used in all views of a document. Use setView(), if the highlighting should only appear in a specific view. Further, if the additional highlighting should not be printed call setAttributeOnlyForViews() with the parameter true.
MovingRange Example
In the following example, we assume the KTextEditor::Document has the contents:
In order to highlight the function name printText with a yellow background color, the following code is needed:
MovingRanges are invalidated automatically when a document is cleared or closed. Therefore, to avoid invalid ranges, make sure to read the API documentation about MovingInterface::aboutToDeleteMovingInterfaceContent().
- See also
- Cursor, MovingCursor, Range, MovingInterface, MovingRangeFeedback
- Since
- 4.5
Definition at line 132 of file movingrange.h.
Member Typedef Documentation
◆ InsertBehaviors
Stores a combination of InsertBehavior values.
Definition at line 149 of file movingrange.h.
Member Enumeration Documentation
◆ EmptyBehavior
Behavior of range if it becomes empty.
Enumerator | |
---|---|
AllowEmpty | allow range to be empty |
InvalidateIfEmpty | invalidate range, if it becomes empty |
Definition at line 154 of file movingrange.h.
◆ InsertBehavior
Determine how the range reacts to characters inserted immediately outside the range.
- See also
- InsertBehaviors
Definition at line 140 of file movingrange.h.
Constructor & Destructor Documentation
◆ ~MovingRange()
|
virtualdefault |
Destruct the moving range.
◆ MovingRange() [1/2]
|
protecteddefault |
For inherited class only.
◆ MovingRange() [2/2]
|
delete |
no copy constructor, don't allow this to be copied.
Member Function Documentation
◆ attribute()
|
pure virtual |
Gets the active Attribute for this range.
- Returns
- a pointer to the active attribute
Implemented in Kate::TextRange.
◆ attributeOnlyForViews()
|
pure virtual |
Is this range's attribute only visible in views, not for example prints? Default is false.
- Returns
- range visible only for views
Implemented in Kate::TextRange.
◆ contains() [1/2]
|
inline |
Check whether the this range wholly encompasses range.
- Parameters
-
range range to check
- Returns
- true, if this range contains range, otherwise false
Definition at line 424 of file movingrange.h.
◆ contains() [2/2]
|
inline |
Check to see if cursor
is contained within this range, ie >= start() and < end().
- Parameters
-
cursor the position to test for containment
- Returns
- true if the cursor is contained within this range, otherwise false.
Definition at line 436 of file movingrange.h.
◆ containsColumn()
|
inline |
Check whether the range contains column.
- Parameters
-
column column to check
- Returns
- true if the range contains column, otherwise false
Definition at line 460 of file movingrange.h.
◆ containsLine()
|
inline |
Returns true if this range wholly encompasses line
.
- Parameters
-
line line to check
- Returns
- true if the line is wholly encompassed by this range, otherwise false.
Definition at line 448 of file movingrange.h.
◆ document()
|
pure virtual |
Gets the document to which this range is bound.
- Returns
- a pointer to the document
Implemented in Kate::TextRange.
◆ emptyBehavior()
|
pure virtual |
Will this range invalidate itself if it becomes empty?
- Returns
- behavior on becoming empty
Implemented in Kate::TextRange.
◆ end()
|
pure virtual |
◆ feedback()
|
pure virtual |
Gets the active MovingRangeFeedback for this range.
- Returns
- a pointer to the active MovingRangeFeedback
Implemented in Kate::TextRange.
◆ insertBehaviors()
|
pure virtual |
◆ isEmpty()
|
inline |
Returns true if this range contains no characters, ie.
the start() and end() positions are the same.
- Returns
- true if the range contains no characters, otherwise false
Definition at line 405 of file movingrange.h.
◆ numberOfLines()
|
inline |
◆ onSingleLine()
|
inline |
Check whether the start() and end() cursors of this range are on the same line.
- Returns
- true if both the start and end positions are on the same line, otherwise false
Definition at line 508 of file movingrange.h.
◆ operator Range()
|
inline |
Convert this clever range into a dumb one.
Equal to toRange, allowing to use implicit conversion.
- Returns
- normal range
Definition at line 385 of file movingrange.h.
◆ operator=()
|
delete |
no assignment operator, no copying around clever ranges.
◆ overlaps()
bool MovingRange::overlaps | ( | const Range & | range | ) | const |
Check whether the this range overlaps with range.
- Parameters
-
range range to check against
- Returns
- true, if this range overlaps with range, otherwise false
Definition at line 29 of file movingapi.cpp.
◆ overlapsColumn()
|
inline |
Check to see if this range overlaps column
; that is, if column
is between start().column() and end().column().
This function is most likely to be useful in relation to block text editing.
- Parameters
-
column the column to test
- Returns
- true if the column is between the range's starting and ending columns, otherwise false.
Definition at line 496 of file movingrange.h.
◆ overlapsLine()
|
inline |
Check whether the range overlaps at least part of line.
- Parameters
-
line line to check
- Returns
- true, if the range overlaps at least part of line, otherwise false
Definition at line 481 of file movingrange.h.
◆ setAttribute()
|
pure virtual |
Sets the currently active attribute for this range.
This will trigger update of the relevant view parts, if the attribute changed.
- Parameters
-
attribute Attribute to assign to this range. If null, simply removes the previous Attribute.
Implemented in Kate::TextRange.
◆ setAttributeOnlyForViews()
|
pure virtual |
Set if this range's attribute is only visible in views, not for example prints.
- Parameters
-
onlyForViews attribute only valid for views
Implemented in Kate::TextRange.
◆ setEmptyBehavior()
|
pure virtual |
Set if this range will invalidate itself if it becomes empty.
- Parameters
-
emptyBehavior behavior on becoming empty
Implemented in Kate::TextRange.
◆ setFeedback()
|
pure virtual |
Sets the currently active MovingRangeFeedback for this range.
This will trigger evaluation if feedback must be send again (for example if mouse is already inside range).
- Parameters
-
feedback MovingRangeFeedback to assign to this range. If null, simply removes the previous MovingRangeFeedback.
Implemented in Kate::TextRange.
◆ setInsertBehaviors()
|
pure virtual |
Set insert behaviors.
- Parameters
-
insertBehaviors new insert behaviors
Implemented in Kate::TextRange.
◆ setRange() [1/4]
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Set the range of this range A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
start new start for this clever range end new end for this clever range
Definition at line 23 of file movingapi.cpp.
◆ setRange() [2/4]
|
pure virtual |
Set the range of this range.
A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
range new range for this clever range
Implemented in Kate::TextRange.
◆ setRange() [3/4]
|
pure virtual |
Set the range of this range and the connected attribute.
Avoids internal overhead of separate setting that.
A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
range new range for this clever range attribute Attribute to assign to this range. If null, simply removes the previous Attribute.
- Since
- 6.0
Implemented in Kate::TextRange.
◆ setRange() [4/4]
|
pure virtual |
Set the range of this range and the connected attribute and Z-depth.
Avoids internal overhead of separate setting that.
A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
range new range for this clever range attribute Attribute to assign to this range. If null, simply removes the previous Attribute. zDepth new Z-depth of this range
- Since
- 6.0
Implemented in Kate::TextRange.
◆ setView()
|
pure virtual |
Sets the currently active view for this range.
This will trigger update of the relevant view parts, if the view changed. Set view before the attribute, that will avoid not needed redraws.
- Parameters
-
view View to assign to this range. If null, simply removes the previous view.
Implemented in Kate::TextRange.
◆ setZDepth()
|
pure virtual |
Set the current Z-depth of this range.
Ranges with smaller Z-depth than others will win during rendering. This will trigger update of the relevant view parts, if the depth changed. Set depth before the attribute, that will avoid not needed redraws. Default is 0.0.
- Parameters
-
zDepth new Z-depth of this range
Implemented in Kate::TextRange.
◆ start()
|
pure virtual |
◆ toLineRange()
|
inline |
Convert this MovingRange to a simple LineRange.
- Returns
- LineRange from the start line to the end line of this range.
Definition at line 394 of file movingrange.h.
◆ toRange()
|
inline |
Convert this clever range into a dumb one.
- Returns
- normal range
Definition at line 376 of file movingrange.h.
◆ view()
|
pure virtual |
Gets the active view for this range.
Might be already invalid, internally only used for pointer comparisons.
- Returns
- a pointer to the active view
Implemented in Kate::TextRange.
◆ zDepth()
|
pure virtual |
Gets the current Z-depth of this range.
Ranges with smaller Z-depth than others will win during rendering. Default is 0.0.
Defined depths for common kind of ranges use in editor components implementing this interface, smaller depths are more more in the foreground and will win during rendering:
- Selection == -100000.0
- Search == -10000.0
- Bracket Highlighting == -1000.0
- Folding Hover == -100.0
- Returns
- current Z-depth of this range
Implemented in Kate::TextRange.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Mon Nov 18 2024 12:11:28 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.