Kate::TextRange
#include <katetextrange.h>
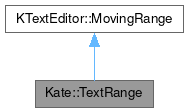
Additional Inherited Members | |
![]() | |
enum | EmptyBehavior { AllowEmpty = 0x0 , InvalidateIfEmpty = 0x1 } |
enum | InsertBehavior { DoNotExpand = 0x0 , ExpandLeft = 0x1 , ExpandRight = 0x2 } |
typedef QFlags< InsertBehavior > | InsertBehaviors |
![]() | |
MovingRange () | |
Detailed Description
Class representing a 'clever' text range.
It will automagically move if the text inside the buffer it belongs to is modified. By intention no subclass of KTextEditor::Range, must be converted manually. A TextRange is allowed to be empty. If you call setInvalidateIfEmpty(true), a TextRange will become automatically invalid as soon as start() == end() position holds.
Definition at line 35 of file katetextrange.h.
Constructor & Destructor Documentation
◆ TextRange() [1/2]
Kate::TextRange::TextRange | ( | TextBuffer * | buffer, |
KTextEditor::Range | range, | ||
InsertBehaviors | insertBehavior, | ||
EmptyBehavior | emptyBehavior = AllowEmpty ) |
Construct a text range.
A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
buffer parent text buffer range The initial text range assumed by the new range. insertBehavior Define whether the range should expand when text is inserted adjacent to the range. emptyBehavior Define whether the range should invalidate itself on becoming empty.
Definition at line 16 of file katetextrange.cpp.
◆ TextRange() [2/2]
|
delete |
No copy constructor, don't allow this to be copied.
◆ ~TextRange()
|
override |
Destruct the text block.
Definition at line 36 of file katetextrange.cpp.
Member Function Documentation
◆ attribute()
|
inlineoverridevirtual |
Gets the active Attribute for this range.
- Returns
- a pointer to the active attribute
Implements KTextEditor::MovingRange.
Definition at line 240 of file katetextrange.h.
◆ attributeOnlyForViews()
|
inlineoverridevirtual |
Is this range's attribute only visible in views, not for example prints?
Default is false.
- Returns
- range visible only for views
Implements KTextEditor::MovingRange.
Definition at line 287 of file katetextrange.h.
◆ document()
|
overridevirtual |
Gets the document to which this range is bound.
- Returns
- a pointer to the document
Implements KTextEditor::MovingRange.
Definition at line 300 of file katetextrange.cpp.
◆ emptyBehavior()
|
inlineoverridevirtual |
Will this range invalidate itself if it becomes empty?
- Returns
- behavior on becoming empty
Implements KTextEditor::MovingRange.
Definition at line 90 of file katetextrange.h.
◆ end()
|
inlineoverridevirtual |
Retrieve end cursor of this range, read-only.
- Returns
- end cursor
Implements KTextEditor::MovingRange.
Definition at line 173 of file katetextrange.h.
◆ endInternal()
|
inline |
Nonvirtual version of end(), which is faster.
- Returns
- end cursor
Definition at line 182 of file katetextrange.h.
◆ feedback()
|
inlineoverridevirtual |
Gets the active MovingRangeFeedback for this range.
- Returns
- a pointer to the active MovingRangeFeedback
Implements KTextEditor::MovingRange.
Definition at line 268 of file katetextrange.h.
◆ hasAttribute()
|
inline |
- Returns
- whether a nonzero attribute is set. This is faster than checking attribute(), because the reference-counting is omitted.
Definition at line 249 of file katetextrange.h.
◆ insertBehaviors()
|
overridevirtual |
Get current insert behaviors.
- Returns
- current insert behaviors
Implements KTextEditor::MovingRange.
Definition at line 79 of file katetextrange.cpp.
◆ operator KTextEditor::Range()
|
inline |
Convert this clever range into a dumb one.
Equal to toRange, allowing to use implicit conversion.
- Returns
- normal range
Definition at line 210 of file katetextrange.h.
◆ operator=()
No assignment operator, no copying around.
◆ setAttribute()
|
overridevirtual |
Sets the currently active attribute for this range.
This will trigger update of the relevant view parts.
- Parameters
-
attribute Attribute to assign to this range. If null, simply removes the previous Attribute.
Implements KTextEditor::MovingRange.
Definition at line 233 of file katetextrange.cpp.
◆ setAttributeOnlyForViews()
|
overridevirtual |
Set if this range's attribute is only visible in views, not for example prints.
- Parameters
-
onlyForViews attribute only valid for views
Implements KTextEditor::MovingRange.
Definition at line 278 of file katetextrange.cpp.
◆ setEmptyBehavior()
|
overridevirtual |
Set if this range will invalidate itself if it becomes empty.
- Parameters
-
emptyBehavior behavior on becoming empty
Implements KTextEditor::MovingRange.
Definition at line 94 of file katetextrange.cpp.
◆ setFeedback()
|
overridevirtual |
Sets the currently active MovingRangeFeedback for this range.
This will trigger evaluation if feedback must be send again (for example if mouse is already inside range).
- Parameters
-
feedback MovingRangeFeedback to assign to this range. If null, simply removes the previous MovingRangeFeedback.
Implements KTextEditor::MovingRange.
Definition at line 260 of file katetextrange.cpp.
◆ setInsertBehaviors()
|
overridevirtual |
Set insert behaviors.
- Parameters
-
insertBehaviors new insert behaviors
Implements KTextEditor::MovingRange.
Definition at line 62 of file katetextrange.cpp.
◆ setRange() [1/4]
|
inline |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Set the range of this range A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
start new start for this clever range end new end for this clever range
Definition at line 117 of file katetextrange.h.
◆ setRange() [2/4]
|
overridevirtual |
Set the range of this range.
A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
range new range for this clever range
Implements KTextEditor::MovingRange.
Definition at line 110 of file katetextrange.cpp.
◆ setRange() [3/4]
|
overridevirtual |
Set the range of this range and the connected attribute.
Avoids internal overhead of separate setting that.
A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
range new range for this clever range attribute Attribute to assign to this range. If null, simply removes the previous Attribute.
- Since
- 6.0
Implements KTextEditor::MovingRange.
Definition at line 169 of file katetextrange.cpp.
◆ setRange() [4/4]
|
overridevirtual |
Set the range of this range and the connected attribute and Z-depth.
Avoids internal overhead of separate setting that.
A TextRange is not allowed to be empty, as soon as start == end position, it will become automatically invalid!
- Parameters
-
range new range for this clever range attribute Attribute to assign to this range. If null, simply removes the previous Attribute. zDepth new Z-depth of this range
- Since
- 6.0
Implements KTextEditor::MovingRange.
Definition at line 176 of file katetextrange.cpp.
◆ setView()
|
overridevirtual |
Sets the currently active view for this range.
This will trigger update of the relevant view parts, if the view changed. Set view before the attribute, that will avoid not needed redraws.
- Parameters
-
view View to assign to this range. If null, simply removes the previous view.
Implements KTextEditor::MovingRange.
Definition at line 216 of file katetextrange.cpp.
◆ setZDepth()
|
overridevirtual |
Set the current Z-depth of this range.
Ranges with smaller Z-depth than others will win during rendering. This will trigger update of the relevant view parts, if the depth changed. Set depth before the attribute, that will avoid not needed redraws. Default is 0.0.
- Parameters
-
zDepth new Z-depth of this range
Implements KTextEditor::MovingRange.
Definition at line 284 of file katetextrange.cpp.
◆ spansMultipleBlocks()
|
inline |
Definition at line 321 of file katetextrange.h.
◆ start()
|
inlineoverridevirtual |
Retrieve start cursor of this range, read-only.
- Returns
- start cursor
Implements KTextEditor::MovingRange.
Definition at line 155 of file katetextrange.h.
◆ startInternal()
|
inline |
Non-virtual version of start(), which is faster.
- Returns
- start cursor
Definition at line 164 of file katetextrange.h.
◆ toLineRange()
|
inline |
Hides parent's impl of toLineRange() and uses non-virtual functions internally.
Definition at line 190 of file katetextrange.h.
◆ toRange()
|
inline |
Convert this clever range into a dumb one.
- Returns
- normal range
Definition at line 199 of file katetextrange.h.
◆ view()
|
inlineoverridevirtual |
Gets the active view for this range.
Might be already invalid, internally only used for pointer comparisons.
- Returns
- a pointer to the active view
Implements KTextEditor::MovingRange.
Definition at line 220 of file katetextrange.h.
◆ zDepth()
|
inlineoverridevirtual |
Gets the current Z-depth of this range.
Ranges with smaller Z-depth than others will win during rendering. Default is 0.0.
- Returns
- current Z-depth of this range
Implements KTextEditor::MovingRange.
Definition at line 305 of file katetextrange.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:56:22 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.