Style
#include <style.h>
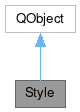
Public Types | |
enum | StyleType : uint { Light = 0 , Dark , Adaptive , Auto , TrueBlack , Inverted } |
Properties | |
QColor | accentColor |
QVariant | adaptiveColorSchemeSource |
uint | contentMargins |
QString | currentIconTheme |
QFont | defaultFont |
int | defaultFontSize |
uint | defaultPadding |
uint | defaultSpacing |
bool | enableEffects |
GroupSizes * | fontSizes |
QFont | h1Font |
QFont | h2Font |
uint | iconSize |
GroupSizes * | iconSizes |
bool | menusHaveIcons |
QFont | monospacedFont |
bool | playSounds |
uint | radiusV |
uint | rowHeight |
uint | rowHeightAlt |
uint | scrollBarPolicy |
GroupSizes * | space |
StyleType | styleType |
QML_ELEMENTuint | toolBarHeight |
uint | toolBarHeightAlt |
bool | translucencyAvailable |
Units * | units |
![]() | |
objectName | |
Signals | |
void | accentColorChanged (QColor color) |
void | adaptiveColorSchemeSourceChanged (QVariant source) |
void | colorSchemeChanged () |
void | contentMarginsChanged () |
void | currentIconThemeChanged (QString currentIconTheme) |
void | defaultFontChanged () |
void | defaultPaddingChanged () |
void | defaultSpacingChanged () |
void | enableEffectsChanged (bool enableEffects) |
void | fontSizesChanged () |
void | h1FontChanged () |
void | h2FontChanged () |
void | iconSizeChanged (uint size) |
void | monospacedFontChanged () |
void | playSoundsChanged (bool) |
void | radiusVChanged (uint radius) |
void | scrollBarPolicyChanged (uint) |
void | styleTypeChanged (StyleType type) |
void | translucencyAvailableChanged (bool translucencyAvailable) |
Public Slots | |
int | mapToIconSizes (const int &size) |
Public Member Functions | |
QColor | accentColor () const |
QVariant | adaptiveColorSchemeSource () const |
QString | currentIconTheme () const |
bool | enableEffects () const |
uint | iconSize () const |
bool | menusHaveIcons () const |
bool | playSounds () const |
uint | scrollBarPolicy () const |
void | setAccentColor (const QColor &color) |
void | setAdaptiveColorSchemeSource (const QVariant &source) |
void | setRadiusV (const uint &radius) |
void | setStyleType (const StyleType &type) |
void | setTranslucencyAvailable (const bool &value) |
StyleType | styleType () const |
bool | translucencyAvailable () const |
void | unsetAccentColor () |
void | unsetAdaptiveColorSchemeSource () |
void | unsetStyeType () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | eventFilter (QObject *watched, QEvent *event) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
Member Enumeration Documentation
◆ StyleType
enum Style::StyleType : uint |
The different options for the color scheme style.
Enumerator | |
---|---|
Light | A light variant designed for Maui. |
Dark | A dark variant designed for Maui. |
Adaptive | Picks the color scheme based on an source input, such as an image. The generated color palette determines if it is a dark or light scheme, and also its accent color. |
Auto | Picks the colors from the system palette, usually from Plasma color-scheme files.
|
TrueBlack | A fully black color palette with a full white accent color. This is might be useful as a accessibility enhance or for performance on E-Ink and AMOLED displays. |
Inverted | A fully white color palette with a true black accent color. This is the inverted version of the TrueBlack type. |
Property Documentation
◆ accentColor
|
readwrite |
Sets the color to be used for highlighted, active, checked and such states of the UI elements.
By default this color is set to the global preferences via MauiMan. This can be overridden by each application to a custom color. To reset it back to the system preference set the property to undefined
.
◆ adaptiveColorSchemeSource
|
readwrite |
The source for picking up the application color palette when the style type is set to Style.Adaptive.
The source can be an image URL, and QQC2 Image, or a QQC2 Item, or even an icon name. By default the source for this is set to the MauiMan wallpaper source preference.
◆ contentMargins
uint Style::contentMargins |
The preferred size for the margins in the browsing views, such as the ListBrowser and GridBrowser, but also for the margins in menus.
- Note
- This property is read only. It can only be modified from the MauiMan global preferences.
◆ currentIconTheme
|
read |
◆ defaultFont
QFont Style::defaultFont |
◆ defaultFontSize
int Style::defaultFontSize |
◆ defaultPadding
uint Style::defaultPadding |
The preferred padding size for the UI elements, such a menu entries, buttons, bars, etc.
The padding refers to the outer-space around the visible background area of the elements.
- Note
- This property is read only. It can only be modified from the MauiMan global preferences.
◆ defaultSpacing
uint Style::defaultSpacing |
◆ enableEffects
|
read |
Whether special effects are desired.
This can be tweaked in the MauiMan system preferences in cases where the resources need to be preserved. Consider using this property when using special effects in your applications.
- Note
- This property is read-only. It can only be modified from the MauiMan global preferences.
◆ fontSizes
GroupSizes * Style::fontSizes |
The group of different standard font sizes for the MauiKit applications.
- See also
- GroupSizes
◆ h1Font
QFont Style::h1Font |
◆ h2Font
◆ iconSize
|
read |
◆ iconSizes
GroupSizes * Style::iconSizes |
The group of different standard icon sizes for consistency in the MauiKit apps.
- See also
- GroupSizes Values are the standard: 16, 22, 32 ,48, 64, 128 [pixels]
◆ menusHaveIcons
|
read |
◆ monospacedFont
QFont Style::monospacedFont |
◆ playSounds
|
read |
◆ radiusV
uint Style::radiusV |
◆ rowHeight
uint Style::rowHeight |
◆ rowHeightAlt
uint Style::rowHeightAlt |
◆ scrollBarPolicy
|
read |
◆ space
GroupSizes * Style::space |
The group of different standard spacing values for consistency in the MauiKit apps.
- See also
- GroupSizes
◆ styleType
|
readwrite |
◆ toolBarHeight
QML_ELEMENTuint Style::toolBarHeight |
◆ toolBarHeightAlt
uint Style::toolBarHeightAlt |
An alternative size for the tab bars, this is a bit smaller then the toolBarHeight
.
- See also
- toolBarHeight
◆ translucencyAvailable
|
read |
◆ units
Units * Style::units |
Member Function Documentation
◆ accentColor()
◆ adaptiveColorSchemeSource()
◆ currentIconTheme()
◆ enableEffects()
◆ eventFilter()
◆ iconSize()
◆ mapToIconSizes
|
slot |
◆ menusHaveIcons()
◆ playSounds()
◆ scrollBarPolicy()
◆ setAccentColor()
◆ setAdaptiveColorSchemeSource()
void Style::setAdaptiveColorSchemeSource | ( | const QVariant & | source | ) |
◆ setRadiusV()
◆ setStyleType()
◆ setTranslucencyAvailable()
void Style::setTranslucencyAvailable | ( | const bool & | value | ) |
◆ styleType()
Style::StyleType Style::styleType | ( | ) | const |
◆ translucencyAvailable()
◆ unsetAccentColor()
◆ unsetAdaptiveColorSchemeSource()
◆ unsetStyeType()
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:57:11 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.