KMessageDialog
#include <KMessageDialog>
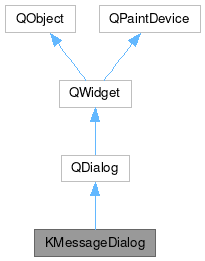
Public Types | |
enum | ButtonType { Ok = 1 , Cancel = 2 , PrimaryAction = 3 , SecondaryAction = 4 } |
enum | Type { QuestionTwoActions = 1 , QuestionTwoActionsCancel = 2 , WarningTwoActions = 3 , WarningTwoActionsCancel = 4 , WarningContinueCancel = 5 , Information = 6 , Error = 8 } |
![]() | |
enum | DialogCode |
![]() | |
enum | RenderFlag |
![]() | |
enum | PaintDeviceMetric |
Public Member Functions | |
KMessageDialog (KMessageDialog::Type type, const QString &text, QWidget *parent=nullptr) | |
KMessageDialog (KMessageDialog::Type type, const QString &text, WId parent_id) | |
~KMessageDialog () override | |
bool | isDontAskAgainChecked () const |
bool | isNotifyEnabled () const |
void | setButtons (const KGuiItem &primaryAction=KGuiItem(), const KGuiItem &secondaryAction=KGuiItem(), const KGuiItem &cancelAction=KGuiItem()) |
void | setCaption (const QString &caption) |
void | setDetails (const QString &details) |
void | setDontAskAgainChecked (bool isChecked) |
void | setDontAskAgainText (const QString &dontAskAgainText) |
void | setIcon (const QIcon &icon) |
void | setListWidgetItems (const QStringList &strlist) |
void | setNotifyEnabled (bool enable) |
void | setOpenExternalLinks (bool isAllowed) |
![]() | |
QDialog (QWidget *parent, Qt::WindowFlags f) | |
virtual void | accept () |
void | accepted () |
virtual void | done (int r) |
virtual int | exec () |
void | finished (int result) |
bool | isSizeGripEnabled () const const |
virtual QSize | minimumSizeHint () const const override |
virtual void | open () |
virtual void | reject () |
void | rejected () |
int | result () const const |
void | setModal (bool modal) |
void | setResult (int i) |
void | setSizeGripEnabled (bool) |
virtual void | setVisible (bool visible) override |
virtual QSize | sizeHint () const const override |
![]() | |
QWidget (QWidget *parent, Qt::WindowFlags f) | |
bool | acceptDrops () const const |
QString | accessibleDescription () const const |
QString | accessibleName () const const |
QList< QAction * > | actions () const const |
void | activateWindow () |
QAction * | addAction (const QIcon &icon, const QString &text) |
QAction * | addAction (const QIcon &icon, const QString &text, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QIcon &icon, const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QIcon &icon, const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text) |
QAction * | addAction (const QString &text, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, Args &&... args) |
QAction * | addAction (const QString &text, const QKeySequence &shortcut, const QObject *receiver, const char *member, Qt::ConnectionType type) |
QAction * | addAction (const QString &text, const QObject *receiver, const char *member, Qt::ConnectionType type) |
void | addAction (QAction *action) |
void | addActions (const QList< QAction * > &actions) |
void | adjustSize () |
bool | autoFillBackground () const const |
QPalette::ColorRole | backgroundRole () const const |
QBackingStore * | backingStore () const const |
QSize | baseSize () const const |
QWidget * | childAt (const QPoint &p) const const |
QWidget * | childAt (int x, int y) const const |
QRect | childrenRect () const const |
QRegion | childrenRegion () const const |
void | clearFocus () |
void | clearMask () |
bool | close () |
QMargins | contentsMargins () const const |
QRect | contentsRect () const const |
Qt::ContextMenuPolicy | contextMenuPolicy () const const |
QCursor | cursor () const const |
void | customContextMenuRequested (const QPoint &pos) |
WId | effectiveWinId () const const |
void | ensurePolished () const const |
Qt::FocusPolicy | focusPolicy () const const |
QWidget * | focusProxy () const const |
QWidget * | focusWidget () const const |
const QFont & | font () const const |
QFontInfo | fontInfo () const const |
QFontMetrics | fontMetrics () const const |
QPalette::ColorRole | foregroundRole () const const |
QRect | frameGeometry () const const |
QSize | frameSize () const const |
const QRect & | geometry () const const |
QPixmap | grab (const QRect &rectangle) |
void | grabGesture (Qt::GestureType gesture, Qt::GestureFlags flags) |
void | grabKeyboard () |
void | grabMouse () |
void | grabMouse (const QCursor &cursor) |
int | grabShortcut (const QKeySequence &key, Qt::ShortcutContext context) |
QGraphicsEffect * | graphicsEffect () const const |
QGraphicsProxyWidget * | graphicsProxyWidget () const const |
bool | hasEditFocus () const const |
bool | hasFocus () const const |
virtual bool | hasHeightForWidth () const const |
bool | hasMouseTracking () const const |
bool | hasTabletTracking () const const |
int | height () const const |
virtual int | heightForWidth (int w) const const |
void | hide () |
Qt::InputMethodHints | inputMethodHints () const const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const const |
void | insertAction (QAction *before, QAction *action) |
void | insertActions (QAction *before, const QList< QAction * > &actions) |
bool | isActiveWindow () const const |
bool | isAncestorOf (const QWidget *child) const const |
bool | isEnabled () const const |
bool | isEnabledTo (const QWidget *ancestor) const const |
bool | isFullScreen () const const |
bool | isHidden () const const |
bool | isMaximized () const const |
bool | isMinimized () const const |
bool | isModal () const const |
bool | isTopLevel () const const |
bool | isVisible () const const |
bool | isVisibleTo (const QWidget *ancestor) const const |
bool | isWindow () const const |
bool | isWindowModified () const const |
QLayout * | layout () const const |
Qt::LayoutDirection | layoutDirection () const const |
QLocale | locale () const const |
void | lower () |
QPoint | mapFrom (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapFrom (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapFromGlobal (const QPoint &pos) const const |
QPointF | mapFromGlobal (const QPointF &pos) const const |
QPoint | mapFromParent (const QPoint &pos) const const |
QPointF | mapFromParent (const QPointF &pos) const const |
QPoint | mapTo (const QWidget *parent, const QPoint &pos) const const |
QPointF | mapTo (const QWidget *parent, const QPointF &pos) const const |
QPoint | mapToGlobal (const QPoint &pos) const const |
QPointF | mapToGlobal (const QPointF &pos) const const |
QPoint | mapToParent (const QPoint &pos) const const |
QPointF | mapToParent (const QPointF &pos) const const |
QRegion | mask () const const |
int | maximumHeight () const const |
QSize | maximumSize () const const |
int | maximumWidth () const const |
int | minimumHeight () const const |
QSize | minimumSize () const const |
int | minimumWidth () const const |
void | move (const QPoint &) |
void | move (int x, int y) |
QWidget * | nativeParentWidget () const const |
QWidget * | nextInFocusChain () const const |
QRect | normalGeometry () const const |
void | overrideWindowFlags (Qt::WindowFlags flags) |
virtual QPaintEngine * | paintEngine () const const override |
const QPalette & | palette () const const |
QWidget * | parentWidget () const const |
QPoint | pos () const const |
QWidget * | previousInFocusChain () const const |
QWIDGETSIZE_MAX QWIDGETSIZE_MAX | |
void | raise () |
QRect | rect () const const |
void | releaseKeyboard () |
void | releaseMouse () |
void | releaseShortcut (int id) |
void | removeAction (QAction *action) |
void | render (QPaintDevice *target, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | render (QPainter *painter, const QPoint &targetOffset, const QRegion &sourceRegion, RenderFlags renderFlags) |
void | repaint () |
void | repaint (const QRect &rect) |
void | repaint (const QRegion &rgn) |
void | repaint (int x, int y, int w, int h) |
void | resize (const QSize &) |
void | resize (int w, int h) |
bool | restoreGeometry (const QByteArray &geometry) |
QByteArray | saveGeometry () const const |
QScreen * | screen () const const |
void | scroll (int dx, int dy) |
void | scroll (int dx, int dy, const QRect &r) |
void | setAcceptDrops (bool on) |
void | setAccessibleDescription (const QString &description) |
void | setAccessibleName (const QString &name) |
void | setAttribute (Qt::WidgetAttribute attribute, bool on) |
void | setAutoFillBackground (bool enabled) |
void | setBackgroundRole (QPalette::ColorRole role) |
void | setBaseSize (const QSize &) |
void | setBaseSize (int basew, int baseh) |
void | setContentsMargins (const QMargins &margins) |
void | setContentsMargins (int left, int top, int right, int bottom) |
void | setContextMenuPolicy (Qt::ContextMenuPolicy policy) |
void | setCursor (const QCursor &) |
void | setDisabled (bool disable) |
void | setEditFocus (bool enable) |
void | setEnabled (bool) |
void | setFixedHeight (int h) |
void | setFixedSize (const QSize &s) |
void | setFixedSize (int w, int h) |
void | setFixedWidth (int w) |
void | setFocus () |
void | setFocus (Qt::FocusReason reason) |
void | setFocusPolicy (Qt::FocusPolicy policy) |
void | setFocusProxy (QWidget *w) |
void | setFont (const QFont &) |
void | setForegroundRole (QPalette::ColorRole role) |
void | setGeometry (const QRect &) |
void | setGeometry (int x, int y, int w, int h) |
void | setGraphicsEffect (QGraphicsEffect *effect) |
void | setHidden (bool hidden) |
void | setInputMethodHints (Qt::InputMethodHints hints) |
void | setLayout (QLayout *layout) |
void | setLayoutDirection (Qt::LayoutDirection direction) |
void | setLocale (const QLocale &locale) |
void | setMask (const QBitmap &bitmap) |
void | setMask (const QRegion ®ion) |
void | setMaximumHeight (int maxh) |
void | setMaximumSize (const QSize &) |
void | setMaximumSize (int maxw, int maxh) |
void | setMaximumWidth (int maxw) |
void | setMinimumHeight (int minh) |
void | setMinimumSize (const QSize &) |
void | setMinimumSize (int minw, int minh) |
void | setMinimumWidth (int minw) |
void | setMouseTracking (bool enable) |
void | setPalette (const QPalette &) |
void | setParent (QWidget *parent) |
void | setParent (QWidget *parent, Qt::WindowFlags f) |
void | setScreen (QScreen *screen) |
void | setShortcutAutoRepeat (int id, bool enable) |
void | setShortcutEnabled (int id, bool enable) |
void | setSizeIncrement (const QSize &) |
void | setSizeIncrement (int w, int h) |
void | setSizePolicy (QSizePolicy) |
void | setSizePolicy (QSizePolicy::Policy horizontal, QSizePolicy::Policy vertical) |
void | setStatusTip (const QString &) |
void | setStyle (QStyle *style) |
void | setStyleSheet (const QString &styleSheet) |
void | setTabletTracking (bool enable) |
void | setToolTip (const QString &) |
void | setToolTipDuration (int msec) |
void | setUpdatesEnabled (bool enable) |
void | setupUi (QWidget *widget) |
void | setWhatsThis (const QString &) |
void | setWindowFilePath (const QString &filePath) |
void | setWindowFlag (Qt::WindowType flag, bool on) |
void | setWindowFlags (Qt::WindowFlags type) |
void | setWindowIcon (const QIcon &icon) |
void | setWindowIconText (const QString &) |
void | setWindowModality (Qt::WindowModality windowModality) |
void | setWindowModified (bool) |
void | setWindowOpacity (qreal level) |
void | setWindowRole (const QString &role) |
void | setWindowState (Qt::WindowStates windowState) |
void | setWindowTitle (const QString &) |
void | show () |
void | showFullScreen () |
void | showMaximized () |
void | showMinimized () |
void | showNormal () |
QSize | size () const const |
QSize | sizeIncrement () const const |
QSizePolicy | sizePolicy () const const |
void | stackUnder (QWidget *w) |
QString | statusTip () const const |
QStyle * | style () const const |
QString | styleSheet () const const |
bool | testAttribute (Qt::WidgetAttribute attribute) const const |
QString | toolTip () const const |
int | toolTipDuration () const const |
QWidget * | topLevelWidget () const const |
bool | underMouse () const const |
void | ungrabGesture (Qt::GestureType gesture) |
void | unsetCursor () |
void | unsetLayoutDirection () |
void | unsetLocale () |
void | update () |
void | update (const QRect &rect) |
void | update (const QRegion &rgn) |
void | update (int x, int y, int w, int h) |
void | updateGeometry () |
bool | updatesEnabled () const const |
QRegion | visibleRegion () const const |
QString | whatsThis () const const |
int | width () const const |
QWidget * | window () const const |
QString | windowFilePath () const const |
Qt::WindowFlags | windowFlags () const const |
QWindow * | windowHandle () const const |
QIcon | windowIcon () const const |
void | windowIconChanged (const QIcon &icon) |
QString | windowIconText () const const |
void | windowIconTextChanged (const QString &iconText) |
Qt::WindowModality | windowModality () const const |
qreal | windowOpacity () const const |
QString | windowRole () const const |
Qt::WindowStates | windowState () const const |
QString | windowTitle () const const |
void | windowTitleChanged (const QString &title) |
Qt::WindowType | windowType () const const |
WId | winId () const const |
int | x () const const |
int | y () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
![]() | |
int | colorCount () const const |
int | depth () const const |
qreal | devicePixelRatio () const const |
qreal | devicePixelRatioF () const const |
int | height () const const |
int | heightMM () const const |
int | logicalDpiX () const const |
int | logicalDpiY () const const |
bool | paintingActive () const const |
int | physicalDpiX () const const |
int | physicalDpiY () const const |
int | width () const const |
int | widthMM () const const |
Static Public Member Functions | |
static void | beep (KMessageDialog::Type type, const QString &text=QString(), QWidget *dialog=nullptr) |
![]() | |
QWidget * | createWindowContainer (QWindow *window, QWidget *parent, Qt::WindowFlags flags) |
QWidget * | find (WId id) |
QWidget * | keyboardGrabber () |
QWidget * | mouseGrabber () |
void | setTabOrder (QWidget *first, QWidget *second) |
void | setTabOrder (std::initializer_list< QWidget * > widgets) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
void | showEvent (QShowEvent *event) override |
![]() | |
virtual void | closeEvent (QCloseEvent *e) override |
virtual void | contextMenuEvent (QContextMenuEvent *e) override |
virtual bool | eventFilter (QObject *o, QEvent *e) override |
virtual void | keyPressEvent (QKeyEvent *e) override |
virtual void | resizeEvent (QResizeEvent *) override |
![]() | |
virtual void | actionEvent (QActionEvent *event) |
virtual void | changeEvent (QEvent *event) |
void | create (WId window, bool initializeWindow, bool destroyOldWindow) |
void | destroy (bool destroyWindow, bool destroySubWindows) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | enterEvent (QEnterEvent *event) |
virtual bool | event (QEvent *event) override |
virtual void | focusInEvent (QFocusEvent *event) |
bool | focusNextChild () |
virtual bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *event) |
bool | focusPreviousChild () |
virtual void | hideEvent (QHideEvent *event) |
virtual void | initPainter (QPainter *painter) const const override |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyReleaseEvent (QKeyEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual int | metric (PaintDeviceMetric m) const const override |
virtual void | mouseDoubleClickEvent (QMouseEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual void | moveEvent (QMoveEvent *event) |
virtual bool | nativeEvent (const QByteArray &eventType, void *message, qintptr *result) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | tabletEvent (QTabletEvent *event) |
void | updateMicroFocus (Qt::InputMethodQuery query) |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
KMessageDialog creates a message box similar to the ones you get from KMessageBox, but that can be used asynchronously, i.e.
you can show the dialog by using show()
or open()
.
This class contructs a dialog similar to the dialogs the KMessageBox convenience functions create. The main difference is that the KMessageBox methods typically use exec()
to show the dialogs; one of the main disadvantages of using exec()
, is that it starts a nested eventloop, which could lead to nasty crashes.
Another difference is that the API is supposed to be slightly easier to use as it has various methods to set up the dialog, e.g. setCaption(), setDetails() ...etc.
By default, appropriate buttons based on the dialog type are added (since 5.85) (e.g. an "OK" button is added to an Information dialog), you can set custom buttons by using the setButtons() method.
The QDialog::done() slot is called to set the result of the dialog, which will emit the QDialog::finished() signal with that result. The result is one of the KMessageDialog::ButtonType enum. This is useful as you can tell exactly which button was clicked by the user. E.g.:
- the secondary action button having been clicked, in which case you may still want to save the status of the "Do not ask again" CheckBox
- the "Cancel" button having been clicked, in which case you ideally will ignore the status of the "Do not ask again" CheckBox
For "warning" dialogs, i.e. dialogs with a potentially destructive action, the default button is set to a button with the QDialogButtonBox::RejectRole. If the "Cancel" button
* is used, it will be the default, otherwise the secondary action button.
This class intends to be very flexible with the buttons that can be used, since you can call the setButtons() method with a KGuiItem that has custom text/icon.
Since Frameworks 5.97 a notification sound is played when the dialog opens like KMessageBox does, this can be controlled using the setNotifyEnabled() method.
Example:
QStringLiteral("Back or forward?"),nullptr);dlg->setCaption(QStringLiteral("Window Title"));dlg->setDetails(QStringLiteral("Some more details."));dlg->setDontAskAgainText(QStringLiteral("Do not ask again"));dlg->setDontAskAgainChecked(false);// Delete the dialog when it's closeddlg->setAttribute(Qt::WA_DeleteOnClose);// Make the dialog window modaldlg->setWindowModality(Qt::WindowModal);switch(button) {// The user clicked the primary action, handle the result...// save the "do not ask again" box status...break;// The user clicked the secondary action, handle the result...// save the "do not ask again" box status...break;case KMessageDialog::Cancel:// The user clicked cancel, reject the changes...break;default:break;}});dlg->show();@ QuestionTwoActionsCancelQuestion dialog with two buttons and Cancel;.Definition kmessagedialog.h:124KMessageDialog(KMessageDialog::Type type, const QString &text, QWidget *parent=nullptr)Constructs a KMessageDialog.Definition kmessagedialog.cpp:64KGuiItem back(BidiMode useBidi)Returns the 'Back' gui item, like Konqueror's back button.Definition kstandardguiitem.cpp:255KGuiItem forward(BidiMode useBidi)Returns the 'Forward' gui item, like Konqueror's forward button.Definition kstandardguiitem.cpp:261void finished(int result)int result() const constQMetaObject::Connection connect(const QObject *sender, PointerToMemberFunction signal, Functor functor)WA_DeleteOnCloseWindowModal- Since
- 5.77
Definition at line 106 of file kmessagedialog.h.
Member Enumeration Documentation
◆ ButtonType
Button types.
- Since
- 5.100
Enumerator | |
---|---|
Ok | Ok button. |
Cancel | Cancel button. |
PrimaryAction | Primary action button. |
SecondaryAction | Secondary action button. |
Definition at line 115 of file kmessagedialog.h.
◆ Type
enum KMessageDialog::Type |
Definition at line 122 of file kmessagedialog.h.
Constructor & Destructor Documentation
◆ KMessageDialog() [1/2]
|
explicit |
Constructs a KMessageDialog.
Buttons based on the dialog type are set by default in some cases, using KStandardGuiItem instances. For the dialog types Information and Error the button is set to KStandardGuiItem::ok(). For the type WarningContinueCancel the buttons are set to KStandardGuiItem::cont() & KStandardGuiItem::cancel().
For the other Quesion* and Warning* types the buttons are to be set explicitly.
- Parameters
-
type the dialog Type, one of KMessageDialog::Type enum text the text message that is going to be displayed in the dialog parent a QWidget* that will be set as the dialog parent
Definition at line 64 of file kmessagedialog.cpp.
◆ KMessageDialog() [2/2]
|
explicit |
This constructor takes the window Id of the parent window, instead of a QWidget*.
- Parameters
-
type the dialog Type, one of KMessageDialog::Type enum text the text message that is going to be displayed in the dialog parent_id the native parent's window system identifier
Definition at line 199 of file kmessagedialog.cpp.
◆ ~KMessageDialog()
|
override |
Destructor.
Definition at line 209 of file kmessagedialog.cpp.
Member Function Documentation
◆ beep()
|
static |
Manually play the notification sound.
When implementing your entirely own message box, not using KMessageDialog, you can call this function to play the relevant notification sound (if enabled).
- Note
- You don't need to call this when using KMessageDialog, it plays the sound automatically.
- Parameters
-
type The message box type text The message box contents, for accessibility purposes. dialog The dialog that was displayed
- Since
- 6.0
- See also
- setNotifyEnabled
Definition at line 474 of file kmessagedialog.cpp.
◆ isDontAskAgainChecked()
bool KMessageDialog::isDontAskAgainChecked | ( | ) | const |
This can be used to query the status of the "Do not ask again" checkbox; returns true
if the box is checked and false
otherwise.
- Note
- This method will return
false
if a checkbox widget isn't shown in the dialog. The dialog will not show a checkbox if setDontAskAgainText() was not used previously to add a checkbox to begin with.
Definition at line 436 of file kmessagedialog.cpp.
◆ isNotifyEnabled()
bool KMessageDialog::isNotifyEnabled | ( | ) | const |
Whether a KNotification is emitted when the dialog is shown.
This typically plays a notification sound. Default is true.
- Since
- 5.97
- See also
- KMessageBox::Notify
Definition at line 455 of file kmessagedialog.cpp.
◆ setButtons()
void KMessageDialog::setButtons | ( | const KGuiItem & | primaryAction = KGuiItem(), |
const KGuiItem & | secondaryAction = KGuiItem(), | ||
const KGuiItem & | cancelAction = KGuiItem() ) |
Sets the buttons in the buttom box.
Using this method, you can customize the behavior based on your use-case, by using a KGuiItem to get a button with custom text and icon.
Since 5.85 buttons based on the dialog type are added by default (see KMessageDialog(KMessageDialog::Type, const QString &, QWidget *) for details). Before, this method had to be called explicitly to have any buttons added to the dialog.
- Note
- For dialog types Information and Error only one button (KStandardGuiItem::ok()) is added to the dialog.
- Parameters
-
primaryAction the action for the primary button. Reported in the result for dialog types Information and Error as KMessageDialog::Ok enum value, otherwise as KMessageDialog::PrimaryAction. secondaryAction the action for the secondary button. Reported in the result as KMessageDialog::SecondaryAction enum value. Ignored with all dialog types without a "secondary" action. cancelAction the action for the cancel button. Reported in the result as KMessageDialog::Cancel enum value. Ignored with all dialog types without a Cancel button.
Definition at line 337 of file kmessagedialog.cpp.
◆ setCaption()
void KMessageDialog::setCaption | ( | const QString & | caption | ) |
This can be used to set the title of the dialog window.
If you pass an empty QString(), a generic title will be used depending on the dialog Type. E.g. for KMessageDialog::WarningTwoActions, "Warning" will be used.
Definition at line 214 of file kmessagedialog.cpp.
◆ setDetails()
void KMessageDialog::setDetails | ( | const QString & | details | ) |
This will add a KCollapsibleGroupBox with a title "Details", as the class name implies it is collapsible (and collapsed by default); you can use it to add a more detailed explanation of what the dialog is trying to tell the user.
If details
is empty, the details widget will not be shown.
Definition at line 318 of file kmessagedialog.cpp.
◆ setDontAskAgainChecked()
void KMessageDialog::setDontAskAgainChecked | ( | bool | isChecked | ) |
This can be used to set the initial status of the "Do not ask again" checkbox, checked or unchecked, by setting isChecked
to true
or false
respectively.
You need to call setDontAskAgainText() first to actually show a checkbox in the dialog, otherwise calling this function will have no effect.
Definition at line 424 of file kmessagedialog.cpp.
◆ setDontAskAgainText()
void KMessageDialog::setDontAskAgainText | ( | const QString & | dontAskAgainText | ) |
This will add a "Do not ask again" checkbox to the dialog with the text from dontAskAgainText
.
You can set the initial status of the checkbox by using setDontAskAgainChecked().
If dontAskAgainText
is empty, no checkbox will be shown.
Typical usage of this checkbox is for recurring questions, e.g. showing a dialog to confirm moving files/directories to trash, the user can then set the checkbox so as not to be asked about that again.
You can get the state of the checkbox by using isDontAskAgainChecked().
Definition at line 418 of file kmessagedialog.cpp.
◆ setIcon()
void KMessageDialog::setIcon | ( | const QIcon & | icon | ) |
This can be used to set an icon that will be shown next to the main text message.
If you pass a null QIcon() a generic icon based on the dialog Type will be used. E.g. for KMessageDialog::QuestionTwoActions, QMessageBox::Question will be used.
Definition at line 246 of file kmessagedialog.cpp.
◆ setListWidgetItems()
void KMessageDialog::setListWidgetItems | ( | const QStringList & | strlist | ) |
This will add a QListWidget to the dialog and populate it with strlist
.
If strlist
is empty, the list widget will not be shown.
Definition at line 287 of file kmessagedialog.cpp.
◆ setNotifyEnabled()
void KMessageDialog::setNotifyEnabled | ( | bool | enable | ) |
Whether to emit a KNotification when the dialog is shown.
This typically plays a notification sound.
- Since
- 5.97
- See also
- KMessageBox::Notify
Definition at line 460 of file kmessagedialog.cpp.
◆ setOpenExternalLinks()
void KMessageDialog::setOpenExternalLinks | ( | bool | isAllowed | ) |
Sets the text labels in the dialog to either allow opening external links or not.
Definition at line 448 of file kmessagedialog.cpp.
◆ showEvent()
|
overrideprotectedvirtual |
Reimplemented from QDialog.
Definition at line 465 of file kmessagedialog.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:54:50 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.