NET
#include <netwm_def.h>
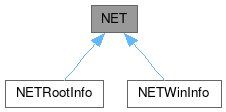
Public Types | |
enum | { OnAllDesktops = -1 } |
enum | Action { ActionMove = 1u << 0 , ActionResize = 1u << 1 , ActionMinimize = 1u << 2 , ActionShade = 1u << 3 , ActionStick = 1u << 4 , ActionMaxVert = 1u << 5 , ActionMaxHoriz = 1u << 6 , ActionMax = ActionMaxVert | ActionMaxHoriz , ActionFullScreen = 1u << 7 , ActionChangeDesktop = 1u << 8 , ActionClose = 1u << 9 } |
typedef QFlags< Action > | Actions |
enum | DesktopLayoutCorner { DesktopLayoutCornerTopLeft = 0 , DesktopLayoutCornerTopRight = 1 , DesktopLayoutCornerBottomLeft = 2 , DesktopLayoutCornerBottomRight = 3 } |
enum | Direction { TopLeft = 0 , Top = 1 , TopRight = 2 , Right = 3 , BottomRight = 4 , Bottom = 5 , BottomLeft = 6 , Left = 7 , Move = 8 , KeyboardSize = 9 , KeyboardMove = 10 , MoveResizeCancel = 11 } |
enum | MappingState { Visible = 1 , Withdrawn = 0 , Iconic = 3 } |
enum | Orientation { OrientationHorizontal = 0 , OrientationVertical = 1 } |
typedef QFlags< Property > | Properties |
typedef QFlags< Property2 > | Properties2 |
enum | Property { Supported = 1u << 0 , ClientList = 1u << 1 , ClientListStacking = 1u << 2 , NumberOfDesktops = 1u << 3 , DesktopGeometry = 1u << 4 , DesktopViewport = 1u << 5 , CurrentDesktop = 1u << 6 , DesktopNames = 1u << 7 , ActiveWindow = 1u << 8 , WorkArea = 1u << 9 , SupportingWMCheck = 1u << 10 , VirtualRoots = 1u << 11 , CloseWindow = 1u << 13 , WMMoveResize = 1u << 14 , WMName = 1u << 15 , WMVisibleName = 1u << 16 , WMDesktop = 1u << 17 , WMWindowType = 1u << 18 , WMState = 1u << 19 , WMStrut = 1u << 20 , WMIconGeometry = 1u << 21 , WMIcon = 1u << 22 , WMPid = 1u << 23 , WMHandledIcons = 1u << 24 , WMPing = 1u << 25 , XAWMState = 1u << 27 , WMFrameExtents = 1u << 28 , WMIconName = 1u << 29 , WMVisibleIconName = 1u << 30 , WMGeometry = 1u << 31 , WMAllProperties = ~0u } |
enum | Property2 { WM2UserTime = 1u << 0 , WM2StartupId = 1u << 1 , WM2TransientFor = 1u << 2 , WM2GroupLeader = 1u << 3 , WM2AllowedActions = 1u << 4 , WM2RestackWindow = 1u << 5 , WM2MoveResizeWindow = 1u << 6 , WM2ExtendedStrut = 1u << 7 , WM2KDETemporaryRules = 1u << 8 , WM2WindowClass = 1u << 9 , WM2WindowRole = 1u << 10 , WM2ClientMachine = 1u << 11 , WM2ShowingDesktop = 1u << 12 , WM2Opacity = 1u << 13 , WM2DesktopLayout = 1u << 14 , WM2FullPlacement = 1u << 15 , WM2FullscreenMonitors = 1u << 16 , WM2FrameOverlap = 1u << 17 , WM2Activities = 1u << 18 , WM2BlockCompositing = 1u << 19 , WM2KDEShadow = 1u << 20 , WM2Urgency = 1u << 21 , WM2Input = 1u << 22 , WM2Protocols = 1u << 23 , WM2InitialMappingState = 1u << 24 , WM2IconPixmap = 1u << 25 , WM2OpaqueRegion = 1u << 25 , WM2DesktopFileName = 1u << 26 , WM2GTKFrameExtents = 1u << 27 , WM2AppMenuServiceName = 1u << 28 , WM2AppMenuObjectPath = 1u << 29 , WM2GTKApplicationId = 1u << 30 , WM2GTKShowWindowMenu = 1u << 31 , WM2AllProperties = ~0u } |
enum | Protocol { NoProtocol = 0 , TakeFocusProtocol = 1 << 0 , DeleteWindowProtocol = 1 << 1 , PingProtocol = 1 << 2 , SyncRequestProtocol = 1 << 3 , ContextHelpProtocol = 1 << 4 } |
typedef QFlags< Protocol > | Protocols |
enum | RequestSource { FromUnknown = 0 , FromApplication = 1 , FromTool = 2 } |
enum | Role { Client , WindowManager } |
enum | State { Modal = 1u << 0 , Sticky = 1u << 1 , MaxVert = 1u << 2 , MaxHoriz = 1u << 3 , Max = MaxVert | MaxHoriz , Shaded = 1u << 4 , SkipTaskbar = 1u << 5 , KeepAbove = 1u << 6 , SkipPager = 1u << 7 , Hidden = 1u << 8 , FullScreen = 1u << 9 , KeepBelow = 1u << 10 , DemandsAttention = 1u << 11 , SkipSwitcher = 1u << 12 , Focused = 1u << 13 } |
typedef QFlags< State > | States |
enum | WindowType { Unknown = -1 , Normal = 0 , Desktop = 1 , Dock = 2 , Toolbar = 3 , Menu = 4 , Dialog = 5 , Override = 6 , TopMenu = 7 , Utility = 8 , Splash = 9 , DropdownMenu = 10 , PopupMenu = 11 , Tooltip = 12 , Notification = 13 , ComboBox = 14 , DNDIcon = 15 , OnScreenDisplay = 16 , CriticalNotification = 17 , AppletPopup = 18 } |
enum | WindowTypeMask { NormalMask = 1u << 0 , DesktopMask = 1u << 1 , DockMask = 1u << 2 , ToolbarMask = 1u << 3 , MenuMask = 1u << 4 , DialogMask = 1u << 5 , OverrideMask = 1u << 6 , TopMenuMask = 1u << 7 , UtilityMask = 1u << 8 , SplashMask = 1u << 9 , DropdownMenuMask = 1u << 10 , PopupMenuMask = 1u << 11 , TooltipMask = 1u << 12 , NotificationMask = 1u << 13 , ComboBoxMask = 1u << 14 , DNDIconMask = 1u << 15 , OnScreenDisplayMask = 1u << 16 , CriticalNotificationMask = 1u << 17 , AppletPopupMask = 1u << 18 , AllTypesMask = 0U - 1 } |
typedef QFlags< WindowTypeMask > | WindowTypes |
Static Public Member Functions | |
static int | timestampCompare (unsigned long time1, unsigned long time2) |
static int | timestampDiff (unsigned long time1, unsigned long time2) |
static bool | typeMatchesMask (WindowType type, WindowTypes mask) |
Detailed Description
Base namespace class.
The NET API is an implementation of the NET Window Manager Specification.
This class is the base class for the NETRootInfo and NETWinInfo classes, which are used to retrieve and modify the properties of windows. To keep the namespace relatively clean, all enums are defined here.
Definition at line 334 of file netwm_def.h.
Member Typedef Documentation
◆ Actions
typedef QFlags< Action > NET::Actions |
Stores a combination of Action values.
Definition at line 660 of file netwm_def.h.
◆ Properties
typedef QFlags< Property > NET::Properties |
Stores a combination of Property values.
Definition at line 750 of file netwm_def.h.
◆ Properties2
typedef QFlags< Property2 > NET::Properties2 |
Stores a combination of Property2 values.
Definition at line 827 of file netwm_def.h.
◆ Protocols
typedef QFlags< Protocol > NET::Protocols |
Stores a combination of Protocol values.
Definition at line 892 of file netwm_def.h.
◆ States
typedef QFlags< State > NET::States |
Stores a combination of State values.
Definition at line 581 of file netwm_def.h.
◆ WindowTypes
typedef QFlags< WindowTypeMask > NET::WindowTypes |
Stores a combination of WindowTypeMask values.
Definition at line 478 of file netwm_def.h.
Member Enumeration Documentation
◆ anonymous enum
anonymous enum |
Sentinel value to indicate that the client wishes to be visible on all desktops.
Definition at line 833 of file netwm_def.h.
◆ Action
enum NET::Action |
Actions that can be done with a window (_NET_WM_ALLOWED_ACTIONS).
- See also
- Actions
Definition at line 644 of file netwm_def.h.
◆ DesktopLayoutCorner
Starting corner for desktop layout.
Definition at line 867 of file netwm_def.h.
◆ Direction
enum NET::Direction |
Direction for WMMoveResize.
When a client wants the Window Manager to start a WMMoveResize, it should specify one of:
- TopLeft
- Top
- TopRight
- Right
- BottomRight
- Bottom
- BottomLeft
- Left
- Move (for movement only)
- KeyboardSize (resizing via keyboard)
- KeyboardMove (movement via keyboard)
Definition at line 602 of file netwm_def.h.
◆ MappingState
enum NET::MappingState |
Client window mapping state.
The class automatically watches the mapping state of the client windows, and uses the mapping state to determine how to set/change different properties. Note that this is very lowlevel and you most probably don't want to use this state.
Enumerator | |
---|---|
Visible | indicates the client window is visible to the user. |
Withdrawn | indicates that neither the client window nor its icon is visible. |
Iconic | indicates that the client window is not visible, but its icon is. This can be when the window is minimized or when it's on a different virtual desktop. See also NET::Hidden. |
Definition at line 623 of file netwm_def.h.
◆ Orientation
enum NET::Orientation |
Definition at line 859 of file netwm_def.h.
◆ Property
enum NET::Property |
Supported properties.
Clients and Window Managers must define which properties/protocols it wants to support.
Root/Desktop window properties and protocols:
- Supported
- ClientList
- ClientListStacking
- NumberOfDesktops
- DesktopGeometry
- DesktopViewport
- CurrentDesktop
- DesktopNames
- ActiveWindow
- WorkArea
- SupportingWMCheck
- VirtualRoots
- CloseWindow
- WMMoveResize
Client window properties and protocols:
- WMName
- WMVisibleName
- WMDesktop
- WMWindowType
- WMState
- WMStrut (obsoleted by WM2ExtendedStrut)
- WMGeometry
- WMFrameExtents
- WMIconGeometry
- WMIcon
- WMIconName
- WMVisibleIconName
- WMHandledIcons
- WMPid
- WMPing
ICCCM properties (provided for convenience):
- XAWMState
- See also
- Properties
Definition at line 708 of file netwm_def.h.
◆ Property2
enum NET::Property2 |
Supported properties.
This enum is an extension to NET::Property, because them enum is limited only to 32 bits.
Client window properties and protocols:
- WM2UserTime
- WM2StartupId
- WM2TransientFor mainwindow for the window (WM_TRANSIENT_FOR)
- WM2GroupLeader group leader (window_group in WM_HINTS)
- WM2AllowedActions
- WM2RestackWindow
- WM2MoveResizeWindow
- WM2ExtendedStrut
- WM2TemporaryRules internal, for kstart
- WM2WindowClass WM_CLASS
- WM2WindowRole WM_WINDOW_ROLE
- WM2ClientMachine WM_CLIENT_MACHINE
- WM2ShowingDesktop
- WM2Opacity _NET_WM_WINDOW_OPACITY
- WM2DesktopLayout _NET_DESKTOP_LAYOUT
- WM2FullPlacement _NET_WM_FULL_PLACEMENT
- WM2FullscreenMonitors _NET_WM_FULLSCREEN_MONITORS
- WM2Urgency urgency hint in WM_HINTS (see ICCCM 4.1.2.4)
- WM2Input input hint (input in WM_HINTS, see ICCCM 4.1.2.4)
- WM2Protocols see NET::Protocol
- WM2InitialMappingState initial state hint of WM_HINTS (see ICCCM 4.1.2.4)
- WM2IconPixmap icon pixmap and mask in WM_HINTS (see ICCCM 4.1.2.4)
- WM2OpaqueRegion
- WM2DesktopFileName the base name of the desktop file name or the full path to the desktop file
- WM2GTKFrameExtents extents of the shadow drawn by the client
- WM2GTKApplicationId _GTK_APPLICATION_ID
- WM2GTKShowWindowMenu _GTK_SHOW_WINDOW_MENU
- See also
- Properties2
Definition at line 788 of file netwm_def.h.
◆ Protocol
enum NET::Protocol |
Protocols supported by the client.
See ICCCM 4.1.2.7.
- See also
- Protocols
- Since
- 5.3
Definition at line 881 of file netwm_def.h.
◆ RequestSource
enum NET::RequestSource |
Source of the request.
Definition at line 841 of file netwm_def.h.
◆ Role
enum NET::Role |
Application role.
This is used internally to determine how several action should be performed (if at all).
Enumerator | |
---|---|
Client | indicates that the application is a client application. |
WindowManager | indicates that the application is a window manager application. |
Definition at line 342 of file netwm_def.h.
◆ State
enum NET::State |
Window state.
To set the state of a window, you'll typically do something like:
for example to not show the window on the taskbar, desktop pager, or window switcher. winId() is a function of QWidget()
Note that KeepAbove (StaysOnTop) and KeepBelow are meant as user preference and applications should avoid setting these states themselves.
- See also
- States
Enumerator | |
---|---|
Modal | indicates that this is a modal dialog box. The WM_TRANSIENT_FOR hint MUST be set to indicate which window the dialog is a modal for, or set to the root window if the dialog is a modal for its window group. |
Sticky | indicates that the Window Manager SHOULD keep the window's position fixed on the screen, even when the virtual desktop scrolls. Note that this is different from being kept on all desktops. |
MaxVert | indicates that the window is vertically maximized. |
MaxHoriz | indicates that the window is horizontally maximized. |
Max | convenience value. Equal to MaxVert | MaxHoriz. |
Shaded | indicates that the window is shaded (rolled-up). |
SkipTaskbar | indicates that a window should not be included on a taskbar. |
KeepAbove | indicates that a window should on top of most windows (but below fullscreen windows). |
SkipPager | indicates that a window should not be included on a pager. |
Hidden | indicates that a window should not be visible on the screen (e.g. when minimised). Only the window manager is allowed to change it. |
FullScreen | indicates that a window should fill the entire screen and have no window decorations. |
KeepBelow | indicates that a window should be below most windows (but above any desktop windows). |
DemandsAttention | there was an attempt to activate this window, but the window manager prevented this. E.g. taskbar should mark such window specially to bring user's attention to this window. Only the window manager is allowed to change it. |
SkipSwitcher | indicates that a window should not be included on a switcher.
|
Focused | indicates that a client should render as though it has focus Only the window manager is allowed to change it.
|
Definition at line 503 of file netwm_def.h.
◆ WindowType
enum NET::WindowType |
Window type.
Enumerator | |
---|---|
Unknown | indicates that the window did not define a window type. |
Normal | indicates that this is a normal, top-level window |
Desktop | indicates a desktop feature. This can include a single window containing desktop icons with the same dimensions as the screen, allowing the desktop environment to have full control of the desktop, without the need for proxying root window clicks. |
Dock | indicates a dock or panel feature |
Toolbar | indicates a toolbar window |
Menu | indicates a pinnable (torn-off) menu window |
Dialog | indicates that this is a dialog window |
Override |
|
TopMenu | indicates a toplevel menu (AKA macmenu). This is a KDE extension to the _NET_WM_WINDOW_TYPE mechanism. |
Utility | indicates a utility window |
Splash | indicates that this window is a splash screen window. |
DropdownMenu | indicates a dropdown menu (from a menubar typically) |
PopupMenu | indicates a popup menu (a context menu typically) |
Tooltip | indicates a tooltip window |
Notification | indicates a notification window |
ComboBox | indicates that the window is a list for a combobox |
DNDIcon | indicates a window that represents the dragged object during DND operation |
OnScreenDisplay | indicates an On Screen Display window (such as volume feedback)
|
CriticalNotification | indicates a critical notification (such as battery is running out)
|
AppletPopup | indicates that this window is an applet. |
Definition at line 357 of file netwm_def.h.
◆ WindowTypeMask
enum NET::WindowTypeMask |
Values for WindowType when they should be OR'ed together, e.g.
for the properties argument of the NETRootInfo constructor.
- See also
- WindowTypes
Enumerator | |
---|---|
NormalMask |
|
DesktopMask |
|
DockMask |
|
ToolbarMask |
|
MenuMask |
|
DialogMask |
|
OverrideMask |
|
TopMenuMask |
|
UtilityMask |
|
SplashMask |
|
DropdownMenuMask |
|
PopupMenuMask |
|
TooltipMask |
|
NotificationMask |
|
ComboBoxMask |
|
DNDIconMask |
|
OnScreenDisplayMask | NON STANDARD.
|
CriticalNotificationMask | NON STANDARD.
|
AppletPopupMask | NON STANDARD.
|
AllTypesMask | All window types. |
Definition at line 453 of file netwm_def.h.
Member Function Documentation
◆ timestampCompare()
|
static |
◆ timestampDiff()
|
static |
Returns a difference of two X timestamps, time2 - time1, where time2 must be later than time1, as returned by timestampCompare().
◆ typeMatchesMask()
|
static |
Returns true if the given window type matches the mask given using WindowTypeMask flags.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:52:11 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.