NETRootInfo
#include <netwm.h>
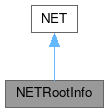
Public Types | |
enum | { PROTOCOLS , WINDOW_TYPES , STATES , PROTOCOLS2 , ACTIONS , PROPERTIES_SIZE } |
![]() | |
enum | { OnAllDesktops = -1 } |
enum | Action { ActionMove = 1u << 0 , ActionResize = 1u << 1 , ActionMinimize = 1u << 2 , ActionShade = 1u << 3 , ActionStick = 1u << 4 , ActionMaxVert = 1u << 5 , ActionMaxHoriz = 1u << 6 , ActionMax = ActionMaxVert | ActionMaxHoriz , ActionFullScreen = 1u << 7 , ActionChangeDesktop = 1u << 8 , ActionClose = 1u << 9 } |
typedef QFlags< Action > | Actions |
enum | DesktopLayoutCorner { DesktopLayoutCornerTopLeft = 0 , DesktopLayoutCornerTopRight = 1 , DesktopLayoutCornerBottomLeft = 2 , DesktopLayoutCornerBottomRight = 3 } |
enum | Direction { TopLeft = 0 , Top = 1 , TopRight = 2 , Right = 3 , BottomRight = 4 , Bottom = 5 , BottomLeft = 6 , Left = 7 , Move = 8 , KeyboardSize = 9 , KeyboardMove = 10 , MoveResizeCancel = 11 } |
enum | MappingState { Visible = 1 , Withdrawn = 0 , Iconic = 3 } |
enum | Orientation { OrientationHorizontal = 0 , OrientationVertical = 1 } |
typedef QFlags< Property > | Properties |
typedef QFlags< Property2 > | Properties2 |
enum | Property { Supported = 1u << 0 , ClientList = 1u << 1 , ClientListStacking = 1u << 2 , NumberOfDesktops = 1u << 3 , DesktopGeometry = 1u << 4 , DesktopViewport = 1u << 5 , CurrentDesktop = 1u << 6 , DesktopNames = 1u << 7 , ActiveWindow = 1u << 8 , WorkArea = 1u << 9 , SupportingWMCheck = 1u << 10 , VirtualRoots = 1u << 11 , CloseWindow = 1u << 13 , WMMoveResize = 1u << 14 , WMName = 1u << 15 , WMVisibleName = 1u << 16 , WMDesktop = 1u << 17 , WMWindowType = 1u << 18 , WMState = 1u << 19 , WMStrut = 1u << 20 , WMIconGeometry = 1u << 21 , WMIcon = 1u << 22 , WMPid = 1u << 23 , WMHandledIcons = 1u << 24 , WMPing = 1u << 25 , XAWMState = 1u << 27 , WMFrameExtents = 1u << 28 , WMIconName = 1u << 29 , WMVisibleIconName = 1u << 30 , WMGeometry = 1u << 31 , WMAllProperties = ~0u } |
enum | Property2 { WM2UserTime = 1u << 0 , WM2StartupId = 1u << 1 , WM2TransientFor = 1u << 2 , WM2GroupLeader = 1u << 3 , WM2AllowedActions = 1u << 4 , WM2RestackWindow = 1u << 5 , WM2MoveResizeWindow = 1u << 6 , WM2ExtendedStrut = 1u << 7 , WM2KDETemporaryRules = 1u << 8 , WM2WindowClass = 1u << 9 , WM2WindowRole = 1u << 10 , WM2ClientMachine = 1u << 11 , WM2ShowingDesktop = 1u << 12 , WM2Opacity = 1u << 13 , WM2DesktopLayout = 1u << 14 , WM2FullPlacement = 1u << 15 , WM2FullscreenMonitors = 1u << 16 , WM2FrameOverlap = 1u << 17 , WM2Activities = 1u << 18 , WM2BlockCompositing = 1u << 19 , WM2KDEShadow = 1u << 20 , WM2Urgency = 1u << 21 , WM2Input = 1u << 22 , WM2Protocols = 1u << 23 , WM2InitialMappingState = 1u << 24 , WM2IconPixmap = 1u << 25 , WM2OpaqueRegion = 1u << 25 , WM2DesktopFileName = 1u << 26 , WM2GTKFrameExtents = 1u << 27 , WM2AppMenuServiceName = 1u << 28 , WM2AppMenuObjectPath = 1u << 29 , WM2GTKApplicationId = 1u << 30 , WM2GTKShowWindowMenu = 1u << 31 , WM2AllProperties = ~0u } |
enum | Protocol { NoProtocol = 0 , TakeFocusProtocol = 1 << 0 , DeleteWindowProtocol = 1 << 1 , PingProtocol = 1 << 2 , SyncRequestProtocol = 1 << 3 , ContextHelpProtocol = 1 << 4 } |
typedef QFlags< Protocol > | Protocols |
enum | RequestSource { FromUnknown = 0 , FromApplication = 1 , FromTool = 2 } |
enum | Role { Client , WindowManager } |
enum | State { Modal = 1u << 0 , Sticky = 1u << 1 , MaxVert = 1u << 2 , MaxHoriz = 1u << 3 , Max = MaxVert | MaxHoriz , Shaded = 1u << 4 , SkipTaskbar = 1u << 5 , KeepAbove = 1u << 6 , SkipPager = 1u << 7 , Hidden = 1u << 8 , FullScreen = 1u << 9 , KeepBelow = 1u << 10 , DemandsAttention = 1u << 11 , SkipSwitcher = 1u << 12 , Focused = 1u << 13 } |
typedef QFlags< State > | States |
enum | WindowType { Unknown = -1 , Normal = 0 , Desktop = 1 , Dock = 2 , Toolbar = 3 , Menu = 4 , Dialog = 5 , Override = 6 , TopMenu = 7 , Utility = 8 , Splash = 9 , DropdownMenu = 10 , PopupMenu = 11 , Tooltip = 12 , Notification = 13 , ComboBox = 14 , DNDIcon = 15 , OnScreenDisplay = 16 , CriticalNotification = 17 , AppletPopup = 18 } |
enum | WindowTypeMask { NormalMask = 1u << 0 , DesktopMask = 1u << 1 , DockMask = 1u << 2 , ToolbarMask = 1u << 3 , MenuMask = 1u << 4 , DialogMask = 1u << 5 , OverrideMask = 1u << 6 , TopMenuMask = 1u << 7 , UtilityMask = 1u << 8 , SplashMask = 1u << 9 , DropdownMenuMask = 1u << 10 , PopupMenuMask = 1u << 11 , TooltipMask = 1u << 12 , NotificationMask = 1u << 13 , ComboBoxMask = 1u << 14 , DNDIconMask = 1u << 15 , OnScreenDisplayMask = 1u << 16 , CriticalNotificationMask = 1u << 17 , AppletPopupMask = 1u << 18 , AllTypesMask = 0U - 1 } |
typedef QFlags< WindowTypeMask > | WindowTypes |
Public Member Functions | |
NETRootInfo (const NETRootInfo &rootinfo) | |
NETRootInfo (xcb_connection_t *connection, NET::Properties properties, NET::Properties2 properties2=NET::Properties2(), int screen=-1, bool doActivate=true) | |
NETRootInfo (xcb_connection_t *connection, xcb_window_t supportWindow, const char *wmName, NET::Properties properties, NET::WindowTypes windowTypes, NET::States states, NET::Properties2 properties2, NET::Actions actions, int screen=-1, bool doActivate=true) | |
virtual | ~NETRootInfo () |
void | activate () |
xcb_window_t | activeWindow () const |
const xcb_window_t * | clientList () const |
int | clientListCount () const |
const xcb_window_t * | clientListStacking () const |
int | clientListStackingCount () const |
void | closeWindowRequest (xcb_window_t window) |
int | currentDesktop (bool ignore_viewport=false) const |
NETSize | desktopGeometry () const |
QSize | desktopLayoutColumnsRows () const |
NET::DesktopLayoutCorner | desktopLayoutCorner () const |
NET::Orientation | desktopLayoutOrientation () const |
const char * | desktopName (int desktop) const |
NETPoint | desktopViewport (int desktop) const |
NET::Properties | event (xcb_generic_event_t *event) |
void | event (xcb_generic_event_t *event, NET::Properties *properties, NET::Properties2 *properties2=nullptr) |
bool | isSupported (NET::Action action) const |
bool | isSupported (NET::Property property) const |
bool | isSupported (NET::Property2 property) const |
bool | isSupported (NET::State state) const |
bool | isSupported (NET::WindowTypeMask type) const |
void | moveResizeRequest (xcb_window_t window, int x_root, int y_root, Direction direction, xcb_button_t button=XCB_BUTTON_INDEX_ANY, RequestSource source=RequestSource::FromUnknown) |
void | moveResizeWindowRequest (xcb_window_t window, int flags, int x, int y, int width, int height) |
int | numberOfDesktops (bool ignore_viewport=false) const |
const NETRootInfo & | operator= (const NETRootInfo &rootinfo) |
NET::Actions | passedActions () const |
NET::Properties | passedProperties () const |
NET::Properties2 | passedProperties2 () const |
NET::States | passedStates () const |
NET::WindowTypes | passedWindowTypes () const |
void | restackRequest (xcb_window_t window, RequestSource source, xcb_window_t above, int detail, xcb_timestamp_t timestamp) |
xcb_window_t | rootWindow () const |
void | sendPing (xcb_window_t window, xcb_timestamp_t timestamp) |
void | setActiveWindow (xcb_window_t window) |
void | setActiveWindow (xcb_window_t window, NET::RequestSource src, xcb_timestamp_t timestamp, xcb_window_t active_window) |
void | setClientList (const xcb_window_t *windows, unsigned int count) |
void | setClientListStacking (const xcb_window_t *windows, unsigned int count) |
void | setCurrentDesktop (int desktop, bool ignore_viewport=false) |
void | setDesktopGeometry (const NETSize &geometry) |
void | setDesktopLayout (NET::Orientation orientation, int columns, int rows, NET::DesktopLayoutCorner corner) |
void | setDesktopName (int desktop, const char *desktopName) |
void | setDesktopViewport (int desktop, const NETPoint &viewport) |
void | setNumberOfDesktops (int numberOfDesktops) |
void | setShowingDesktop (bool showing) |
void | setSupported (NET::Action property, bool on=true) |
void | setSupported (NET::Property property, bool on=true) |
void | setSupported (NET::Property2 property, bool on=true) |
void | setSupported (NET::State property, bool on=true) |
void | setSupported (NET::WindowTypeMask property, bool on=true) |
void | setVirtualRoots (const xcb_window_t *windows, unsigned int count) |
void | setWorkArea (int desktop, const NETRect &workArea) |
bool | showingDesktop () const |
void | showWindowMenuRequest (xcb_window_t window, int device_id, int x_root, int y_root) |
NET::Actions | supportedActions () const |
NET::Properties | supportedProperties () const |
NET::Properties2 | supportedProperties2 () const |
NET::States | supportedStates () const |
NET::WindowTypes | supportedWindowTypes () const |
xcb_window_t | supportWindow () const |
const xcb_window_t * | virtualRoots () const |
int | virtualRootsCount () const |
const char * | wmName () const |
NETRect | workArea (int desktop) const |
xcb_connection_t * | xcbConnection () const |
Protected Member Functions | |
virtual void | addClient (xcb_window_t window) |
virtual void | changeActiveWindow (xcb_window_t window, NET::RequestSource src, xcb_timestamp_t timestamp, xcb_window_t active_window) |
virtual void | changeCurrentDesktop (int desktop) |
virtual void | changeDesktopGeometry (int desktop, const NETSize &geom) |
virtual void | changeDesktopViewport (int desktop, const NETPoint &viewport) |
virtual void | changeNumberOfDesktops (int numberOfDesktops) |
virtual void | changeShowingDesktop (bool showing) |
virtual void | closeWindow (xcb_window_t window) |
virtual void | gotPing (xcb_window_t window, xcb_timestamp_t timestamp) |
virtual void | moveResize (xcb_window_t window, int x_root, int y_root, unsigned long direction, xcb_button_t button, RequestSource source) |
virtual void | moveResizeWindow (xcb_window_t window, int flags, int x, int y, int width, int height) |
virtual void | removeClient (xcb_window_t window) |
virtual void | restackWindow (xcb_window_t window, RequestSource source, xcb_window_t above, int detail, xcb_timestamp_t timestamp) |
virtual void | showWindowMenu (xcb_window_t window, int device_id, int x_root, int y_root) |
virtual void | virtual_hook (int id, void *data) |
Additional Inherited Members | |
![]() | |
static int | timestampCompare (unsigned long time1, unsigned long time2) |
static int | timestampDiff (unsigned long time1, unsigned long time2) |
static bool | typeMatchesMask (WindowType type, WindowTypes mask) |
Detailed Description
Common API for root window properties/protocols.
The NETRootInfo class provides a common API for clients and window managers to set/read/change properties on the root window as defined by the NET Window Manager Specification..
- See also
- NET
- NETWinInfo
Member Enumeration Documentation
◆ anonymous enum
Constructor & Destructor Documentation
◆ NETRootInfo() [1/3]
NETRootInfo::NETRootInfo | ( | xcb_connection_t * | connection, |
xcb_window_t | supportWindow, | ||
const char * | wmName, | ||
NET::Properties | properties, | ||
NET::WindowTypes | windowTypes, | ||
NET::States | states, | ||
NET::Properties2 | properties2, | ||
NET::Actions | actions, | ||
int | screen = -1, | ||
bool | doActivate = true ) |
Window Managers should use this constructor to create a NETRootInfo object, which will be used to set/update information stored on the rootWindow.
The application role is automatically set to WindowManager when using this constructor.
- Parameters
-
connection XCB connection supportWindow The Window id of the supportWindow. The supportWindow must be created by the window manager as a child of the rootWindow. The supportWindow must not be destroyed until the Window Manager exits. wmName A string which should be the window manager's name (ie. "KWin" or "Blackbox"). properties The properties the window manager supports windowTypes The window types the window manager supports states The states the window manager supports properties2 The properties2 the window manager supports actions The actions the window manager supports screen For Window Managers that support multiple screen (ie. "multiheaded") displays, the screen number may be explicitly defined. If this argument is omitted, the default screen will be used. doActivate true to activate the window
◆ NETRootInfo() [2/3]
NETRootInfo::NETRootInfo | ( | xcb_connection_t * | connection, |
NET::Properties | properties, | ||
NET::Properties2 | properties2 = NET::Properties2(), | ||
int | screen = -1, | ||
bool | doActivate = true ) |
Clients should use this constructor to create a NETRootInfo object, which will be used to query information set on the root window.
The application role is automatically set to Client when using this constructor.
- Parameters
-
connection XCB connection properties The properties the client is interested in. properties2 The properties2 the client is interested in. properties_size The number of elements in the properties array. screen For Clients that support multiple screen (ie. "multiheaded") displays, the screen number may be explicitly defined. If this argument is omitted, the default screen will be used. doActivate true to call activate() to do an initial data read/update of the query information.
◆ NETRootInfo() [3/3]
NETRootInfo::NETRootInfo | ( | const NETRootInfo & | rootinfo | ) |
Creates a shared copy of the specified NETRootInfo object.
- Parameters
-
rootinfo the NETRootInfo object to copy
◆ ~NETRootInfo()
|
virtual |
Destroys the NETRootInfo object.
Member Function Documentation
◆ activate()
void NETRootInfo::activate | ( | ) |
Window Managers must call this after creating the NETRootInfo object, and before using any other method in the class.
This method sets initial data on the root window and does other post-construction duties.
Clients must also call this after creating the object to do an initial data read/update.
◆ activeWindow()
xcb_window_t NETRootInfo::activeWindow | ( | ) | const |
◆ addClient()
|
inlineprotectedvirtual |
A Client should subclass NETRootInfo and reimplement this function when it wants to know when a window has been added.
- Parameters
-
window the id of the window to add
◆ changeActiveWindow()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the active (focused) window.
- Parameters
-
window the id of the window to activate src the source from which the request came timestamp the timestamp of the user action causing this request active_window active window of the requesting application, if any
◆ changeCurrentDesktop()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the current desktop.
- Parameters
-
desktop the number of the desktop
◆ changeDesktopGeometry()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the specified desktop geometry.
- Parameters
-
desktop the number of the desktop geom the new size
◆ changeDesktopViewport()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the specified desktop viewport.
- Parameters
-
desktop the number of the desktop viewport the new position of the viewport
◆ changeNumberOfDesktops()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to change the number of desktops.
- Parameters
-
numberOfDesktops the new number of desktops
◆ changeShowingDesktop()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a pager made a request to change showing the desktop.
See _NET_SHOWING_DESKTOP for details.
- Parameters
-
showing whether to activate the showing desktop mode
◆ clientList()
const xcb_window_t * NETRootInfo::clientList | ( | ) | const |
Returns an array of Window id's, which contain all managed windows.
- Returns
- the array of Window id's
- See also
- clientListCount()
◆ clientListCount()
int NETRootInfo::clientListCount | ( | ) | const |
Returns the number of managed windows in clientList array.
- Returns
- the number of managed windows in the clientList array
- See also
- clientList()
◆ clientListStacking()
const xcb_window_t * NETRootInfo::clientListStacking | ( | ) | const |
Returns an array of Window id's, which contain all managed windows in stacking order.
- Returns
- the array of Window id's in stacking order
- See also
- clientListStackingCount()
◆ clientListStackingCount()
int NETRootInfo::clientListStackingCount | ( | ) | const |
Returns the number of managed windows in the clientListStacking array.
- Returns
- the number of Window id's in the client list
- See also
- clientListStacking()
◆ closeWindow()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to close a window.
- Parameters
-
window the id of the window to close
◆ closeWindowRequest()
void NETRootInfo::closeWindowRequest | ( | xcb_window_t | window | ) |
◆ currentDesktop()
int NETRootInfo::currentDesktop | ( | bool | ignore_viewport = false | ) | const |
Returns the current desktop.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. They are however mapped to virtual desktops if needed.
- Parameters
-
ignore_viewport if false, viewport is mapped to virtual desktops
- Returns
- the number of the current desktop
◆ desktopGeometry()
NETSize NETRootInfo::desktopGeometry | ( | ) | const |
◆ desktopLayoutColumnsRows()
QSize NETRootInfo::desktopLayoutColumnsRows | ( | ) | const |
◆ desktopLayoutCorner()
NET::DesktopLayoutCorner NETRootInfo::desktopLayoutCorner | ( | ) | const |
◆ desktopLayoutOrientation()
NET::Orientation NETRootInfo::desktopLayoutOrientation | ( | ) | const |
◆ desktopName()
const char * NETRootInfo::desktopName | ( | int | desktop | ) | const |
◆ desktopViewport()
NETPoint NETRootInfo::desktopViewport | ( | int | desktop | ) | const |
Returns the viewport of the specified desktop.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. You should use calls for virtual desktops, viewport is mapped to them if needed.
- Parameters
-
desktop the number of the desktop
- Returns
- the position of the desktop's viewport
◆ event() [1/2]
NET::Properties NETRootInfo::event | ( | xcb_generic_event_t * | event | ) |
This function takes the passed XEvent and returns an OR'ed list of NETRootInfo properties that have changed.
The new information will be read immediately by the class. This overloaded version returns only a single mask, and therefore cannot check state of all properties like the other variant.
- Parameters
-
event the event
- Returns
- the properties
◆ event() [2/2]
void NETRootInfo::event | ( | xcb_generic_event_t * | event, |
NET::Properties * | properties, | ||
NET::Properties2 * | properties2 = nullptr ) |
This function takes the passed xcb_generic_event_t and returns the updated properties in the passed in arguments.
The new information will be read immediately by the class. It is possible to pass in a null pointer in the arguments. In that case the passed in argument will obviously not be updated, but the class will process the information nevertheless.
- Parameters
-
event the event properties The NET::Properties that changed properties2 The NET::Properties2 that changed
- Since
- 5.0
◆ gotPing()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to receive replies to the _NET_WM_PING protocol.
- Parameters
-
window the window from which the reply came timestamp timestamp of the ping
◆ isSupported() [1/5]
bool NETRootInfo::isSupported | ( | NET::Action | action | ) | const |
◆ isSupported() [2/5]
bool NETRootInfo::isSupported | ( | NET::Property | property | ) | const |
◆ isSupported() [3/5]
bool NETRootInfo::isSupported | ( | NET::Property2 | property | ) | const |
◆ isSupported() [4/5]
bool NETRootInfo::isSupported | ( | NET::State | state | ) | const |
◆ isSupported() [5/5]
bool NETRootInfo::isSupported | ( | NET::WindowTypeMask | type | ) | const |
◆ moveResize()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to start a move/resize.
- Parameters
-
window The window that wants to move/resize x_root X position of the cursor relative to the root window. y_root Y position of the cursor relative to the root window. direction One of NET::Direction (see base class documentation for a description of the different directions). button the button which should be pressed. source who initiated the move resize operation.
◆ moveResizeRequest()
void NETRootInfo::moveResizeRequest | ( | xcb_window_t | window, |
int | x_root, | ||
int | y_root, | ||
Direction | direction, | ||
xcb_button_t | button = XCB_BUTTON_INDEX_ANY, | ||
RequestSource | source = RequestSource::FromUnknown ) |
Clients (such as pagers/taskbars) that wish to start a WMMoveResize (where the window manager controls the resize/movement, i.e.
_NET_WM_MOVERESIZE) should call this function. This will send a request to the Window Manager.
- Parameters
-
window The client window that would be resized/moved. x_root X position of the cursor relative to the root window. y_root Y position of the cursor relative to the root window. direction One of NET::Direction (see base class documentation for a description of the different directions). button the button which should be pressed. source who initiated the move resize operation.
◆ moveResizeWindow()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a pager made a request to move/resize a window.
See _NET_MOVERESIZE_WINDOW for details.
- Parameters
-
window the id of the window to more/resize flags Flags specifying the operation (see _NET_MOVERESIZE_WINDOW description) x Requested X position for the window y Requested Y position for the window width Requested width for the window height Requested height for the window
◆ moveResizeWindowRequest()
void NETRootInfo::moveResizeWindowRequest | ( | xcb_window_t | window, |
int | flags, | ||
int | x, | ||
int | y, | ||
int | width, | ||
int | height ) |
Clients (such as pagers/taskbars) that wish to move/resize a window using WM2MoveResizeWindow (_NET_MOVERESIZE_WINDOW) should call this function.
This will send a request to the Window Manager. See _NET_MOVERESIZE_WINDOW description for details.
- Parameters
-
window The client window that would be resized/moved. flags Flags specifying the operation (see _NET_MOVERESIZE_WINDOW description) x Requested X position for the window y Requested Y position for the window width Requested width for the window height Requested height for the window
◆ numberOfDesktops()
int NETRootInfo::numberOfDesktops | ( | bool | ignore_viewport = false | ) | const |
Returns the number of desktops.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. They are however mapped to virtual desktops if needed.
- Parameters
-
ignore_viewport if false, viewport is mapped to virtual desktops
- Returns
- the number of desktops
◆ operator=()
const NETRootInfo & NETRootInfo::operator= | ( | const NETRootInfo & | rootinfo | ) |
◆ passedActions()
NET::Actions NETRootInfo::passedActions | ( | ) | const |
- Returns
- the actions argument passed to the constructor.
- Since
- 5.0
◆ passedProperties()
NET::Properties NETRootInfo::passedProperties | ( | ) | const |
- Returns
- the properties argument passed to the constructor.
◆ passedProperties2()
NET::Properties2 NETRootInfo::passedProperties2 | ( | ) | const |
- Returns
- the properties2 argument passed to the constructor.
- Since
- 5.0
◆ passedStates()
NET::States NETRootInfo::passedStates | ( | ) | const |
- Returns
- the states argument passed to the constructor.
- Since
- 5.0
◆ passedWindowTypes()
NET::WindowTypes NETRootInfo::passedWindowTypes | ( | ) | const |
- Returns
- the windowTypes argument passed to the constructor.
- Since
- 5.0
◆ removeClient()
|
inlineprotectedvirtual |
A Client should subclass NETRootInfo and reimplement this function when it wants to know when a window has been removed.
- Parameters
-
window the id of the window to remove
◆ restackRequest()
void NETRootInfo::restackRequest | ( | xcb_window_t | window, |
RequestSource | source, | ||
xcb_window_t | above, | ||
int | detail, | ||
xcb_timestamp_t | timestamp ) |
◆ restackWindow()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to restack a window.
See _NET_RESTACK_WINDOW for details.
- Parameters
-
window the id of the window to restack source the source of the request above other window in the restack request detail restack detail timestamp the timestamp of the request
◆ rootWindow()
xcb_window_t NETRootInfo::rootWindow | ( | ) | const |
◆ sendPing()
void NETRootInfo::sendPing | ( | xcb_window_t | window, |
xcb_timestamp_t | timestamp ) |
◆ setActiveWindow() [1/2]
void NETRootInfo::setActiveWindow | ( | xcb_window_t | window | ) |
◆ setActiveWindow() [2/2]
void NETRootInfo::setActiveWindow | ( | xcb_window_t | window, |
NET::RequestSource | src, | ||
xcb_timestamp_t | timestamp, | ||
xcb_window_t | active_window ) |
Requests that the specified window becomes the active (focused) one.
- Parameters
-
window the id of the new active window src whether the request comes from normal application or from a pager or similar tool timestamp X server timestamp of the user action that caused the request active_window active window of the requesting application, if any
◆ setClientList()
void NETRootInfo::setClientList | ( | const xcb_window_t * | windows, |
unsigned int | count ) |
◆ setClientListStacking()
void NETRootInfo::setClientListStacking | ( | const xcb_window_t * | windows, |
unsigned int | count ) |
◆ setCurrentDesktop()
void NETRootInfo::setCurrentDesktop | ( | int | desktop, |
bool | ignore_viewport = false ) |
Sets the current desktop to the specified desktop.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. It is however mapped to virtual desktops if needed.
- Parameters
-
desktop the number of the desktop ignore_viewport if false, viewport is mapped to virtual desktops
◆ setDesktopGeometry()
void NETRootInfo::setDesktopGeometry | ( | const NETSize & | geometry | ) |
Sets the desktop geometry to the specified geometry.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. You should use calls for virtual desktops, viewport is mapped to them if needed.
- Parameters
-
geometry the new size of the desktop
◆ setDesktopLayout()
void NETRootInfo::setDesktopLayout | ( | NET::Orientation | orientation, |
int | columns, | ||
int | rows, | ||
NET::DesktopLayoutCorner | corner ) |
◆ setDesktopName()
void NETRootInfo::setDesktopName | ( | int | desktop, |
const char * | desktopName ) |
Sets the name of the specified desktop.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. Viewport is mapped to virtual desktops if needed, but not for this call.
- Parameters
-
desktop the number of the desktop desktopName the new name of the desktop
◆ setDesktopViewport()
void NETRootInfo::setDesktopViewport | ( | int | desktop, |
const NETPoint & | viewport ) |
Sets the viewport for the current desktop to the specified point.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. You should use calls for virtual desktops, viewport is mapped to them if needed.
- Parameters
-
desktop the number of the desktop viewport the new position of the desktop's viewport
◆ setNumberOfDesktops()
void NETRootInfo::setNumberOfDesktops | ( | int | numberOfDesktops | ) |
Sets the number of desktops to the specified number.
NOTE: KDE uses virtual desktops and does not directly support viewport in any way. Viewport is mapped to virtual desktops if needed, but not for this call.
- Parameters
-
numberOfDesktops the number of desktops
◆ setShowingDesktop()
void NETRootInfo::setShowingDesktop | ( | bool | showing | ) |
◆ setSupported() [1/5]
void NETRootInfo::setSupported | ( | NET::Action | property, |
bool | on = true ) |
◆ setSupported() [2/5]
void NETRootInfo::setSupported | ( | NET::Property | property, |
bool | on = true ) |
◆ setSupported() [3/5]
void NETRootInfo::setSupported | ( | NET::Property2 | property, |
bool | on = true ) |
◆ setSupported() [4/5]
void NETRootInfo::setSupported | ( | NET::State | property, |
bool | on = true ) |
◆ setSupported() [5/5]
void NETRootInfo::setSupported | ( | NET::WindowTypeMask | property, |
bool | on = true ) |
◆ setVirtualRoots()
void NETRootInfo::setVirtualRoots | ( | const xcb_window_t * | windows, |
unsigned int | count ) |
◆ setWorkArea()
void NETRootInfo::setWorkArea | ( | int | desktop, |
const NETRect & | workArea ) |
◆ showingDesktop()
bool NETRootInfo::showingDesktop | ( | ) | const |
◆ showWindowMenu()
|
inlineprotectedvirtual |
A Window Manager should subclass NETRootInfo and reimplement this function when it wants to know when a Client made a request to show a window menu.
- Parameters
-
window The window that wants to move/resize device_id GTK device id. x_root X position of the cursor relative to the root window. y_root Y position of the cursor relative to the root window.
◆ showWindowMenuRequest()
void NETRootInfo::showWindowMenuRequest | ( | xcb_window_t | window, |
int | device_id, | ||
int | x_root, | ||
int | y_root ) |
Clients that wish to show the window menu using WM2GTKShowWindowMenu (_GTK_SHOW_WINDOW_MENU) should call this function.
This will send a request to the Window Manager. See _GTK_SHOW_WINDOW_MENU description for details.
- Parameters
-
window The client window that would be resized/moved. device_id GTK device id x Requested X position for the menu relative to the root window y Requested Y position for the menu relative to the root window
◆ supportedActions()
NET::Actions NETRootInfo::supportedActions | ( | ) | const |
In the Window Manager mode, this is equivalent to the actions argument passed to the constructor.
In the Client mode, if NET::Supported was passed in the properties argument, the returned value are all actions supported by the Window Manager. Other supported protocols and properties are returned by the specific methods.
- Since
- 5.0
◆ supportedProperties()
NET::Properties NETRootInfo::supportedProperties | ( | ) | const |
In the Window Manager mode, this is equivalent to the properties argument passed to the constructor.
In the Client mode, if NET::Supported was passed in the properties argument, the returned value are all properties supported by the Window Manager. Other supported protocols and properties are returned by the specific methods.
◆ supportedProperties2()
NET::Properties2 NETRootInfo::supportedProperties2 | ( | ) | const |
In the Window Manager mode, this is equivalent to the properties2 argument passed to the constructor.
In the Client mode, if NET::Supported was passed in the properties argument, the returned value are all properties2 supported by the Window Manager. Other supported protocols and properties are returned by the specific methods.
- Since
- 5.0
◆ supportedStates()
NET::States NETRootInfo::supportedStates | ( | ) | const |
In the Window Manager mode, this is equivalent to the states argument passed to the constructor.
In the Client mode, if NET::Supported was passed in the properties argument, the returned value are all states supported by the Window Manager. Other supported protocols and properties are returned by the specific methods.
- Since
- 5.0
◆ supportedWindowTypes()
NET::WindowTypes NETRootInfo::supportedWindowTypes | ( | ) | const |
In the Window Manager mode, this is equivalent to the windowTypes argument passed to the constructor.
In the Client mode, if NET::Supported was passed in the properties argument, the returned value are all window types supported by the Window Manager. Other supported protocols and properties are returned by the specific methods.
- Since
- 5.0
◆ supportWindow()
xcb_window_t NETRootInfo::supportWindow | ( | ) | const |
◆ virtual_hook()
|
protectedvirtual |
◆ virtualRoots()
const xcb_window_t * NETRootInfo::virtualRoots | ( | ) | const |
Returns an array of Window id's, which contain the virtual root windows.
- Returns
- the array of Window id's
- See also
- virtualRootsCount()
◆ virtualRootsCount()
int NETRootInfo::virtualRootsCount | ( | ) | const |
Returns the number of window in the virtualRoots array.
- Returns
- the number of Window id's in the virtual root array
- See also
- virtualRoots()
◆ wmName()
const char * NETRootInfo::wmName | ( | ) | const |
◆ workArea()
NETRect NETRootInfo::workArea | ( | int | desktop | ) | const |
◆ xcbConnection()
xcb_connection_t * NETRootInfo::xcbConnection | ( | ) | const |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:14:35 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.