Dialog
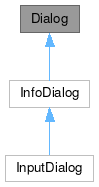
Properties | |
real | absoluteMaximumHeight |
real | absoluteMaximumWidth |
listTAction | customFooterActions |
alias | dialogChildren |
alias | dialogData |
bool | flatFooterButtons |
Component | footerLeadingComponent |
Component | footerTrailingComponent |
real | maximumHeight |
real | maximumWidth |
real | preferredHeight |
real | preferredWidth |
bool | showCloseButton |
Public Member Functions | |
void | customFooterButton (T.Action action) |
void | standardButton (button) |
Detailed Description
Popup dialog that is used for short tasks and user interaction.
Dialog consists of three components: the header, the content, and the footer.
By default, the header is a heading with text specified by the title
property.
By default, the footer consists of a row of buttons specified by the standardButtons
and customFooterActions
properties.
The implicitHeight
and implicitWidth
of the dialog contentItem is the primary hint used for the dialog size. The dialog will be the minimum size required for the header, footer and content unless it is larger than maximumHeight
and maximumWidth
. Use preferredHeight
and preferredWidth
in order to manually specify a size for the dialog.
If the content height exceeds the maximum height of the dialog, the dialog's contents will become scrollable.
If the contentItem is a ListView, the dialog will take care of the necessary scrollbars and scrolling behaviour. Do not attempt to nest ListViews (it must be the top level item), as the scrolling behaviour will not be handled. Use ListView's header
and footer
instead.
Example for a selection dialog:
Example with scrolling (ListView scrolling behaviour is handled by the Dialog):
There are also sub-components of the Dialog that target specific usecases, and can reduce boilerplate code if used:
- See also
- PromptDialog
- MenuDialog
Definition at line 105 of file Dialog.qml.
Property Documentation
◆ absoluteMaximumHeight
|
read |
This property sets the absolute maximum height the dialog can have.
The height restriction is solely applied on the content, so if the maximum height given is not larger than the height of the header and footer, it will be ignored.
This is the window height, subtracted by largeSpacing on both the top and bottom.
- Remarks
- This property is read-only
Definition at line 133 of file Dialog.qml.
◆ absoluteMaximumWidth
|
read |
This property holds the absolute maximum width the dialog can have.
By default, it is the window width, subtracted by largeSpacing on both the top and bottom.
- Remarks
- This property is read-only
Definition at line 141 of file Dialog.qml.
◆ customFooterActions
|
read |
This property holds the custom actions displayed in the footer.
Example usage:
- See also
- org::kde::kirigami::Action
Definition at line 225 of file Dialog.qml.
◆ dialogChildren
|
read |
This property holds the content items of the dialog.
The initial height and width of the dialog is calculated from the implicitWidth
and implicitHeight
of the content.
Definition at line 121 of file Dialog.qml.
◆ dialogData
|
read |
This property holds the dialog's contents; includes Items and QtObjects.
- Remarks
- This is the default property
Definition at line 112 of file Dialog.qml.
◆ flatFooterButtons
|
read |
This property sets whether the footer button style should be flat.
Definition at line 194 of file Dialog.qml.
◆ footerLeadingComponent
|
read |
This property holds the component to the left of the footer buttons.
Definition at line 182 of file Dialog.qml.
◆ footerTrailingComponent
|
read |
his property holds the component to the right of the footer buttons.
Definition at line 186 of file Dialog.qml.
◆ maximumHeight
|
read |
This property holds the maximum height the dialog can have (including the header and footer).
The height restriction is solely enforced on the content, so if the maximum height given is not larger than the height of the header and footer, it will be ignored.
By default, this is absoluteMaximumHeight
.
Definition at line 152 of file Dialog.qml.
◆ maximumWidth
|
read |
This property holds the maximum width the dialog can have.
By default, this is absoluteMaximumWidth
.
Definition at line 158 of file Dialog.qml.
◆ preferredHeight
|
read |
This property holds the preferred height of the dialog.
The content will receive a hint for how tall it should be to have the dialog to be this height.
If the content, header or footer require more space, then the height of the dialog will expand to the necessary amount of space.
Definition at line 168 of file Dialog.qml.
◆ preferredWidth
|
read |
This property holds the preferred width of the dialog.
The content will receive a hint for how wide it should be to have the dialog be this wide.
If the content, header or footer require more space, then the width of the dialog will expand to the necessary amount of space.
Definition at line 178 of file Dialog.qml.
◆ showCloseButton
|
read |
This property sets whether to show the close button in the header.
Definition at line 190 of file Dialog.qml.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:18:46 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.