KActionCategory
#include <KActionCategory>
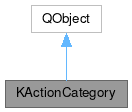
Properties | |
QString | text |
![]() | |
objectName | |
Adding Actions | |
Add a action to the category. This methods are provided for your convenience. They call the corresponding method of KActionCollection. | |
QAction * | addAction (const QString &name, QAction *action) |
QAction * | addAction (KStandardAction::StandardAction actionType, const QObject *receiver=nullptr, const char *member=nullptr) |
QAction * | addAction (KStandardAction::StandardAction actionType, const QString &name, const QObject *receiver=nullptr, const char *member=nullptr) |
QAction * | addAction (const QString &name, const QObject *receiver=nullptr, const char *member=nullptr) |
template<class ActionType > | |
ActionType * | add (const QString &name, const QObject *receiver=nullptr, const char *member=nullptr) |
const QList< QAction * > | actions () const |
KActionCollection * | collection () const |
QString | text () const |
void | setText (const QString &text) |
Detailed Description
Categorize actions for KShortcutsEditor.
KActionCategory provides a second level to organize the actions in KShortcutsEditor.
The first possibility is using more than one action collection. Each actions collection becomes a top level node.
- action collection 1
- first action
- second action
- third action
- action collection 2
- first action
- second action
- third action
Using KActionCategory it's possible to group the actions of one collection.
- action collection 1
- first action
- first category
- action 1 in category
- action 2 in category
- second action
Usage
The usage is analog to action collections. Just create a category and use it instead of the collection to create the actions.
The synchronization between KActionCollection and KActionCategory is done internally. There is for example no need to remove actions from a category. It is done implicitly if the action is removed from the associated collection.
Definition at line 84 of file kactioncategory.h.
Property Documentation
◆ text
|
readwrite |
Definition at line 88 of file kactioncategory.h.
Constructor & Destructor Documentation
◆ KActionCategory()
|
explicit |
Default constructor.
Definition at line 25 of file kactioncategory.cpp.
◆ ~KActionCategory()
|
overridedefault |
Destructor.
Member Function Documentation
◆ actions()
Returns the actions belonging to this category.
Definition at line 34 of file kactioncategory.cpp.
◆ add()
|
inline |
Definition at line 119 of file kactioncategory.h.
◆ addAction() [1/4]
QAction * KActionCategory::addAction | ( | const QString & | name, |
const QObject * | receiver = nullptr, | ||
const char * | member = nullptr ) |
Definition at line 60 of file kactioncategory.cpp.
◆ addAction() [2/4]
Definition at line 39 of file kactioncategory.cpp.
◆ addAction() [3/4]
QAction * KActionCategory::addAction | ( | KStandardAction::StandardAction | actionType, |
const QObject * | receiver = nullptr, | ||
const char * | member = nullptr ) |
Definition at line 46 of file kactioncategory.cpp.
◆ addAction() [4/4]
QAction * KActionCategory::addAction | ( | KStandardAction::StandardAction | actionType, |
const QString & | name, | ||
const QObject * | receiver = nullptr, | ||
const char * | member = nullptr ) |
Definition at line 53 of file kactioncategory.cpp.
◆ collection()
KActionCollection * KActionCategory::collection | ( | ) | const |
The action collection this category is associated with.
Definition at line 75 of file kactioncategory.cpp.
◆ setText()
Set the action categorys descriptive text.
Definition at line 85 of file kactioncategory.cpp.
◆ text()
QString KActionCategory::text | ( | ) | const |
The action categorys descriptive text.
Definition at line 80 of file kactioncategory.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:21:12 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.