KHelpMenu
#include <KHelpMenu>
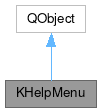
Public Types | |
enum | MenuId { menuHelpContents = 0 , menuWhatsThis = 1 , menuAboutApp = 2 , menuAboutKDE = 3 , menuReportBug = 4 , menuSwitchLanguage = 5 , menuDonate = 6 } |
Signals | |
void | showAboutApplication () |
Public Slots | |
void | aboutApplication () |
void | aboutKDE () |
void | appHelpActivated () |
void | contextHelpActivated () |
void | donate () |
void | reportBug () |
void | switchApplicationLanguage () |
Public Member Functions | |
KHelpMenu (QWidget *parent, const KAboutData &aboutData, bool showWhatsThis=true) | |
KHelpMenu (QWidget *parent=nullptr, const QString &aboutAppText=QString(), bool showWhatsThis=true) | |
~KHelpMenu () override | |
QAction * | action (MenuId id) const |
QMenu * | menu () |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Standard KDE help menu with dialog boxes.
This class provides the standard KDE help menu with the default "about" dialog boxes and help entry.
This class is used in KMainWindow so normally you don't need to use this class yourself. However, if you need the help menu or any of its dialog boxes in your code that is not subclassed from KMainWindow you should use this class.
The usage is simple:
or if you just want to open a dialog box:
IMPORTANT: The first time you use KHelpMenu::menu(), a QMenu object is allocated. Only one object is created by the class so if you call KHelpMenu::menu() twice or more, the same pointer is returned. The class will destroy the popupmenu in the destructor so do not delete this pointer yourself.
The KHelpMenu object will be deleted when its parent is destroyed but you can delete it yourself if you want. The code below will always work.
Using your own "about application" dialog box:
The standard "about application" dialog box is quite simple. If you need a dialog box with more functionality you must design that one yourself. When you want to display the dialog, you simply need to connect the help menu signal showAboutApplication() to your slot.
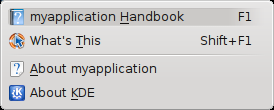
KHelpMenu respects Kiosk settings (see the KAuthorized namespace in the KConfig framework). In particular, system administrators can disable items on this menu using some subset of the following configuration:
[KDE Action Restrictions][$i] actions/help_contents=false actions/help_whats_this=false actions/help_report_bug=false actions/switch_application_language=false actions/help_about_app=false actions/help_about_kde=false
Definition at line 108 of file khelpmenu.h.
Member Enumeration Documentation
◆ MenuId
enum KHelpMenu::MenuId |
Enumerator | |
---|---|
menuDonate |
|
Definition at line 161 of file khelpmenu.h.
Constructor & Destructor Documentation
◆ KHelpMenu() [1/2]
|
explicit |
Constructor.
- Parameters
-
parent The parent of the dialog boxes. The boxes are modeless and will be centered with respect to the parent. aboutAppText User definable string that is used in the default application dialog box. showWhatsThis Decides whether a "What's this" entry will be added to the dialog.
Definition at line 79 of file khelpmenu.cpp.
◆ KHelpMenu() [2/2]
KHelpMenu::KHelpMenu | ( | QWidget * | parent, |
const KAboutData & | aboutData, | ||
bool | showWhatsThis = true ) |
Constructor.
This alternative constructor is mainly useful if you want to override the standard actions (aboutApplication(), aboutKDE(), helpContents(), reportBug, and optionally whatsThis).
- Parameters
-
parent The parent of the dialog boxes. The boxes are modeless and will be centered with respect to the parent. aboutData User and app data used in the About app dialog showWhatsThis Decides whether a "What's this" entry will be added to the dialog.
Definition at line 89 of file khelpmenu.cpp.
◆ ~KHelpMenu()
|
override |
Destructor.
Destroys dialogs and the menu pointer returned by menu
Definition at line 99 of file khelpmenu.cpp.
Member Function Documentation
◆ aboutApplication
|
slot |
Opens an application specific dialog box.
The method will try to open the about box using the following steps:
- If the showAboutApplication() signal is connected, then it will be called. This means there is an application defined aboutBox.
- If the aboutData was set in the constructor a KAboutApplicationDialog will be created.
- Else a default about box using the aboutAppText from the constructor will be created.
Definition at line 234 of file khelpmenu.cpp.
◆ aboutKDE
|
slot |
Opens the standard "About KDE" dialog box.
Definition at line 247 of file khelpmenu.cpp.
◆ action()
Returns the QAction * associated with the given parameter Will return a nullptr if menu() has not been called.
- Parameters
-
id The id of the action of which you want to get QAction *
Definition at line 201 of file khelpmenu.cpp.
◆ appHelpActivated
|
slot |
Opens the help page for the application.
The application name is used as a key to determine what to display and the system will attempt to open <appName>/index.html.
Definition at line 229 of file khelpmenu.cpp.
◆ contextHelpActivated
|
slot |
Activates What's This help for the application.
Definition at line 312 of file khelpmenu.cpp.
◆ donate
|
slot |
◆ menu()
QMenu * KHelpMenu::menu | ( | ) |
Returns a popup menu you can use in the menu bar or where you need it.
The returned menu is configured with an icon, a title and menu entries. Therefore adding the returned pointer to your menu is enough to have access to the help menu.
Note: This method will only create one instance of the menu. If you call this method twice or more the same pointer is returned.
Definition at line 140 of file khelpmenu.cpp.
◆ reportBug
|
slot |
Opens the standard "Report Bugs" dialog box.
Definition at line 256 of file khelpmenu.cpp.
◆ showAboutApplication
|
signal |
This signal is emitted from aboutApplication() if no "about application" string has been defined.
The standard application specific dialog box that is normally activated in aboutApplication() will not be displayed when this signal is emitted.
◆ switchApplicationLanguage
|
slot |
Opens the changing default application language dialog box.
Definition at line 265 of file khelpmenu.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Jul 26 2024 11:51:48 by doxygen 1.11.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.