NetworkManager::ActiveConnection
#include <activeconnection.h>
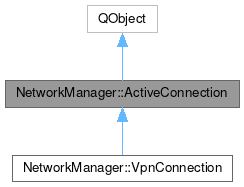
Public Types | |
typedef QList< Ptr > | List |
typedef QSharedPointer< ActiveConnection > | Ptr |
enum | Reason { UknownReason = 0 , None , UserDisconnected , DeviceDisconnected , ServiceStopped , IpConfigInvalid , ConnectTimeout , ServiceStartTimeout , ServiceStartFailed , NoSecrets , LoginFailed , ConnectionRemoved , DependencyFailed , DeviceRealizeFailed , DeviceRemoved } |
enum | State { Unknown = 0 , Activating , Activated , Deactivating , Deactivated } |
![]() | |
typedef | QObjectList |
Signals | |
void | connectionChanged (const NetworkManager::Connection::Ptr &connection) |
void | default4Changed (bool isDefault) |
void | default6Changed (bool isDefault) |
void | devicesChanged () |
void | dhcp4ConfigChanged () |
void | dhcp6ConfigChanged () |
void | idChanged (const QString &id) |
void | ipV4ConfigChanged () |
void | ipV6ConfigChanged () |
void | masterChanged (const QString &uni) |
void | specificObjectChanged (const QString &path) |
void | stateChanged (NetworkManager::ActiveConnection::State state) |
void | stateChangedReason (NetworkManager::ActiveConnection::State state, NetworkManager::ActiveConnection::Reason reason) |
void | typeChanged (NetworkManager::ConnectionSettings::ConnectionType type) |
void | uuidChanged (const QString &uuid) |
void | vpnChanged (bool isVpn) |
Public Member Functions | |
ActiveConnection (const QString &path, QObject *parent=nullptr) | |
~ActiveConnection () override | |
Connection::Ptr | connection () const |
bool | default4 () const |
bool | default6 () const |
QStringList | devices () const |
Dhcp4Config::Ptr | dhcp4Config () const |
Dhcp6Config::Ptr | dhcp6Config () const |
QString | id () const |
IpConfig | ipV4Config () const |
IpConfig | ipV6Config () const |
bool | isValid () const |
QString | master () const |
QString | path () const |
QString | specificObject () const |
NetworkManager::ActiveConnection::State | state () const |
NetworkManager::ConnectionSettings::ConnectionType | type () const |
QString | uuid () const |
bool | vpn () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
NETWORKMANAGERQT_NO_EXPORT | ActiveConnection (ActiveConnectionPrivate &dd, QObject *parent=nullptr) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
ActiveConnectionPrivate *const | d_ptr |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
An active connection.
Definition at line 28 of file activeconnection.h.
Member Typedef Documentation
◆ List
Definition at line 34 of file activeconnection.h.
◆ Ptr
Definition at line 33 of file activeconnection.h.
Member Enumeration Documentation
◆ Reason
Definition at line 46 of file activeconnection.h.
◆ State
Enum describing possible active connection states.
Definition at line 38 of file activeconnection.h.
Constructor & Destructor Documentation
◆ ActiveConnection() [1/2]
Creates a new ActiveConnection object.
- Parameters
-
path the DBus path of the device
Definition at line 48 of file activeconnection.cpp.
◆ ~ActiveConnection()
|
override |
Destroys an ActiveConnection object.
Definition at line 108 of file activeconnection.cpp.
◆ ActiveConnection() [2/2]
|
explicitprotected |
Definition at line 81 of file activeconnection.cpp.
Member Function Documentation
◆ connection()
NetworkManager::Connection::Ptr ActiveConnection::connection | ( | ) | const |
Returns a valid NetworkManager::Connection object.
Definition at line 119 of file activeconnection.cpp.
◆ connectionChanged
|
signal |
This signal is emitted when the connection path has changed.
◆ default4()
bool ActiveConnection::default4 | ( | ) | const |
Whether this connection has the default IPv4 route.
Definition at line 131 of file activeconnection.cpp.
◆ default4Changed
|
signal |
The state of the default IPv4 route changed.
◆ default6()
bool ActiveConnection::default6 | ( | ) | const |
Whether this connection has the default IPv6 route.
Definition at line 137 of file activeconnection.cpp.
◆ default6Changed
|
signal |
The state of the default IPv6 route changed.
◆ devices()
QStringList ActiveConnection::devices | ( | ) | const |
List of devices UNIs which are part of this connection.
Definition at line 221 of file activeconnection.cpp.
◆ devicesChanged
|
signal |
The list of devices changed.
◆ dhcp4Config()
NetworkManager::Dhcp4Config::Ptr ActiveConnection::dhcp4Config | ( | ) | const |
The Dhcp4Config object describing the DHCP options returned by the DHCP server (assuming the connection used DHCP).
Only valid when the connection is in the NM_ACTIVE_CONNECTION_STATE_ACTIVATED state
Definition at line 143 of file activeconnection.cpp.
◆ dhcp4ConfigChanged
|
signal |
Emitted when the DHCP configuration for IPv4 of this network has changed.
◆ dhcp6Config()
NetworkManager::Dhcp6Config::Ptr ActiveConnection::dhcp6Config | ( | ) | const |
The Dhcp6Config object describing the DHCP options returned by the DHCP server (assuming the connection used DHCP).
Only valid when the connection is in the NM_ACTIVE_CONNECTION_STATE_ACTIVATED state
Definition at line 152 of file activeconnection.cpp.
◆ dhcp6ConfigChanged
|
signal |
Emitted when the DHCP configuration for IPv6 of this network has changed.
◆ id()
QString ActiveConnection::id | ( | ) | const |
The Id of the connection.
Definition at line 179 of file activeconnection.cpp.
◆ idChanged
|
signal |
The id
changed.
◆ ipV4Config()
NetworkManager::IpConfig ActiveConnection::ipV4Config | ( | ) | const |
The Ip4Config object describing the configuration of the connection.
Only valid when the connection is in the NM_ACTIVE_CONNECTION_STATE_ACTIVATED state
Definition at line 161 of file activeconnection.cpp.
◆ ipV4ConfigChanged
|
signal |
Emitted when the IPv4 configuration of this network has changed.
◆ ipV6Config()
NetworkManager::IpConfig ActiveConnection::ipV6Config | ( | ) | const |
The Ip6Config object describing the configuration of the connection.
Only valid when the connection is in the NM_ACTIVE_CONNECTION_STATE_ACTIVATED state
Definition at line 170 of file activeconnection.cpp.
◆ ipV6ConfigChanged
|
signal |
Emitted when the IPv6 configuration of this network has changed.
◆ isValid()
bool ActiveConnection::isValid | ( | ) | const |
Returns true is this object holds a valid connection.
Definition at line 113 of file activeconnection.cpp.
◆ master()
QString ActiveConnection::master | ( | ) | const |
Returns the uni of master device if the connection is a slave.
Definition at line 191 of file activeconnection.cpp.
◆ masterChanged
|
signal |
The master device changed.
◆ path()
QString ActiveConnection::path | ( | ) | const |
Return path of the connection object.
Definition at line 125 of file activeconnection.cpp.
◆ specificObject()
QString ActiveConnection::specificObject | ( | ) | const |
The path of the specific object associated with the connection.
Definition at line 197 of file activeconnection.cpp.
◆ specificObjectChanged
|
signal |
The path
to the specific object changed.
◆ state()
NetworkManager::ActiveConnection::State ActiveConnection::state | ( | ) | const |
The current state of the connection.
Definition at line 203 of file activeconnection.cpp.
◆ stateChanged
|
signal |
The state
changed.
◆ stateChangedReason
|
signal |
The state
changed because of reason reason
(never emitted in runtime NM < 1.8.0)
◆ type()
NetworkManager::ConnectionSettings::ConnectionType ActiveConnection::type | ( | ) | const |
The type of the connection.
Definition at line 185 of file activeconnection.cpp.
◆ typeChanged
|
signal |
The type
changed.
◆ uuid()
QString ActiveConnection::uuid | ( | ) | const |
The UUID of the connection.
Definition at line 215 of file activeconnection.cpp.
◆ uuidChanged
|
signal |
The uuid
changed.
◆ vpn()
bool ActiveConnection::vpn | ( | ) | const |
Whether this is a VPN connection.
Definition at line 209 of file activeconnection.cpp.
◆ vpnChanged
|
signal |
The VPN property changed.
Member Data Documentation
◆ d_ptr
|
protected |
Definition at line 225 of file activeconnection.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 3 2025 11:54:18 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.