NetworkManager::Connection
#include <connection.h>
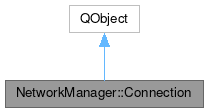
Public Types | |
typedef QList< Ptr > | List |
typedef QSharedPointer< Connection > | Ptr |
Signals | |
void | removed (const QString &path) |
void | unsavedChanged (bool unsaved) |
void | updated () |
Public Member Functions | |
Connection (const QString &path, QObject *parent=nullptr) | |
QDBusPendingReply | clearSecrets () |
bool | isUnsaved () const |
bool | isValid () const |
QString | name () const |
QString | path () const |
QDBusPendingReply | remove () |
QDBusPendingReply | save () |
QDBusPendingReply< NMVariantMapMap > | secrets (const QString &setting) |
ConnectionSettings::Ptr | settings () |
QDBusPendingReply | update (const NMVariantMapMap &settings) |
QDBusPendingReply | updateUnsaved (const NMVariantMapMap &settings) |
QString | uuid () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
This class represents a single network connection configuration.
Definition at line 27 of file connection.h.
Member Typedef Documentation
◆ List
typedef QList<Ptr> NetworkManager::Connection::List |
Definition at line 32 of file connection.h.
◆ Ptr
Definition at line 31 of file connection.h.
Constructor & Destructor Documentation
◆ Connection()
Constructs a connection object for the given path.
Definition at line 33 of file connection.cpp.
◆ ~Connection()
|
override |
Definition at line 61 of file connection.cpp.
Member Function Documentation
◆ clearSecrets()
QDBusPendingReply Connection::clearSecrets | ( | ) |
Clear the secrets belonging to this network connection profile.
- Since
- 5.8.0
Definition at line 124 of file connection.cpp.
◆ isUnsaved()
bool Connection::isUnsaved | ( | ) | const |
If set, indicates that the in-memory state of the connection does not match the on-disk state.
This flag will be set when updateUnsaved() is called or when any connection details change, and cleared when the connection is saved to disk via save() or from internal operations.
- Since
- 0.9.9.0
Definition at line 84 of file connection.cpp.
◆ isValid()
bool Connection::isValid | ( | ) | const |
Returns if this connection is valid.
Definition at line 66 of file connection.cpp.
◆ name()
QString Connection::name | ( | ) | const |
Returns the name of this connection.
Definition at line 78 of file connection.cpp.
◆ path()
QString Connection::path | ( | ) | const |
Returns the path (DBus) of this connection.
Definition at line 136 of file connection.cpp.
◆ remove()
QDBusPendingReply Connection::remove | ( | ) |
Removes the connection from NetworkManager database, this operation does not ask for confirmation but a policykit rule might prevent it from being removed without the proper password.
Definition at line 130 of file connection.cpp.
◆ removed
|
signal |
Emitted when the connection was removed.
- Parameters
-
path connections's path.
◆ save()
QDBusPendingReply Connection::save | ( | ) |
Saves a "dirty" connection (that had previously been updated with updateUnsaved()) to persistent storage.
- Since
- 0.9.9.0
Definition at line 118 of file connection.cpp.
◆ secrets()
QDBusPendingReply< NMVariantMapMap > Connection::secrets | ( | const QString & | setting | ) |
Retrieves this connections's secrets (passwords and / or encryption keys).
- Parameters
-
setting the setting identifier.
Definition at line 100 of file connection.cpp.
◆ settings()
NetworkManager::ConnectionSettings::Ptr Connection::settings | ( | ) |
Returns the settings of this connection.
Definition at line 90 of file connection.cpp.
◆ unsavedChanged
|
signal |
Emitted when the connection unsaved state changes.
◆ update()
QDBusPendingReply Connection::update | ( | const NMVariantMapMap & | settings | ) |
Update the connection with new settings
and properties, replacing all previous settings and properties.
Secrets may be part of the update request, and will be either stored in persistent storage or given to a Secret Agent for storage, depending on the request.
Definition at line 106 of file connection.cpp.
◆ updated
|
signal |
Emitted when the connection settings changes.
◆ updateUnsaved()
QDBusPendingReply Connection::updateUnsaved | ( | const NMVariantMapMap & | settings | ) |
Update the connection with new settings
and properties (replacing all previous settings and properties) but do not immediately save the connection to disk.
Secrets may be part of the update request and may sent to a Secret Agent for storage, depending on the flags associated with each secret.
Use the save() method to save these changes to disk. Note that unsaved changes will be lost if the connection is reloaded from disk (either automatically on file change or due to an explicit reloadConnections() call).
- Since
- 0.9.9.0
Definition at line 112 of file connection.cpp.
◆ uuid()
QString Connection::uuid | ( | ) | const |
Returns the unique identifier of this connection.
Definition at line 72 of file connection.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:09:59 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.