NetworkManager::SecretAgent
#include <secretagent.h>
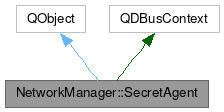
Public Types | |
typedef QFlags< Capability > | Capabilities |
enum | Capability { NoCapability = 0 , VpnHints = 0x01 } |
enum | Error { NotAuthorized , InvalidConnection , UserCanceled , AgentCanceled , InternalError , NoSecrets } |
enum | GetSecretsFlag { None = 0 , AllowInteraction = 0x01 , RequestNew = 0x02 , UserRequested = 0x04 } |
typedef QFlags< GetSecretsFlag > | GetSecretsFlags |
Public Slots | |
virtual void | CancelGetSecrets (const QDBusObjectPath &connection_path, const QString &setting_name)=0 |
virtual void | DeleteSecrets (const NMVariantMapMap &connection, const QDBusObjectPath &connection_path)=0 |
virtual NMVariantMapMap | GetSecrets (const NMVariantMapMap &connection, const QDBusObjectPath &connection_path, const QString &setting_name, const QStringList &hints, uint flags)=0 |
virtual void | SaveSecrets (const NMVariantMapMap &connection, const QDBusObjectPath &connection_path)=0 |
Public Member Functions | |
SecretAgent (const QString &id, NetworkManager::SecretAgent::Capabilities capabilities, QObject *parent=nullptr) | |
SecretAgent (const QString &id, QObject *parent=nullptr) | |
void | sendError (Error error, const QString &explanation, const QDBusMessage &callMessage=QDBusMessage()) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
bool | calledFromDBus () const const |
QDBusConnection | connection () const const |
bool | isDelayedReply () const const |
void | sendErrorReply (const QString &name, const QString &msg) const const |
void | sendErrorReply (QDBusError::ErrorType type, const QString &msg) const const |
void | setDelayedReply (bool enable) const const |
Detailed Description
Implementation of a private D-Bus interface used by secret agents that store and provide secrets to NetworkManager.
If an agent provides secrets to NetworkManager as part of connection creation, and the some of those secrets are "agent owned" the agent should store those secrets itself and should not expect its SaveSecrets() method to be called. SaveSecrets() will be called eg if some program other than the agent itself (like a connection editor) changes the secrets out of band.
Definition at line 29 of file secretagent.h.
Member Typedef Documentation
◆ Capabilities
Definition at line 64 of file secretagent.h.
◆ GetSecretsFlags
Definition at line 55 of file secretagent.h.
Member Enumeration Documentation
◆ Capability
Capabilities to pass to secret agents.
Enumerator | |
---|---|
NoCapability | No capability. |
VpnHints | Pass hints to secret agent. |
Definition at line 60 of file secretagent.h.
◆ Error
enum NetworkManager::SecretAgent::Error |
Definition at line 33 of file secretagent.h.
◆ GetSecretsFlag
Flags modifying the behavior of GetSecrets request.
Enumerator | |
---|---|
None | No special behavior; by default no user interaction is allowed and requests for secrets are fulfilled from persistent storage, or if no secrets are available an error is returned. |
AllowInteraction | Allows the request to interact with the user, possibly prompting via UI for secrets if any are required, or if none are found in persistent storage. |
RequestNew | Explicitly prompt for new secrets from the user. This flag signals that NetworkManager thinks any existing secrets are invalid or wrong. This flag implies that interaction is allowed. |
UserRequested | Set if the request was initiated by user-requested action via the D-Bus interface, as opposed to automatically initiated by NetworkManager in response to (for example) scan results or carrier changes. |
Definition at line 45 of file secretagent.h.
Constructor & Destructor Documentation
◆ SecretAgent() [1/2]
|
explicit |
Registers a SecretAgent with the id
on NetworkManager Optionally add a capabilities argument.
Definition at line 90 of file secretagent.cpp.
◆ SecretAgent() [2/2]
|
explicit |
Definition at line 97 of file secretagent.cpp.
◆ ~SecretAgent()
|
override |
Definition at line 104 of file secretagent.cpp.
Member Function Documentation
◆ CancelGetSecrets
|
pure virtualslot |
Called when the subclass should cancel an outstanding request to get secrets for a given connection.
Cancelling the request MUST sendError() with the original DBus message using AgentCanceled param as the error type.
- Parameters
-
connection_path Object path of the connection for which, if secrets for the given 'setting_name' are being requested, the request should be canceled. setting_name Setting name for which secrets for this connection were originally being requested.
◆ DeleteSecrets
|
pure virtualslot |
Called when the subclass should delete the secrets contained in the connection from backing storage.
- Parameters
-
connection Nested settings maps containing the connection properties (sans secrets), for which the agent should delete the secrets from backing storage. connection_path Object path of the connection for which the agent should delete secrets from backing storage.
◆ GetSecrets
|
pure virtualslot |
Called when the subclass should retrieve and return secrets.
If the request is canceled, called function should call sendError(), in this case the return value is ignored.
- Parameters
-
connection Nested settings maps containing the connection for which secrets are being requested. This may contain system-owned secrets if the agent has successfully authenticated to modify system network settings and the GetSecrets request flags allow user interaction. connection_path Object path of the connection for which secrets are being requested. setting_name Setting name for which secrets are being requested. hints Array of strings of key names in the requested setting for which NetworkManager thinks a secrets may be required, and/or well-known identifiers and data that may be useful to the client in processing the secrets request. Note that it's not always possible to determine which secret is required, so in some cases no hints may be given. The Agent should return any secrets it has, or that it thinks are required, regardless of what hints NetworkManager sends in this request. flags Flags which modify the behavior of the secrets request (see GetSecretsFlag)
◆ SaveSecrets
|
pure virtualslot |
Called when the subclass should save the secrets contained in the connection to backing storage.
- Parameters
-
connection Nested settings maps containing the connection for which secrets are being saved. This may contain system-owned secrets if the agent has successfully authenticated to modify system network settings and the GetSecrets request flags allow user interaction. connection_path Object path of the connection for which the agent should save secrets to backing storage.
◆ sendError()
void NetworkManager::SecretAgent::sendError | ( | Error | error, |
const QString & | explanation, | ||
const QDBusMessage & | callMessage = QDBusMessage() ) const |
Send to NetworkManager the error
the subclass has found, the explanation
is useful for debugging purposes, and the callMessage
is ONLY needed if setDelayedReply() was set to true
when the method was called.
Definition at line 110 of file secretagent.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:52:50 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.