Syndication::ElementWrapper
#include <elementwrapper.h>
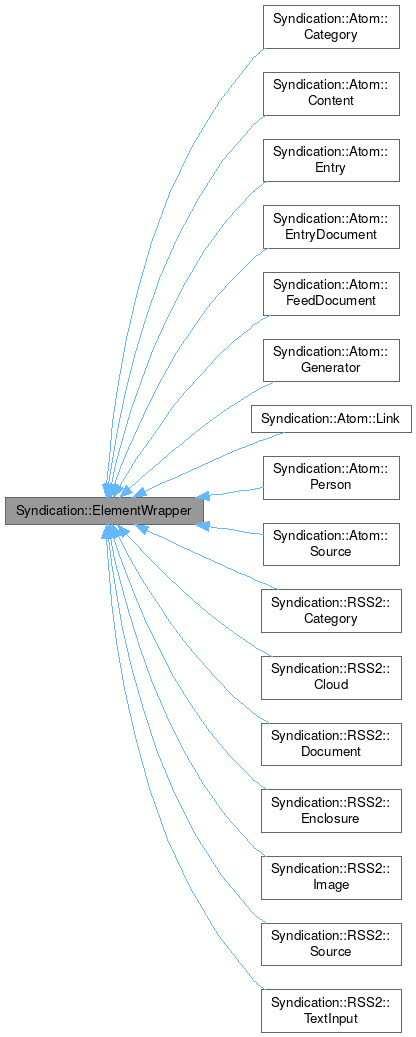
Static Public Member Functions | |
static QString | childNodesAsXML (const QDomElement &parent) |
Detailed Description
A wrapper for XML elements.
This is the base class for the (lazy) wrappers used in the RSS2 and Atom parsers. The wrapped element can be accessed via element(). It also contains several helper functions for XML processing.
Definition at line 29 of file elementwrapper.h.
Constructor & Destructor Documentation
◆ ElementWrapper() [1/3]
Syndication::ElementWrapper::ElementWrapper | ( | ) |
creates a element wrapper wrapping a null element.
isNull() will return true
for these instances.
Definition at line 32 of file elementwrapper.cpp.
◆ ElementWrapper() [2/3]
Syndication::ElementWrapper::ElementWrapper | ( | const ElementWrapper & | other | ) |
Copy constructor.The instances share the same element.
- Parameters
-
other the element wrapper to copy
Definition at line 39 of file elementwrapper.cpp.
◆ ElementWrapper() [3/3]
Syndication::ElementWrapper::ElementWrapper | ( | const QDomElement & | element | ) |
Creates an element wrapper wrapping the DOM element element
.
- Parameters
-
element the element to wrap
Definition at line 44 of file elementwrapper.cpp.
◆ ~ElementWrapper()
|
virtual |
destructor
Definition at line 53 of file elementwrapper.cpp.
Member Function Documentation
◆ attribute()
QString Syndication::ElementWrapper::attribute | ( | const QString & | name, |
const QString & | defValue = QString() ) const |
Returns the attribute called name.
If the attribute does not exist defValue is returned. (which is a null string by default).
- Parameters
-
name tag name defValue the default value
Definition at line 259 of file elementwrapper.cpp.
◆ attributeNS()
QString Syndication::ElementWrapper::attributeNS | ( | const QString & | nsURI, |
const QString & | localName, | ||
const QString & | defValue = QString() ) const |
Returns the attribute with the local name
localName and the namespace URI nsURI
.
If the attribute does not exist defValue
is returned (which is a null string by default).
- Parameters
-
nsURI namespace URI localName local tag name defValue the default value
Definition at line 264 of file elementwrapper.cpp.
◆ childNodesAsXML() [1/2]
QString Syndication::ElementWrapper::childNodesAsXML | ( | ) | const |
returns the child nodes of the wrapped element as XML.
See childNodesAsXML(const QDomElement& parent) for details
- Returns
- XML serialization of the wrapped element's children
Definition at line 199 of file elementwrapper.cpp.
◆ childNodesAsXML() [2/2]
|
static |
concatenates the XML representations of all children.
Example: If parent
is an xhtml:body
element like
this function returns
namespace and xml:base information are preserved.
- Parameters
-
parent the DOM element whose children should be returned as XML
- Returns
- XML serialization of parent's children
Definition at line 168 of file elementwrapper.cpp.
◆ completeURI()
completes relative URIs with a prefix specified via xml:base.
Example:
is completed to http://www.foo.org/announcements/bar.html
See also xmlBase().
- Parameters
-
uri a possibly relative URI
- Returns
- the resolved, absolute URI (using xml:base), if
uri
is a relative, valid URI. Ifuri
is not valid, absolute, or no xml:base is set in the scope of this element,uri
is returned unmodified.
Definition at line 121 of file elementwrapper.cpp.
◆ element()
const QDomElement & Syndication::ElementWrapper::element | ( | ) | const |
returns the wrapped resource.
Definition at line 73 of file elementwrapper.cpp.
◆ elementsByTagName()
QList< QDomElement > Syndication::ElementWrapper::elementsByTagName | ( | const QString & | tagName | ) | const |
returns all child elements with tag name tagName
Contrary to QDomElement::elementsByTagName() only direct descendents are returned.
- Parameters
-
tagName the tag name of the elements to extract
- Returns
- a list of child elements with the given tag name
Definition at line 204 of file elementwrapper.cpp.
◆ elementsByTagNameNS()
QList< QDomElement > Syndication::ElementWrapper::elementsByTagNameNS | ( | const QString & | nsURI, |
const QString & | tagName ) const |
returns all child elements with tag name tagname
and namespace URI nsURI
.
Contrary to QDomElement::elementsByTagNameNS() only direct descendents are returned
- Parameters
-
nsURI the namespace URI tagName the local name (local within its namespace) of the element to search for
- Returns
- a list of child elements with the given namespace URI and tag name
Definition at line 236 of file elementwrapper.cpp.
◆ extractElementText()
extracts the text from a child element, ignoring namespaces.
For instance, when the wrapped element is <thisElement>
:
extractElementText
("title") will return the text content of title
, "Hi there".
- Parameters
-
tagName the name of the element to extract
- Returns
- the (trimmed) text content of
tagName
, or a null string if there is no such tag
Definition at line 156 of file elementwrapper.cpp.
◆ extractElementTextNS()
QString Syndication::ElementWrapper::extractElementTextNS | ( | const QString & | namespaceURI, |
const QString & | localName ) const |
extracts the text from a child element, respecting namespaces.
If there is more than one child with the same tag name, the first one is processed. For instance, when the wrapped element is <hisElement>
:
will return the text content of atom:title
, "Hi there". (Assuming that "atom" is defined as "http://www.w3.org/2005/Atom")
- Parameters
-
namespaceURI the namespace URI of the element to extract localName the local name (local within its namespace) of the element to extract
- Returns
- the (trimmed) text content of
localName
, or a null string if there is no such tag
Definition at line 162 of file elementwrapper.cpp.
◆ firstElementByTagNameNS()
QDomElement Syndication::ElementWrapper::firstElementByTagNameNS | ( | const QString & | nsURI, |
const QString & | tagName ) const |
searches the direct children of the wrapped element for an element with a given namespace and tag name.
- Parameters
-
nsURI the namespace URI tagName the local name (local within its namespace) of the element to search for
- Returns
- the first child element with the given namespace URI and tag name, or a null element if no such element was found.
Definition at line 218 of file elementwrapper.cpp.
◆ hasAttribute()
bool Syndication::ElementWrapper::hasAttribute | ( | const QString & | name | ) | const |
Returns true if this element has an attribute called name
; otherwise returns false
.
- Parameters
-
name the attribute name (without namespace)
Definition at line 269 of file elementwrapper.cpp.
◆ hasAttributeNS()
bool Syndication::ElementWrapper::hasAttributeNS | ( | const QString & | nsURI, |
const QString & | localName ) const |
Returns true if this element has an attribute with the local name localName and the namespace URI nsURI; otherwise returns false.
- Parameters
-
nsURI namespace URI localName local attribute name
Definition at line 274 of file elementwrapper.cpp.
◆ isNull()
bool Syndication::ElementWrapper::isNull | ( | ) | const |
returns whether the wrapped element is a null element
- Returns
true
if isNull() is true for the wrapped element,false
otherwise
Definition at line 68 of file elementwrapper.cpp.
◆ operator=()
ElementWrapper & Syndication::ElementWrapper::operator= | ( | const ElementWrapper & | other | ) |
Assigns another element wrapper to this one.
Both instances share the same wrapped element instance.
- Parameters
-
other the element wrapper to assign
- Returns
- reference to this instance
Definition at line 57 of file elementwrapper.cpp.
◆ operator==()
bool Syndication::ElementWrapper::operator== | ( | const ElementWrapper & | other | ) | const |
compares two wrappers.
Two wrappers are equal if and only if the wrapped elements are equal.
- Parameters
-
other another element wrapper to compare to
Definition at line 63 of file elementwrapper.cpp.
◆ text()
QString Syndication::ElementWrapper::text | ( | ) | const |
Returns the wrapped element's text or an empty string.
For more information, see QDomElement::text();
Definition at line 254 of file elementwrapper.cpp.
◆ xmlBase()
QString Syndication::ElementWrapper::xmlBase | ( | ) | const |
returns the xml:base value to be used for the wrapped element.
The xml:base attribute establishes the base URI for resolving any relative references found in its scope (its own element and all descendants). (See also completeURI())
- Returns
- the xml:base value, or a null string if not set
Definition at line 78 of file elementwrapper.cpp.
◆ xmlLang()
QString Syndication::ElementWrapper::xmlLang | ( | ) | const |
returns the xml:lang value to be used for the wrapped element.
The xml:lang attribute indicates the natural language for its element and all descendants.
- Returns
- the xml:lang value, or a null string if not set
Definition at line 132 of file elementwrapper.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:54:11 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.