Akonadi::Monitor
#include <monitor.h>
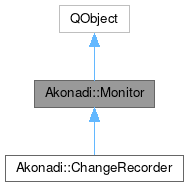
Public Types | |
enum | Type { Collections = 1 , Items , Tags , Subscribers , Notifications } |
Signals | |
void | allMonitored (bool monitored) |
void | collectionAdded (const Akonadi::Collection &collection, const Akonadi::Collection &parent) |
void | collectionChanged (const Akonadi::Collection &collection) |
void | collectionChanged (const Akonadi::Collection &collection, const QSet< QByteArray > &attributeNames) |
void | collectionMonitored (const Akonadi::Collection &collection, bool monitored) |
void | collectionMoved (const Akonadi::Collection &collection, const Akonadi::Collection &source, const Akonadi::Collection &destination) |
void | collectionRemoved (const Akonadi::Collection &collection) |
void | collectionStatisticsChanged (Akonadi::Collection::Id id, const Akonadi::CollectionStatistics &statistics) |
void | collectionSubscribed (const Akonadi::Collection &collection, const Akonadi::Collection &parent) |
void | collectionUnsubscribed (const Akonadi::Collection &collection) |
void | debugNotification (const Akonadi::ChangeNotification ¬ification) |
void | itemAdded (const Akonadi::Item &item, const Akonadi::Collection &collection) |
void | itemChanged (const Akonadi::Item &item, const QSet< QByteArray > &partIdentifiers) |
void | itemLinked (const Akonadi::Item &item, const Akonadi::Collection &collection) |
void | itemMonitored (const Akonadi::Item &item, bool monitored) |
void | itemMoved (const Akonadi::Item &item, const Akonadi::Collection &collectionSource, const Akonadi::Collection &collectionDestination) |
void | itemRemoved (const Akonadi::Item &item) |
void | itemsFlagsChanged (const Akonadi::Item::List &items, const QSet< QByteArray > &addedFlags, const QSet< QByteArray > &removedFlags) |
void | itemsLinked (const Akonadi::Item::List &items, const Akonadi::Collection &collection) |
void | itemsMoved (const Akonadi::Item::List &items, const Akonadi::Collection &collectionSource, const Akonadi::Collection &collectionDestination) |
void | itemsRemoved (const Akonadi::Item::List &items) |
void | itemsTagsChanged (const Akonadi::Item::List &items, const QSet< Akonadi::Tag > &addedTags, const QSet< Akonadi::Tag > &removedTags) |
void | itemsUnlinked (const Akonadi::Item::List &items, const Akonadi::Collection &collection) |
void | itemUnlinked (const Akonadi::Item &item, const Akonadi::Collection &collection) |
void | mimeTypeMonitored (const QString &mimeType, bool monitored) |
void | monitorReady () |
void | notificationSubscriberAdded (const Akonadi::NotificationSubscriber &subscriber) |
void | notificationSubscriberChanged (const Akonadi::NotificationSubscriber &subscriber) |
void | notificationSubscriberRemoved (const Akonadi::NotificationSubscriber &subscriber) |
void | resourceMonitored (const QByteArray &identifier, bool monitored) |
void | tagAdded (const Akonadi::Tag &tag) |
void | tagChanged (const Akonadi::Tag &tag) |
void | tagMonitored (const Akonadi::Tag &tag, bool monitored) |
void | tagRemoved (const Akonadi::Tag &tag) |
void | typeMonitored (const Akonadi::Monitor::Type type, bool monitored) |
Public Member Functions | |
Monitor (QObject *parent=nullptr) | |
~Monitor () override | |
CollectionFetchScope & | collectionFetchScope () |
Collection::List | collectionsMonitored () const |
bool | exclusive () const |
void | fetchChangedOnly (bool enable) |
void | fetchCollection (bool enable) |
void | fetchCollectionStatistics (bool enable) |
void | ignoreSession (Session *session) |
bool | isAllMonitored () const |
ItemFetchScope & | itemFetchScope () |
QList< Item::Id > | itemsMonitoredEx () const |
QStringList | mimeTypesMonitored () const |
int | numItemsMonitored () const |
int | numMimeTypesMonitored () const |
int | numResourcesMonitored () const |
QList< QByteArray > | resourcesMonitored () const |
Session * | session () const |
void | setAllMonitored (bool monitored=true) |
void | setCollectionFetchScope (const CollectionFetchScope &fetchScope) |
void | setCollectionMonitored (const Collection &collection, bool monitored=true) |
void | setCollectionMoveTranslationEnabled (bool enabled) |
void | setExclusive (bool exclusive=true) |
void | setItemFetchScope (const ItemFetchScope &fetchScope) |
void | setItemMonitored (const Item &item, bool monitored=true) |
void | setMimeTypeMonitored (const QString &mimetype, bool monitored=true) |
void | setResourceMonitored (const QByteArray &resource, bool monitored=true) |
void | setSession (Akonadi::Session *session) |
void | setTagFetchScope (const TagFetchScope &fetchScope) |
void | setTagMonitored (const Tag &tag, bool monitored=true) |
void | setTypeMonitored (Type type, bool monitored=true) |
TagFetchScope & | tagFetchScope () |
QList< Tag::Id > | tagsMonitored () const |
QList< Type > | typesMonitored () const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
Monitors an item or collection for changes.
The Monitor emits signals if some of these objects are changed or removed or new ones are added to the Akonadi storage.
There are various ways to filter these notifications. There are three types of filter evaluation:
- (-) removal-only filter, ie. if the filter matches the notification is dropped, if not filter evaluation continues with the next one
- (+) pass-exit filter, ie. if the filter matches the notification is delivered, if not evaluation is continued
- (f) final filter, ie. evaluation ends here if the corresponding filter criteria is set, the notification is delivered depending on the result, evaluation is only continued if no filter criteria is defined
The following filter are available, listed in evaluation order: (1) ignored sessions (-) (2) monitor everything (+) (3a) resource and mimetype filters (f) (items only) (3b) resource filters (f) (collections only) (4) item is monitored (+) (5) collection is monitored (+)
Optionally, the changed objects can be fetched automatically from the server. To enable this, see itemFetchScope() and collectionFetchScope().
Note that as a consequence of rule 3a, it is not possible to monitor (more than zero resources OR more than zero mimetypes) AND more than zero collections.
- Todo
Distinguish between monitoring collection properties and collection content.
Special case for collection content counts changed
Member Enumeration Documentation
◆ Type
Enumerator | |
---|---|
Collections | This must be kept in sync with Akonadi::NotificationMessageV2::Type |
Subscribers | Listen to subscription changes of other Monitors connected to Akonadi. This is only for debugging purposes and should not be used in real applications.
|
Notifications | Listens to all notifications being emitted by the server and provides additional information about them. This is only for debugging purposes and should not be used in real applications.
|
Constructor & Destructor Documentation
◆ Monitor()
|
explicit |
Creates a new monitor.
- Parameters
-
parent The parent object.
Definition at line 21 of file monitor.cpp.
◆ ~Monitor()
|
override |
Destroys the monitor.
Definition at line 43 of file monitor.cpp.
Member Function Documentation
◆ allMonitored
|
signal |
This signal is emitted if the Monitor starts or stops monitoring everything.
- Parameters
-
monitored Whether everything is now being monitored or not.
- Since
- 4.3
◆ collectionAdded
|
signal |
This signal is emitted if a new collection has been added to a monitored collection in the Akonadi storage.
- Parameters
-
collection The new collection. parent The parent collection.
◆ collectionChanged [1/2]
|
signal |
This signal is emitted if a monitored collection has been changed (properties or content).
- Parameters
-
collection The changed collection.
◆ collectionChanged [2/2]
|
signal |
This signal is emitted if a monitored collection has been changed (properties or attributes).
- Parameters
-
collection The changed collection. attributeNames The names of the collection attributes that have been changed.
- Since
- 4.4
◆ collectionFetchScope()
CollectionFetchScope & Monitor::collectionFetchScope | ( | ) |
Returns the collection fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable. See the CollectionFetchScope documentation for an example.
- Returns
- a reference to the current collection fetch scope
- See also
- setCollectionFetchScope() for replacing the current collection fetch scope
- Since
- 4.4
Definition at line 235 of file monitor.cpp.
◆ collectionMonitored
|
signal |
This signal is emitted if the Monitor starts or stops monitoring collection
explicitly.
- Parameters
-
collection The collection monitored Whether the collection is now being monitored or not.
- Since
- 4.3
◆ collectionMoved
|
signal |
This signals is emitted if a monitored collection has been moved.
- Parameters
-
collection The moved collection. source The previous parent collection. destination The new parent collection.
- Since
- 4.4
◆ collectionRemoved
|
signal |
This signal is emitted if a monitored collection has been removed from the Akonadi storage.
- Parameters
-
collection The removed collection.
◆ collectionsMonitored()
|
nodiscard |
Returns the list of collections being monitored.
- Since
- 4.3
Definition at line 259 of file monitor.cpp.
◆ collectionStatisticsChanged
|
signal |
This signal is emitted if the statistics information of a monitored collection has changed.
- Parameters
-
id The collection identifier of the changed collection. statistics The updated collection statistics, invalid if automatic fetching of statistics changes is disabled.
◆ collectionSubscribed
|
signal |
This signal is emitted if a collection has been subscribed to by the user.
It will be emitted even for unmonitored collections as the check for whether to monitor it has not been applied yet.
- Parameters
-
collection The subscribed collection parent The parent collection of the subscribed collection.
- Since
- 4.6
◆ collectionUnsubscribed
|
signal |
This signal is emitted if a user unsubscribes from a collection.
- Parameters
-
collection The unsubscribed collection
- Since
- 4.6
◆ debugNotification
|
signal |
This signal is emitted when Notifications are monitored and the server emits any change notification.
- Since
- 5.4
- Note
- Getting introspection into all change notifications only makes sense if you want to globally debug Notifications. There is no reason to use this in regular applications.
◆ exclusive()
|
nodiscard |
Definition at line 173 of file monitor.cpp.
◆ fetchChangedOnly()
void Monitor::fetchChangedOnly | ( | bool | enable | ) |
Instructs the monitor to fetch only those parts that were changed and were requested in the fetch scope.
This is taken in account only for item modifications. Example usage:
In the example if an item was changed, but its payload was not, the full payload will not be retrieved. If the item's payload was changed, the monitor retrieves the changed payload as well.
The default is to fetch everything requested.
- Since
- 4.8
- Parameters
-
enable true
to enable the feature,false
means everything that was requested will be fetched.
Definition at line 221 of file monitor.cpp.
◆ fetchCollection()
void Monitor::fetchCollection | ( | bool | enable | ) |
Enables automatic fetching of changed collections from the Akonadi storage.
- Parameters
-
enable true
enables automatic fetching,false
disables automatic fetching.
Definition at line 193 of file monitor.cpp.
◆ fetchCollectionStatistics()
void Monitor::fetchCollectionStatistics | ( | bool | enable | ) |
Enables automatic fetching of changed collection statistics information from the Akonadi storage.
- Parameters
-
enable true
to enables automatic fetching,false
disables automatic fetching.
Definition at line 199 of file monitor.cpp.
◆ ignoreSession()
void Monitor::ignoreSession | ( | Session * | session | ) |
Ignores all change notifications caused by the given session.
This overrides all other settings on this session.
- Parameters
-
session The session you want to ignore.
Definition at line 179 of file monitor.cpp.
◆ isAllMonitored()
|
nodiscard |
◆ itemAdded
|
signal |
This signal is emitted if an item has been added to a monitored collection in the Akonadi storage.
- Parameters
-
item The new item. collection The collection the item has been added to.
◆ itemChanged
|
signal |
This signal is emitted if a monitored item has changed, e.g.
item parts have been modified.
- Parameters
-
item The changed item. partIdentifiers The identifiers of the item parts that has been changed.
◆ itemFetchScope()
ItemFetchScope & Monitor::itemFetchScope | ( | ) |
Returns the item fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable. See the ItemFetchScope documentation for an example.
- Returns
- a reference to the current item fetch scope
- See also
- setItemFetchScope() for replacing the current item fetch scope
Definition at line 213 of file monitor.cpp.
◆ itemLinked
|
signal |
This signal is emitted if a reference to an item is added to a virtual collection.
- Parameters
-
item The linked item. collection The collection the item is linked to.
- Since
- 4.2
◆ itemMonitored
|
signal |
This signal is emitted if the Monitor starts or stops monitoring item
explicitly.
- Parameters
-
item The item monitored Whether the item is now being monitored or not.
- Since
- 4.3
◆ itemMoved
|
signal |
This signal is emitted if a monitored item has been moved between two collections.
- Parameters
-
item The moved item. collectionSource The collection the item has been moved from. collectionDestination The collection the item has been moved to.
◆ itemRemoved
|
signal |
This signal is emitted if.
- a monitored item has been removed from the Akonadi storage or
- a item has been removed from a monitored collection.
- Parameters
-
item The removed item.
◆ itemsFlagsChanged
|
signal |
This signal is emitted if flags of monitored items have changed.
- Parameters
-
items Items that were changed addedFlags Flags that have been added to each item in items
removedFlags Flags that have been removed from each item in items
- Since
- 4.11
◆ itemsLinked
|
signal |
This signal is emitted if a reference to multiple items is added to a virtual collection.
- Parameters
-
items The linked items collection The collections the items are linked to
- Since
- 4.11
◆ itemsMonitoredEx()
Returns the set of items being monitored.
Faster version (at least on 32-bit systems) of itemsMonitored().
- Since
- 4.6
Definition at line 265 of file monitor.cpp.
◆ itemsMoved
|
signal |
This is signal is emitted when multiple monitored items have been moved between two collections.
- Parameters
-
items Moved items collectionSource The collection the items have been moved from. collectionDestination The collection the items have been moved to.
- Since
- 4.11
◆ itemsRemoved
|
signal |
This signal is emitted if monitored items have been removed from Akonadi storage of items have been removed from a monitored collection.
- Parameters
-
items Removed items
- Since
- 4.11
◆ itemsTagsChanged
|
signal |
This signal is emitted if tags of monitored items have changed.
- Parameters
-
items Items that were changed addedTags Tags that have been added to each item in items
.removedTags Tags that have been removed from each item in items
- Since
- 4.13
◆ itemsUnlinked
|
signal |
This signal is emitted if a reference to items is removed from a virtual collection.
- Parameters
-
items The unlinked items collection The collections the items are unlinked from
- Since
- 4.11
◆ itemUnlinked
|
signal |
This signal is emitted if a reference to an item is removed from a virtual collection.
- Parameters
-
item The unlinked item. collection The collection the item is unlinked from.
- Since
- 4.2
◆ mimeTypeMonitored
|
signal |
This signal is emitted if the Monitor starts or stops monitoring mimeType
explicitly.
- Parameters
-
mimeType The mimeType. monitored Whether the mimeType is now being monitored or not.
- Since
- 4.3
◆ mimeTypesMonitored()
|
nodiscard |
◆ notificationSubscriberAdded
|
signal |
This signal is emitted when Subscribers are monitored and a new subscriber subscribers to the server.
- Parameters
-
subscriber The new subscriber
- Since
- 5.4
- Note
- Monitoring for subscribers and listening to this signal only makes sense if you want to globally debug Monitors. There is no reason to use this in regular applications.
◆ notificationSubscriberChanged
|
signal |
This signal is emitted when Subscribers are monitored and an existing subscriber changes its subscription.
- Parameters
-
subscriber The changed subscriber
- Since
- 5.4
- Note
- Monitoring for subscribers and listening to this signal only makes sense if you want to globally debug Monitors. There is no reason to use this in regular applications.
◆ notificationSubscriberRemoved
|
signal |
This signal is emitted when Subscribers are monitored and an existing subscriber unsubscribes from the server.
- Parameters
-
subscriber The removed subscriber
- Since
- 5.4
- Note
- Monitoring for subscribers and listening to this signal only makes sense if you want to globally debug Monitors. There is no reason to use this in regular applications.
◆ numItemsMonitored()
|
nodiscard |
Returns the number of items being monitored.
Optimization.
- Since
- 4.14.3
Definition at line 274 of file monitor.cpp.
◆ numMimeTypesMonitored()
|
nodiscard |
Returns the number of mimetypes being monitored.
Optimization.
- Since
- 4.14.3
Definition at line 304 of file monitor.cpp.
◆ numResourcesMonitored()
|
nodiscard |
Returns the number of resources being monitored.
Optimization.
- Since
- 4.14.3
Definition at line 316 of file monitor.cpp.
◆ resourceMonitored
|
signal |
This signal is emitted if the Monitor starts or stops monitoring the resource with the identifier identifier
explicitly.
- Parameters
-
identifier The identifier of the resource. monitored Whether the resource is now being monitored or not.
- Since
- 4.3
◆ resourcesMonitored()
|
nodiscard |
Returns the set of identifiers for resources being monitored.
- Since
- 4.3
Definition at line 310 of file monitor.cpp.
◆ session()
|
nodiscard |
Returns the Session used by the monitor to communicate with Akonadi.
- Since
- 4.4
Definition at line 349 of file monitor.cpp.
◆ setAllMonitored()
void Akonadi::Monitor::setAllMonitored | ( | bool | monitored = true | ) |
Sets whether all items shall be monitored.
- Parameters
-
monitored sets all items as monitored if set as true
Note that if a session is being ignored, this takes precedence over setAllMonitored() on that session.
Definition at line 150 of file monitor.cpp.
◆ setCollectionFetchScope()
void Monitor::setCollectionFetchScope | ( | const CollectionFetchScope & | fetchScope | ) |
Sets the collection fetch scope.
Controls which collections are monitored and how much of a collection's data is fetched from the server.
- Parameters
-
fetchScope The new scope for collection fetch operations.
- See also
- collectionFetchScope()
- Since
- 4.4
Definition at line 227 of file monitor.cpp.
◆ setCollectionMonitored()
void Monitor::setCollectionMonitored | ( | const Collection & | collection, |
bool | monitored = true ) |
Sets whether the specified collection shall be monitored for changes.
If monitoring is turned on for the collection, all notifications for items in that collection will be emitted, and its child collections will also be monitored. Note that move notifications will be emitted if either one of the collections involved is being monitored.
Note that if a session is being ignored, this takes precedence over setCollectionMonitored() on that session.
- Parameters
-
collection The collection to monitor. If this collection is Collection::root(), all collections in the Akonadi storage will be monitored. monitored Whether to monitor the collection.
Definition at line 48 of file monitor.cpp.
◆ setCollectionMoveTranslationEnabled()
void Monitor::setCollectionMoveTranslationEnabled | ( | bool | enabled | ) |
Allows to enable/disable collection move translation.
If enabled (the default), move notifications are automatically translated into add/remove notifications if the source/destination is outside of the monitored collection hierarchy.
- Parameters
-
enabled enables collection move translation if set as true
- Since
- 4.9
Definition at line 355 of file monitor.cpp.
◆ setExclusive()
void Monitor::setExclusive | ( | bool | exclusive = true | ) |
Definition at line 165 of file monitor.cpp.
◆ setItemFetchScope()
void Monitor::setItemFetchScope | ( | const ItemFetchScope & | fetchScope | ) |
Sets the item fetch scope.
Controls how much of an item's data is fetched from the server, e.g. whether to fetch the full item payload or only meta data.
- Parameters
-
fetchScope The new scope for item fetch operations.
- See also
- itemFetchScope()
Definition at line 205 of file monitor.cpp.
◆ setItemMonitored()
void Monitor::setItemMonitored | ( | const Item & | item, |
bool | monitored = true ) |
Sets whether the specified item shall be monitored for changes.
Note that if a session is being ignored, this takes precedence over setItemMonitored() on that session.
- Parameters
-
item The item to monitor. monitored Whether to monitor the item.
Definition at line 65 of file monitor.cpp.
◆ setMimeTypeMonitored()
void Monitor::setMimeTypeMonitored | ( | const QString & | mimetype, |
bool | monitored = true ) |
Sets whether items of the specified mime type shall be monitored for changes.
If monitoring is turned on for the mime type, all notifications for items matching that mime type will be emitted, but notifications for collections matching that mime type will only be emitted if this is otherwise specified, for example by setCollectionMonitored().
Note that if a session is being ignored, this takes precedence over setMimeTypeMonitored() on that session.
- Parameters
-
mimetype The mime type to monitor. monitored Whether to monitor the mime type.
Definition at line 99 of file monitor.cpp.
◆ setResourceMonitored()
void Monitor::setResourceMonitored | ( | const QByteArray & | resource, |
bool | monitored = true ) |
Sets whether the specified resource shall be monitored for changes.
If monitoring is turned on for the resource, all notifications for collections and items in that resource will be emitted.
Note that if a session is being ignored, this takes precedence over setResourceMonitored() on that session.
- Parameters
-
resource The resource identifier. monitored Whether to monitor the resource.
Definition at line 82 of file monitor.cpp.
◆ setSession()
void Monitor::setSession | ( | Akonadi::Session * | session | ) |
Sets the session used by the Monitor to communicate with the Akonadi server.
If not set, the Akonadi::Session::defaultSession is used.
- Parameters
-
session the session to be set
- Since
- 4.4
Definition at line 328 of file monitor.cpp.
◆ setTagFetchScope()
void Monitor::setTagFetchScope | ( | const TagFetchScope & | fetchScope | ) |
Sets the tag fetch scope.
Controls how much of an tag's data is fetched from the server.
- Parameters
-
fetchScope The new scope for tag fetch operations.
- See also
- tagFetchScope()
Definition at line 243 of file monitor.cpp.
◆ setTagMonitored()
void Monitor::setTagMonitored | ( | const Tag & | tag, |
bool | monitored = true ) |
Sets whether the specified tag shall be monitored for changes.
Same rules as for item monitoring apply.
- Parameters
-
tag Tag to monitor. monitored Whether to monitor the tag.
- Since
- 4.13
Definition at line 116 of file monitor.cpp.
◆ setTypeMonitored()
void Monitor::setTypeMonitored | ( | Monitor::Type | type, |
bool | monitored = true ) |
Sets whether given type (Collection, Item, Tag should be monitored).
By default all types are monitored, but once you change one, you have to explicitly enable all other types you want to monitor.
- Parameters
-
type Type to monitor. monitored Whether to monitor the type
- Since
- 4.13
Definition at line 133 of file monitor.cpp.
◆ tagAdded
|
signal |
This signal is emitted if a tag has been added to Akonadi storage.
- Parameters
-
tag The added tag
- Since
- 4.13
◆ tagChanged
|
signal |
This signal is emitted if a monitored tag is changed on the server.
- Parameters
-
tag The changed tag.
- Since
- 4.13
◆ tagFetchScope()
TagFetchScope & Monitor::tagFetchScope | ( | ) |
Returns the tag fetch scope.
Since this returns a reference it can be used to conveniently modify the current scope in-place, i.e. by calling a method on the returned reference without storing it in a local variable.
- Returns
- a reference to the current tag fetch scope
- See also
- setTagFetchScope() for replacing the current tag fetch scope
Definition at line 251 of file monitor.cpp.
◆ tagMonitored
|
signal |
This signal is emitted if the Monitor starts or stops monitoring tag
explicitly.
- Parameters
-
tag The tag. monitored Whether the tag is now being monitored or not.
- Since
- 4.13
◆ tagRemoved
|
signal |
This signal is emitted if a monitored tag is removed from the server storage.
The monitor will also emit itemTagsChanged() signal for all monitored items (if any) that were tagged by tag
.
- Parameters
-
tag The removed tag.
- Since
- 4.13
◆ tagsMonitored()
|
nodiscard |
◆ typeMonitored
|
signal |
This signal is emitted if the Monitor starts or stops monitoring type
explicitly.
- Parameters
-
type The type. monitored Whether the type is now being monitored or not.
- Since
- 4.13
◆ typesMonitored()
|
nodiscard |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:53:10 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.