Akonadi::Server::QueryBuilder
#include <querybuilder.h>
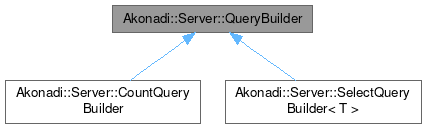
Public Types | |
enum | ConditionType { WhereCondition , HavingCondition , NUM_CONDITIONS } |
enum | JoinType { InnerJoin , LeftJoin , LeftOuterJoin } |
enum | QueryType { Select , Insert , Update , Delete } |
Public Member Functions | |
QueryBuilder (const QSqlQuery &tableQuery, const QString &tableQueryAlias) | |
QueryBuilder (const QString &table, QueryType type=Select) | |
QueryBuilder (DataStore *store, const QSqlQuery &tableQuery, const QString &tableQueryAlias) | |
QueryBuilder (DataStore *store, const QString &table, QueryType type=Select) | |
QueryBuilder (QueryBuilder &&) noexcept | |
void | addAggregation (const QString &col, const QString &aggregate) |
void | addAggregation (const Query::Case &caseStmt, const QString &aggregate) |
void | addColumn (const QString &col) |
void | addColumn (const Query::Case &caseStmt) |
void | addColumnCondition (const QString &column, Query::CompareOperator op, const QString &column2, ConditionType type=WhereCondition) |
void | addColumns (const QStringList &cols) |
void | addCondition (const Query::Condition &condition, ConditionType type=WhereCondition) |
void | addGroupColumn (const QString &column) |
void | addGroupColumns (const QStringList &columns) |
void | addJoin (JoinType joinType, const QString &table, const QString &col1, const QString &col2) |
void | addJoin (JoinType joinType, const QString &table, const Query::Condition &condition) |
void | addSortColumn (const QString &column, Query::SortOrder order=Query::Ascending) |
void | addValueCondition (const QString &column, Query::CompareOperator op, const QList< qint64 > &value, ConditionType type=WhereCondition) |
void | addValueCondition (const QString &column, Query::CompareOperator op, const QSet< qint64 > &value, ConditionType type=WhereCondition) |
void | addValueCondition (const QString &column, Query::CompareOperator op, const QVariant &value, ConditionType type=WhereCondition) |
bool | exec () |
QString | getTable () const |
QString | getTableWithColumn (const QString &column) const |
qint64 | insertId () |
QueryBuilder & | operator= (QueryBuilder &&) noexcept |
QSqlQuery & | query () |
void | setColumnValue (const QString &column, const QVariant &value) |
template<typename T> | |
void | setColumnValues (const QString &column, const QList< T > &values) |
void | setDatabaseType (DbType::Type type) |
void | setDistinct (bool distinct) |
void | setForUpdate (bool forUpdate=true) |
void | setIdentificationColumn (const QString &column) |
void | setLimit (int limit, int offset=-1) |
void | setSubQueryMode (Query::LogicOperator op, ConditionType type=WhereCondition) |
Protected Member Functions | |
DataStore * | dataStore () const |
Detailed Description
Helper class to construct arbitrary SQL queries.
Definition at line 32 of file querybuilder.h.
Member Enumeration Documentation
◆ ConditionType
Defines the place at which a condition should be evaluated.
Enumerator | |
---|---|
WhereCondition | add condition to WHERE part of the query |
HavingCondition | add condition to HAVING part of the query NOTE: only supported for SELECT queries |
Definition at line 61 of file querybuilder.h.
◆ JoinType
When the same table gets joined as both, Inner- and LeftJoin, it will be merged into a single InnerJoin since it is more restrictive.
Enumerator | |
---|---|
InnerJoin | NOTE: only supported for UPDATE and SELECT queries. |
LeftJoin | NOTE: only supported for SELECT queries. |
LeftOuterJoin | NOTE: only supported for SELECT queries. |
Definition at line 49 of file querybuilder.h.
◆ QueryType
enum Akonadi::Server::QueryBuilder::QueryType |
Definition at line 37 of file querybuilder.h.
Constructor & Destructor Documentation
◆ QueryBuilder() [1/5]
|
explicit |
Creates a new query builder.
- Parameters
-
table The main table to operate on.
Definition at line 111 of file querybuilder.cpp.
◆ QueryBuilder() [2/5]
QueryBuilder::QueryBuilder | ( | DataStore * | store, |
const QString & | table, | ||
QueryBuilder::QueryType | type = Select ) |
Definition at line 116 of file querybuilder.cpp.
◆ QueryBuilder() [3/5]
|
explicit |
Creates a new query builder with subquery in FROM clause for SELECT queries.
- Parameters
-
tableQuery must be a valid select query tableQueryAlias alias name for table query
Definition at line 136 of file querybuilder.cpp.
◆ QueryBuilder() [4/5]
QueryBuilder::QueryBuilder | ( | DataStore * | store, |
const QSqlQuery & | tableQuery, | ||
const QString & | tableQueryAlias ) |
Definition at line 141 of file querybuilder.cpp.
◆ QueryBuilder() [5/5]
|
noexcept |
Definition at line 162 of file querybuilder.cpp.
◆ ~QueryBuilder()
QueryBuilder::~QueryBuilder | ( | ) |
Definition at line 219 of file querybuilder.cpp.
Member Function Documentation
◆ addAggregation() [1/2]
Adds an aggregation statement.
- Parameters
-
col The column to aggregate on aggregate The aggregation function.
Definition at line 636 of file querybuilder.cpp.
◆ addAggregation() [2/2]
void QueryBuilder::addAggregation | ( | const Query::Case & | caseStmt, |
const QString & | aggregate ) |
Adds and aggregation statement with CASE.
- Parameters
-
caseStmt The case statement to aggregate on aggregate The aggregation function.
Definition at line 641 of file querybuilder.cpp.
◆ addColumn() [1/2]
void QueryBuilder::addColumn | ( | const QString & | col | ) |
Adds the given column to a select query.
- Parameters
-
col The column to add.
Definition at line 624 of file querybuilder.cpp.
◆ addColumn() [2/2]
void QueryBuilder::addColumn | ( | const Query::Case & | caseStmt | ) |
Adds the given case statement to a select query.
- Parameters
-
caseStmt The case statement to add.
Definition at line 629 of file querybuilder.cpp.
◆ addColumnCondition()
void QueryBuilder::addColumnCondition | ( | const QString & | column, |
Query::CompareOperator | op, | ||
const QString & | column2, | ||
ConditionType | type = WhereCondition ) |
Add a WHERE or HAVING condition which compares a column with another column.
- Parameters
-
column The column that should be compared. op The operator used for comparison. column2 The column column
is compared to.type Defines whether this condition should be part of the WHERE or the HAVING part of the query. Defaults to WHERE.
Definition at line 276 of file querybuilder.cpp.
◆ addColumns()
void QueryBuilder::addColumns | ( | const QStringList & | cols | ) |
Adds the given columns to a select query.
- Parameters
-
cols The columns you want to select.
Definition at line 619 of file querybuilder.cpp.
◆ addCondition()
void QueryBuilder::addCondition | ( | const Query::Condition & | condition, |
ConditionType | type = WhereCondition ) |
Add a WHERE condition.
Use this to build hierarchical conditions.
- Parameters
-
condition The condition that the resultset should satisfy. type Defines whether this condition should be part of the WHERE or the HAVING part of the query. Defaults to WHERE.
Definition at line 760 of file querybuilder.cpp.
◆ addGroupColumn()
void QueryBuilder::addGroupColumn | ( | const QString & | column | ) |
Add a GROUP BY column.
NOTE: Only supported in SELECT queries.
- Parameters
-
column Name of the column to use for grouping.
Definition at line 771 of file querybuilder.cpp.
◆ addGroupColumns()
void QueryBuilder::addGroupColumns | ( | const QStringList & | columns | ) |
Add list of columns to GROUP BY.
NOTE: Only supported in SELECT queries.
- Parameters
-
columns Names of columns to use for grouping.
Definition at line 777 of file querybuilder.cpp.
◆ addJoin() [1/2]
void QueryBuilder::addJoin | ( | JoinType | joinType, |
const QString & | table, | ||
const QString & | col1, | ||
const QString & | col2 ) |
Join a table to the query.
This is a convenience method to create simple joins like e.g. 'LEFT JOIN t ON c1 = c2'.
NOTE: make sure the JoinType
is supported by the current QueryType
- Parameters
-
joinType The type of JOIN you want to add. table The table to join. col1 The first column for the ON statement. col2 The second column for the ON statement.
Definition at line 251 of file querybuilder.cpp.
◆ addJoin() [2/2]
void QueryBuilder::addJoin | ( | JoinType | joinType, |
const QString & | table, | ||
const Query::Condition & | condition ) |
Join a table to the query.
NOTE: make sure the JoinType
is supported by the current QueryType
- Parameters
-
joinType The type of JOIN you want to add. table The table to join. condition the ON condition for this join.
Definition at line 236 of file querybuilder.cpp.
◆ addSortColumn()
void QueryBuilder::addSortColumn | ( | const QString & | column, |
Query::SortOrder | order = Query::Ascending ) |
Add sort column.
- Parameters
-
column Name of the column to sort. order Sort order
Definition at line 766 of file querybuilder.cpp.
◆ addValueCondition() [1/3]
void QueryBuilder::addValueCondition | ( | const QString & | column, |
Query::CompareOperator | op, | ||
const QList< qint64 > & | value, | ||
ConditionType | type = WhereCondition ) |
Add a WHERE or HAVING condition which compares a column with a given value.
This is an overload specially for passing a list of IDs, which is a fairly common case in Akonadi.
- Parameters
-
column The column that should be compared. op The operator used for comparison. value The value column
is compared to.type Defines whether this condition should be part of the WHERE or the HAVING part of the query. Defaults to WHERE.
Definition at line 264 of file querybuilder.cpp.
◆ addValueCondition() [2/3]
void QueryBuilder::addValueCondition | ( | const QString & | column, |
Query::CompareOperator | op, | ||
const QSet< qint64 > & | value, | ||
ConditionType | type = WhereCondition ) |
Add a WHERE or HAVING condition which compares a column with a given value.
This is an overload specially for passing a set of IDs, which is a fairly common case in Akonadi.
- Parameters
-
column The column that should be compared. op The operator used for comparison. value The value column
is compared to.type Defines whether this condition should be part of the WHERE or the HAVING part of the query. Defaults to WHERE.
Definition at line 270 of file querybuilder.cpp.
◆ addValueCondition() [3/3]
void QueryBuilder::addValueCondition | ( | const QString & | column, |
Query::CompareOperator | op, | ||
const QVariant & | value, | ||
ConditionType | type = WhereCondition ) |
Add a WHERE or HAVING condition which compares a column with a given value.
- Parameters
-
column The column that should be compared. op The operator used for comparison. value The value column
is compared to.type Defines whether this condition should be part of the WHERE or the HAVING part of the query. Defaults to WHERE.
Definition at line 258 of file querybuilder.cpp.
◆ dataStore()
|
inlineprotected |
Definition at line 347 of file querybuilder.h.
◆ exec()
bool QueryBuilder::exec | ( | ) |
Executes the query, returns true on success.
Definition at line 508 of file querybuilder.cpp.
◆ getTable()
QString QueryBuilder::getTable | ( | ) | const |
Returns the name of the main table or subquery.
Definition at line 841 of file querybuilder.cpp.
◆ getTableWithColumn()
Returns concatenated table name with column name.
- Parameters
-
column Column name.
- Note
- Pass only
column
that are not prefixed by table name.
Definition at line 846 of file querybuilder.cpp.
◆ insertId()
qint64 QueryBuilder::insertId | ( | ) |
Returns the ID of the newly created record (only valid for INSERT queries)
- Note
- This will assert when being used with setIdentificationColumn() called with an empty string.
- Returns
- -1 if invalid
Definition at line 813 of file querybuilder.cpp.
◆ operator=()
|
noexcept |
Definition at line 189 of file querybuilder.cpp.
◆ query()
|
inline |
Returns the query, only valid after exec().
Definition at line 291 of file querybuilder.h.
◆ setColumnValue()
Sets a column to the given value (only valid for INSERT and UPDATE queries).
Calling this function resets any values set by setColumnValues().
- Parameters
-
column Column to change. value The value column
should be set to.
Definition at line 783 of file querybuilder.cpp.
◆ setColumnValues()
|
inline |
Set column to given values (only valid for INSERT query).
This will result in the query inserting multiple rows. The values must contain the same number of elements for each column otherwise the query will fail.
Calling this function resets any values set by setColumnValue().
- Parameters
-
column Column to insert into. values Values to be set for the column
.
Definition at line 258 of file querybuilder.h.
◆ setDatabaseType()
void QueryBuilder::setDatabaseType | ( | DbType::Type | type | ) |
Sets the database which should execute the query.
Unfortunately the SQL "standard" is not interpreted in the same way everywhere...
Definition at line 231 of file querybuilder.cpp.
◆ setDistinct()
void QueryBuilder::setDistinct | ( | bool | distinct | ) |
Specify whether duplicates should be included in the result.
- Parameters
-
distinct true
to remove duplicates,false
is the default
Definition at line 797 of file querybuilder.cpp.
◆ setForUpdate()
void QueryBuilder::setForUpdate | ( | bool | forUpdate = true | ) |
Indicate to the database to acquire an exclusive lock on the rows already during SELECT statement.
Only makes sense in SELECT queries.
Definition at line 836 of file querybuilder.cpp.
◆ setIdentificationColumn()
void QueryBuilder::setIdentificationColumn | ( | const QString & | column | ) |
Sets the column used for identification in an INSERT statement.
The default is "id", only change this on tables without such a column (usually n:m helper tables).
- Parameters
-
column Name of the identification column, empty string to disable this.
- Note
- This only affects PostgreSQL.
- See also
- insertId()
Definition at line 808 of file querybuilder.cpp.
◆ setLimit()
void QueryBuilder::setLimit | ( | int | limit, |
int | offset = -1 ) |
Limits the amount of retrieved rows.
- Parameters
-
limit the maximum number of rows to retrieve. offset offset of the first row to retrieve. The default value for offset
is -1, indicating no offset.
- Note
- This has no effect on anything but SELECT queries.
Definition at line 802 of file querybuilder.cpp.
◆ setSubQueryMode()
void QueryBuilder::setSubQueryMode | ( | Query::LogicOperator | op, |
ConditionType | type = WhereCondition ) |
Define how WHERE or HAVING conditions are combined.
- Todo
- Give this method a better name.
- Parameters
-
op The logical operator that should be used to combine the conditions. type Defines whether the operator should be used for WHERE or for HAVING conditions. Defaults to WHERE conditions.
Definition at line 754 of file querybuilder.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:50:41 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.