KGAPI2::EventFetchJob
#include <eventfetchjob.h>
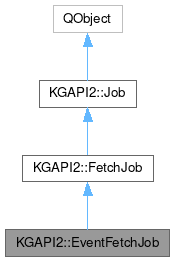
Properties | |
bool | fetchDeleted |
quint64 | fetchOnlyUpdated |
QString | filter |
QString | syncToken |
quint64 | timeMax |
quint64 | timeMin |
![]() | |
bool | isRunning |
int | maxTimeout |
![]() | |
objectName | |
Public Member Functions | |
EventFetchJob (const QString &calendarId, const AccountPtr &account, QObject *parent=nullptr) | |
EventFetchJob (const QString &eventId, const QString &calendarId, const AccountPtr &account, QObject *parent=nullptr) | |
~EventFetchJob () override | |
QList< Event::EventType > | eventTypes () const |
bool | fetchDeleted () |
quint64 | fetchOnlyUpdated () const |
QString | filter () const |
void | setEventTypes (const QList< Event::EventType > eventTypes) |
void | setFetchDeleted (bool fetchDeleted=true) |
void | setFetchOnlyUpdated (quint64 timestamp) |
void | setFilter (const QString &query) |
void | setSyncToken (const QString &syncToken) |
void | setTimeMax (quint64 timestamp) |
void | setTimeMin (quint64 timestamp) |
QString | syncToken () const |
quint64 | timeMax () const |
quint64 | timeMin () const |
![]() | |
FetchJob (const KGAPI2::AccountPtr &account, QObject *parent=nullptr) | |
FetchJob (QObject *parent=nullptr) | |
~FetchJob () override | |
virtual ObjectsList | items () const |
![]() | |
Job (const AccountPtr &account, QObject *parent=nullptr) | |
Job (QObject *parent=nullptr) | |
~Job () override | |
AccountPtr | account () const |
KGAPI2::Error | error () const |
QString | errorString () const |
QStringList | fields () const |
bool | isRunning () const |
int | maxTimeout () const |
bool | prettyPrint () const |
void | restart () |
void | setAccount (const AccountPtr &account) |
void | setFields (const QStringList &fields) |
void | setMaxTimeout (int maxTimeout) |
void | setPrettyPrint (bool prettyPrint) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
bool | handleError (int errorCode, const QByteArray &rawData) override |
ObjectsList | handleReplyWithItems (const QNetworkReply *reply, const QByteArray &rawData) override |
void | start () override |
![]() | |
void | aboutToStart () override |
void | dispatchRequest (QNetworkAccessManager *accessManager, const QNetworkRequest &request, const QByteArray &data, const QString &contentType) override |
void | handleReply (const QNetworkReply *reply, const QByteArray &rawData) override |
![]() | |
virtual void | aboutToFinish () |
virtual void | emitFinished () |
virtual void | emitProgress (int processed, int total) |
virtual void | enqueueRequest (const QNetworkRequest &request, const QByteArray &data=QByteArray(), const QString &contentType=QString()) |
void | setError (KGAPI2::Error error) |
void | setErrorString (const QString &errorString) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
void | finished (KGAPI2::Job *job) |
void | progress (KGAPI2::Job *job, int processed, int total) |
![]() | |
static QString | buildSubfields (const QString &field, const QStringList &fields) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
A job to fetch all events from given calendar in user's Google Calendar account.
- Since
- 2.0
Definition at line 27 of file eventfetchjob.h.
Property Documentation
◆ fetchDeleted
|
readwrite |
Whether to fetch deleted events as well.
When an event is deleted from Google Calendar, it's stored as a placeholder on Google server and can still be retrieved. Such event will have KGAPI2::Event::deleted set to true
.
By default, the job will fetch deleted events.
This property does not have any effect when fetching a specific event and can be modified only when the job is not running.
- See also
- setFetchDeleted, fetchDeleted
Definition at line 45 of file eventfetchjob.h.
◆ fetchOnlyUpdated
|
readwrite |
Timestamp to fetch only events modified since then.
When set, this job will only fetch events that have been modified since given timestamp.
By default the timestamp is 0 and all events are fetched.
This property does not have any effect when fetching a specific event and can be modified only when the job is not running.
- See also
- setFetchOnlyUpdated, fetchOnlyUpdated
Definition at line 60 of file eventfetchjob.h.
◆ filter
|
readwrite |
A filter to fetch only events matching fulltext filter.
By default the property is empty and no filter is applied.
This property does not have any effect when fetching a specific event and can be modified only when the job is not running.
Definition at line 102 of file eventfetchjob.h.
◆ syncToken
|
readwrite |
A token to fetch updates incrementally.
By default the property is empty. Properties timeMin, timeMax, updatedMin will be ignored if sync token is specified
- See also
- setSyncToken, syncToken
Definition at line 112 of file eventfetchjob.h.
◆ timeMax
|
readwrite |
Timestamp of the newest event that will be fetched.
Only events occurring before or precisely at the time indicated by this property will be fetched.
By default the timestamp is 0 and no limit is applied.
This property does not have any effect when fetching a specific event and can be modified only when the job is not running.
- See also
- setMaxTime, maxTime
Definition at line 75 of file eventfetchjob.h.
◆ timeMin
|
readwrite |
Timestamp of the oldest event that will be fetched.
Only events occurring after or precisely at the time indicated by this property will be fetched.
By default the timestamp is 0 and no limit is applied.
This property does not have any effect when fetching a specific event and can be modified only when the job is not running.
- See also
- setMinTime, minTime
Definition at line 90 of file eventfetchjob.h.
Constructor & Destructor Documentation
◆ EventFetchJob() [1/2]
|
explicit |
Constructs a job that will fetch all events from a calendar with given calendarId
.
Result of this job might not contain all events, depending on configured filters.
- Parameters
-
calendarId ID of calendar from which to fetch events account Account to authenticate the request parent
Definition at line 36 of file eventfetchjob.cpp.
◆ EventFetchJob() [2/2]
|
explicit |
Constructs a job that will fetch an event with given eventId
from a calendar with given calendarId
.
Note that none of the filter, fetchOnlyUpdated, timeMax or timeMin properties is applied in this case.
- Parameters
-
eventId ID of event to fetch calendarId ID of calendar in which the event is account Account to authenticate the request parent
Definition at line 43 of file eventfetchjob.cpp.
◆ ~EventFetchJob()
|
overridedefault |
Destructor.
Member Function Documentation
◆ eventTypes()
QList< Event::EventType > KGAPI2::EventFetchJob::eventTypes | ( | ) | const |
Returns the types of events to retrieve.
◆ fetchDeleted()
|
nodiscard |
Returns whether deleted events are fetched.
◆ fetchOnlyUpdated()
|
nodiscard |
Returns whether the job will fetch only modified events.
- Returns
- 0 when all events will be fetched, a timestamp of since when the modified events will be fetched.
Definition at line 78 of file eventfetchjob.cpp.
◆ filter()
|
nodiscard |
Returns fulltext filter string.
Definition at line 133 of file eventfetchjob.cpp.
◆ handleError()
|
overrideprotectedvirtual |
KGAPI2::Job::handleError implementation.
- Parameters
-
errorCode rawData
Reimplemented from KGAPI2::Job.
Definition at line 172 of file eventfetchjob.cpp.
◆ handleReplyWithItems()
|
overrideprotectedvirtual |
KGAPI2::FetchJob::handleReplyWithItems implementation.
- Parameters
-
reply rawData
Reimplemented from KGAPI2::FetchJob.
Definition at line 185 of file eventfetchjob.cpp.
◆ setEventTypes()
void KGAPI2::EventFetchJob::setEventTypes | ( | const QList< Event::EventType > | eventTypes | ) |
Sets the types of events to retrieve.
Default set is EventType::Default, EventType::FocusTime and EventType::OutOfOffice.
◆ setFetchDeleted()
void EventFetchJob::setFetchDeleted | ( | bool | fetchDeleted = true | ) |
Sets whether to fetch deleted events.
- Parameters
-
fetchDeleted
Definition at line 53 of file eventfetchjob.cpp.
◆ setFetchOnlyUpdated()
void EventFetchJob::setFetchOnlyUpdated | ( | quint64 | timestamp | ) |
Sets the job to fetch only events modified since timestamp
.
- Parameters
-
timestamp
Definition at line 68 of file eventfetchjob.cpp.
◆ setFilter()
void EventFetchJob::setFilter | ( | const QString & | query | ) |
◆ setSyncToken()
void EventFetchJob::setSyncToken | ( | const QString & | syncToken | ) |
Sets token for incremental updates.
- Parameters
-
syncToken
Definition at line 98 of file eventfetchjob.cpp.
◆ setTimeMax()
void EventFetchJob::setTimeMax | ( | quint64 | timestamp | ) |
Sets timestamp of newest event that can be fetched.
- Parameters
-
timestamp
Definition at line 83 of file eventfetchjob.cpp.
◆ setTimeMin()
void EventFetchJob::setTimeMin | ( | quint64 | timestamp | ) |
Sets timestamp of older events that can be fetched.
- Parameters
-
timestamp
Definition at line 108 of file eventfetchjob.cpp.
◆ start()
|
overrideprotectedvirtual |
KGAPI2::Job::start implementation.
Implements KGAPI2::Job.
Definition at line 138 of file eventfetchjob.cpp.
◆ syncToken()
|
nodiscard |
Token for next incremental update.
Definition at line 103 of file eventfetchjob.cpp.
◆ timeMax()
|
nodiscard |
Returns upper limit for event occurrence.
Definition at line 93 of file eventfetchjob.cpp.
◆ timeMin()
|
nodiscard |
Returns lower boundary for events occurrence.
Definition at line 118 of file eventfetchjob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:48:08 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.