KGAPI2::FetchJob
#include <fetchjob.h>
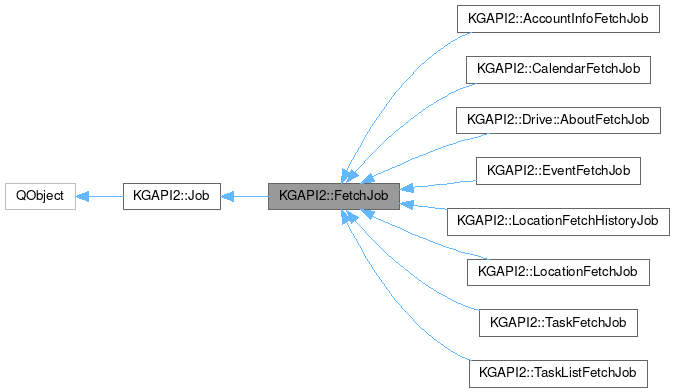
Public Member Functions | |
FetchJob (const KGAPI2::AccountPtr &account, QObject *parent=nullptr) | |
FetchJob (QObject *parent=nullptr) | |
~FetchJob () override | |
virtual ObjectsList | items () const |
![]() | |
Job (const AccountPtr &account, QObject *parent=nullptr) | |
Job (QObject *parent=nullptr) | |
~Job () override | |
AccountPtr | account () const |
KGAPI2::Error | error () const |
QString | errorString () const |
QStringList | fields () const |
bool | isRunning () const |
int | maxTimeout () const |
bool | prettyPrint () const |
void | restart () |
void | setAccount (const AccountPtr &account) |
void | setFields (const QStringList &fields) |
void | setMaxTimeout (int maxTimeout) |
void | setPrettyPrint (bool prettyPrint) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
void | aboutToStart () override |
void | dispatchRequest (QNetworkAccessManager *accessManager, const QNetworkRequest &request, const QByteArray &data, const QString &contentType) override |
void | handleReply (const QNetworkReply *reply, const QByteArray &rawData) override |
virtual ObjectsList | handleReplyWithItems (const QNetworkReply *reply, const QByteArray &rawData) |
![]() | |
virtual void | aboutToFinish () |
virtual void | emitFinished () |
virtual void | emitProgress (int processed, int total) |
virtual void | enqueueRequest (const QNetworkRequest &request, const QByteArray &data=QByteArray(), const QString &contentType=QString()) |
virtual bool | handleError (int statusCode, const QByteArray &rawData) |
void | setError (KGAPI2::Error error) |
void | setErrorString (const QString &errorString) |
virtual void | start ()=0 |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | isRunning |
int | maxTimeout |
![]() | |
objectName | |
![]() | |
void | finished (KGAPI2::Job *job) |
void | progress (KGAPI2::Job *job, int processed, int total) |
![]() | |
static QString | buildSubfields (const QString &field, const QStringList &fields) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | QObjectList |
Detailed Description
Abstract superclass for all jobs that fetch resources from Google.
- Since
- 2.0
Definition at line 24 of file fetchjob.h.
Constructor & Destructor Documentation
◆ FetchJob() [1/2]
|
explicit |
Constructor for jobs that don't require authentication.
- Parameters
-
parent
Definition at line 24 of file fetchjob.cpp.
◆ FetchJob() [2/2]
|
explicit |
Constructor for jobs that require authentication.
- Parameters
-
account Account to use to authenticate the requests sent by this job parent
Definition at line 30 of file fetchjob.cpp.
◆ ~FetchJob()
|
override |
Destructor.
Definition at line 36 of file fetchjob.cpp.
Member Function Documentation
◆ aboutToStart()
|
overrideprotectedvirtual |
KGAPI::Job::aboutToStart implementation.
Reimplemented from KGAPI2::Job.
Definition at line 64 of file fetchjob.cpp.
◆ dispatchRequest()
|
overrideprotectedvirtual |
KGAPI::Job::dispatchRequest implementation.
- Parameters
-
accessManager request data contentType
Implements KGAPI2::Job.
Definition at line 51 of file fetchjob.cpp.
◆ handleReply()
|
overrideprotectedvirtual |
KGAPI::Job::handleReply implementation.
- Parameters
-
reply rawData
Implements KGAPI2::Job.
Definition at line 59 of file fetchjob.cpp.
◆ handleReplyWithItems()
|
protectedvirtual |
A reply handler that returns items parsed from @ rawData.
This method can be reimplemented in a FetchJob subclasses. It is called automatically when a reply is received and the returned items are stored in FetchJob and accessible via FetchJob::items when the job has finished.
If you need more control over handling reply and items, you can reimplement FetchJob::handleReply. Note that reimplementing FetchJob::handleReply usually requires reimplementing FetchJob::items as well and storing the parsed items in your implementation.
- Parameters
-
reply A QNetworkReply received from Google server rawData Content of body of the reply
. Don't use QNetworkReply::readAll(), because the content has already been read by Job implementation and thus it would return empty data.
- Returns
- Items parsed from
rawData
Reimplemented in KGAPI2::AccountInfoFetchJob, KGAPI2::CalendarFetchJob, KGAPI2::Drive::AboutFetchJob, KGAPI2::EventFetchJob, KGAPI2::LocationFetchHistoryJob, KGAPI2::LocationFetchJob, KGAPI2::TaskFetchJob, and KGAPI2::TaskListFetchJob.
Definition at line 71 of file fetchjob.cpp.
◆ items()
|
virtual |
Returns all items fetched by this job.
Returns all items fetch by this job. This method can be called only from handler of Job::finished signal. Calling this method on a running job will print a warning and return an empty list.
- Returns
- All items fetched by this job.
Definition at line 41 of file fetchjob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:03:45 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.