KGAPI2::Job
#include <job.h>
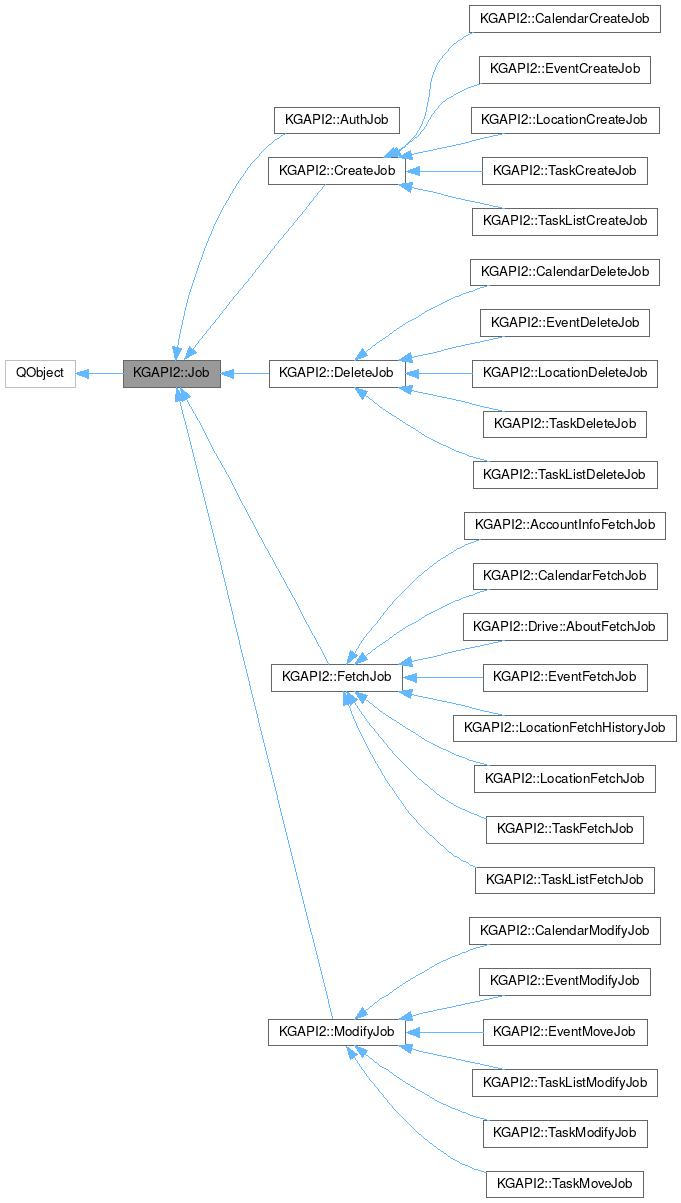
Properties | |
bool | isRunning |
int | maxTimeout |
![]() | |
objectName | |
Signals | |
void | finished (KGAPI2::Job *job) |
void | progress (KGAPI2::Job *job, int processed, int total) |
Public Member Functions | |
Job (const AccountPtr &account, QObject *parent=nullptr) | |
Job (QObject *parent=nullptr) | |
~Job () override | |
AccountPtr | account () const |
KGAPI2::Error | error () const |
QString | errorString () const |
QStringList | fields () const |
bool | isRunning () const |
int | maxTimeout () const |
bool | prettyPrint () const |
void | restart () |
void | setAccount (const AccountPtr &account) |
void | setFields (const QStringList &fields) |
void | setMaxTimeout (int maxTimeout) |
void | setPrettyPrint (bool prettyPrint) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static QString | buildSubfields (const QString &field, const QStringList &fields) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
virtual void | aboutToFinish () |
virtual void | aboutToStart () |
virtual void | dispatchRequest (QNetworkAccessManager *accessManager, const QNetworkRequest &request, const QByteArray &data, const QString &contentType)=0 |
virtual void | emitFinished () |
virtual void | emitProgress (int processed, int total) |
virtual void | enqueueRequest (const QNetworkRequest &request, const QByteArray &data=QByteArray(), const QString &contentType=QString()) |
virtual bool | handleError (int statusCode, const QByteArray &rawData) |
virtual void | handleReply (const QNetworkReply *reply, const QByteArray &rawData)=0 |
void | setError (KGAPI2::Error error) |
void | setErrorString (const QString &errorString) |
virtual void | start ()=0 |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
Detailed Description
Abstract base class for all jobs in LibKGAPI.
Usual workflow of Job subclasses is to reimplement Job::start, Job::dispatchRequest and Job::handleReply, then enqueue a QNetworkRequest using Job::enqueueRequest. Authorization headers and standard query parameters will be set by Job class. The request will automatically be scheduled in a queue and dispatched by calling Job::dispatchRequest implementation. When a reply is received, the Job will automatically perform error handling and if there is no error, the reply is passed to implementation of Job::handleReply.
Job is automatically started when program enters an event loop.
- Since
- 2.0
Property Documentation
◆ isRunning
|
read |
Whether the job is running.
This property indicates whether the job is running or not. The value is set to true
when the job is started (see Job::start) and back to false
right before Job::finished is emitted.
- See also
- Job::isRunning, Job::finished
◆ maxTimeout
|
readwrite |
Maximum interval between requests.
Some Google APIs have a quota on maximum amount of requests per account per second. When this quota is exceeded, the Job will automatically increase the interval between dispatching requests, wait for a while and then try again. If however the interval is increased over maxTimeout
, the job will fail and finish immediately. By default maxTimeout
is -1
, which allows the interval to be increased indefinitely.
- See also
- Job::maxTimeout, Job::setMaxTimeout
Constructor & Destructor Documentation
◆ Job() [1/2]
|
explicit |
◆ Job() [2/2]
|
explicit |
Constructor for jobs that require authentication.
- Parameters
-
account Account to use to authenticate the requests send by this job parent
- See also
- Job::Account, Job::setAccount
◆ ~Job()
Member Function Documentation
◆ aboutToFinish()
|
protectedvirtual |
This method is invoked right before finished() is emitted.
Subclasses can reimplement this method to do a final cleanup before the Job::finished() signal is emitted.
- Note
- Note that after Job::finished() the job is not running anymore and therefore the job should not modify any data accessible by user.
◆ aboutToStart()
|
protectedvirtual |
This method is invoked right before Job::start() is called.
Subclasses should reset their internal state and call parent implementation.
Reimplemented in KGAPI2::CreateJob, KGAPI2::FetchJob, and KGAPI2::ModifyJob.
◆ account()
AccountPtr Job::account | ( | ) | const |
◆ buildSubfields()
|
static |
◆ dispatchRequest()
|
protectedpure virtual |
Dispatches request
via accessManager
.
Because different types of request require different HTTP method to be used, subclasses must reimplement this method and use respective HTTP method to send the request
via accessManager
.
- Parameters
-
accessManager QNetworkAccessManager used to dispatch the request request Request to dispatch data Data to sent in the body of the request contentType Content-Type of data
Implemented in KGAPI2::AuthJob, KGAPI2::CreateJob, KGAPI2::DeleteJob, KGAPI2::Drive::FileAbstractResumableJob, KGAPI2::EventMoveJob, KGAPI2::FetchJob, KGAPI2::ModifyJob, and KGAPI2::TaskMoveJob.
◆ emitFinished()
|
protectedvirtual |
Emits Job::finished() signal.
Subclasses should always use this method instead of directly emitting Job::finished().
◆ emitProgress()
|
protectedvirtual |
Emit progress() signal.
Subclasses should always use this method instead of directly emitting Job::progress().
- Parameters
-
processed Amount of already processed items total Total amount of items to process
◆ enqueueRequest()
|
protectedvirtual |
Enqueues request
in dispatcher queue.
Subclasses should call this method to enqueue the request
in main job queue. The request is automatically dispatched, and reply is handled. Authorization headers and standards query parameters will be applied.
- Parameters
-
request Request to enqueue data Data to be send in body of the request contentType Content type of data
◆ error()
Error Job::error | ( | ) | const |
Error code.
This method can only be called after the job has emitted Job::finished signal. Calling this method on a running job will always return KGAPI2::NoError.
- Returns
- Returns code of occurred error or KGAPI2::NoError when no error has occurred.
◆ errorString()
QString Job::errorString | ( | ) | const |
Error string.
This method can only be called after the job has emitted Job::finished signal. Calling this method on a running job will always return an empty string.
- Returns
- Returns localized description of error or an empty string if no error has occurred.
◆ fields()
QStringList Job::fields | ( | ) | const |
◆ finished
|
signal |
Emitted when job
has finished.
The signal is emitted every time, no matter whether the job is successful or an error has occurred.
Subclasses should never ever emit this signal directly. Use Job::emitFinished instead.
- Parameters
-
job The job that has finished
- See also
- emitFinished()
◆ handleError()
|
protectedvirtual |
Called when an error occurs.
This allows subclasses to handle error cases that might be specific for their particular API they are implementing. The re-implementation should return true
if the error was handled and KGAPI should not perform any more error handling. If the error was not handled, the re-implementation should return false
, which is also what the default implementation returns.
- Parameters
-
statusCode The status code of the respons. rawData Raw data of the server response.
- Returns
- Returns true when the error was handled by the subclass, false otherwise.
Reimplemented in KGAPI2::EventFetchJob.
◆ handleReply()
|
protectedpure virtual |
Called when a reply is received.
Subclasses must reimplement this method to handle reply content.
- Parameters
-
reply A reply received from server rawData Raw content of the reply. Don't use QNetworkReply::readAll, because this method has already been called by Job and thus it would return nothing.
Implemented in KGAPI2::AuthJob, KGAPI2::CalendarDeleteJob, KGAPI2::CreateJob, KGAPI2::DeleteJob, KGAPI2::Drive::FileAbstractResumableJob, KGAPI2::EventDeleteJob, KGAPI2::FetchJob, KGAPI2::ModifyJob, KGAPI2::TaskDeleteJob, KGAPI2::TaskListDeleteJob, and KGAPI2::TaskMoveJob.
◆ isRunning()
bool Job::isRunning | ( | ) | const |
Whether job is running.
A job is considered running from the moment it's started until until Job::finished is emitted. Some methods should not be called when a job is running.
- Returns
- Returns whether this job is currently running.
- See also
- start()
◆ maxTimeout()
int Job::maxTimeout | ( | ) | const |
Maximum quota limit.
- Returns
- Returns maximum timeout in seconds or -1 if there is no timeout set.
- See also
- Job::setMaxTimeout
◆ prettyPrint()
bool Job::prettyPrint | ( | ) | const |
◆ progress
|
signal |
Emitted when a job progress changes.
Note that some jobs might not provide progress information, thus this signal will never be emitted.
- Parameters
-
job The job that the information relates to processed Amount of already processed items total Total amount of items to process
◆ restart()
void Job::restart | ( | ) |
Restarts this job.
When a job finishes, it's possible to run it again, without having to create a new job.
The job will throw away all results retrieved in previous run and retrieve everything again.
- See also
- Job::aboutToStart
◆ setAccount()
void Job::setAccount | ( | const AccountPtr & | account | ) |
◆ setError()
|
protected |
◆ setErrorString()
|
protected |
Set job error description to errorString
.
- Parameters
-
errorString Error description to set
- See also
- Job::errorString
◆ setFields()
void Job::setFields | ( | const QStringList & | fields | ) |
◆ setMaxTimeout()
void Job::setMaxTimeout | ( | int | maxTimeout | ) |
Set maximum quota timeout.
Sets maximum interval for which the job should wait before trying to submit a request that has previously failed due to exceeded quota.
Default timeout is 1 seconds, then after every failed request the timeout is increased exponentially until reaching maxTimeout
.
- Parameters
-
maxTimeout Maximum timeout (in seconds), or -1
for no timeout
◆ setPrettyPrint()
void Job::setPrettyPrint | ( | bool | prettyPrint | ) |
◆ start()
|
protectedpure virtual |
This method is invoked when job is started.
Job is automatically started when application enters event loop.
Implemented in KGAPI2::AccountInfoFetchJob, KGAPI2::AuthJob, KGAPI2::CalendarCreateJob, KGAPI2::CalendarDeleteJob, KGAPI2::CalendarFetchJob, KGAPI2::CalendarModifyJob, KGAPI2::Drive::AboutFetchJob, KGAPI2::Drive::FileAbstractResumableJob, KGAPI2::EventCreateJob, KGAPI2::EventDeleteJob, KGAPI2::EventFetchJob, KGAPI2::EventModifyJob, KGAPI2::EventMoveJob, KGAPI2::LocationCreateJob, KGAPI2::LocationDeleteJob, KGAPI2::LocationFetchHistoryJob, KGAPI2::LocationFetchJob, KGAPI2::TaskCreateJob, KGAPI2::TaskDeleteJob, KGAPI2::TaskFetchJob, KGAPI2::TaskListCreateJob, KGAPI2::TaskListDeleteJob, KGAPI2::TaskListFetchJob, KGAPI2::TaskListModifyJob, KGAPI2::TaskModifyJob, and KGAPI2::TaskMoveJob.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:13:27 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.