KGAPI2::TaskCreateJob
#include <taskcreatejob.h>
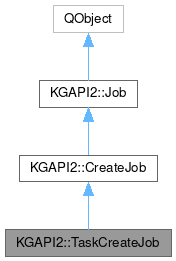
Properties | |
QString | parentItem |
QString | previous |
![]() | |
bool | isRunning |
int | maxTimeout |
![]() | |
objectName | |
Public Member Functions | |
TaskCreateJob (const TaskPtr &task, const QString &taskListId, const AccountPtr &account, QObject *parent=nullptr) | |
TaskCreateJob (const TasksList &tasks, const QString &taskListId, const AccountPtr &account, QObject *parent=nullptr) | |
~TaskCreateJob () override | |
QString | parentItem () const |
QString | previous () const |
void | setParentItem (const QString &parentId) |
void | setPrevious (const QString &previousId) |
![]() | |
CreateJob (const KGAPI2::AccountPtr &account, QObject *parent=nullptr) | |
CreateJob (QObject *parent=nullptr) | |
~CreateJob () override | |
virtual ObjectsList | items () const |
![]() | |
Job (const AccountPtr &account, QObject *parent=nullptr) | |
Job (QObject *parent=nullptr) | |
~Job () override | |
AccountPtr | account () const |
KGAPI2::Error | error () const |
QString | errorString () const |
QStringList | fields () const |
bool | isRunning () const |
int | maxTimeout () const |
bool | prettyPrint () const |
void | restart () |
void | setAccount (const AccountPtr &account) |
void | setFields (const QStringList &fields) |
void | setMaxTimeout (int maxTimeout) |
void | setPrettyPrint (bool prettyPrint) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
ObjectsList | handleReplyWithItems (const QNetworkReply *reply, const QByteArray &rawData) override |
void | start () override |
![]() | |
void | aboutToStart () override |
void | dispatchRequest (QNetworkAccessManager *accessManager, const QNetworkRequest &request, const QByteArray &data, const QString &contentType) override |
void | handleReply (const QNetworkReply *reply, const QByteArray &rawData) override |
![]() | |
virtual void | aboutToFinish () |
virtual void | emitFinished () |
virtual void | emitProgress (int processed, int total) |
virtual void | enqueueRequest (const QNetworkRequest &request, const QByteArray &data=QByteArray(), const QString &contentType=QString()) |
virtual bool | handleError (int statusCode, const QByteArray &rawData) |
void | setError (KGAPI2::Error error) |
void | setErrorString (const QString &errorString) |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
![]() | |
void | finished (KGAPI2::Job *job) |
void | progress (KGAPI2::Job *job, int processed, int total) |
![]() | |
static QString | buildSubfields (const QString &field, const QStringList &fields) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Detailed Description
A job to create one or more new tasks in Google Tasks.
- Since
- 2.0
Definition at line 25 of file taskcreatejob.h.
Property Documentation
◆ parentItem
|
readwrite |
Specified ID of item that the newly created tasks will be subtasks of.
By default this property is empty and all tasks are created in the top level of their parent tasklist
This property can only be modified when job is not running.
- See also
- setParentItem, parentItem
Definition at line 40 of file taskcreatejob.h.
◆ previous
|
readwrite |
Previous sibling task identifier.
If the task is created at the first position among its siblings, this parameter is omitted.
This property can only be modified when job is not running.
- See also
- setPrevious, previous
Definition at line 50 of file taskcreatejob.h.
Constructor & Destructor Documentation
◆ TaskCreateJob() [1/2]
|
explicit |
Constructs a job that will create given task
in a tasklist with id taskListId
.
- Parameters
-
task Task to store taskListId ID of tasklist to create the task in account Account to authenticate the request parent
Definition at line 38 of file taskcreatejob.cpp.
◆ TaskCreateJob() [2/2]
|
explicit |
Constructs a job that will create given tasks
in a tasklist with id taskListId
.
- Parameters
-
tasks Tasks to store taskListId ID of tasklist to create the task in account Account to authenticate the request parent
Definition at line 46 of file taskcreatejob.cpp.
◆ ~TaskCreateJob()
|
overridedefault |
Destructor.
Member Function Documentation
◆ handleReplyWithItems()
|
overrideprotectedvirtual |
A reply handler that returns items parsed from @ rawData.
This method can be reimplemented in FetchJob subclasses. It is called automatically when a reply is received and the returned items are stored in FetchJob and accessible via FetchJob::items when the job has finished.
If you need more control over handling reply and items, you can reimplement FetchJob::handleReply. Note that reimplementing FetchJob::handleReply usually requires reimplementing FetchJob::items as well and storing the parsed items in your implementation.
- Parameters
-
reply A QNetworkReply received from Google's server rawData Content of body of the reply
. Don't use QNetworkReply::readAll(), because the content has already been read by Job implementation and thus it would return empty data.
- Returns
- Items parsed from
rawData
Reimplemented from KGAPI2::CreateJob.
Definition at line 111 of file taskcreatejob.cpp.
◆ parentItem()
QString TaskCreateJob::parentItem | ( | ) | const |
Returns ID of task the new items will be stored as subtasks of.
Definition at line 56 of file taskcreatejob.cpp.
◆ previous()
QString TaskCreateJob::previous | ( | ) | const |
Previous sibling task identifier.
If the task is created at the first position among its siblings, this parameter is omitted.
Definition at line 71 of file taskcreatejob.cpp.
◆ setParentItem()
void TaskCreateJob::setParentItem | ( | const QString & | parentId | ) |
Sets ID of parent task to create new tasks in.
- Parameters
-
parentId
Definition at line 61 of file taskcreatejob.cpp.
◆ setPrevious()
void TaskCreateJob::setPrevious | ( | const QString & | previousId | ) |
Sets previous sibling task identifier.
If the task is created at the first position among its siblings, this parameter is omitted.
- Parameters
-
previousId
Definition at line 76 of file taskcreatejob.cpp.
◆ start()
|
overrideprotectedvirtual |
This method is invoked when job is started.
Job is automatically started when application enters event loop.
Implements KGAPI2::Job.
Definition at line 86 of file taskcreatejob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Fri Dec 27 2024 11:55:30 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.