MessageList::Core::ThemeDelegate
#include <themedelegate.h>
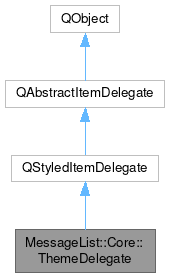
Public Member Functions | |
ThemeDelegate (QAbstractItemView *parent) | |
void | generalFontChanged () |
const Theme::Column * | hitColumn () const |
int | hitColumnIndex () const |
const Theme::ContentItem * | hitContentItem () const |
QRect | hitContentItemRect () const |
bool | hitContentItemRight () const |
const QModelIndex & | hitIndex () const |
Item * | hitItem () const |
QRect | hitItemRect () const |
const Theme::Row * | hitRow () const |
int | hitRowIndex () const |
bool | hitRowIsMessageRow () const |
QRect | hitRowRect () const |
bool | hitTest (const QPoint &viewportPoint, bool exact=true) |
void | setTheme (const Theme *theme) |
QSize | sizeHintForItemTypeAndColumn (Item::Type type, int column, const Item *item=nullptr) const |
const Theme * | theme () const |
![]() | |
QStyledItemDelegate (QObject *parent) | |
virtual QWidget * | createEditor (QWidget *parent, const QStyleOptionViewItem &option, const QModelIndex &index) const const override |
virtual QString | displayText (const QVariant &value, const QLocale &locale) const const |
QItemEditorFactory * | itemEditorFactory () const const |
virtual void | setEditorData (QWidget *editor, const QModelIndex &index) const const override |
void | setItemEditorFactory (QItemEditorFactory *factory) |
virtual void | setModelData (QWidget *editor, QAbstractItemModel *model, const QModelIndex &index) const const override |
virtual void | updateEditorGeometry (QWidget *editor, const QStyleOptionViewItem &option, const QModelIndex &index) const const override |
![]() | |
QAbstractItemDelegate (QObject *parent) | |
void | closeEditor (QWidget *editor, QAbstractItemDelegate::EndEditHint hint) |
void | commitData (QWidget *editor) |
virtual void | destroyEditor (QWidget *editor, const QModelIndex &index) const const |
virtual bool | helpEvent (QHelpEvent *event, QAbstractItemView *view, const QStyleOptionViewItem &option, const QModelIndex &index) |
void | sizeHintChanged (const QModelIndex &index) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
virtual Item * | itemFromIndex (const QModelIndex &index) const =0 |
void | paint (QPainter *painter, const QStyleOptionViewItem &option, const QModelIndex &index) const override |
QSize | sizeHint (const QStyleOptionViewItem &option, const QModelIndex &index) const override |
![]() | |
virtual bool | editorEvent (QEvent *event, QAbstractItemModel *model, const QStyleOptionViewItem &option, const QModelIndex &index) override |
virtual bool | eventFilter (QObject *editor, QEvent *event) override |
virtual void | initStyleOption (QStyleOptionViewItem *option, const QModelIndex &index) const const |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | EndEditHint |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
EditNextItem | |
EditPreviousItem | |
NoHint | |
RevertModelCache | |
SubmitModelCache | |
![]() | |
typedef | QObjectList |
Detailed Description
The ThemeDelegate paints the message list view message and group items by using the supplied Theme.
Definition at line 30 of file themedelegate.h.
Constructor & Destructor Documentation
◆ ThemeDelegate()
|
explicit |
Definition at line 37 of file themedelegate.cpp.
Member Function Documentation
◆ generalFontChanged()
void ThemeDelegate::generalFontChanged | ( | ) |
Called when the global fonts change (from systemsettings)
Definition at line 1879 of file themedelegate.cpp.
◆ hitColumn()
const Theme::Column * ThemeDelegate::hitColumn | ( | ) | const |
Returns the theme column that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitTest() returned true and only within the same calling function.
Definition at line 1755 of file themedelegate.cpp.
◆ hitColumnIndex()
|
nodiscard |
Returns the index of the theme column that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitTest() returned true and only within the same calling function. This is the same as hitIndex().column().
Definition at line 1760 of file themedelegate.cpp.
◆ hitContentItem()
const Theme::ContentItem * ThemeDelegate::hitContentItem | ( | ) | const |
Returns the theme content item that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitTest() returned true and only within the same calling function. This function may also return a null content item when hitTest() returned true. This means that the item was globally hit but no content item was exactly hit (the user might have clicked inside a blank unused space instead).
Definition at line 1785 of file themedelegate.cpp.
◆ hitContentItemRect()
|
nodiscard |
Returns the bounding rect of the content item that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitTest() returned true and only within the same calling function. The result of this function is to be considered invalid also when hitContentItem() returns 0.
Definition at line 1795 of file themedelegate.cpp.
◆ hitContentItemRight()
|
nodiscard |
Returns true if the hit theme content item was a right item and false otherwise.
The result of this function is valid only if hitContentItem() returns true.
Definition at line 1790 of file themedelegate.cpp.
◆ hitIndex()
const QModelIndex & ThemeDelegate::hitIndex | ( | ) | const |
Returns the model index that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitTest() returned true and only within the same calling function.
Definition at line 1740 of file themedelegate.cpp.
◆ hitItem()
Item * ThemeDelegate::hitItem | ( | ) | const |
Returns the Item that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitTest() returned true and only within the same calling function.
Definition at line 1745 of file themedelegate.cpp.
◆ hitItemRect()
|
nodiscard |
Returns the visual rectangle of the item that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitTest() returned true and only within the same calling function. Please note that this rectangle refers to a specific item column (and not all of the columns).
Definition at line 1750 of file themedelegate.cpp.
◆ hitRow()
const Theme::Row * ThemeDelegate::hitRow | ( | ) | const |
Returns the theme row that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitTest() returned true and only within the same calling function. This function may also return a null row when hitTest() returned true. This means that the item was globally hit but no row was exactly hit (the user probably hit the margin instead).
Definition at line 1765 of file themedelegate.cpp.
◆ hitRowIndex()
|
nodiscard |
Returns the index of the theme row that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitRow() returns a non null value.
Definition at line 1770 of file themedelegate.cpp.
◆ hitRowIsMessageRow()
bool ThemeDelegate::hitRowIsMessageRow | ( | ) | const |
Returns true if the hitRow() is a message row, false otherwise.
The result of this function has a meaning only if hitRow() returns a non zero result.
Definition at line 1780 of file themedelegate.cpp.
◆ hitRowRect()
|
nodiscard |
Returns the rectangle of the row that was reported as hit by the previous call to hitTest().
The result of this function is valid only if hitTest() returned true and only within the same calling function. The result of this function is also invalid if hitRow() returns 0.
Definition at line 1775 of file themedelegate.cpp.
◆ hitTest()
bool ThemeDelegate::hitTest | ( | const QPoint & | viewportPoint, |
bool | exact = true ) |
Performs a hit test on the specified viewport point.
Returns true if the point hit something and false otherwise. When the hit test is successful then the hitIndex(), hitItem(), hitColumn(), hitRow(), and hitContentItem() function will return information about the item that was effectively hit. If exact is set to true then hitTest() will return true only if the viewportPoint is exactly over an item. If exact is set to false then the hitTest() function will do its best to find the closest object to be actually "hit": this is useful, for example, in drag and drop operations.
Definition at line 1235 of file themedelegate.cpp.
◆ itemFromIndex()
|
protectedpure virtual |
Returns the Item for the specified model index.
Pure virtual: must be reimplemented by derived classes.
◆ paint()
|
overrideprotectedvirtual |
Reimplemented from QStyledItemDelegate.
Reimplemented from QStyledItemDelegate.
Definition at line 569 of file themedelegate.cpp.
◆ setTheme()
void ThemeDelegate::setTheme | ( | const Theme * | theme | ) |
Definition at line 45 of file themedelegate.cpp.
◆ sizeHint()
|
overrideprotectedvirtual |
Reimplemented from QStyledItemDelegate.
Reimplemented from QStyledItemDelegate.
Definition at line 1850 of file themedelegate.cpp.
◆ sizeHintForItemTypeAndColumn()
|
nodiscard |
Returns a heuristic sizeHint() for the specified item type and column.
The hint is based on the contents of the theme (and not of any message or group header).
Definition at line 1800 of file themedelegate.cpp.
◆ theme()
const Theme * ThemeDelegate::theme | ( | ) | const |
Definition at line 1907 of file themedelegate.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:51:55 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.