MessageList::StorageModel
#include <storagemodel.h>
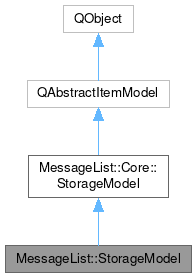
Public Member Functions | |
StorageModel (QAbstractItemModel *model, QItemSelectionModel *selectionModel, QObject *parent=nullptr) | |
Akonadi::Collection | collectionForId (Akonadi::Collection::Id colId) const |
int | columnCount (const QModelIndex &parent=QModelIndex()) const override |
bool | containsOutboundMessages () const override |
QVariant | data (const QModelIndex &index, int role=Qt::DisplayRole) const override |
Akonadi::Collection::List | displayedCollections () const |
void | fillMessageItemThreadingData (MessageList::Core::MessageItem *mi, int row, ThreadingDataSubset subset) const override |
QString | id () const override |
QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const override |
bool | initializeMessageItem (MessageList::Core::MessageItem *mi, int row, bool bUseReceiver) const override |
int | initialUnreadRowCountGuess () const override |
virtual bool | isOutBoundFolder (const Akonadi::Collection &c) const |
Akonadi::Item | itemForRow (int row) const |
KMime::Message::Ptr | messageForRow (int row) const |
QMimeData * | mimeData (const QList< MessageList::Core::MessageItem * > &) const override |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const const |
QModelIndex | parent (const QModelIndex &index) const override |
Akonadi::Collection | parentCollectionForRow (int row) const |
void | resetModelStorage () |
int | rowCount (const QModelIndex &parent=QModelIndex()) const override |
void | setMessageItemStatus (MessageList::Core::MessageItem *mi, int row, Akonadi::MessageStatus status) override |
void | updateMessageItemData (MessageList::Core::MessageItem *mi, int row) const override |
![]() | |
StorageModel (QObject *parent=nullptr) | |
unsigned long | preSelectedMessage () const |
void | savePreSelectedMessage (unsigned long uniqueIdOfMessage) |
![]() | |
QAbstractItemModel (QObject *parent) | |
virtual QModelIndex | buddy (const QModelIndex &index) const const |
virtual bool | canDropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) const const |
virtual bool | canFetchMore (const QModelIndex &parent) const const |
bool | checkIndex (const QModelIndex &index, CheckIndexOptions options) const const |
virtual bool | clearItemData (const QModelIndex &index) |
void | columnsAboutToBeInserted (const QModelIndex &parent, int first, int last) |
void | columnsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | columnsInserted (const QModelIndex &parent, int first, int last) |
void | columnsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationColumn) |
void | columnsRemoved (const QModelIndex &parent, int first, int last) |
void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight, const QList< int > &roles) |
virtual bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) |
virtual void | fetchMore (const QModelIndex &parent) |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const const |
virtual bool | hasChildren (const QModelIndex &parent) const const |
bool | hasIndex (int row, int column, const QModelIndex &parent) const const |
virtual QVariant | headerData (int section, Qt::Orientation orientation, int role) const const |
void | headerDataChanged (Qt::Orientation orientation, int first, int last) |
bool | insertColumn (int column, const QModelIndex &parent) |
virtual bool | insertColumns (int column, int count, const QModelIndex &parent) |
bool | insertRow (int row, const QModelIndex &parent) |
virtual bool | insertRows (int row, int count, const QModelIndex &parent) |
virtual QMap< int, QVariant > | itemData (const QModelIndex &index) const const |
void | layoutAboutToBeChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
void | layoutChanged (const QList< QPersistentModelIndex > &parents, QAbstractItemModel::LayoutChangeHint hint) |
virtual QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits, Qt::MatchFlags flags) const const |
virtual QStringList | mimeTypes () const const |
void | modelAboutToBeReset () |
void | modelReset () |
bool | moveColumn (const QModelIndex &sourceParent, int sourceColumn, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveColumns (const QModelIndex &sourceParent, int sourceColumn, int count, const QModelIndex &destinationParent, int destinationChild) |
bool | moveRow (const QModelIndex &sourceParent, int sourceRow, const QModelIndex &destinationParent, int destinationChild) |
virtual bool | moveRows (const QModelIndex &sourceParent, int sourceRow, int count, const QModelIndex &destinationParent, int destinationChild) |
virtual void | multiData (const QModelIndex &index, QModelRoleDataSpan roleDataSpan) const const |
bool | removeColumn (int column, const QModelIndex &parent) |
virtual bool | removeColumns (int column, int count, const QModelIndex &parent) |
bool | removeRow (int row, const QModelIndex &parent) |
virtual bool | removeRows (int row, int count, const QModelIndex &parent) |
virtual void | revert () |
virtual QHash< int, QByteArray > | roleNames () const const |
void | rowsAboutToBeInserted (const QModelIndex &parent, int start, int end) |
void | rowsAboutToBeMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsAboutToBeRemoved (const QModelIndex &parent, int first, int last) |
void | rowsInserted (const QModelIndex &parent, int first, int last) |
void | rowsMoved (const QModelIndex &sourceParent, int sourceStart, int sourceEnd, const QModelIndex &destinationParent, int destinationRow) |
void | rowsRemoved (const QModelIndex &parent, int first, int last) |
virtual bool | setData (const QModelIndex &index, const QVariant &value, int role) |
virtual bool | setHeaderData (int section, Qt::Orientation orientation, const QVariant &value, int role) |
virtual bool | setItemData (const QModelIndex &index, const QMap< int, QVariant > &roles) |
virtual QModelIndex | sibling (int row, int column, const QModelIndex &index) const const |
virtual void | sort (int column, Qt::SortOrder order) |
virtual QSize | span (const QModelIndex &index) const const |
virtual bool | submit () |
virtual Qt::DropActions | supportedDragActions () const const |
virtual Qt::DropActions | supportedDropActions () const const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Additional Inherited Members | |
![]() | |
enum | ThreadingDataSubset { PerfectThreadingOnly , PerfectThreadingPlusReferences , PerfectThreadingReferencesAndSubject } |
![]() | |
enum | CheckIndexOption |
enum | LayoutChangeHint |
![]() | |
objectName | |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | CheckIndexOptions |
DoNotUseParent | |
HorizontalSortHint | |
IndexIsValid | |
NoLayoutChangeHint | |
NoOption | |
ParentIsInvalid | |
VerticalSortHint | |
![]() | |
typedef | QObjectList |
![]() | |
void | beginInsertColumns (const QModelIndex &parent, int first, int last) |
void | beginInsertRows (const QModelIndex &parent, int first, int last) |
bool | beginMoveColumns (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
bool | beginMoveRows (const QModelIndex &sourceParent, int sourceFirst, int sourceLast, const QModelIndex &destinationParent, int destinationChild) |
void | beginRemoveColumns (const QModelIndex &parent, int first, int last) |
void | beginRemoveRows (const QModelIndex &parent, int first, int last) |
void | beginResetModel () |
void | changePersistentIndex (const QModelIndex &from, const QModelIndex &to) |
void | changePersistentIndexList (const QModelIndexList &from, const QModelIndexList &to) |
QModelIndex | createIndex (int row, int column, const void *ptr) const const |
QModelIndex | createIndex (int row, int column, quintptr id) const const |
void | endInsertColumns () |
void | endInsertRows () |
void | endMoveColumns () |
void | endMoveRows () |
void | endRemoveColumns () |
void | endRemoveRows () |
void | endResetModel () |
QModelIndexList | persistentIndexList () const const |
virtual void | resetInternalData () |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Detailed Description
The Akonadi specific implementation of the Core::StorageModel.
Definition at line 35 of file storagemodel.h.
Constructor & Destructor Documentation
◆ StorageModel()
|
explicit |
Create a StorageModel wrapping the specified folder.
Definition at line 77 of file storagemodel.cpp.
Member Function Documentation
◆ collectionForId()
|
nodiscard |
Definition at line 490 of file storagemodel.cpp.
◆ columnCount()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 376 of file storagemodel.cpp.
◆ containsOutboundMessages()
|
nodiscardoverridevirtual |
Returns true if this StorageModel (folder) contains outbound messages and false otherwise.
Implements MessageList::Core::StorageModel.
Definition at line 169 of file storagemodel.cpp.
◆ data()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 360 of file storagemodel.cpp.
◆ displayedCollections()
|
nodiscard |
Definition at line 128 of file storagemodel.cpp.
◆ fillMessageItemThreadingData()
|
overridevirtual |
This method should use the inner model implementation to fill in the specified subset of threading data for the specified MessageItem from the underlying storage slot at the specified row index.
Implements MessageList::Core::StorageModel.
Definition at line 287 of file storagemodel.cpp.
◆ id()
|
nodiscardoverridevirtual |
Returns an unique id for this Storage collection.
FIXME: This could be embedded in "name()" ?
Implements MessageList::Core::StorageModel.
Definition at line 144 of file storagemodel.cpp.
◆ index()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 384 of file storagemodel.cpp.
◆ initializeMessageItem()
|
nodiscardoverridevirtual |
This method should use the inner model implementation to fill in the base data for the specified MessageItem from the underlying storage slot at the specified row index.
Must return true if the data fetch was successful and false otherwise. For base data we intend: subject, sender, receiver, senderOrReceiver, size, date, encryption state, signature state and status. If bUseReceiver is true then the "senderOrReceiver" field must be set to the receiver, otherwise it must be set to the sender.
Implements MessageList::Core::StorageModel.
Definition at line 199 of file storagemodel.cpp.
◆ initialUnreadRowCountGuess()
|
nodiscardoverridevirtual |
Returns (a guess for) the number of unread messages: must be pessimistic (i.e.
if you have no idea just return rowCount(), which is also what the default implementation does). This must be (and is) queried ONLY when the folder is first opened. It doesn't actually need to keep the number of messages in sync as they later arrive to the storage.
Reimplemented from MessageList::Core::StorageModel.
Definition at line 183 of file storagemodel.cpp.
◆ isOutBoundFolder()
|
nodiscardvirtual |
Definition at line 161 of file storagemodel.cpp.
◆ itemForRow()
|
nodiscard |
Definition at line 460 of file storagemodel.cpp.
◆ messageForRow()
|
nodiscard |
Definition at line 465 of file storagemodel.cpp.
◆ mimeData() [1/2]
|
overridevirtual |
The implementation-specific mime data for this list of items.
Called when the user initiates a drag from the messagelist.
Implements MessageList::Core::StorageModel.
Definition at line 406 of file storagemodel.cpp.
◆ mimeData() [2/2]
|
virtual |
Reimplemented from MessageList::Core::StorageModel.
◆ parent()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 392 of file storagemodel.cpp.
◆ parentCollectionForRow()
|
nodiscard |
Definition at line 470 of file storagemodel.cpp.
◆ resetModelStorage()
void MessageList::StorageModel::resetModelStorage | ( | ) |
Definition at line 508 of file storagemodel.cpp.
◆ rowCount()
|
nodiscardoverridevirtual |
Implements QAbstractItemModel.
Definition at line 398 of file storagemodel.cpp.
◆ setMessageItemStatus()
|
overridevirtual |
This method should use the inner model implementation to associate the new status to the specified message item.
The new status should be stored (but doesn't need to be set as mi->status() itself as the caller is responsible for this).
Implements MessageList::Core::StorageModel.
Definition at line 350 of file storagemodel.cpp.
◆ updateMessageItemData()
|
overridevirtual |
This method should use the inner model implementation to re-fill the date, the status, the encryption state, the signature state and eventually update the min/max dates for the specified MessageItem from the underlying storage slot at the specified row index.
Implements MessageList::Core::StorageModel.
Definition at line 325 of file storagemodel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 11:51:55 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.