KDbCursor
#include <KDbCursor.h>
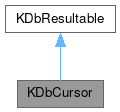
Public Types | |
enum class | Option { None = 0 , Buffered = 1 } |
typedef QFlags< Option > | Options |
Public Member Functions | |
qint64 | at () const |
bool | bof () const |
virtual bool | close () |
KDbConnection * | connection () |
const KDbConnection * | connection () const |
bool | containsRecordIdInfo () const |
bool | deleteAllRecords () |
bool | deleteRecord (KDbRecordData *data, bool useRecordId=false) |
bool | eof () const |
int | fieldCount () const |
bool | insertRecord (KDbRecordData *data, KDbRecordEditBuffer *buf, bool getRecrordId=false) |
bool | isBuffered () const |
bool | isOpened () const |
bool | moveFirst () |
virtual bool | moveLast () |
virtual bool | moveNext () |
virtual bool | movePrev () |
bool | open () |
Options | options () const |
KDbQueryColumnInfo::Vector | orderByColumnList () const |
KDbQuerySchema * | query () const |
QList< QVariant > | queryParameters () const |
KDbEscapedString | rawSql () const |
virtual const char ** | recordData () const =0 |
bool | reopen () |
void | setBuffered (bool buffered) |
void | setOrderByColumnList (const QString &column1, const QString &column2=QString(), const QString &column3=QString(), const QString &column4=QString(), const QString &column5=QString()) |
void | setOrderByColumnList (const QStringList &columnNames) |
void | setQueryParameters (const QList< QVariant > ¶ms) |
KDbRecordData * | storeCurrentRecord () const |
bool | storeCurrentRecord (KDbRecordData *data) const |
bool | updateRecord (KDbRecordData *data, KDbRecordEditBuffer *buf, bool useRecordId=false) |
virtual QVariant | value (int i)=0 |
![]() | |
KDbResultable (const KDbResultable &other) | |
void | clearResult () |
KDbMessageHandler * | messageHandler () const |
KDbResultable & | operator= (const KDbResultable &other) |
KDbResult | result () const |
virtual QString | serverResultName () const |
void | setMessageHandler (KDbMessageHandler *handler) |
void | showMessage () |
Protected Types | |
enum class | FetchResult { Invalid , Error , Ok , End } |
Protected Member Functions | |
KDbCursor (KDbConnection *conn, const KDbEscapedString &sql, Options options=KDbCursor::Option::None) | |
KDbCursor (KDbConnection *conn, KDbQuerySchema *query, Options options=KDbCursor::Option::None) | |
void | clearBuffer () |
virtual void | drv_appendCurrentRecordToBuffer ()=0 |
virtual void | drv_bufferMovePointerNext ()=0 |
virtual void | drv_bufferMovePointerPrev ()=0 |
virtual void | drv_bufferMovePointerTo (qint64 at)=0 |
virtual void | drv_clearBuffer () |
virtual bool | drv_close ()=0 |
virtual void | drv_getNextRecord ()=0 |
virtual bool | drv_open (const KDbEscapedString &sql)=0 |
virtual bool | drv_storeCurrentRecord (KDbRecordData *data) const =0 |
bool | getNextRecord () |
void | init (KDbConnection *conn) |
Protected Attributes | |
bool | m_afterLast |
qint64 | m_at |
bool | m_buffering_completed |
FetchResult | m_fetchResult |
int | m_fieldCount |
int | m_fieldsToStoreInRecord |
int | m_logicalFieldCount |
KDbCursor::Options | m_options |
KDbQuerySchema * | m_query |
int | m_records_in_buf |
KDbQueryColumnInfo::Vector * | m_visibleFieldsExpanded |
![]() | |
Private *const | d |
KDbResult | m_result |
Detailed Description
Provides database cursor functionality.
Cursor can be defined in two ways:
- by passing KDbQuerySchema object to KDbConnection::executeQuery() or KDbConnection::prepareQuery(); then query is defined for in engine-independent way – this is recommended usage
- by passing raw query statement string to KDbConnection::executeQuery() or KDbConnection::prepareQuery(); then query may be defined for in engine-dependent way – this is not recommended usage, but convenient when we can't or do not want to allocate KDbQuerySchema object, while we know that the query statement is syntactically and logically ok in our context.
You can move cursor to next record with moveNext() and move back with movePrev(). The cursor is always positioned on record, not between records, with exception that after open() it is positioned before the first record (if any) – then bof() equals true. The cursor can also be positioned after the last record (if any) with moveNext() – then eof() equals true. For example, if you have four records, 1, 2, 3, 4, then after calling open(), moveNext(), moveNext(), moveNext(), movePrev() you are going through records: 1, 2, 3, 2.
Cursor can be buffered or unbuferred.
- Warning
- Buffered cursors are not implemented!
Buffering in this class is not related to any SQL engine capatibilities for server-side cursors (eg. like 'DECLARE CURSOR' statement) - buffered data is at client (application) side. Any record retrieved in buffered cursor will be stored inside an internal buffer and reused when needed. Unbuffered cursor always requires one record fetching from db connection at every step done with moveNext(), movePrev(), etc.
Notes:
- Do not use delete operator for KDbCursor objects - this will fail; use KDbConnection::deleteCursor() instead.
- KDbQuerySchema object is not owned by KDbCursor object that uses it.
Definition at line 68 of file KDbCursor.h.
Member Typedef Documentation
◆ Options
typedef QFlags< Option > KDbCursor::Options |
Definition at line 77 of file KDbCursor.h.
Member Enumeration Documentation
◆ FetchResult
|
strongprotected |
Possible results of record fetching, used for m_fetchResult.
Enumerator | |
---|---|
Invalid | used before starting the fetching, result is not known yet |
Error | error of fetching |
Ok | the data is fetched |
End | at the end of data |
Definition at line 309 of file KDbCursor.h.
◆ Option
|
strong |
Options that describe behavior of database cursor.
Definition at line 73 of file KDbCursor.h.
Constructor & Destructor Documentation
◆ KDbCursor() [1/2]
|
protected |
Cursor will operate on conn, raw SQL statement sql will be used to execute query.
Definition at line 66 of file KDbCursor.cpp.
◆ KDbCursor() [2/2]
|
protected |
Cursor will operate on conn, query schema will be used to execute query.
Definition at line 78 of file KDbCursor.cpp.
◆ ~KDbCursor()
|
overrideprotected |
Definition at line 123 of file KDbCursor.cpp.
Member Function Documentation
◆ at()
|
inline |
- Returns
- current internal position of the cursor's query. We are counting records from 0. Value -1 means that cursor does not point to any valid record (this happens eg. after open(), close(), and after moving after last record or before first one.
Definition at line 161 of file KDbCursor.h.
◆ bof()
|
inline |
- Returns
- true if current position is before first record.
Definition at line 152 of file KDbCursor.h.
◆ clearBuffer()
|
protected |
clears buffer with reimplemented drv_clearBuffer(). */
Definition at line 413 of file KDbCursor.cpp.
◆ close()
|
virtual |
Closes previously opened cursor. If the cursor is closed, nothing happens.
Definition at line 256 of file KDbCursor.cpp.
◆ connection() [1/2]
KDbConnection * KDbCursor::connection | ( | ) |
- Returns
- connection used for the cursor
Definition at line 148 of file KDbCursor.cpp.
◆ connection() [2/2]
const KDbConnection * KDbCursor::connection | ( | ) | const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Since
- 3.1
Definition at line 153 of file KDbCursor.cpp.
◆ containsRecordIdInfo()
bool KDbCursor::containsRecordIdInfo | ( | ) | const |
- Returns
- true if ROWID information is available for each record. ROWID information is available if KDbDriverBehavior::ROW_ID_FIELD_RETURNS_LAST_AUTOINCREMENTED_VALUE == false for a KDb database driver and the master table has no primary key defined. Phisically, ROWID value is returned after last returned field, so data vector's length is expanded by one.
Definition at line 178 of file KDbCursor.cpp.
◆ deleteAllRecords()
bool KDbCursor::deleteAllRecords | ( | ) |
- Todo
- doesn't update cursor's buffer YET!
Definition at line 526 of file KDbCursor.cpp.
◆ deleteRecord()
bool KDbCursor::deleteRecord | ( | KDbRecordData * | data, |
bool | useRecordId = false ) |
- Todo
- doesn't update cursor's buffer YET!
Definition at line 517 of file KDbCursor.cpp.
◆ drv_appendCurrentRecordToBuffer()
|
protectedpure virtual |
Stores currently fetched record's values in appropriate place of the buffer. Note for driver developers: This place can be computed using m_at. Do not change value of m_at or any other KDbCursor members, only change your internal structures like pointer to current record, etc. If your database engine's API function (for record fetching) do not allocates such a space, you want to allocate a space for current record. Otherwise, reuse existing structure, what could be more efficient. All functions like drv_appendCurrentRecordToBuffer() operates on the buffer, i.e. array of stored records. You are not forced to have any particular fixed structure for buffer item or buffer itself - the structure is internal and only methods like storeCurrentRecord() visible to public.
◆ drv_bufferMovePointerNext()
|
protectedpure virtual |
Moves pointer (that points to the buffer) – to next item in this buffer. Note for driver developers: probably just execute "your_pointer++" is enough.
◆ drv_bufferMovePointerPrev()
|
protectedpure virtual |
Like drv_bufferMovePointerNext() but execute "your_pointer--".
◆ drv_bufferMovePointerTo()
|
protectedpure virtual |
Moves pointer (that points to the buffer) to a new place: at.
◆ drv_clearBuffer()
|
inlineprotectedvirtual |
Clears cursor's buffer if this was allocated (only for buffered cursor type). Otherwise do nothing. For reimplementing. Default implementation does nothing.
Definition at line 285 of file KDbCursor.h.
◆ drv_open()
|
protectedpure virtual |
Note for driver developers: this method should initialize engine-specific cursor's resources using an SQL statement sql. It is not required to store sql statement somewhere in your KDbCursor subclass (it is already stored in m_query or m_rawStatement, depending query type) - only pass it to proper engine's function.
◆ drv_storeCurrentRecord()
|
protectedpure virtual |
Puts current record's data into data (makes a deep copy of each field). This method has unspecified behavior if the cursor is not at valid record.
- Returns
- true on success. Note: For reimplementation in driver's code. Shortly, this method translates a record data from internal representation (probably also used in buffer) to simple public KDbRecordData representation.
◆ eof()
|
inline |
- Returns
- true if current position is after last record.
Definition at line 147 of file KDbCursor.h.
◆ fieldCount()
|
inline |
- Returns
- number of fields available for this cursor. This never includes ROWID column or other internal columns (e.g. lookup).
Definition at line 167 of file KDbCursor.h.
◆ getNextRecord()
|
protected |
Internal: cares about proper flag setting depending on result of drv_getNextRecord() and depending on wherher a cursor is buffered.
Definition at line 424 of file KDbCursor.cpp.
◆ init()
|
protected |
Definition at line 91 of file KDbCursor.cpp.
◆ insertRecord()
bool KDbCursor::insertRecord | ( | KDbRecordData * | data, |
KDbRecordEditBuffer * | buf, | ||
bool | getRecrordId = false ) |
- Todo
- doesn't update cursor's buffer YET!
Definition at line 507 of file KDbCursor.cpp.
◆ isBuffered()
bool KDbCursor::isBuffered | ( | ) | const |
- Returns
- true if the cursor is buffered.
Definition at line 398 of file KDbCursor.cpp.
◆ isOpened()
bool KDbCursor::isOpened | ( | ) | const |
- Returns
- true if the cursor is opened.
Definition at line 173 of file KDbCursor.cpp.
◆ moveFirst()
bool KDbCursor::moveFirst | ( | ) |
Moves current position to the first record and retrieves it.
- Returns
- true if the first record was retrieved. False could mean that there was an error or there is no record available.
Definition at line 285 of file KDbCursor.cpp.
◆ moveLast()
|
virtual |
Moves current position to the last record and retrieves it.
- Returns
- true if the last record was retrieved. False could mean that there was an error or there is no record available.
Definition at line 329 of file KDbCursor.cpp.
◆ moveNext()
|
virtual |
Moves current position to the next record and retrieves it.
Definition at line 351 of file KDbCursor.cpp.
◆ movePrev()
|
virtual |
Moves current position to the next record and retrieves it. Currently it's only supported for buffered cursors.
Definition at line 362 of file KDbCursor.cpp.
◆ open()
bool KDbCursor::open | ( | ) |
Opens the cursor using data provided on creation. The data might be either KDbQuerySchema or a raw SQL statement.
Definition at line 202 of file KDbCursor.cpp.
◆ options()
KDbCursor::Options KDbCursor::options | ( | ) | const |
- Returns
- cursor options
Definition at line 168 of file KDbCursor.cpp.
◆ orderByColumnList()
KDbQueryColumnInfo::Vector KDbCursor::orderByColumnList | ( | ) | const |
- Returns
- a list of fields contained in ORDER BY section of the query.
- See also
- setOrderBy(const QStringList&)
Definition at line 602 of file KDbCursor.cpp.
◆ query()
KDbQuerySchema * KDbCursor::query | ( | ) | const |
- Returns
- query schema used to define this cursor or 0 if the cursor is not defined by a query schema but by a raw SQL statement.
Definition at line 158 of file KDbCursor.cpp.
◆ queryParameters()
- Returns
- query parameters assigned to this cursor
Definition at line 607 of file KDbCursor.cpp.
◆ rawSql()
KDbEscapedString KDbCursor::rawSql | ( | ) | const |
- Returns
- raw query statement used to define this cursor or null string if raw statement instead (but KDbQuerySchema is defined instead).
Definition at line 163 of file KDbCursor.cpp.
◆ recordData()
|
pure virtual |
[PROTOTYPE]
- Returns
- current record data or
nullptr
if there is no current records.
◆ reopen()
bool KDbCursor::reopen | ( | ) |
Closes and then opens again the same cursor. If the cursor is not opened it is just opened and result of this open is returned. Otherwise, true is returned if cursor is successfully closed and then opened.
Definition at line 277 of file KDbCursor.cpp.
◆ setBuffered()
void KDbCursor::setBuffered | ( | bool | buffered | ) |
Sets this cursor to buffered type or not. See description of buffered and nonbuffered cursors in class description. This method only works if cursor is not opened (isOpened()==false). You can close already opened cursor and then switch this option on/off.
Definition at line 403 of file KDbCursor.cpp.
◆ setOrderByColumnList() [1/2]
void KDbCursor::setOrderByColumnList | ( | const QString & | column1, |
const QString & | column2 = QString(), | ||
const QString & | column3 = QString(), | ||
const QString & | column4 = QString(), | ||
const QString & | column5 = QString() ) |
Convenience method, similar to setOrderByColumnList(const QStringList&).
- Todo
- implement this
Convenience method, similar to setOrderBy(const QStringList&).
- Todo
- implement this, like above
- Todo
- add ORDER BY info to debugString()
Definition at line 590 of file KDbCursor.cpp.
◆ setOrderByColumnList() [2/2]
void KDbCursor::setOrderByColumnList | ( | const QStringList & | columnNames | ) |
Sets a list of columns for ORDER BY section of the query. Only works when the cursor has been created using KDbQuerySchema object (i.e. when query()!=0; does not work with raw statements). Each name on the list must be a field or alias present within the query and must not be covered by aliases. If one or more names cannot be found within the query, the method will have no effect. Any previous ORDER BY settings will be removed.
The order list provided here has priority over a list defined in the KDbQuerySchema object itseld (using KDbQuerySchema::setOrderByColumnList()). The KDbQuerySchema object itself is not modifed by this method: only order of records retrieved by this cursor is affected.
Use this method before calling open(). You can also call reopen() after calling this method to see effects of applying records order.
- Todo
- implement this
- Todo
- implement this: all field names should be found, exit otherwise
- Todo
- if (!d->orderByColumnList)
Definition at line 580 of file KDbCursor.cpp.
◆ setQueryParameters()
Sets query parameters params for this cursor.
Definition at line 612 of file KDbCursor.cpp.
◆ storeCurrentRecord() [1/2]
KDbRecordData * KDbCursor::storeCurrentRecord | ( | ) | const |
Allocates a new KDbRecordData and stores data in it (makes a deep copy of each field). If the cursor is not at valid record, the result is undefined.
- Returns
- newly created record data object or 0 on error.
Definition at line 183 of file KDbCursor.cpp.
◆ storeCurrentRecord() [2/2]
bool KDbCursor::storeCurrentRecord | ( | KDbRecordData * | data | ) | const |
Puts current record's data into data (makes a deep copy of each field). If the cursor is not at valid record, the result is undefined.
- Returns
- true on success.
false
is returned if data isnullptr
.
Definition at line 193 of file KDbCursor.cpp.
◆ updateRecord()
bool KDbCursor::updateRecord | ( | KDbRecordData * | data, |
KDbRecordEditBuffer * | buf, | ||
bool | useRecordId = false ) |
- Todo
- doesn't update cursor's buffer YET!
Definition at line 498 of file KDbCursor.cpp.
◆ value()
|
pure virtual |
- Returns
- a value stored in column number i (counting from 0). It has unspecified behavior if the cursor is not at valid record. Note for driver developers: If i is >= than m_fieldCount, null QVariant value should be returned. To return a value typically you can use a pointer to internal structure that contain current record data (buffered or unbuffered).
Member Data Documentation
◆ m_afterLast
|
protected |
Definition at line 299 of file KDbCursor.h.
◆ m_at
|
protected |
Definition at line 300 of file KDbCursor.h.
◆ m_buffering_completed
|
protected |
true if we already have all records stored in the buffer
Definition at line 320 of file KDbCursor.h.
◆ m_fetchResult
|
protected |
result of a record fetching
Definition at line 316 of file KDbCursor.h.
◆ m_fieldCount
|
protected |
cached field count information
Definition at line 301 of file KDbCursor.h.
◆ m_fieldsToStoreInRecord
|
protected |
Used by storeCurrentRecord(), reimplement if needed (e.g.
PostgreSQL driver, when m_containsRecordIdInfo is true sets m_fieldCount+1 here)
Definition at line 302 of file KDbCursor.h.
◆ m_logicalFieldCount
|
protected |
logical field count, i.e. without internal values like Record Id or lookup
Definition at line 305 of file KDbCursor.h.
◆ m_options
|
protected |
cursor options that describes its behavior
Definition at line 306 of file KDbCursor.h.
◆ m_query
|
protected |
Definition at line 298 of file KDbCursor.h.
◆ m_records_in_buf
|
protected |
number of records currently stored in the buffer
Definition at line 319 of file KDbCursor.h.
◆ m_visibleFieldsExpanded
|
protected |
Useful e.g. for value(int) method to obtain access to schema definition.
Definition at line 324 of file KDbCursor.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:54:34 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.