KDbQuerySchema
#include <KDbQuerySchema.h>
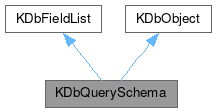
Public Types | |
enum class | ColumnsOrderMode { UnexpandedList , UnexpandedListWithoutAsterisks , ExpandedList } |
enum class | ExpandMode { Unexpanded , Expanded } |
enum class | FieldsExpandedMode { Default , Unique , WithInternalFields , WithInternalFieldsAndRecordId } |
Static Public Member Functions | |
static KDbEscapedString | sqlColumnsList (const KDbQueryColumnInfo::List &infolist, KDbConnection *conn=nullptr, KDb::IdentifierEscapingType escapingType=KDb::DriverEscaping) |
![]() | |
static KDbEscapedString | sqlFieldsList (const KDbField::List &list, KDbConnection *conn, const QString &separator=QLatin1String(","), const QString &tableOrAlias=QString(), KDb::IdentifierEscapingType escapingType=KDb::DriverEscaping) |
Protected Member Functions | |
bool | addAsteriskInternal (KDbQueryAsterisk *asterisk, bool visible) |
bool | addExpressionInternal (const KDbExpression &expr, bool visible) |
KDbQuerySchemaFieldsExpanded * | computeFieldsExpanded (KDbConnection *conn) const |
KDbQueryColumnInfo::Vector | fieldsExpandedInternal (KDbConnection *conn, FieldsExpandedMode mode, bool onlyVisible) const |
bool | insertFieldInternal (int position, KDbField *field, int bindToTable, bool visible) |
void | setWhereExpressionInternal (const KDbExpression &expr) |
Protected Attributes | |
KDbQuerySchemaPrivate *const | d |
Additional Inherited Members | |
![]() | |
QString | caption |
QString | description |
int | id |
QString | name |
int | type |
Detailed Description
KDbQuerySchema provides information about database query.
The query that can be executed using KDb-compatible SQL database engine or used as an introspection tool. KDb parser builds KDbQuerySchema objects by parsing SQL statements.
Definition at line 45 of file KDbQuerySchema.h.
Member Enumeration Documentation
◆ ColumnsOrderMode
|
strong |
Mode for columnsOrder()
- Since
- 3.1
Definition at line 525 of file KDbQuerySchema.h.
◆ ExpandMode
|
strong |
Mode for field() and columnInfo()
- Since
- 3.1
Enumerator | |
---|---|
Unexpanded | All fields are returned even if duplicated. |
Expanded | Expanded list of the query fields is computed so queries with asterisks are processed well. |
Definition at line 386 of file KDbQuerySchema.h.
◆ FieldsExpandedMode
|
strong |
Mode for fieldsExpanded() and visibleFieldsExpanded()
- Since
- 3.1
Definition at line 452 of file KDbQuerySchema.h.
Constructor & Destructor Documentation
◆ KDbQuerySchema() [1/3]
KDbQuerySchema::KDbQuerySchema | ( | ) |
Creates empty query object (without columns).
Definition at line 46 of file KDbQuerySchema.cpp.
◆ KDbQuerySchema() [2/3]
|
explicit |
Creates query schema object that is equivalent to "SELECT * FROM table" sql command. Schema of table is used to contruct this query – it is defined by just adding all the fields to the query in natural order. To avoid problems (e.g. with fields added outside of Kexi using ALTER TABLE) we do not use "all-tables query asterisk" (see KDbQueryAsterisk) item to achieve this effect.
Properties such as the name and caption of the query are inherited from table schema.
We consider that query schema based on table is not (a least yet) stored in a system table, so query connection is set to nullptr
(even if tableSchema's connection is not nullptr
). Id of the created query is set to 0.
Definition at line 53 of file KDbQuerySchema.cpp.
◆ KDbQuerySchema() [3/3]
KDbQuerySchema::KDbQuerySchema | ( | const KDbQuerySchema & | querySchema, |
KDbConnection * | conn ) |
Copy constructor. Creates deep copy of querySchema. KDbQueryAsterisk objects are deeply copied while only pointers to KDbField objects are copied.
Definition at line 79 of file KDbQuerySchema.cpp.
◆ ~KDbQuerySchema()
|
override |
Definition at line 103 of file KDbQuerySchema.cpp.
Member Function Documentation
◆ addAsterisk()
bool KDbQuerySchema::addAsterisk | ( | KDbQueryAsterisk * | asterisk | ) |
Appends asterisk at the and of columns list.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Sets the asterisk as invisible.
- Since
- 3.1
Definition at line 304 of file KDbQuerySchema.cpp.
◆ addAsteriskInternal()
|
protected |
Internal method used by add*Asterisk() methods.
Appends asterisk at the and of columns list, sets visibility.
Definition at line 292 of file KDbQuerySchema.cpp.
◆ addExpression()
bool KDbQuerySchema::addExpression | ( | const KDbExpression & | expr | ) |
Appends a column built on top of expr expression.
This creates a new KDbField object and adds it to the query schema using addField().
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
In addition sets column's visibility to false
.
- Since
- 3.1
Definition at line 271 of file KDbQuerySchema.cpp.
◆ addExpressionInternal()
|
protected |
Internal method used by all add*Expression methods.
Appends expression expr at the and of columns list, sets visibility.
Definition at line 255 of file KDbQuerySchema.cpp.
◆ addField() [1/2]
bool KDbQuerySchema::addField | ( | KDbField * | field | ) |
Appends field to the columns list.
The field will be visible. Use addInvisibleField(field) to add an invisible field. The field is not bound to any particular table within the query.
- See also
- insertField()
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
The field is not bound to any particular table within the query. In addition sets field's visibility to false
. It will not be bound to any table in this query.
- Since
- 3.1
Definition at line 216 of file KDbQuerySchema.cpp.
◆ addField() [2/2]
bool KDbQuerySchema::addField | ( | KDbField * | field, |
int | bindToTable ) |
Appends field to the columns list. Also binds to a table at bindToTable position. Use bindToTable==-1 if no table should be bound. The field will be visible. Use addInvisibleField(field, bindToTable) to add an invisible field.
- See also
- insertField()
- tableBoundToColumn(int columnPosition)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Also binds to a table at bindToTable position. In addition sets field's visibility to false
.
- See also
- tableBoundToColumn(int columnPosition)
- Since
- 3.1
Definition at line 221 of file KDbQuerySchema.cpp.
◆ addInvisibleAsterisk()
bool KDbQuerySchema::addInvisibleAsterisk | ( | KDbQueryAsterisk * | asterisk | ) |
Definition at line 309 of file KDbQuerySchema.cpp.
◆ addInvisibleExpression()
bool KDbQuerySchema::addInvisibleExpression | ( | const KDbExpression & | expr | ) |
Definition at line 276 of file KDbQuerySchema.cpp.
◆ addInvisibleField() [1/2]
bool KDbQuerySchema::addInvisibleField | ( | KDbField * | field | ) |
Definition at line 226 of file KDbQuerySchema.cpp.
◆ addInvisibleField() [2/2]
bool KDbQuerySchema::addInvisibleField | ( | KDbField * | field, |
int | bindToTable ) |
Definition at line 231 of file KDbQuerySchema.cpp.
◆ addRelationship()
Appends a new relationship defined by field1 and field2. Both fields should belong to two different tables of this query. This is convenience function useful for a typical cases. It automatically creates KDbRelationship object for this query. If one of the fields are primary keys, it will be detected and appropriate master-detail relation will be established. This functiuon does nothing if the arguments are invalid.
Definition at line 1198 of file KDbQuerySchema.cpp.
◆ addTable()
void KDbQuerySchema::addTable | ( | KDbTableSchema * | table, |
const QString & | alias = QString() ) |
Appends table schema as one of tables used in a query. If alias is not empty, it will be assigned to this table using setTableAlias(position, alias).
Definition at line 468 of file KDbQuerySchema.cpp.
◆ addToWhereExpression()
bool KDbQuerySchema::addToWhereExpression | ( | KDbField * | field, |
const QVariant & | value, | ||
KDbToken | relation = '=', | ||
QString * | errorMessage = nullptr, | ||
QString * | errorDescription = nullptr ) |
Appends a part to WHERE expression.
Simplifies creating of WHERE expression if used instead of setWhereExpression().
- Returns
false
if the newly constructed WHERE expression is not valid. validate() is called to check this. On failure the WHERE expression for this query is left unchanged. In this case a string pointed by errorMessage (if provided) is set to a general error message and a string pointed by errorDescription (if provided) is set to a detailed description of the error.
- Todo
- date, time
Definition at line 1299 of file KDbQuerySchema.cpp.
◆ asterisks()
KDbField::List * KDbQuerySchema::asterisks | ( | ) | const |
- Returns
- list of KDbQueryAsterisk objects defined for this query. It is never
nullptr
.
Definition at line 669 of file KDbQuerySchema.cpp.
◆ autoIncrementFields()
KDbQueryColumnInfo::List * KDbQuerySchema::autoIncrementFields | ( | KDbConnection * | conn | ) | const |
- Returns
- a list of field infos for all auto-incremented fields from master table of this query. This result is cached for efficiency. fieldsExpanded() is used for that.
Definition at line 1211 of file KDbQuerySchema.cpp.
◆ autoIncrementSqlFieldsList()
KDbEscapedString KDbQuerySchema::autoIncrementSqlFieldsList | ( | KDbConnection * | conn | ) | const |
- Returns
- cached list of autoincrement fields created using sqlColumnsList() on a list returned by autoIncrementFields(). The field names are escaped using driver escaping.
Definition at line 1251 of file KDbQuerySchema.cpp.
◆ clear()
|
overridevirtual |
Removes all columns and their aliases from the columns list, removes all tables and their aliases from the tables list within this query. Sets master table information to nullptr
. Does not destroy any objects though. Clears name and all other properties.
- See also
- KDbFieldList::clear()
Reimplemented from KDbFieldList.
Definition at line 108 of file KDbQuerySchema.cpp.
◆ columnAlias()
QString KDbQuerySchema::columnAlias | ( | int | position | ) | const |
- Returns
- alias of a column at position or empty string if there is no alias for this column or if there is no such column within the query defined. If the column is an expression and has no alias defined, a new unique alias will be generated automatically on this call.
Definition at line 544 of file KDbQuerySchema.cpp.
◆ columnAliasesCount()
int KDbQuerySchema::columnAliasesCount | ( | ) | const |
- Returns
- number of column aliases
Definition at line 539 of file KDbQuerySchema.cpp.
◆ columnInfo()
KDbQueryColumnInfo * KDbQuerySchema::columnInfo | ( | KDbConnection * | conn, |
const QString & | identifier, | ||
ExpandMode | mode = ExpandMode::Expanded ) const |
Like KDbQuerySchema::field(const QString& name) but returns not only KDbField object for identifier but entire KDbQueryColumnInfo object. identifier can be:
- a fieldname
- an aliasname
- a tablename.fieldname
- a tablename.aliasname Note that if there are two occurrrences of the same name, only the first is accessible using this method. For instance, calling columnInfo("name") for "SELECT t1.name, t2.name FROM t1, t2" statement will only return the column related to t1.name and not t2.name, so you'll need to explicitly specify "t2.name" as the identifier to get the second column.
Definition at line 707 of file KDbQuerySchema.cpp.
◆ columnPositionForAlias()
int KDbQuerySchema::columnPositionForAlias | ( | const QString & | name | ) | const |
- Returns
- column position that has defined alias name. If there is no such alias, -1 is returned.
Definition at line 637 of file KDbQuerySchema.cpp.
◆ columnsOrder()
QHash< KDbQueryColumnInfo *, int > KDbQuerySchema::columnsOrder | ( | KDbConnection * | conn, |
ColumnsOrderMode | mode = ColumnsOrderMode::ExpandedList ) const |
- Returns
- a hash for fast lookup of query columns' order.
- If options is UnexpandedList, each KDbQueryColumnInfo pointer is mapped to the index within (unexpanded) list of fields, i.e. "*" or "table.*" asterisks are considered to be single items.
- If options is UnexpandedListWithoutAsterisks, each KDbQueryColumnInfo pointer is mapped to the index within (unexpanded) list of columns that come from asterisks like "*" or "table.*" are not included in the map at all.
- If options is ExpandedList (the default) this method provides is exactly opposite information compared to vector returned by fieldsExpanded().
This method's result is cached by the KDbQuerySchema object. Note: indices of internal fields (see internalFields()) are also returned here - in this case the index is counted as a sum of size(e) + i (where "e" is the list of expanded fields and i is the column index within internal fields list). This feature is used eg. at the end of KDbConnection::updateRecord() where need indices of fields (including internal) to update all the values in memory.
Example use: let t be table (int id, name text, surname text) and q be query defined by a statement "select * from t".
- columnsOrder(ExpandedList) will return the following map: KDbQueryColumnInfo(id)->0, KDbQueryColumnInfo(name)->1, KDbQueryColumnInfo(surname)->2.
- columnsOrder(UnexpandedList) will return the following map: KDbQueryColumnInfo(id)->0, KDbQueryColumnInfo(name)->0, KDbQueryColumnInfo(surname)->0 because the column list is not expanded. This way you can use the returned index to get KDbField* pointer using field(int) method of KDbFieldList superclass.
- columnsOrder(UnexpandedListWithoutAsterisks) will return the following map: KDbQueryColumnInfo(id)->0,
Definition at line 1146 of file KDbQuerySchema.cpp.
◆ computeFieldsExpanded()
|
protected |
- Todo
- (js): perhaps not all fields should be appended here
- Todo
- KDbQuerySchema itself will also support lookup fields...
Definition at line 804 of file KDbQuerySchema.cpp.
◆ contains()
bool KDbQuerySchema::contains | ( | KDbTableSchema * | table | ) | const |
- Returns
true
if the query uses table.
Definition at line 513 of file KDbQuerySchema.cpp.
◆ expandedOrInternalField()
KDbQueryColumnInfo * KDbQuerySchema::expandedOrInternalField | ( | KDbConnection * | conn, |
int | index ) const |
- Returns
- info for expanded of internal field at index index. The returned field can be either logical or internal (for lookup), the latter case is
true
if index >= fieldsExpanded().count(). Equivalent of KDbQuerySchema::fieldsExpanded(WithInternalFields).at(index).
Definition at line 790 of file KDbQuerySchema.cpp.
◆ field() [1/6]
- Returns
- field with name name or
nullptr
if there is no such a field.
Reimplemented from KDbFieldList.
Definition at line 89 of file KDbFieldList.cpp.
◆ field() [2/6]
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Reimplemented from KDbFieldList.
Definition at line 92 of file KDbFieldList.cpp.
◆ field() [3/6]
|
overridevirtual |
Reimplemented from KDbFieldList.
Definition at line 702 of file KDbQuerySchema.cpp.
◆ field() [4/6]
|
overridevirtual |
- Returns
- field id or
nullptr
if there is no such a field.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Reimplemented from KDbFieldList.
Definition at line 697 of file KDbQuerySchema.cpp.
◆ field() [5/6]
KDbField * KDbQuerySchema::field | ( | KDbConnection * | conn, |
const QString & | identifier, | ||
ExpandMode | mode = ExpandMode::Expanded ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 691 of file KDbQuerySchema.cpp.
◆ field() [6/6]
const KDbField * KDbQuerySchema::field | ( | KDbConnection * | conn, |
const QString & | identifier, | ||
ExpandMode | mode = ExpandMode::Expanded ) const |
- Returns
- field for identifier or
nullptr
if no field for this name was found within the query. fieldsExpanded() method is used to lookup expanded list of the query fields, so queries with asterisks are processed well. If a field has alias defined, name is not taken into account, but only its alias. If a field has no alias:- field's name is checked
- field's table and field's name are checked in a form of "tablename.fieldname", so you can provide identifier in this form to avoid ambiguity.
If there are more than one fields with the same name equal to identifier, first-found is returned (checking is performed from first to last query field). Structures needed to compute result of this method are cached, so only first usage costs o(n) - another usages cost o(1).
Example: Let query be defined by "SELECT T.B AS X, T.* FROM T" statement and let T be table containing fields A, B, C. Expanded list of columns for the query is: T.B AS X, T.A, T.B, T.C.
- Calling field("B") will return a pointer to third query column (not the first, because it is covered by "X" alias). Additionally, calling field("X") will return the same pointer.
- Calling field("T.A") will return the same pointer as field("A").
This method is also a product of inheritance from KDbFieldList.
Definition at line 684 of file KDbQuerySchema.cpp.
◆ fieldsExpanded()
|
inline |
- Returns
- fully expanded list of fields. KDbQuerySchema::fields() returns vector of fields used for the query columns, but in a case when there are asterisks defined for the query, it does not expand KDbQueryAsterisk objects to field lists but return every asterisk as-is. This could be inconvenient when you need just a fully expanded list of fields, so this method does the work for you.
If options is Unique, each field is returned in the vector only once (first found field is selected). Note however, that the same field can be returned more than once if it has attached a different alias. For example, let t be TABLE( a, b ) and let query be defined by "SELECT *, a AS alfa FROM t" statement. Both fieldsExpanded(Default) and fieldsExpanded(Unique) will return [ a, b, a (alfa) ] list. On the other hand, for query defined by "SELECT *, a FROM t" statement, fieldsExpanded(Default) will return [ a, b, a ] list while fieldsExpanded(Unique) will return [ a, b ] list.
If options is WithInternalFields or WithInternalFieldsAndRecordID, additional internal fields are also appended to the vector.
If options is WithInternalFieldsAndRecordId, one fake BigInteger column is appended to make space for Record ID column used by KDbCursor implementations. For example, let city_id in TABLE persons(surname, city_id) reference cities.id in TABLE cities(id, name) and let query q be defined by "SELECT * FROM persons" statement. We want to display persons' city names instead of city_id's. To do this, cities.name has to be retrieved as well, so the following statement should be used: "SELECT * FROM persons, cities.name LEFT OUTER JOIN cities ON persons.city_id=cities.id". Thus, calling fieldsExpanded(WithInternalFieldsAndRecordId) will return 4 elements instead of 2: persons.surname, persons.city_id, cities.name, {ROWID}. The {ROWID} item is the placeholder used for fetching ROWID by KDb cursors.
By default, all fields are returned in the vector even if there are multiple occurrences of one or more (options == Default).
Note: You should assign the resulted vector in your space - it will be shared and implicity copied on any modification. This method's result is cached by KDbQuerySchema object.
- Todo
- js: UPDATE CACHE!
Definition at line 501 of file KDbQuerySchema.h.
◆ fieldsExpandedInternal()
|
protected |
Used by fieldsExpanded(KDbConnection*, FieldsExpandedMode) and visibleFieldsExpanded(KDbConnection*, FieldsExpandedMode).
Definition at line 715 of file KDbQuerySchema.cpp.
◆ findTableField()
Convenience function.
- Returns
- table field by searching through all tables in this query. The field does not need to be included on the list of query columns. Similarly, query aliases are not taken into account.
fieldOrTableAndFieldName string may contain table name and field name with '.' character between them, e.g. "mytable.myfield". This is recommended way to avoid ambiguity. 0 is returned if the query has no such table defined of the table has no such field defined. If you do not provide a table name, the first field found is returned.
KDbQuerySchema::table("mytable")->field("myfield") could be alternative for findTableField("mytable.myfield") but it can crash if "mytable" is not defined in the query.
- See also
- KDb::splitToTableAndFieldParts()
Definition at line 518 of file KDbQuerySchema.cpp.
◆ hasColumnAlias() [1/2]
bool KDbQuerySchema::hasColumnAlias | ( | const QString & | name | ) | const |
Provided for convenience.
- Returns
true
if non empty alias name is defined for any column.
Definition at line 642 of file KDbQuerySchema.cpp.
◆ hasColumnAlias() [2/2]
bool KDbQuerySchema::hasColumnAlias | ( | int | position | ) | const |
Provided for convenience.
- Returns
true
if a column at position has non empty alias defined within the query. If there is no alias for this column, or if there is no such column in the query defined,false
is returned.
Definition at line 549 of file KDbQuerySchema.cpp.
◆ hasTableAlias() [1/2]
bool KDbQuerySchema::hasTableAlias | ( | const QString & | name | ) | const |
Provided for convenience.
- Returns
true
if non empty table alias name is defined for a table.
Definition at line 632 of file KDbQuerySchema.cpp.
◆ hasTableAlias() [2/2]
bool KDbQuerySchema::hasTableAlias | ( | int | position | ) | const |
Provided for convenience.
- Returns
true
if a table at position (within FROM section of the query) has non empty alias defined. If there is no alias for this table, or if there is no such table in the query defined,false
is returned.
Definition at line 627 of file KDbQuerySchema.cpp.
◆ insertField() [1/2]
|
overridevirtual |
Inserts field to the columns list at position. Inserted field will not be owned by this KDbQuerySchema object, but by the corresponding KDbTableSchema.
KDbQueryAsterisk can be also passed as field. See the KDbQueryAsterisk class description.
- Note
- After inserting a field, corresponding table will be automatically added to query's tables list if it is not present there (see tables()). KDbField must have its table assigned.
The inserted field will be visible. Use insertInvisibleField(position, field) to add an invisible field.
The field is not bound to any particular table within the query.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
bindToTable is a table index within the query for which the field should be bound. If bindToTable is -1, no particular table will be bound.
- See also
- tableBoundToColumn(int columnPosition)
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
In addition sets field's visibility to false
. It will not be bound to any table in this query.
- Since
- 3.1
Reimplemented from KDbFieldList.
Definition at line 116 of file KDbQuerySchema.cpp.
◆ insertField() [2/2]
bool KDbQuerySchema::insertField | ( | int | position, |
KDbField * | field, | ||
int | bindToTable ) |
Definition at line 126 of file KDbQuerySchema.cpp.
◆ insertFieldInternal()
|
protected |
Internal method used by all insert*Field methods.
The new column can also be explicitly bound to a specific position on tables list. bindToTable is a table index within the query for which the field should be bound. If bindToTable is -1, no particular table will be bound.
- See also
- tableBoundToColumn(int columnPosition)
Definition at line 136 of file KDbQuerySchema.cpp.
◆ insertInvisibleField() [1/2]
bool KDbQuerySchema::insertInvisibleField | ( | int | position, |
KDbField * | field ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
In addition sets field's visibility to false
. bindToTable is a table index within the query for which the field should be bound. If bindToTable is -1, no particular table will be bound.
- See also
- tableBoundToColumn(int columnPosition)
- Since
- 3.1
Definition at line 121 of file KDbQuerySchema.cpp.
◆ insertInvisibleField() [2/2]
bool KDbQuerySchema::insertInvisibleField | ( | int | position, |
KDbField * | field, | ||
int | bindToTable ) |
Definition at line 131 of file KDbQuerySchema.cpp.
◆ internalFields()
KDbQueryColumnInfo::Vector KDbQuerySchema::internalFields | ( | KDbConnection * | conn | ) | const |
- Returns
- list of internal fields used for lookup columns.
Definition at line 784 of file KDbQuerySchema.cpp.
◆ isColumnVisible()
bool KDbQuerySchema::isColumnVisible | ( | int | position | ) | const |
- Returns
- visibility flag for column at position. By default column is visible.
Definition at line 281 of file KDbQuerySchema.cpp.
◆ masterTable()
KDbTableSchema * KDbQuerySchema::masterTable | ( | ) | const |
- Returns
- table that is master to this query. All potentially-editable columns within this query belong just to this table. This method also can return
nullptr
if there are no tables at all, or if previously assigned master table schema has been removed with removeTable(). Every query that has at least one table defined, should have assigned a master table. If no master table is assigned explicitly, but only one table used in this query, a single table is returned here, even if there are table aliases, (e.g. "T" table is returned for "SELECT T1.A, T2.B FROM T T1, T T2" statement).
Definition at line 436 of file KDbQuerySchema.cpp.
◆ orderByColumnList() [1/2]
KDbOrderByColumnList * KDbQuerySchema::orderByColumnList | ( | ) |
- Returns
- a list of columns listed in ORDER BY section of the query. Read notes for setOrderByColumnList().
Definition at line 1366 of file KDbQuerySchema.cpp.
◆ orderByColumnList() [2/2]
const KDbOrderByColumnList * KDbQuerySchema::orderByColumnList | ( | ) | const |
- See also
- orderByColumnList()
Definition at line 1371 of file KDbQuerySchema.cpp.
◆ parameters()
QList< KDbQuerySchemaParameter > KDbQuerySchema::parameters | ( | KDbConnection * | conn | ) | const |
- Returns
- query schema parameters. These are taked from the WHERE section (a tree of expression items).
Definition at line 1376 of file KDbQuerySchema.cpp.
◆ pkeyFieldCount()
int KDbQuerySchema::pkeyFieldCount | ( | KDbConnection * | conn | ) |
- Returns
- number of master table's primary key fields included in this query. This method is useful to quickly check whether the vector returned by pkeyFieldsOrder() if filled completely.
User e.g. in KDbConnection::updateRecord() to check if entire primary key information is specified.
Examples: let table T has (ID1 INTEGER, ID2 INTEGER, A INTEGER) fields, and let (ID1, ID2) is T's primary key.
- The query defined by "SELECT * FROM T" statement contains all T's primary key's fields as T is the master table, and thus pkeyFieldCount() will return 2 (both primary key's fields are in the fieldsExpanded() list), and pkeyFieldsOrder() will return vector {0, 1}.
- The query defined by "SELECT A, ID2 FROM T" statement, and thus pkeyFieldCount() will return 1 (only one primary key's field is in the fieldsExpanded() list), and pkeyFieldsOrder() will return vector {-1, 1}, as second primary key's field is at position #1 and first field is not specified at all within the query.
Definition at line 1192 of file KDbQuerySchema.cpp.
◆ pkeyFieldsOrder()
QVector< int > KDbQuerySchema::pkeyFieldsOrder | ( | KDbConnection * | conn | ) | const |
- Returns
- table describing order of primary key (PKEY) fields within the query. Indexing is performed against vector returned by fieldsExpanded(). It is usable for e.g. Connection::updateRecord(), when we need to locate each primary key's field in a constant time.
Returned vector is owned and cached by KDbQuerySchema object. When you assign it, it is implicity shared. Its size is equal to number of primary key fields defined for master table (masterTable()->primaryKey()->fieldCount()).
Each element of the returned vector:
- can belong to [0..fieldsExpanded().count()-1] if there is such primary key's field in the fieldsExpanded() list.
- can be equal to -1 if there is no such primary key's field in the fieldsExpanded() list.
If there are more than one primary key's field included in the query, only first-found column (oin the fieldsExpanded() list) for each pkey's field is included.
Returns empty vector if there is no master table or no master table's pkey.
- See also
- example for pkeyFieldCount().
- Todo
- js: UPDATE CACHE!
Definition at line 1158 of file KDbQuerySchema.cpp.
◆ relationships()
QList< KDbRelationship * > * KDbQuerySchema::relationships | ( | ) | const |
- Returns
- a list of relationships defined for this query. It is never
nullptr
.
Definition at line 664 of file KDbQuerySchema.cpp.
◆ removeField()
|
overridevirtual |
Removes field from the columns list. Use with care.
- Todo
- should we also remove table for this field or asterisk?
Reimplemented from KDbFieldList.
Definition at line 236 of file KDbQuerySchema.cpp.
◆ removeTable()
void KDbQuerySchema::removeTable | ( | KDbTableSchema * | table | ) |
Removes table schema from this query. This does not destroy table object but only takes it out of the list. If this table was master for the query, master table information is also invalidated.
- Todo
- remove fields!
Definition at line 492 of file KDbQuerySchema.cpp.
◆ setColumnAlias()
bool KDbQuerySchema::setColumnAlias | ( | int | position, |
const QString & | alias ) |
Sets alias for a column at position, within the query. Passing empty string to alias clears alias for a given column.
Definition at line 554 of file KDbQuerySchema.cpp.
◆ setColumnVisible()
void KDbQuerySchema::setColumnVisible | ( | int | position, |
bool | visible ) |
Sets visibility flag for column at position to visible.
Definition at line 286 of file KDbQuerySchema.cpp.
◆ setMasterTable()
void KDbQuerySchema::setMasterTable | ( | KDbTableSchema * | table | ) |
Sets master table of this query to table. This table should be also added to query's tables list using addTable(). If table equals nullptr
, nothing is performed.
- See also
- masterTable()
Definition at line 457 of file KDbQuerySchema.cpp.
◆ setOrderByColumnList()
void KDbQuerySchema::setOrderByColumnList | ( | const KDbOrderByColumnList & | list | ) |
Sets a list of columns for ORDER BY section of the query. Each name on the list must be a field or alias present within the query and must not be covered by aliases. If one or more names cannot be found within the query, the method will have no effect. Any previous ORDER BY settings will be removed.
Note that this information is cleared whenever you call methods that modify list of columns (KDbQueryColumnInfo), i.e. insertField(), addField(), removeField(), addExpression(), etc. (because KDbOrderByColumn items can point to a KDbQueryColumnInfo that's removed by these methods), so you should use setOrderByColumnList() method after the query is completely built.
Definition at line 1359 of file KDbQuerySchema.cpp.
◆ setStatement()
void KDbQuerySchema::setStatement | ( | const KDbEscapedString & | sql | ) |
Forces a raw SQL statement sql for the query. This means that no statement is composed from KDbQuerySchema's content.
Definition at line 679 of file KDbQuerySchema.cpp.
◆ setTableAlias()
bool KDbQuerySchema::setTableAlias | ( | int | position, |
const QString & | alias ) |
Sets alias for a table at position (within FROM section of the query). Passing empty sting to alias clears alias for a given table (only for specified position).
Definition at line 647 of file KDbQuerySchema.cpp.
◆ setWhereExpression()
bool KDbQuerySchema::setWhereExpression | ( | const KDbExpression & | expr, |
QString * | errorMessage = nullptr, | ||
QString * | errorDescription = nullptr ) |
Sets a WHERE expression exp.
Previously set WHERE expression will be removed. A null expression (KDbExpression()) can be passed to remove existing WHERE expresssion.
- Returns
false
if expr is not a valid WHERE expression. validate() is called to check this. On failure the WHERE expression for this query is cleared. In this case a string pointed by errorMessage (if provided) is set to a general error message and a string pointed by errorDescription (if provided) is set to a detailed description of the error.
Definition at line 1275 of file KDbQuerySchema.cpp.
◆ setWhereExpressionInternal()
|
protected |
Internal method used by a query parser.
◆ sqlColumnsList()
|
static |
- Returns
- a string that is a result of concatenating all column names for infolist, with "," between each one. This is usable e.g. as argument like "field1,field2" for "INSERT INTO (xxx) ..". The result of this method is effectively cached, and it is invalidated when set of fields changes (e.g. using clear() or addField()).
This method is similar to KDbFieldList::sqlFieldsList() it just uses KDbQueryColumnInfo::List instead of KDbField::List.
escapingType can be used to alter default escaping type. If conn is not provided for DriverEscaping, no escaping is performed.
Definition at line 1234 of file KDbQuerySchema.cpp.
◆ statement()
KDbEscapedString KDbQuerySchema::statement | ( | ) | const |
- Returns
- a preset statement (if any).
Definition at line 674 of file KDbQuerySchema.cpp.
◆ table()
KDbTableSchema * KDbQuerySchema::table | ( | const QString & | tableName | ) | const |
- Returns
- table with name tableName or 0 if this query has no such table.
- Todo
- maybe use tables_byname?
Definition at line 502 of file KDbQuerySchema.cpp.
◆ tableAlias() [1/2]
- Returns
- alias of a table tableName (within FROM section) or empty value if there is no alias for this table or if there is no such table within the query defined.
Definition at line 579 of file KDbQuerySchema.cpp.
◆ tableAlias() [2/2]
QString KDbQuerySchema::tableAlias | ( | int | position | ) | const |
- Returns
- alias of a table at position (within FROM section) or null string if there is no alias for this table or if there is no such table within the query defined.
Definition at line 574 of file KDbQuerySchema.cpp.
◆ tableAliasesCount()
int KDbQuerySchema::tableAliasesCount | ( | ) | const |
- Returns
- number of table aliases
Definition at line 569 of file KDbQuerySchema.cpp.
◆ tableAliasOrName()
- Returns
- alias of a table tableName (within FROM section). If there is no alias for this table, its name is returned. Empty value is returned if there is no such table within the query defined.
Definition at line 588 of file KDbQuerySchema.cpp.
◆ tableBoundToColumn()
int KDbQuerySchema::tableBoundToColumn | ( | int | columnPosition | ) | const |
- Returns
- a table position (within FROM section), that is bound to column at columnPosition (within SELECT section). This information can be used to find if there is alias defined for a table that is referenced by a given column.
For example, for "SELECT t2.id FROM table1 t1, table2 t2" query statement, columnBoundToTable(0) returns 1, what means that table at position 1 (within FROM section) is bound to column at position 0, so we can now call tableAlias(1) to see if we have used alias for this column (t2.id) or just a table name (table2.id).
These checks are performed e.g. by KDbConnection::selectStatement() to construct a statement string maximally identical to originally defined query statement.
-1 is returned if:
- columnPosition is out of range (i.e. < 0 or >= fieldCount())
- a column at columnPosition is not bound to any table (i.e. no database field is used for this column, e.g. "1" constant for "SELECT 1 from table" query statement)
Definition at line 206 of file KDbQuerySchema.cpp.
◆ tablePosition()
int KDbQuerySchema::tablePosition | ( | const QString & | tableName | ) | const |
- Returns
- position (within the FROM section) of table tableName. -1 is returned if there's no such table declared in the FROM section.
- See also
- tablePositions()
Definition at line 602 of file KDbQuerySchema.cpp.
◆ tablePositionForAlias()
int KDbQuerySchema::tablePositionForAlias | ( | const QString & | name | ) | const |
- Returns
- table position (within FROM section) that has attached alias name. If there is no such alias, -1 is returned. Only first table's position attached for this alias is returned. It is not especially bad, since aliases rarely can be duplicated, what leads to ambiguity. Duplicated aliases are only allowed for trivial queries that have no database fields used within their columns, e.g. "SELECT 1 from table1 t, table2 t" is ok but "SELECT t.id from table1 t, table2 t" is not.
Definition at line 597 of file KDbQuerySchema.cpp.
◆ tablePositions()
- Returns
- a list of all occurrences of table tableName (within the FROM section). E.g. for "SELECT * FROM table t, table t2" tablePositions("table") returns {0, 1} list. Empty list is returned if there's no table tableName used in the FROM section at all.
- See also
- tablePosition()
Definition at line 614 of file KDbQuerySchema.cpp.
◆ tables()
QList< KDbTableSchema * > * KDbQuerySchema::tables | ( | ) | const |
- Returns
- list of tables used in this query. It is never
nullptr
. The list also includes master table.
- See also
- masterTable()
Definition at line 463 of file KDbQuerySchema.cpp.
◆ validate()
bool KDbQuerySchema::validate | ( | QString * | errorMessage = nullptr, |
QString * | errorDescription = nullptr ) |
- Returns
true
if this query is valid
Detailed validation is performed in the same way as parsing of query statements by the KDbParser. Example :Let the query be "SELECT <fields> FROM <tables> WHERE <whereExpression>". First each field from <fields> (
- See also
- fields()) is validated using KDbField::expression().validate(). Then the <whereExpression> (
- whereExpression()) is validated using KDbExpression::validate().
On error a string pointed by errorMessage (if provided) is set to a general error message and a string pointed by errorDescription (if provided) is set to a detailed description of the error.
- Todo
- add tests
Definition at line 1393 of file KDbQuerySchema.cpp.
◆ visibleFieldsExpanded()
|
inline |
Like fieldsExpanded() but returns only visible fields.
Definition at line 508 of file KDbQuerySchema.h.
◆ whereExpression()
KDbExpression KDbQuerySchema::whereExpression | ( | ) | const |
- Returns
- WHERE expression or 0 if this query has no WHERE expression
Definition at line 1354 of file KDbQuerySchema.cpp.
Member Data Documentation
◆ d
|
protected |
Definition at line 748 of file KDbQuerySchema.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.