KDbExpression
#include <KDbExpression.h>
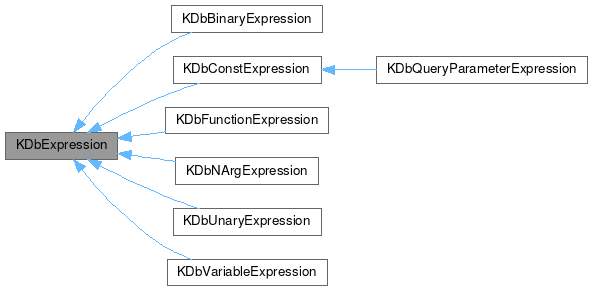
Static Public Member Functions | |
static KDb::ExpressionClass | classForToken (KDbToken token) |
Protected Member Functions | |
KDbExpression (const ExplicitlySharedExpressionDataPointer &ptr) | |
KDbExpression (KDbExpressionData *data) | |
KDbExpression (KDbExpressionData *data, KDb::ExpressionClass aClass, KDbToken token) | |
void | appendChild (const ExplicitlySharedExpressionDataPointer &child) |
void | appendChild (const KDbExpression &child) |
bool | checkBeforeInsert (const ExplicitlySharedExpressionDataPointer &child) |
QList< ExplicitlySharedExpressionDataPointer > | children () const |
int | indexOfChild (const KDbExpression &child, int from=0) const |
void | insertChild (int i, const KDbExpression &child) |
void | insertEmptyChild (int i) |
int | lastIndexOfChild (const KDbExpression &child, int from=-1) const |
void | prependChild (const KDbExpression &child) |
bool | removeChild (const KDbExpression &child) |
void | removeChild (int i) |
void | setLeftOrRight (const KDbExpression &right, int index) |
KDbExpression | takeChild (int i) |
Protected Attributes | |
ExplicitlySharedExpressionDataPointer | d |
Detailed Description
The KDbExpression class represents a base class for all expressions.
Definition at line 51 of file KDbExpression.h.
Constructor & Destructor Documentation
◆ KDbExpression() [1/4]
KDbExpression::KDbExpression | ( | ) |
Constructs a null expression.
- See also
- KDbExpression::isNull()
Definition at line 263 of file KDbExpression.cpp.
◆ ~KDbExpression()
|
virtual |
Definition at line 287 of file KDbExpression.cpp.
◆ KDbExpression() [2/4]
|
explicitprotected |
Definition at line 276 of file KDbExpression.cpp.
◆ KDbExpression() [3/4]
|
protected |
Definition at line 269 of file KDbExpression.cpp.
◆ KDbExpression() [4/4]
|
explicitprotected |
Definition at line 282 of file KDbExpression.cpp.
Member Function Documentation
◆ appendChild() [1/2]
|
protected |
Definition at line 461 of file KDbExpression.cpp.
◆ appendChild() [2/2]
|
protected |
Definition at line 375 of file KDbExpression.cpp.
◆ checkBeforeInsert()
|
protected |
Definition at line 448 of file KDbExpression.cpp.
◆ children()
|
protected |
- Returns
- the list of children expressions.
Definition at line 370 of file KDbExpression.cpp.
◆ classForToken()
|
static |
- Returns
- expression class for token token.
- Todo
- support more tokens
Definition at line 596 of file KDbExpression.cpp.
◆ clone()
KDbExpression KDbExpression::clone | ( | ) | const |
Creates a deep (not shallow) copy of the KDbExpression.
Definition at line 300 of file KDbExpression.cpp.
◆ debug()
QDebug KDbExpression::debug | ( | QDebug | dbg, |
KDb::ExpressionCallStack * | callStack ) const |
Definition at line 484 of file KDbExpression.cpp.
◆ expressionClass()
KDb::ExpressionClass KDbExpression::expressionClass | ( | ) | const |
- Returns
- class identifier of this expression. Default expressionClass is KDb::UnknownExpression.
Definition at line 315 of file KDbExpression.cpp.
◆ getQueryParameters()
void KDbExpression::getQueryParameters | ( | QList< KDbQuerySchemaParameter > * | params | ) |
Collects query parameters (messages and types) recursively and saves them to params. The leaf nodes are objects of QueryParameterExpr class.
- Note
- params must not be 0.
Definition at line 478 of file KDbExpression.cpp.
◆ indexOfChild()
|
protected |
Definition at line 438 of file KDbExpression.cpp.
◆ insertChild()
|
protected |
Definition at line 388 of file KDbExpression.cpp.
◆ insertEmptyChild()
|
protected |
Used for inserting placeholders, e.g. in KDbBinaryExpression::KDbBinaryExpression()
Definition at line 398 of file KDbExpression.cpp.
◆ isBinary()
bool KDbExpression::isBinary | ( | ) | const |
Definition at line 511 of file KDbExpression.cpp.
◆ isConst()
bool KDbExpression::isConst | ( | ) | const |
Definition at line 516 of file KDbExpression.cpp.
◆ isDateTimeType()
bool KDbExpression::isDateTimeType | ( | ) | const |
- Returns
- true if type of this object belong to a group of time, date and date/time types.
A covenience method.
- See also
- type()
Definition at line 360 of file KDbExpression.cpp.
◆ isFPNumericType()
bool KDbExpression::isFPNumericType | ( | ) | const |
- Returns
- true if type of this object belong to a group of floating-point numeric types.
A covenience method.
- See also
- type()
Definition at line 355 of file KDbExpression.cpp.
◆ isFunction()
bool KDbExpression::isFunction | ( | ) | const |
Definition at line 526 of file KDbExpression.cpp.
◆ isIntegerType()
bool KDbExpression::isIntegerType | ( | ) | const |
- Returns
- true if type of this object belong to a group of integer types.
A covenience method.
- See also
- type()
Definition at line 345 of file KDbExpression.cpp.
◆ isNArg()
bool KDbExpression::isNArg | ( | ) | const |
Definition at line 501 of file KDbExpression.cpp.
◆ isNull()
bool KDbExpression::isNull | ( | ) | const |
- Returns
- true if this expression is null. Equivalent of expressionClass() == KDb::UnknownExpression.
- Note
- Returns false for expressions of type KDbField::Null (SQL's NULL).
Definition at line 295 of file KDbExpression.cpp.
◆ isNumericType()
bool KDbExpression::isNumericType | ( | ) | const |
- Returns
- true if type of this object belong to a group of numeric types.
A covenience method.
- See also
- type()
Definition at line 350 of file KDbExpression.cpp.
◆ isQueryParameter()
bool KDbExpression::isQueryParameter | ( | ) | const |
Definition at line 531 of file KDbExpression.cpp.
◆ isTextType()
bool KDbExpression::isTextType | ( | ) | const |
- Returns
- true if type of this object belong to a group of text types.
A covenience method.
- See also
- type()
Definition at line 340 of file KDbExpression.cpp.
◆ isUnary()
bool KDbExpression::isUnary | ( | ) | const |
Definition at line 506 of file KDbExpression.cpp.
◆ isValid()
bool KDbExpression::isValid | ( | ) | const |
- Returns
- true if type of this object is not KDbField::InvalidType.
A covenience method.
- See also
- type()
Definition at line 335 of file KDbExpression.cpp.
◆ isVariable()
bool KDbExpression::isVariable | ( | ) | const |
Definition at line 521 of file KDbExpression.cpp.
◆ lastIndexOfChild()
|
protected |
Definition at line 443 of file KDbExpression.cpp.
◆ operator!=()
bool KDbExpression::operator!= | ( | const KDbExpression & | e | ) | const |
Definition at line 496 of file KDbExpression.cpp.
◆ operator==()
bool KDbExpression::operator== | ( | const KDbExpression & | e | ) | const |
Definition at line 491 of file KDbExpression.cpp.
◆ parent()
KDbExpression KDbExpression::parent | ( | ) | const |
- Returns
- the parent expression.
Definition at line 365 of file KDbExpression.cpp.
◆ prependChild()
|
protected |
Definition at line 380 of file KDbExpression.cpp.
◆ removeChild() [1/2]
|
protected |
Definition at line 407 of file KDbExpression.cpp.
◆ removeChild() [2/2]
|
protected |
Definition at line 415 of file KDbExpression.cpp.
◆ setExpressionClass()
void KDbExpression::setExpressionClass | ( | KDb::ExpressionClass | aClass | ) |
Sets expression class aClass for this expression.
Definition at line 320 of file KDbExpression.cpp.
◆ setLeftOrRight()
|
protected |
Only for KDbBinaryExpression::setLeft() and KDbBinaryExpression::setRight()
Definition at line 574 of file KDbExpression.cpp.
◆ setToken()
void KDbExpression::setToken | ( | KDbToken | token | ) |
Sets token token for this expression.
Definition at line 310 of file KDbExpression.cpp.
◆ takeChild()
|
protected |
Definition at line 425 of file KDbExpression.cpp.
◆ toBinary()
KDbBinaryExpression KDbExpression::toBinary | ( | ) | const |
Definition at line 549 of file KDbExpression.cpp.
◆ toConst()
KDbConstExpression KDbExpression::toConst | ( | ) | const |
Definition at line 554 of file KDbExpression.cpp.
◆ toFunction()
KDbFunctionExpression KDbExpression::toFunction | ( | ) | const |
Definition at line 569 of file KDbExpression.cpp.
◆ token()
KDbToken KDbExpression::token | ( | ) | const |
- Returns
- the token for this expression. Tokens are characters (e.g. '+', '-') or identifiers (e.g. SQL_NULL) of elements used by the KDbSQL parser. By default token is 0.
Definition at line 305 of file KDbExpression.cpp.
◆ toNArg()
KDbNArgExpression KDbExpression::toNArg | ( | ) | const |
Convenience type casts.
Definition at line 539 of file KDbExpression.cpp.
◆ toQueryParameter()
KDbQueryParameterExpression KDbExpression::toQueryParameter | ( | ) | const |
Definition at line 559 of file KDbExpression.cpp.
◆ toString()
KDbEscapedString KDbExpression::toString | ( | const KDbDriver * | driver, |
KDbQuerySchemaParameterValueListIterator * | params = nullptr, | ||
KDb::ExpressionCallStack * | callStack = nullptr ) const |
- Returns
- string as a representation of this expression element by running recursive calls. param, if not 0, points to a list item containing value of a query parameter (used in QueryParameterExpr). The result may depend on the optional driver parameter. If driver is 0, representation for portable KDbSQL dialect is returned.
Definition at line 469 of file KDbExpression.cpp.
◆ toUnary()
KDbUnaryExpression KDbExpression::toUnary | ( | ) | const |
Definition at line 544 of file KDbExpression.cpp.
◆ toVariable()
KDbVariableExpression KDbExpression::toVariable | ( | ) | const |
Definition at line 564 of file KDbExpression.cpp.
◆ type()
KDbField::Type KDbExpression::type | ( | ) | const |
- Returns
- type of this expression, based on effect of its evaluation. Default type is KDbField::InvalidType.
- See also
- isValid()
Definition at line 330 of file KDbExpression.cpp.
◆ validate()
bool KDbExpression::validate | ( | KDbParseInfo * | parseInfo | ) |
- Returns
- true if evaluation of this expression succeeded.
Definition at line 325 of file KDbExpression.cpp.
Member Data Documentation
◆ d
|
protected |
Definition at line 200 of file KDbExpression.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Apr 18 2025 12:15:51 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.