KDbField
#include <KDbField.h>
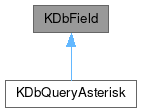
Public Types | |
enum | Constraint { NoConstraints = 0 , AutoInc = 1 , Unique = 2 , PrimaryKey = 4 , ForeignKey = 8 , NotNull = 16 , NotEmpty = 32 , Indexed = 64 } |
typedef QFlags< Constraint > | Constraints |
typedef QHash< QByteArray, QVariant > | CustomPropertiesMap |
typedef KDbUtils::AutodeletedList< KDbField * > | List |
typedef QList< KDbField * >::ConstIterator | ListIterator |
enum | MaxLengthStrategy { DefaultMaxLength , DefinedMaxLength } |
enum | Option { NoOptions = 0 , Unsigned = 1 } |
typedef QFlags< Option > | Options |
typedef QPair< KDbField *, KDbField * > | Pair |
typedef QList< Pair > | PairList |
enum | Type { InvalidType = 0 , Byte = 1 , FirstType = 1 , ShortInteger = 2 , Integer = 3 , BigInteger = 4 , Boolean = 5 , Date = 6 , DateTime = 7 , Time = 8 , Float = 9 , Double = 10 , Text = 11 , LongText = 12 , BLOB = 13 , LastType = 13 , Null = 128 , Asterisk = 129 , Enum = 130 , Map = 131 , Tuple = 132 , LastSpecialType = Tuple } |
enum | TypeGroup { InvalidGroup = 0 , TextGroup = 1 , IntegerGroup = 2 , FloatGroup = 3 , BooleanGroup = 4 , DateTimeGroup = 5 , BLOBGroup = 6 , LastTypeGroup = 6 } |
typedef QVector< KDbField * > | Vector |
Static Public Member Functions | |
static QVariant | convertToType (const QVariant &value, Type type) |
static int | defaultMaxLength () |
static bool | hasEmptyProperty (Type type) |
static bool | isAutoIncrementAllowed (Type type) |
static bool | isDateTimeType (Type type) |
static bool | isFPNumericType (Type type) |
static bool | isIntegerType (Type type) |
static bool | isNumericType (Type type) |
static bool | isTextType (Type type) |
static void | setDefaultMaxLength (int maxLength) |
static int | specialTypesCount () |
static Type | typeForString (const QString &typeString) |
static TypeGroup | typeGroup (Type type) |
static TypeGroup | typeGroupForString (const QString &typeGroupString) |
static QString | typeGroupName (TypeGroup typeGroup) |
static QStringList | typeGroupNames () |
static int | typeGroupsCount () |
static QString | typeGroupString (TypeGroup typeGroup) |
static QString | typeName (Type type) |
static QStringList | typeNames () |
static int | typesCount () |
static QString | typeString (Type type) |
static QVariant::Type | variantType (Type type) |
Protected Member Functions | |
KDbField (KDbFieldList *aParent, int aOrder=-1) | |
KDbField (KDbQuerySchema *querySchema) | |
KDbField (KDbQuerySchema *querySchema, const KDbExpression &expr) | |
virtual KDbField * | copy () |
void | setOrder (int order) |
void | setParent (KDbFieldList *parent) |
Detailed Description
Meta-data for a field.
KDbField provides information about single database field.
KDbField class has defined following members:
- name
- type
- database constraints
- additional options
- maxLength (makes sense mostly for string types)
- maxLengthStrategy (makes sense mostly for string types)
- precision (for floating-point type)
- defaultValue
- caption (user readable name that can be e.g. translated)
- description (user readable name additional text, can be useful for developers)
- defaultWidth (a hint for displaying in tabular mode or as text box)
KDbField can also have assigned expression (see KDbExpression class, and expression() method).
Note that aliases for fields are defined within query, not in KDbField object, because the same field can be used in different queries with different alias.
Notes for advanced use: KDbField obeject is designed to be owned by a parent object. Such a parent object can be KDbTableSchema, if the field defines single table column, or KDbQuerySchema, if the field defines an expression (KDbExpression class).
Using expression class for fields allos to define expressions within queries like "SELECT AVG(price) FROM products"
You can choose whether your field is owned by query or table, using appropriate constructor, or using parameterless constructor and calling setTable() or setQuery() later.
Definition at line 71 of file KDbField.h.
Member Typedef Documentation
◆ Constraints
typedef QFlags< Constraint > KDbField::Constraints |
Definition at line 142 of file KDbField.h.
◆ CustomPropertiesMap
typedef QHash<QByteArray, QVariant> KDbField::CustomPropertiesMap |
A data type used for handling custom properties of a field.
Definition at line 688 of file KDbField.h.
◆ List
typedef KDbUtils::AutodeletedList<KDbField*> KDbField::List |
list of fields
Definition at line 77 of file KDbField.h.
◆ ListIterator
iterator for list of fields
Definition at line 79 of file KDbField.h.
◆ Options
typedef QFlags< Option > KDbField::Options |
Definition at line 149 of file KDbField.h.
◆ Pair
typedef QPair<KDbField*, KDbField*> KDbField::Pair |
fields pair
Definition at line 80 of file KDbField.h.
◆ PairList
typedef QList<Pair> KDbField::PairList |
list of fields pair
Definition at line 81 of file KDbField.h.
◆ Vector
typedef QVector<KDbField*> KDbField::Vector |
vector of fields
Definition at line 78 of file KDbField.h.
Member Enumeration Documentation
◆ Constraint
enum KDbField::Constraint |
Possible constraints defined for a field.
Enumerator | |
---|---|
NotEmpty | only legal for string-like and blob fields |
Definition at line 132 of file KDbField.h.
◆ MaxLengthStrategy
Strategy for defining maximum length of text for this field. Only makes sense if the field type is of Text type. Default strategy is DefinedMaxLength.
Enumerator | |
---|---|
DefaultMaxLength | Default maximum text length defined globally by the application.
|
DefinedMaxLength | Used if setMaxLength() was called to set specific maximum value or to unlimited (0). |
Definition at line 429 of file KDbField.h.
◆ Option
enum KDbField::Option |
Possible options defined for a field.
Definition at line 145 of file KDbField.h.
◆ Type
enum KDbField::Type |
Unified (most common used) types of fields.
Enumerator | |
---|---|
InvalidType | Unsupported/Unimplemented type |
Byte | 1 byte, signed or unsigned |
ShortInteger | First type 2 bytes, signed or unsigned |
Integer | 4 bytes, signed or unsigned |
BigInteger | 8 bytes, signed or unsigned |
Boolean | 0 or 1 |
Float | 4 bytes |
Double | 8 bytes |
Text | Other name: Varchar |
LongText | Other name: Memo |
BLOB | Large binary object |
LastType | This line should be at the end of the list of types! |
Null | Used for fields that are "NULL" expressions. |
Asterisk | Used in KDbQueryAsterisk subclass objects only, not used in table definitions, but only in query definitions |
Enum | An integer internal with a string list of hints |
Map | Mapping from string to string list (more generic than Enum) |
Tuple | A list of values (e.g. arguments of a function) |
LastSpecialType | This line should be at the end of the list of special types! |
Definition at line 84 of file KDbField.h.
◆ TypeGroup
enum KDbField::TypeGroup |
Type groups for fields.
Definition at line 118 of file KDbField.h.
Constructor & Destructor Documentation
◆ KDbField() [1/7]
|
explicit |
Creates a database field as a child of tableSchema table. No other properties are set (even the name), so these should be set later.
Definition at line 205 of file KDbField.cpp.
◆ KDbField() [2/7]
KDbField::KDbField | ( | ) |
Creates a database field. maxLength property is set to 0 (unlimited length). No other properties are set (even the name), so these should be set later.
Definition at line 189 of file KDbField.cpp.
◆ KDbField() [3/7]
KDbField::KDbField | ( | const QString & | name, |
Type | type, | ||
Constraints | constr = NoConstraints, | ||
Options | options = NoOptions, | ||
int | maxLength = 0, | ||
int | precision = 0, | ||
const QVariant & | defaultValue = QVariant(), | ||
const QString & | caption = QString(), | ||
const QString & | description = QString() ) |
Creates a database field with specified properties. For meaning of maxLength argument please refer to setMaxLength().
Definition at line 221 of file KDbField.cpp.
◆ KDbField() [4/7]
KDbField::KDbField | ( | const KDbField & | f | ) |
◆ ~KDbField()
|
virtual |
Definition at line 236 of file KDbField.cpp.
◆ KDbField() [5/7]
|
explicitprotected |
Definition at line 197 of file KDbField.cpp.
◆ KDbField() [6/7]
|
protected |
Creates a database field as a child of querySchema table Assigns expr expression to this field, if present. Used internally by query schemas, e.g. to declare asterisks or to add expression columns. No other properties are set, so these should be set later.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 210 of file KDbField.cpp.
◆ KDbField() [7/7]
|
explicitprotected |
Definition at line 216 of file KDbField.cpp.
Member Function Documentation
◆ caption()
QString KDbField::caption | ( | ) | const |
- Returns
- caption of this field.
Definition at line 316 of file KDbField.cpp.
◆ captionOrName()
QString KDbField::captionOrName | ( | ) | const |
- Returns
- caption of this field or - if empty - return its name.
Definition at line 326 of file KDbField.cpp.
◆ constraints()
KDbField::Constraints KDbField::constraints | ( | ) | const |
- Returns
- the constraints defined for this field.
Definition at line 301 of file KDbField.cpp.
◆ convertToType()
Converts value value to variant corresponding to type type.
Only normal types are supported. If converting is not possible a null value is returned.
- Todo
- use an array of functions?
Definition at line 426 of file KDbField.cpp.
◆ copy()
|
protectedvirtual |
- Returns
- a deep copy of this object. Used in KDbFieldList(const KDbFieldList& fl).
Reimplemented in KDbQueryAsterisk.
Definition at line 374 of file KDbField.cpp.
◆ customProperties()
KDbField::CustomPropertiesMap KDbField::customProperties | ( | ) | const |
- Returns
- all custom properties
Definition at line 1053 of file KDbField.cpp.
◆ customProperty()
QVariant KDbField::customProperty | ( | const QByteArray & | propertyName, |
const QVariant & | defaultValue = QVariant() ) const |
- Returns
- custom property propertyName. If there is no such a property, defaultValue is returned.
Definition at line 1033 of file KDbField.cpp.
◆ defaultMaxLength()
|
static |
- Returns
- default maximum length of text. Default is 0, i.e unlimited length (if the engine supports it).
Definition at line 645 of file KDbField.cpp.
◆ defaultValue()
QVariant KDbField::defaultValue | ( | ) | const |
- Returns
- default value for this field. Null value means there is no default value declared. The variant value is compatible with field's type.
Definition at line 281 of file KDbField.cpp.
◆ description()
QString KDbField::description | ( | ) | const |
- Returns
- description text for this field.
Definition at line 331 of file KDbField.cpp.
◆ enumHint()
QString KDbField::enumHint | ( | int | num | ) |
- Returns
- hint name for enum value num.
Definition at line 346 of file KDbField.cpp.
◆ enumHints()
- Returns
- the hints for enum fields.
Definition at line 341 of file KDbField.cpp.
◆ expression() [1/2]
KDbExpression KDbField::expression | ( | ) |
- Returns
- KDbExpression object if the field value is an expression. Unless the expression is set with setExpression(), it is null.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 1011 of file KDbField.cpp.
◆ expression() [2/2]
const KDbExpression KDbField::expression | ( | ) | const |
Definition at line 1016 of file KDbField.cpp.
◆ hasEmptyProperty() [1/2]
|
inline |
- Returns
- true if this field has EMPTY property (i.e. it is of type string or is a BLOB).
Definition at line 521 of file KDbField.h.
◆ hasEmptyProperty() [2/2]
|
static |
static version of hasEmptyProperty() method
- Returns
- true if this field type has EMPTY property (i.e. it is string or BLOB type)
Definition at line 557 of file KDbField.cpp.
◆ isAutoIncrement()
|
inline |
- Returns
- true if the field is autoincrement (e.g. integer/numeric)
Definition at line 282 of file KDbField.h.
◆ isAutoIncrementAllowed() [1/2]
|
inline |
- Returns
- true if this field can be auto-incremented. Actually, returns true for integer field type.
- See also
- IntegerType, isAutoIncrement()
Definition at line 531 of file KDbField.h.
◆ isAutoIncrementAllowed() [2/2]
|
static |
static version of isAutoIncrementAllowed() method
- Returns
- true if this field type can be auto-incremented.
Definition at line 562 of file KDbField.cpp.
◆ isDateTimeType() [1/2]
|
inline |
- Returns
- true if the field is of any date or time related type
Definition at line 344 of file KDbField.h.
◆ isDateTimeType() [2/2]
|
static |
Static version of isDateTimeType() method
- Returns
- true if the field is of any date or time related type
Definition at line 534 of file KDbField.cpp.
◆ isExpression()
bool KDbField::isExpression | ( | ) | const |
- Returns
- true if there is expression defined for this field. This method is provided for better readibility
- does the same as expression().isNull().
Definition at line 1006 of file KDbField.cpp.
◆ isForeignKey()
|
inline |
- Returns
- true if the field is member of single-field foreign key
Definition at line 297 of file KDbField.h.
◆ isFPNumericType() [1/2]
|
inline |
- Returns
- true if the field is of any floating point numeric type
Definition at line 335 of file KDbField.h.
◆ isFPNumericType() [2/2]
|
static |
static version of isFPNumericType() method
- Returns
- true if the field is of any floating point numeric type
Definition at line 529 of file KDbField.cpp.
◆ isIndexed()
|
inline |
- Returns
- true if the field is indexed using single-field database index.
Definition at line 312 of file KDbField.h.
◆ isIntegerType() [1/2]
|
inline |
- Returns
- true if the field is of any integer type
Definition at line 326 of file KDbField.h.
◆ isIntegerType() [2/2]
|
static |
Static version of isIntegerType() method
- Returns
- true if the field is of any integer type
Definition at line 501 of file KDbField.cpp.
◆ isNotEmpty()
|
inline |
- Returns
- true if the field is not allowed to be null
Definition at line 307 of file KDbField.h.
◆ isNotNull()
|
inline |
- Returns
- true if the field is not allowed to be null
Definition at line 302 of file KDbField.h.
◆ isNumericType() [1/2]
|
inline |
- Returns
- true if the field is of any numeric type (integer or floating point)
Definition at line 317 of file KDbField.h.
◆ isNumericType() [2/2]
|
static |
Static version of isNumericType() method
- Returns
- true if the field is of any numeric type (integer or floating point)
Definition at line 514 of file KDbField.cpp.
◆ isPrimaryKey()
|
inline |
- Returns
- true if the field is member of single-field primary key
Definition at line 287 of file KDbField.h.
◆ isQueryAsterisk()
|
inline |
There can be added asterisks (KDbQueryAsterisk objects) to query schemas' field list. KDbQueryAsterisk subclasses KDbField class, and to check if the given object (pointed by KDbField*) is asterisk or just ordinary field definition, you can call this method. This is just effective version of QObject::isA(). Every KDbQueryAsterisk object returns true here, and every KDbField object returns false.
Definition at line 640 of file KDbField.h.
◆ isTextType() [1/2]
|
inline |
- Returns
- true if the field is of any text type
Definition at line 353 of file KDbField.h.
◆ isTextType() [2/2]
|
static |
Static version of isTextType() method
- Returns
- true if the field is of any text type
Definition at line 546 of file KDbField.cpp.
◆ isUniqueKey()
|
inline |
- Returns
- true if the field is member of single-field unique key
Definition at line 292 of file KDbField.h.
◆ isUnsigned()
|
inline |
if the type has the unsigned attribute
Definition at line 515 of file KDbField.h.
◆ maxLength()
int KDbField::maxLength | ( | ) | const |
- Returns
- maximum length of text allowed for this field. Only meaningful if the type is Text.
- See also
- setMaxLength()
Definition at line 665 of file KDbField.cpp.
◆ maxLengthStrategy()
KDbField::MaxLengthStrategy KDbField::maxLengthStrategy | ( | ) | const |
- Returns
- a hint that indicates if the maximum length of text for this field is based on default setting (defaultMaxLength()) or was explicitly set. Only makes sense if the field type is Text.
Definition at line 655 of file KDbField.cpp.
◆ name()
QString KDbField::name | ( | ) | const |
- Returns
- the name of this field
Definition at line 256 of file KDbField.cpp.
◆ options()
KDbField::Options KDbField::options | ( | ) | const |
- Returns
- options defined for this field.
Definition at line 261 of file KDbField.cpp.
◆ order()
int KDbField::order | ( | ) | const |
- Returns
- order of this field in containing table (counting starts from 0) (-1 if unspecified).
Definition at line 306 of file KDbField.cpp.
◆ parent() [1/2]
KDbFieldList * KDbField::parent | ( | ) |
- Returns
- parent for this field (table, query, etc.)
Definition at line 241 of file KDbField.cpp.
◆ parent() [2/2]
const KDbFieldList * KDbField::parent | ( | ) | const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 246 of file KDbField.cpp.
◆ precision()
int KDbField::precision | ( | ) | const |
- Returns
- precision for numeric and other fields that have both length (scale) and precision (floating point types).
Definition at line 286 of file KDbField.cpp.
◆ query() [1/2]
KDbQuerySchema * KDbField::query | ( | ) |
For special use when the field defines expression.
- Returns
- query schema of query that owns this field or null if it has no query assigned.
- See also
- table()
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 600 of file KDbField.cpp.
◆ query() [2/2]
const KDbQuerySchema * KDbField::query | ( | ) | const |
Definition at line 605 of file KDbField.cpp.
◆ scale()
int KDbField::scale | ( | ) | const |
- Returns
- scale for numeric and other fields that have both length (scale) and precision (floating point types). The scale of a numeric is the count of decimal digits in the fractional part, to the right of the decimal point. The precision of a numeric is the total count of significant digits in the whole number, that is, the number of digits to both sides of the decimal point. So the number 23.5141 has a precision of 6 and a scale of 4. Integers can be considered to have a scale of zero.
Definition at line 291 of file KDbField.cpp.
◆ setAutoIncrement()
void KDbField::setAutoIncrement | ( | bool | a | ) |
Sets auto increment flag. Only available to set true, if isAutoIncrementAllowed() is true.
Definition at line 857 of file KDbField.cpp.
◆ setCaption()
void KDbField::setCaption | ( | const QString & | caption | ) |
Sets caption for this field to caption.
Definition at line 321 of file KDbField.cpp.
◆ setConstraints()
void KDbField::setConstraints | ( | Constraints | c | ) |
Sets constraints to c. If PrimaryKey is set in c, also constraits implied by being primary key are enforced (see setPrimaryKey()). If Indexed is not set in c, constraits implied by not being are enforced as well (see setIndexed()).
Definition at line 630 of file KDbField.cpp.
◆ setCustomProperty()
void KDbField::setCustomProperty | ( | const QByteArray & | propertyName, |
const QVariant & | value ) |
Sets value value for custom property propertyName.
Definition at line 1042 of file KDbField.cpp.
◆ setDefaultMaxLength()
|
static |
Sets default maximum length of text. 0 means unlimited length, greater than 0 means specific maximum length.
Definition at line 650 of file KDbField.cpp.
◆ setDefaultValue() [1/2]
bool KDbField::setDefaultValue | ( | const QByteArray & | def | ) |
Sets default value decoded from QByteArray. Decoding errors are detected (value is strictly checked against field type)
- if one is encountered, default value is cleared (defaultValue()==QVariant()).
- Returns
- true if given value was valid for field type.
- Todo
- if (!ok || (!(d->options & Unsigned) && (-v > 0x080000000 || v > (0x080000000-1))) || ((d->options & Unsigned) && (v < 0 || v > 0x100000000)))
- Todo
- BigInteger support
Definition at line 716 of file KDbField.cpp.
◆ setDefaultValue() [2/2]
void KDbField::setDefaultValue | ( | const QVariant & | def | ) |
Sets default value for this field. Setting null value removes the default value.
- See also
- defaultValue()
Definition at line 711 of file KDbField.cpp.
◆ setDescription()
void KDbField::setDescription | ( | const QString & | description | ) |
Sets description for this field to description.
Definition at line 336 of file KDbField.cpp.
◆ setEnumHints()
Sets the hint for enum fields
Definition at line 351 of file KDbField.cpp.
◆ setExpression()
void KDbField::setExpression | ( | const KDbExpression & | expr | ) |
Sets expression data expr. If there was already expression set, it is removed before new assignment. This KDbField object becames logical owner of expr object, so do not use the expression for other objects (you can call KDbExpression::clone()). Current field's expression is deleted, if exists.
Because the field defines an expression, it should be assigned to a query, not to a table, otherwise this call will not have any effect.
Definition at line 1021 of file KDbField.cpp.
◆ setForeignKey()
void KDbField::setForeignKey | ( | bool | f | ) |
Sets whether the field has to be declared with single-field foreign key. Used in KDbIndexSchema::setForeigKey().
Definition at line 894 of file KDbField.cpp.
◆ setIndexed()
void KDbField::setIndexed | ( | bool | s | ) |
Specifies whether the field is indexed (KDb::Indexed item) (by single-field implicit index) or not. Use this with caution. Since index is used to control unique, not null/empty constratins, setting this to false implies setting:
- setPrimaryKey(false)
- setUniqueKey(false)
- setNotNull(false)
- setNotEmpty(false) because above flags need index to be present. Similarly, setting one of the above flags to true, will automatically do setIndexed(true) for the same reason.
Definition at line 915 of file KDbField.cpp.
◆ setMaxLength()
void KDbField::setMaxLength | ( | int | maxLength | ) |
Sets maximum length for this field. Only works for Text type. It can be specific maximum value or 0 for unlimited length (which will work if engine supports). Resets maxLengthStrategy property to DefinedMaxLength. To reset to default maximum length, call setMaxLength(defaultMaxLength()) and then to indicate this is based on default setting, call setMaxLengthStrategy(DefaultMaxLength).
- See also
- maxLength(), maxLengthStrategy()
Definition at line 670 of file KDbField.cpp.
◆ setMaxLengthStrategy()
void KDbField::setMaxLengthStrategy | ( | MaxLengthStrategy | strategy | ) |
Sets strategy for defining maximum length of text for this field. Only makes sense if the field type is Text. Default strategy is DefinedMaxLength. Changing this value does not affect maxLength property.
Fields with DefaultMaxLength strategy does not follow changes made by calling setDefaultMaxLength() so to update the default maximum lengths in fields, the app has to iterate over all fields of type Text, and reset to the new default as explained in setMaxLength() documentation. See documentation for setMaxLength() for information how to reset maxLength to default value.
- See also
- maxLengthStrategy(), setMaxLength()
Definition at line 660 of file KDbField.cpp.
◆ setName()
void KDbField::setName | ( | const QString & | name | ) |
Sets name name for this field.
Definition at line 615 of file KDbField.cpp.
◆ setNotEmpty()
void KDbField::setNotEmpty | ( | bool | n | ) |
Specifies whether the field has single-field unique constraint or not (KDb::NotEmpty item). Setting this to true implies setting Indexed flag to true (setIndexed(true)), because index is required it control not empty constraint.
Definition at line 908 of file KDbField.cpp.
◆ setNotNull()
void KDbField::setNotNull | ( | bool | n | ) |
Specifies whether the field has single-field unique constraint or not (KDb::NotNull item). Setting this to true implies setting Indexed flag to true (setIndexed(true)), because index is required it control not null constraint.
Definition at line 901 of file KDbField.cpp.
◆ setOptions()
void KDbField::setOptions | ( | Options | options | ) |
Sets options for this field.
Definition at line 266 of file KDbField.cpp.
◆ setOrder()
|
protected |
Sets order of this field in containing table. Counting starts from 0. -1 if unspecified.
Definition at line 311 of file KDbField.cpp.
◆ setParent()
|
protected |
Sets parent for this field.
Definition at line 251 of file KDbField.cpp.
◆ setPrecision()
void KDbField::setPrecision | ( | int | p | ) |
Sets scale for this field. Only works for floating-point types.
Definition at line 676 of file KDbField.cpp.
◆ setPrimaryKey()
void KDbField::setPrimaryKey | ( | bool | p | ) |
Specifies whether the field is single-field primary key or not (KDb::PrimeryKey item). Use this with caution. Setting this to true implies setting:
- setUniqueKey(true)
- setNotNull(true)
- setNotEmpty(true)
- setIndexed(true)
Setting this to false implies setting setAutoIncrement(false).
- Todo
- is this ok for all engines?
Definition at line 867 of file KDbField.cpp.
◆ setQuery()
void KDbField::setQuery | ( | KDbQuerySchema * | query | ) |
For special use when field defines expression. Sets query schema of query that owns this field. This does not adds the field to query object. You do not need to call this method by hand. Call KDbQuerySchema::addField() instead.
- See also
- setQuery()
Definition at line 610 of file KDbField.cpp.
◆ setScale()
void KDbField::setScale | ( | int | s | ) |
Sets scale for this field. Only works for floating-point types.
- See also
- scale()
Definition at line 684 of file KDbField.cpp.
◆ setSubType()
void KDbField::setSubType | ( | const QString & | subType | ) |
Sets (optional) subtype for this field.
- See also
- subType()
Definition at line 276 of file KDbField.cpp.
◆ setTable()
void KDbField::setTable | ( | KDbTableSchema * | table | ) |
Sets table schema of table that owns this field. This does not adds the field to table object. You do not need to call this method by hand. Call KDbTableSchema::addField(KDbField *field) instead.
- See also
- setQuery()
Definition at line 595 of file KDbField.cpp.
◆ setType()
void KDbField::setType | ( | Type | t | ) |
Sets type t for this field. This does nothing if there's expression assigned.
- See also
- setExpression()
Definition at line 620 of file KDbField.cpp.
◆ setUniqueKey()
void KDbField::setUniqueKey | ( | bool | u | ) |
Specifies whether the field has single-field unique constraint or not (KDb::Unique item). Setting this to true implies setting Indexed flag to true (setIndexed(true)), because index is required it control unique constraint.
Definition at line 883 of file KDbField.cpp.
◆ setUnsigned()
void KDbField::setUnsigned | ( | bool | u | ) |
Sets unsigned flag for this field. Only works for integer types.
Definition at line 700 of file KDbField.cpp.
◆ setVisibleDecimalPlaces()
void KDbField::setVisibleDecimalPlaces | ( | int | p | ) |
Sets number of decimal places that should be visible to the user.
- See also
- visibleDecimalPlaces()
Definition at line 692 of file KDbField.cpp.
◆ specialTypesCount()
|
static |
- Returns
- number of special types available (Asterisk, Enum, etc.), that means
Definition at line 363 of file KDbField.cpp.
◆ subType()
QString KDbField::subType | ( | ) | const |
- Returns
- (optional) subtype for this field. Subtype is a string providing additional hint for field's type. E.g. for BLOB type, it can be a MIME type or certain QVariant type name, for example: "QPixmap", "QColor" or "QFont"
Definition at line 271 of file KDbField.cpp.
◆ table() [1/2]
KDbTableSchema * KDbField::table | ( | ) |
- Returns
- table schema of table that owns this field or null if it has no table assigned.
- See also
- query()
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 585 of file KDbField.cpp.
◆ table() [2/2]
const KDbTableSchema * KDbField::table | ( | ) | const |
Definition at line 590 of file KDbField.cpp.
◆ type()
KDbField::Type KDbField::type | ( | ) | const |
- Returns
- a type for this field. If there's expression assigned, type of the expression (after evaluation) is returned instead.
Definition at line 379 of file KDbField.cpp.
◆ typeForString()
|
static |
- Returns
- type for a given nontranslated type string typeString
For example returns KDbType::Integer for "Integer". typeString has to be name of type not greater than KDbField::LastType; KDbField::Null is also supported. For unsupported value KDbField::InvalidType is returned.
Definition at line 491 of file KDbField.cpp.
◆ typeGroup() [1/2]
|
inline |
- Returns
- type group for this field
Definition at line 382 of file KDbField.h.
◆ typeGroup() [2/2]
|
static |
- Returns
- group for type
For example returns KDbField::TextGroup for KDbField::Text type. type has to be an element from KDbField::Type, not greater than KDbField::LastType. For unsupported type KDbField::InvalidGroup is returned.
Definition at line 567 of file KDbField.cpp.
◆ typeGroupForString()
|
static |
- Returns
- type group for a given nontranslated type group typeGroupString
For example returns KDbField::TextGroup for "TextGroup" string. For unsupported value KDbField::InvalidGroup is returned.
Definition at line 496 of file KDbField.cpp.
◆ typeGroupName() [1/2]
|
inline |
- Returns
- a translated type group name for this field
Definition at line 387 of file KDbField.h.
◆ typeGroupName() [2/2]
- Returns
- a translated group name for typeGroup
Definition at line 474 of file KDbField.cpp.
◆ typeGroupNames()
|
static |
- Returns
- list of all available translated type group names
The first element of the list is the name of KDbField::InvalidGroup, the last one is a name of KDbField::LastTypeGroup.
Definition at line 480 of file KDbField.cpp.
◆ typeGroupsCount()
|
static |
- Returns
- number of type groups available
Definition at line 369 of file KDbField.cpp.
◆ typeGroupString() [1/2]
|
inline |
- Returns
- a type group string for this field, for example "Integer" string for KDbField::IntegerGroup.
Definition at line 399 of file KDbField.h.
◆ typeGroupString() [2/2]
- Returns
- a nontranslated type group string for typeGroup, e.g. "IntegerGroup" for IntegerGroup type
Definition at line 485 of file KDbField.cpp.
◆ typeName() [1/2]
|
inline |
- Returns
- a translated type name for this field
Definition at line 377 of file KDbField.h.
◆ typeName() [2/2]
- Returns
- a translated type name for type
type has to be an element from KDbField::Type, not greater than KDbField::LastType. Only normal types and KDbField::Null are supported. For unsupported types empty string is returned.
- See also
- typesCount() specialTypesCount()
Definition at line 458 of file KDbField.cpp.
◆ typeNames()
|
static |
- Returns
- list of all available translated names of normal types
The first element of the list is the name of KDbField::InvalidType, the last one is a name of KDbField::LastType.
- See also
- typesCount() specialTypesCount()
Definition at line 463 of file KDbField.cpp.
◆ typesCount()
|
static |
- Returns
- number of normal types available, i.e. types > InvalidType and <= LastType.
Definition at line 357 of file KDbField.cpp.
◆ typeString() [1/2]
|
inline |
- Returns
- a type string for this field, for example "Integer" string for KDbField::Integer type.
Definition at line 393 of file KDbField.h.
◆ typeString() [2/2]
- Returns
- a nontranslated type string for type
For example returns "Integer" for KDbType::Integer. type has to be an element from KDbField::Type, not greater than KDbField::LastType; KDbField::Null is also supported. For unsupported types empty string is returned.
Definition at line 468 of file KDbField.cpp.
◆ variantType() [1/2]
|
inline |
Converts field's type to QVariant equivalent as accurate as possible.
Definition at line 368 of file KDbField.h.
◆ variantType() [2/2]
|
static |
Converts type type to QVariant equivalent as accurate as possible.
Only normal types are supported.
- See also
- typesCount() specialTypesCount()
Definition at line 387 of file KDbField.cpp.
◆ visibleDecimalPlaces()
int KDbField::visibleDecimalPlaces | ( | ) | const |
- Returns
- number of decimal places that should be visible to the user, e.g. within table view widget, form or printout. Only meaningful if the field type is floating point or (in the future: decimal or currency).
- Any value less than 0 (-1 is the default) means that there should be displayed all digits of the fractional part, except the ending zeros. This is known as "auto" mode. For example, 12.345000 becomes 12.345.
- Value of 0 means that all the fractional part should be hidden (as well as the dot or comma). For example, 12.345000 becomes 12.
- Value N > 0 means that the fractional part should take exactly N digits. If the fractional part is shorter than N, additional zeros are appended. For example, "12.345" becomes "12.345000" if N=6.
Definition at line 296 of file KDbField.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:54:34 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.