KDbTableSchema
#include <KDbTableSchema.h>
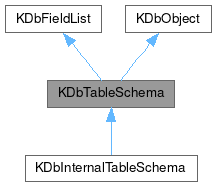
Protected Member Functions | |
KDbTableSchema (KDbConnection *conn, const QString &name=QString()) | |
KDbConnection * | connection () const |
void | setConnection (KDbConnection *conn) |
Additional Inherited Members | |
![]() | |
static KDbEscapedString | sqlFieldsList (const KDbField::List &list, KDbConnection *conn, const QString &separator=QLatin1String(","), const QString &tableOrAlias=QString(), KDb::IdentifierEscapingType escapingType=KDb::DriverEscaping) |
![]() | |
QString | caption |
QString | description |
int | id |
QString | name |
int | type |
Detailed Description
Provides information about native database table that can be stored using KDb database engine.
Definition at line 37 of file KDbTableSchema.h.
Constructor & Destructor Documentation
◆ KDbTableSchema() [1/6]
|
explicit |
Definition at line 72 of file KDbTableSchema.cpp.
◆ KDbTableSchema() [2/6]
|
explicit |
Definition at line 81 of file KDbTableSchema.cpp.
◆ KDbTableSchema() [3/6]
KDbTableSchema::KDbTableSchema | ( | ) |
Definition at line 89 of file KDbTableSchema.cpp.
◆ KDbTableSchema() [4/6]
|
explicit |
Copy constructor. If copyId is true, it's copied as well, otherwise the table id becomes -1, what is usable when we want to store the copy as an independent table.
Definition at line 97 of file KDbTableSchema.cpp.
◆ KDbTableSchema() [5/6]
KDbTableSchema::KDbTableSchema | ( | const KDbTableSchema & | ts, |
int | id ) |
Copy constructor like KDbTableSchema(const KDbTableSchema&, bool). id is set as the table identifier. This is rarely usable, e.g. in project and data migration routines when we need to need deal with unique identifiers;
- See also
- KexiMigrate::performImport().
Definition at line 105 of file KDbTableSchema.cpp.
◆ ~KDbTableSchema()
|
override |
Definition at line 124 of file KDbTableSchema.cpp.
◆ KDbTableSchema() [6/6]
|
explicitprotected |
Automatically retrieves table schema via connection.
Definition at line 115 of file KDbTableSchema.cpp.
Member Function Documentation
◆ addIndex()
bool KDbTableSchema::addIndex | ( | KDbIndexSchema * | index | ) |
Adds index index to this table schema Ownership of the index is transferred to the table schema.
- Returns
- true on success
- Since
- 3.1
Definition at line 186 of file KDbTableSchema.cpp.
◆ anyNonPKField()
KDbField * KDbTableSchema::anyNonPKField | ( | ) |
- Returns
- any field not being a part of primary key of this table. If there is no such field, returns
nullptr
.
Definition at line 359 of file KDbTableSchema.cpp.
◆ clear()
|
overridevirtual |
Removes all fields from the list, clears name and all other properties.
- See also
- KDbFieldList::clear()
Reimplemented from KDbFieldList.
Definition at line 305 of file KDbTableSchema.cpp.
◆ connection()
|
protected |
- Returns
- connection object if table was created/retrieved using a connection, otherwise
nullptr
.
Definition at line 341 of file KDbTableSchema.cpp.
◆ copyIndexFrom()
KDbIndexSchema * KDbTableSchema::copyIndexFrom | ( | const KDbIndexSchema & | index | ) |
Creates a copy of index index with references moved to fields of this table. The new index is added to this table schema. Table fields are taken by name from this table. This way it's possible to copy index owned by other table and add it to another table, e.g. a copied one.
To copy an index from another table, call:
To copy an index within the same table, call:
- Since
- 3.1
- Todo
- All relationships should be also copied
Definition at line 204 of file KDbTableSchema.cpp.
◆ debugFields()
Sends information about fields of this table schema to debug output dbg.
Definition at line 314 of file KDbTableSchema.cpp.
◆ indices()
const QList< KDbIndexSchema * > * KDbTableSchema::indices | ( | ) | const |
Definition at line 181 of file KDbTableSchema.cpp.
◆ indicesIterator()
const QList< KDbIndexSchema * >::ConstIterator KDbTableSchema::indicesIterator | ( | ) | const |
Definition at line 176 of file KDbTableSchema.cpp.
◆ insertField()
|
overridevirtual |
Inserts field into a specified position (index). 'order' property of field is set automatically. false
is returned if field is nullptr
or index is invalid.
Reimplemented from KDbFieldList.
Definition at line 243 of file KDbTableSchema.cpp.
◆ isInternal()
bool KDbTableSchema::isInternal | ( | ) | const |
- Returns
- true if this is internal KDb's table. Internal tables are hidden in applications (if desired) but are available for schema export/import functionality.
Any internal KDb system table's schema (kexi__*) has cleared its KDbObject part, e.g. id=-1 for such table, and no description, caption and so on. This is because it represents a native database table rather that extended Kexi table.
KDbTableSchema object has this property set to false, KDbInternalTableSchema has it set to true.
Definition at line 211 of file KDbTableSchema.cpp.
◆ lookupFields()
QVector< KDbLookupFieldSchema * > KDbTableSchema::lookupFields | ( | ) | const |
- Returns
- list of lookup field schemas for this table. The order is the same as the order of fields within the table.
Definition at line 406 of file KDbTableSchema.cpp.
◆ lookupFieldSchema() [1/3]
KDbLookupFieldSchema * KDbTableSchema::lookupFieldSchema | ( | const KDbField & | field | ) |
- Returns
- lookup field schema for field. 0 is returned if there is no such field in the table or this field has no lookup schema. Note that even id non-zero is returned here, you may want to check whether lookup field's recordSource().name() is empty (if so, the field should behave as there was no lookup field defined at all).
Definition at line 388 of file KDbTableSchema.cpp.
◆ lookupFieldSchema() [2/3]
const KDbLookupFieldSchema * KDbTableSchema::lookupFieldSchema | ( | const KDbField & | field | ) | const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 393 of file KDbTableSchema.cpp.
◆ lookupFieldSchema() [3/3]
KDbLookupFieldSchema * KDbTableSchema::lookupFieldSchema | ( | const QString & | fieldName | ) |
Definition at line 398 of file KDbTableSchema.cpp.
◆ primaryKey() [1/2]
KDbIndexSchema * KDbTableSchema::primaryKey | ( | ) |
- Returns
- list of fields that are primary key of this table. This method never returns
nullptr
value, if there is no primary key, empty KDbIndexSchema object is returned. KDbIndexSchema object is owned by the table schema.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 166 of file KDbTableSchema.cpp.
◆ primaryKey() [2/2]
const KDbIndexSchema * KDbTableSchema::primaryKey | ( | ) | const |
Definition at line 171 of file KDbTableSchema.cpp.
◆ query()
KDbQuerySchema * KDbTableSchema::query | ( | ) |
- Returns
- query schema object that is defined by "select * from <this_table_name>" This query schema object is owned by the table schema object. It is convenient way to get such a query when it is not available otherwise. Always returns non-0.
Definition at line 351 of file KDbTableSchema.cpp.
◆ removeField()
|
overridevirtual |
Reimplemented for internal reasons.
Reimplemented from KDbFieldList.
Definition at line 293 of file KDbTableSchema.cpp.
◆ removeIndex()
bool KDbTableSchema::removeIndex | ( | KDbIndexSchema * | index | ) |
Removes index index from this table schema Ownership of the index is transferred to the table schema.
- Returns
- true on success
- Since
- 3.1
Definition at line 195 of file KDbTableSchema.cpp.
◆ setConnection()
|
protected |
For KDbConnection.
Definition at line 346 of file KDbTableSchema.cpp.
◆ setLookupFieldSchema()
bool KDbTableSchema::setLookupFieldSchema | ( | const QString & | fieldName, |
KDbLookupFieldSchema * | lookupFieldSchema ) |
Sets lookup field schema lookupFieldSchema for fieldName. Passing nullptr
lookupFieldSchema will remove the previously set lookup field.
- Returns
- true if lookupFieldSchema has been added, or false if there is no such field fieldName.
Definition at line 373 of file KDbTableSchema.cpp.
◆ setPrimaryKey()
void KDbTableSchema::setPrimaryKey | ( | KDbIndexSchema * | pkey | ) |
Sets table's primary key index to pkey. Pass pkey as nullptr
to unassign existing primary key. In this case "primary" property of previous primary key KDbIndexSchema object that will be cleared, making it an ordinary index.
If this table already has primary key assigned, it is unassigned using setPrimaryKey(nullptr).
Before assigning as primary key, you should add the index to indices list with addIndex() (this is not done automatically!).
<
Definition at line 216 of file KDbTableSchema.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:54:35 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.