KDbFieldList
#include <KDbFieldList.h>
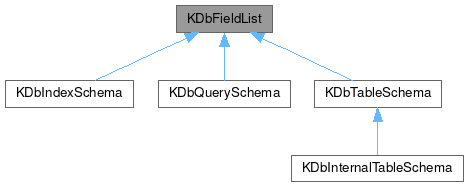
Public Member Functions | |
KDbFieldList (bool owner=false) | |
KDbFieldList (const KDbFieldList &fl, bool deepCopyFields=true) | |
virtual | ~KDbFieldList () |
bool | addField (KDbField *field) |
KDbField::List * | autoIncrementFields () const |
virtual void | clear () |
virtual KDbField * | field (const QString &name) |
virtual const KDbField * | field (const QString &name) const |
virtual KDbField * | field (int id) |
virtual const KDbField * | field (int id) const |
int | fieldCount () const |
KDbField::List * | fields () |
const KDbField::List * | fields () const |
KDbField::ListIterator | fieldsIterator () const |
KDbField::ListIterator | fieldsIteratorConstEnd () const |
bool | hasField (const KDbField &field) const |
int | indexOf (const KDbField &field) const |
virtual bool | insertField (int index, KDbField *field) |
bool | isEmpty () const |
bool | isOwner () const |
virtual bool | moveField (KDbField *field, int newIndex) |
QStringList | names () const |
virtual bool | removeField (KDbField *field) |
bool | renameField (const QString &oldName, const QString &newName) |
bool | renameField (KDbField *field, const QString &newName) |
KDbEscapedString | sqlFieldsList (KDbConnection *conn, const QString &separator=QLatin1String(","), const QString &tableOrAlias=QString(), KDb::IdentifierEscapingType escapingType=KDb::DriverEscaping) const |
KDbFieldList * | subList (const QList< int > &list) |
KDbFieldList * | subList (const QList< QByteArray > &list) |
KDbFieldList * | subList (const QString &n1, const QString &n2=QString(), const QString &n3=QString(), const QString &n4=QString(), const QString &n5=QString(), const QString &n6=QString(), const QString &n7=QString(), const QString &n8=QString(), const QString &n9=QString(), const QString &n10=QString(), const QString &n11=QString(), const QString &n12=QString(), const QString &n13=QString(), const QString &n14=QString(), const QString &n15=QString(), const QString &n16=QString(), const QString &n17=QString(), const QString &n18=QString()) |
KDbFieldList * | subList (const QStringList &list) |
Static Public Member Functions | |
static KDbEscapedString | sqlFieldsList (const KDbField::List &list, KDbConnection *conn, const QString &separator=QLatin1String(","), const QString &tableOrAlias=QString(), KDb::IdentifierEscapingType escapingType=KDb::DriverEscaping) |
Detailed Description
Helper class that stores list of fields.
Definition at line 33 of file KDbFieldList.h.
Constructor & Destructor Documentation
◆ KDbFieldList() [1/2]
|
explicit |
Creates empty list of fields. If owner is true, the list will be owner of any added field, what means that these field will be removed on the list destruction. Otherwise, the list just points any field that was added.
- See also
- isOwner()
Definition at line 67 of file KDbFieldList.cpp.
◆ KDbFieldList() [2/2]
|
explicit |
Copy constructor. If deepCopyFields is true, all fields are deeply copied, else only pointer are copied. Reimplemented in KDbQuerySchema constructor.
- Todo
- IMPORTANT: (API) improve deepCopyFields
Definition at line 74 of file KDbFieldList.cpp.
◆ ~KDbFieldList()
|
virtual |
Destroys the list. If the list owns fields (see constructor), these are also deleted.
Definition at line 90 of file KDbFieldList.cpp.
Member Function Documentation
◆ addField()
bool KDbFieldList::addField | ( | KDbField * | field | ) |
Adds field at the and of field list.
Definition at line 161 of file KDbFieldList.cpp.
◆ autoIncrementFields()
KDbField::List * KDbFieldList::autoIncrementFields | ( | ) | const |
- Returns
- list of autoincremented fields. The list is owned by this KDbFieldList object.
Definition at line 396 of file KDbFieldList.cpp.
◆ clear()
|
virtual |
Removes all fields from the list.
Reimplemented in KDbQuerySchema, and KDbTableSchema.
Definition at line 115 of file KDbFieldList.cpp.
◆ field() [1/4]
- Returns
- field with name name or
nullptr
if there is no such a field.
Reimplemented in KDbQuerySchema.
Definition at line 204 of file KDbFieldList.cpp.
◆ field() [2/4]
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Reimplemented in KDbQuerySchema.
Definition at line 209 of file KDbFieldList.cpp.
◆ field() [3/4]
|
virtual |
- Returns
- field id or
nullptr
if there is no such a field.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Reimplemented in KDbQuerySchema.
Definition at line 194 of file KDbFieldList.cpp.
◆ field() [4/4]
|
virtual |
Definition at line 199 of file KDbFieldList.cpp.
◆ fieldCount()
int KDbFieldList::fieldCount | ( | ) | const |
- Returns
- number of fields in the list.
Definition at line 105 of file KDbFieldList.cpp.
◆ fields() [1/2]
KDbField::List * KDbFieldList::fields | ( | ) |
- Returns
- list of fields
Definition at line 95 of file KDbFieldList.cpp.
◆ fields() [2/2]
const KDbField::List * KDbFieldList::fields | ( | ) | const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 100 of file KDbFieldList.cpp.
◆ fieldsIterator()
KDbField::ListIterator KDbFieldList::fieldsIterator | ( | ) | const |
- Returns
- iterator for fields
Definition at line 340 of file KDbFieldList.cpp.
◆ fieldsIteratorConstEnd()
KDbField::ListIterator KDbFieldList::fieldsIteratorConstEnd | ( | ) | const |
- Returns
- iterator for fields
Definition at line 345 of file KDbFieldList.cpp.
◆ hasField()
bool KDbFieldList::hasField | ( | const KDbField & | field | ) | const |
- Returns
- true if this list contains given field.
Definition at line 214 of file KDbFieldList.cpp.
◆ indexOf()
int KDbFieldList::indexOf | ( | const KDbField & | field | ) | const |
- Returns
- first occurrence of field in the list or -1 if this list does not contain this field.
Definition at line 219 of file KDbFieldList.cpp.
◆ insertField()
|
virtual |
Inserts field into a specified index position.
false
is returned if field is nullptr
or index is invalid.
Note: You can reimplement this method but you should still call this implementation in your subclass.
Reimplemented in KDbQuerySchema, and KDbTableSchema.
Definition at line 123 of file KDbFieldList.cpp.
◆ isEmpty()
bool KDbFieldList::isEmpty | ( | ) | const |
- Returns
- true if the list is empty.
Definition at line 110 of file KDbFieldList.cpp.
◆ isOwner()
bool KDbFieldList::isOwner | ( | ) | const |
- Returns
- true if fields in the list are owned by this list.
Definition at line 350 of file KDbFieldList.cpp.
◆ moveField()
|
virtual |
Moves fiels field from its current position to new position newIndex. If newIndex value is greater than fieldCount()-1, it is appended.
- Returns
false
if this field does not belong to this list or isnullptr
.
Definition at line 180 of file KDbFieldList.cpp.
◆ names()
QStringList KDbFieldList::names | ( | ) | const |
- Returns
- list of field names for this list.
Definition at line 331 of file KDbFieldList.cpp.
◆ removeField()
|
virtual |
Removes field from the field list and deletes it. Use with care.
Note: You can reimplement this method but you should still call this implementation in your subclass.
- Returns
- false if this field does not belong to this list.
Reimplemented in KDbQuerySchema, and KDbTableSchema.
Definition at line 166 of file KDbFieldList.cpp.
◆ renameField() [1/2]
Renames field oldName to newName.
false
is returned if field with oldName name does not exist or field with newName name already exists.
- Note
- Do not use this for physical renaming columns. Use KDbAlterTableHandler instead.
Definition at line 141 of file KDbFieldList.cpp.
◆ renameField() [2/2]
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 151 of file KDbFieldList.cpp.
◆ sqlFieldsList() [1/2]
|
static |
Like above, but this is convenient static function, so you can pass any list here.
Definition at line 356 of file KDbFieldList.cpp.
◆ sqlFieldsList() [2/2]
KDbEscapedString KDbFieldList::sqlFieldsList | ( | KDbConnection * | conn, |
const QString & | separator = QLatin1String(","), | ||
const QString & | tableOrAlias = QString(), | ||
KDb::IdentifierEscapingType | escapingType = KDb::DriverEscaping ) const |
- Returns
- a string that is a result of all field names concatenated and with separator. This is usable e.g. as argument like "field1,field2" for "INSERT INTO (xxx) ..". The result of this method is effectively cached, and it is invalidated when set of fields changes (e.g. using clear() or addField()).
tableOrAlias, if provided is prepended to each field, so the resulting names will be in form tableOrAlias.fieldName. This option is used for building queries with joins, where fields have to be spicified without ambiguity. See KDbConnection::selectStatement() for example use.
escapingType can be used to alter default escaping type. If conn is not provided for DriverEscaping, no escaping is performed.
Definition at line 385 of file KDbFieldList.cpp.
◆ subList() [1/4]
KDbFieldList * KDbFieldList::subList | ( | const QList< int > & | list | ) |
Like above, but with a list of field indices
Definition at line 314 of file KDbFieldList.cpp.
◆ subList() [2/4]
KDbFieldList * KDbFieldList::subList | ( | const QList< QByteArray > & | list | ) |
Definition at line 303 of file KDbFieldList.cpp.
◆ subList() [3/4]
KDbFieldList * KDbFieldList::subList | ( | const QString & | n1, |
const QString & | n2 = QString(), | ||
const QString & | n3 = QString(), | ||
const QString & | n4 = QString(), | ||
const QString & | n5 = QString(), | ||
const QString & | n6 = QString(), | ||
const QString & | n7 = QString(), | ||
const QString & | n8 = QString(), | ||
const QString & | n9 = QString(), | ||
const QString & | n10 = QString(), | ||
const QString & | n11 = QString(), | ||
const QString & | n12 = QString(), | ||
const QString & | n13 = QString(), | ||
const QString & | n14 = QString(), | ||
const QString & | n15 = QString(), | ||
const QString & | n16 = QString(), | ||
const QString & | n17 = QString(), | ||
const QString & | n18 = QString() ) |
Creates and returns a list that contain fields selected by name. At least one field (exising on this list) should be selected, otherwise 0 is returned. Returned KDbFieldList object is not owned by any parent (so you need to destroy yourself) and KDbField objects included in it are not owned by it (but still as before, by 'this' object). Returned list can be usable e.g. as argument for KDbConnection::insertRecord(). 0 is returned if at least one name cannot be found.
Definition at line 251 of file KDbFieldList.cpp.
◆ subList() [4/4]
KDbFieldList * KDbFieldList::subList | ( | const QStringList & | list | ) |
Like above, but for QStringList.
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 285 of file KDbFieldList.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 17:00:43 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.