KDbAlterTableHandler
#include <KDbAlter.h>
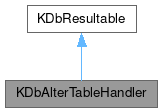
Classes | |
class | ActionBase |
class | ChangeFieldPropertyAction |
class | ExecutionArguments |
class | FieldActionBase |
class | InsertFieldAction |
class | MoveFieldPositionAction |
class | RemoveFieldAction |
Public Types | |
typedef KDbUtils::AutodeletedHash< QByteArray, ActionBase * > | ActionDict |
typedef QHash< QByteArray, ActionBase * >::ConstIterator | ActionDictConstIterator |
typedef KDbUtils::AutodeletedHash< int, ActionDict * > | ActionDictDict |
typedef QHash< int, ActionDict * >::ConstIterator | ActionDictDictConstIterator |
typedef QHash< int, ActionDict * >::Iterator | ActionDictDictIterator |
typedef QHash< QByteArray, ActionBase * >::Iterator | ActionDictIterator |
typedef QList< ActionBase * > | ActionList |
typedef QList< ActionBase * >::ConstIterator | ActionListIterator |
typedef QVector< ActionBase * > | ActionsVector |
enum | AlteringRequirements { PhysicalAlteringRequired = 1 , DataConversionRequired = 2 , MainSchemaAlteringRequired = 4 , ExtendedSchemaAlteringRequired = 8 , SchemaAlteringRequired = ExtendedSchemaAlteringRequired | MainSchemaAlteringRequired } |
Public Member Functions | |
KDbAlterTableHandler (KDbConnection *conn) | |
const ActionList & | actions () const |
void | addAction (ActionBase *action) |
void | clear () |
void | debug () |
KDbTableSchema * | execute (const QString &tableName, ExecutionArguments *args) |
KDbAlterTableHandler & | operator<< (ActionBase *action) |
void | removeAction (int index) |
void | setActions (const ActionList &actions) |
![]() | |
KDbResultable (const KDbResultable &other) | |
void | clearResult () |
KDbMessageHandler * | messageHandler () const |
KDbResultable & | operator= (const KDbResultable &other) |
KDbResult | result () const |
virtual QString | serverResultName () const |
void | setMessageHandler (KDbMessageHandler *handler) |
void | showMessage () |
Static Public Member Functions | |
static int | alteringTypeForProperty (const QByteArray &propertyName) |
Additional Inherited Members | |
![]() | |
Private *const | d |
KDbResult | m_result |
Detailed Description
A tool for handling altering database table schema.
In relational (and other) databases, table schema altering is not an easy task. It may be considered as easy if there is no data that user wants to keep while the table schema is altered. Otherwise, if the table is alredy filled with data, there could be no easy algorithm like:
- Drop existing table
- Create new one with altered schema.
Instead, more complex algorithm is needed. To perform the table schema alteration, a list of well defined atomic operations is used as a "recipe".
- Look at the current data, and: 1.1. analyze what values will be removed (in case of impossible conversion or table field removal); 1.2. analyze what values can be converted (e.g. from numeric types to text), and so on.
- Optimize the atomic actions knowing that sometimes a compilation of one action and another that's opposite to the first means "do nothing". The optimization is a simulating of actions' execution. For example, when both action A="change field name from 'city' to 'town'" and action B="change field name from 'town' to 'city'" is specified, the compilation of the actions means "change field name from 'city' to 'city'", what is a NULL action. On the other hand, we need to execute all the actions on the destination table in proper order, and not just drop them. For the mentioned example, between actions A and B there can be an action like C="change the type of field 'city' to LongText". If A and B were simply removed, C would become invalid (there is no 'city' field).
- Ask user whether she agrees with the results of analysis mentioned in 1. 3.2. Additionally, it may be possible to get some hints from the user, as humans usually know more about logic behind the altered table schema than any machine. If the user provided hints about the altering, apply them to the actions list.
- Create (empty) destination table schema with temporary name, using the information collected so far.
- Copy the data from the source to destionation table. Convert values, move them between fields, using the information collected.
- Remove the source table.
- Rename the destination table to the name previously assigned for the source table.
Notes: The actions 4 to 7 should be performed within a database transaction. [todo] We want to take care about database relationships as well. For example, is a table field is removed, relationships related to this field should be also removed (similar rules as in the Query Designer). Especially, care about primary keys and uniquess (indices). Recreate them when needed. The problem could be if such analysis may require to fetch the entire table data to the client side. Use "SELECT INTO" statements if possible to avoid such a treat.
The KDbAlterTableHandler is used in Kexi's Table Designer. Already opened KDbConnection object is needed.
Use case:
Actions for Alter
Definition at line 109 of file KDbAlter.h.
Member Typedef Documentation
◆ ActionDict
For collecting actions related to a single field.
Definition at line 143 of file KDbAlter.h.
◆ ActionDictConstIterator
QHash<QByteArray,ActionBase*>::ConstIterator KDbAlterTableHandler::ActionDictConstIterator |
Definition at line 146 of file KDbAlter.h.
◆ ActionDictDict
for collecting groups of actions by field UID
Definition at line 144 of file KDbAlter.h.
◆ ActionDictDictConstIterator
QHash<int,ActionDict*>::ConstIterator KDbAlterTableHandler::ActionDictDictConstIterator |
Definition at line 148 of file KDbAlter.h.
◆ ActionDictDictIterator
QHash<int,ActionDict*>::Iterator KDbAlterTableHandler::ActionDictDictIterator |
Definition at line 147 of file KDbAlter.h.
◆ ActionDictIterator
QHash<QByteArray,ActionBase*>::Iterator KDbAlterTableHandler::ActionDictIterator |
Definition at line 145 of file KDbAlter.h.
◆ ActionList
Defines a type for action list.
Definition at line 152 of file KDbAlter.h.
◆ ActionListIterator
QList<ActionBase*>::ConstIterator KDbAlterTableHandler::ActionListIterator |
Defines a type for action list's iterator.
Definition at line 155 of file KDbAlter.h.
◆ ActionsVector
for collecting actions related to a single field
Definition at line 149 of file KDbAlter.h.
Member Enumeration Documentation
◆ AlteringRequirements
Defines flags for possible altering requirements; can be combined.
Definition at line 118 of file KDbAlter.h.
Constructor & Destructor Documentation
◆ KDbAlterTableHandler()
|
explicit |
Definition at line 762 of file KDbAlter.cpp.
◆ ~KDbAlterTableHandler()
|
override |
Definition at line 768 of file KDbAlter.cpp.
Member Function Documentation
◆ actions()
const KDbAlterTableHandler::ActionList & KDbAlterTableHandler::actions | ( | ) | const |
- Returns
- a list of actions for this AlterTable object. Use ActionBase::ListIterator to iterate over the list items.
Definition at line 784 of file KDbAlter.cpp.
◆ addAction()
void KDbAlterTableHandler::addAction | ( | ActionBase * | action | ) |
Appends action for the alter table tool.
Definition at line 773 of file KDbAlter.cpp.
◆ alteringTypeForProperty()
|
static |
Like execute() with simulate set to true, but debug is directed to debugString. This function is used only in the alter table test suite.
- Returns
- a combination of AlteringRequirements values decribing altering type required when a given property field's propertyName is altered. Used internally KDbAlterTableHandler. Moreover it can be also used in the Table Designer's code as a temporary replacement before KDbAlterTableHandler is fully implemented. Thus, it is possible to identify properties that have no PhysicalAlteringRequired flag set (e.g. caption or extended properties like visibleDecimalPlaces.
Definition at line 181 of file KDbAlter.cpp.
◆ clear()
void KDbAlterTableHandler::clear | ( | ) |
Removes all actions from the alter table tool.
Definition at line 794 of file KDbAlter.cpp.
◆ debug()
void KDbAlterTableHandler::debug | ( | ) |
Displays debug information about all actions collected by the handler.
Definition at line 805 of file KDbAlter.cpp.
◆ execute()
KDbTableSchema * KDbAlterTableHandler::execute | ( | const QString & | tableName, |
ExecutionArguments * | args ) |
Performs table alteration using predefined actions for table named tableName, assuming it already exists. The KDbConnection object passed to the constructor must exist, must be connected and a database must be used. The connection must not be read-only.
If args.simulate is true, the execution is only simulated, i.e. al lactions are processed like for regular execution but no changes are performed physically. This mode is used only for debugging purposes.
- Todo
- For some cases, table schema can completely change, so it will be needed to refresh all objects depending on it. Implement this!
Sets args.result to true on success, to false on failure or when the above requirements are not met (then, you can get a detailed error message from KDbObject). When the action has been cancelled (stopped), args.result is set to cancelled value. If args.debugString is not 0, it will be filled with debugging output.
- Returns
- the new table schema object created as a result of schema altering. The old table is returned if recreating table schema was not necessary or args.simulate is true. 0 is returned if args.result is not true.
- Todo
- err msg?
- Todo
- err msg?
- Todo
- err msg?
- Todo
- err msg?
- Todo
- transaction!
copy id
- Todo
- better errmsg?
- Todo
- delete newTable...
- Todo
- support expressions (eg. TODAY()) as a default value
- Todo
- this field can be notNull or notEmpty - check whether the default is ok (or do this checking also in the Table Designer?)
- Todo
- support unique, validatationRule, unsigned flags...
- Todo
- check for foreignKey values...
- Todo
- delete newTable...
- Todo
- delete newTable...
- Todo
- delete newTable...
Definition at line 813 of file KDbAlter.cpp.
◆ operator<<()
KDbAlterTableHandler & KDbAlterTableHandler::operator<< | ( | ActionBase * | action | ) |
Provided for convenience,
- See also
- addAction(const ActionBase& action).
Definition at line 778 of file KDbAlter.cpp.
◆ removeAction()
void KDbAlterTableHandler::removeAction | ( | int | index | ) |
Removes an action from the alter table tool at index index.
Definition at line 789 of file KDbAlter.cpp.
◆ setActions()
void KDbAlterTableHandler::setActions | ( | const ActionList & | actions | ) |
Sets actions for the alter table tool. Previous actions are cleared. actions will be owned by the KDbAlterTableHandler object.
Definition at line 799 of file KDbAlter.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Sat Dec 21 2024 17:00:43 by doxygen 1.12.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.