KDbConnection
#include <KDbConnection.h>
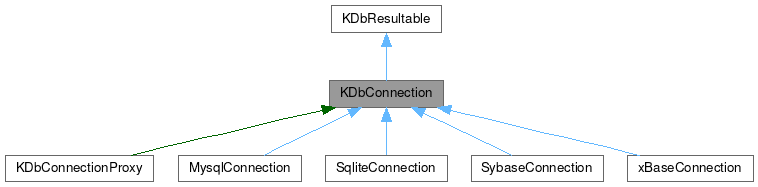
Public Types | |
enum class | AlterTableNameOption { Default = 0 , DropDestination = 1 } |
typedef QFlags< AlterTableNameOption > | AlterTableNameOptions |
enum class | CreateTableOption { Default = 0 , DropDestination = 1 } |
typedef QFlags< CreateTableOption > | CreateTableOptions |
enum class | QueryRecordOption { AddLimitTo1 = 1 , Default = AddLimitTo1 } |
typedef QFlags< QueryRecordOption > | QueryRecordOptions |
Static Public Member Functions | |
static QStringList | kdbSystemTableNames () |
Public Attributes | |
H_INS_REC_ALL | |
Additional Inherited Members | |
![]() | |
Private *const | d |
KDbResult | m_result |
Detailed Description
Provides database connection, allowing queries and data modification.
This class represents a database connection established within a data source. It supports data queries and modification by creating client-side database cursors. Database transactions are supported.
Definition at line 51 of file KDbConnection.h.
Member Typedef Documentation
◆ AlterTableNameOptions
Definition at line 703 of file KDbConnection.h.
◆ CreateTableOptions
Definition at line 628 of file KDbConnection.h.
◆ QueryRecordOptions
Definition at line 487 of file KDbConnection.h.
Member Enumeration Documentation
◆ AlterTableNameOption
|
strong |
Options for altering table name.
- Since
- 3.1
Enumerator | |
---|---|
DropDestination | Drop destination table if exists. |
Definition at line 699 of file KDbConnection.h.
◆ CreateTableOption
|
strong |
Options for creating table.
- Since
- 3.1
Enumerator | |
---|---|
DropDestination | Drop destination table if exists. |
Definition at line 624 of file KDbConnection.h.
◆ QueryRecordOption
|
strong |
Options for querying records.
- Since
- 3.1
Enumerator | |
---|---|
AddLimitTo1 | Adds a "LIMIT 1" clause to the query for optimization purposes (it should not include one already) |
Definition at line 482 of file KDbConnection.h.
Constructor & Destructor Documentation
◆ ~KDbConnection()
|
override |
Opened connection is automatically disconnected and removed from driver's connections list. Note for driver developers: you should call destroy() from you KDbConnection's subclass destructor.
Definition at line 477 of file KDbConnection.cpp.
◆ KDbConnection()
|
protected |
Used by KDbDriver
Definition at line 461 of file KDbConnection.cpp.
Member Function Documentation
◆ addCursor()
|
protected |
Used by KDbCursor class.
Definition at line 3509 of file KDbConnection.cpp.
◆ alterTable()
tristate KDbConnection::alterTable | ( | KDbTableSchema * | tableSchema, |
KDbTableSchema * | newTableSchema ) |
Alters tableSchema using newTableSchema in memory and on the db backend.
- Returns
- true on success, cancelled if altering was cancelled.
- Todo
(js): implement real altering
(js): update any structure (e.g. query) that depend on this table!
- Todo
- (js) implement real altering
- Todo
- (js) update any structure (e.g. query) that depend on this table!
- Todo
- uncomment:
Definition at line 1671 of file KDbConnection.cpp.
◆ alterTableName()
bool KDbConnection::alterTableName | ( | KDbTableSchema * | tableSchema, |
const QString & | newName, | ||
AlterTableNameOptions | options = AlterTableNameOption::Default ) |
Alters name of table.
Alters name of table described by tableSchema to newName. If options include the DropDestination value and table having name newName already exists, it is physically dropped, removed from connection's list of tables and replaced by tableSchema. In this case identifier of tableSchema is set to the dropped table's identifier. This can be useful if tableSchema was created with a temporary name and identifier. It is for example used in KDbAlterTableHandler.
If options do not include the DropDestination value (the default) and table having name newName already exists, false
is returned and ERR_OBJECT_EXISTS
error is set in the connection object.
Table name in the schema of tableSchema is updated on successful altering.
- Returns
- true on success.
- Todo
- alter table name for server DB backends!
- Todo
- what about objects (queries/forms) that use old name?
- Todo
- what about caption?
Definition at line 1700 of file KDbConnection.cpp.
◆ anyAvailableDatabaseName()
|
virtual |
- Returns
- name of any (e.g. first found) database for this connection. This method does not close or open this connection. The method can be used (it is also internally used, e.g. for database dropping) when we need a database name before we can connect and execute any SQL statement (e.g. DROP DATABASE).
The method can return nul lstring, but in this situation no automatic (implicit) connections could be made, what is useful by e.g. dropDatabase().
Note for driver developers: return here a name of database which you are sure is existing. Default implementation returns:
- value that previously had been set using setAvailableDatabaseName() for this connection, if it is not empty
- else (2nd priority): value of KDbDriverBehavior::ALWAYS_AVAILABLE_DATABASE_NAME if it is not empty.
See description of KDbDriverBehavior::ALWAYS_AVAILABLE_DATABASE_NAME member. You may want to reimplement this method only when you need to depend on this connection specifics (e.g. you need to check something remotely).
Reimplemented in KDbConnectionProxy.
Definition at line 3065 of file KDbConnection.cpp.
◆ autoCommit()
bool KDbConnection::autoCommit | ( | ) | const |
- Returns
- true if "auto commit" option is on.
When auto commit is on (the default on for any new KDbConnection object), every SQL statement that manipulates data in the database implicitly starts a new transaction. This transaction is automatically committed after successful statement execution or rolled back on error.
For drivers that do not support transactions (see KDbDriver::features()) this method shouldn't be called because it does nothing ans always returns false.
No internal KDb object should changes this option, although auto commit's behavior depends on database engine's specifics. Engines that support only single transaction per connection (see KDbDriver::SingleTransactions), use this single connection for autocommiting, so if there is already transaction started by the KDb user program (with beginTransaction()), this transaction is committed before any statement that manipulates data. In this situation default transaction is also affected (see defaultTransaction()).
Only for drivers that support nested transactions (KDbDriver::NestedTransactions), autocommiting works independently from previously started transaction,
For other drivers set this option off if you need use transaction for grouping more statements together.
NOTE: nested transactions are not yet implemented in KDb API.
Definition at line 2095 of file KDbConnection.cpp.
◆ beginAutoCommitTransaction()
|
protected |
Internal, for handling autocommited transactions: begins transaction if one is supported.
- Returns
- true if new transaction started successfully or no transactions are supported at all by the driver or if autocommit option is turned off. A handle to a newly created transaction (or
nullptr
on error) is passed to tg parameter.
Special case when used database driver has only single transaction support (KDbDriver::SingleTransactions): and there is already transaction started, it is committed before starting a new one, but only if this transaction has been started inside KDbConnection object. (i.e. by beginAutoCommitTransaction()). Otherwise, a new transaction will not be started, but true will be returned immediately.
Definition at line 1893 of file KDbConnection.cpp.
◆ beginTransaction()
KDbTransaction KDbConnection::beginTransaction | ( | ) |
Starts a new database transaction.
- Returns
- KDbTransaction object. If transaction has been started successfully returned object points to it, otherwise null transaction is returned.
For drivers that allow single transaction per connection (KDbDriver::features() && SingleTransactions) this method can be called once and that transaction will be default one (setDefaultTransaction() will be called). For drivers that allow multiple transactions per connection no default transaction is set automatically in beginTransaction(). setDefaultTransaction() can be called by hand.
- See also
- setDefaultTransaction(), defaultTransaction().
Definition at line 1953 of file KDbConnection.cpp.
◆ checkConnected()
|
protected |
Helper: checks if connection is established; if not: error message is set up and false returned
Definition at line 551 of file KDbConnection.cpp.
◆ checkIfColumnExists()
|
protected |
- Returns
- true if the cursor cursor contains column column, else, sets appropriate error with a message and returns false.
Definition at line 2398 of file KDbConnection.cpp.
◆ checkIsDatabaseUsed()
|
protected |
Helper: checks both if connection is established and database any is used; if not: error message is set up and false returned
Definition at line 562 of file KDbConnection.cpp.
◆ clearResult()
void KDbConnection::clearResult | ( | ) |
Reimplemented, also clears sql string.
- See also
- recentSqlString()
Definition at line 526 of file KDbConnection.cpp.
◆ closeDatabase()
bool KDbConnection::closeDatabase | ( | ) |
Closes currently used database for this connection.
Any active transactions (if supported) are rolled back, so commit these before closing, if you'd like to save your changes.
- Todo
- (js) add CLEVER algorithm here for nested transactions
Definition at line 852 of file KDbConnection.cpp.
◆ commitAutoCommitTransaction()
|
protected |
Internal, for handling autocommited transactions: Commits transaction prevoiusly started with beginAutoCommitTransaction().
- Returns
- true on success or when no transactions are supported at all by the driver.
Special case when used database driver has only single transaction support (KDbDriver::SingleTransactions): if trans has been started outside KDbConnection object (i.e. not by beginAutoCommitTransaction()), the transaction will not be committed.
Definition at line 1924 of file KDbConnection.cpp.
◆ commitTransaction()
bool KDbConnection::commitTransaction | ( | KDbTransaction | transaction = KDbTransaction(), |
KDbTransaction::CommitOptions | options = KDbTransaction::CommitOptions() ) |
Commits specified transaction for this connection.
If transaction is not active and default transaction (obtained from defaultTransaction()) exists, the default one will be committed. If neither the default one is not present returns true
if IgnoreInactive is set in options or false
if IgnoreInactive is not set in options.
- Returns
false
on any error.
On successful commit, transaction object will point to a null transaction. After commiting a default transaction, there is no default transaction anymore.
Definition at line 1992 of file KDbConnection.cpp.
◆ connect()
bool KDbConnection::connect | ( | ) |
Connects to driver with given parameters.
- Returns
- true if successful.
Note: many database drivers may require connData.databaseName() to be specified because explicit database name is needed to perform connection (e.g. SQLite, PostgreSQL). MySQL does not require database name; KDbConnection::useDatabase() can be called later.
Definition at line 494 of file KDbConnection.cpp.
◆ containsTable()
- Returns
- true if table with name tableName exists in the database.
-
false
if it does not exist orcancelled
if error occurred. The lookup is case insensitive. This method can be much faster than tableNames().
Definition at line 1039 of file KDbConnection.cpp.
◆ copyDataBlock()
bool KDbConnection::copyDataBlock | ( | int | sourceObjectID, |
int | destObjectID, | ||
const QString & | dataID = QString() ) |
Copies (potentially large) data, e.g. form's XML representation, referenced by sourceObjectID pointed by optional dataID.
- Returns
- true on success. Does not fail if blocks do not exist. Prior to copying, existing data blocks are removed even if there are no new blocks to copy. Copied data blocks will have destObjectID object identifier assigned. Note that if dataID is not specified, all data blocks found for the sourceObjectID will be copied.
Definition at line 2972 of file KDbConnection.cpp.
◆ copyTable() [1/2]
KDbTableSchema * KDbConnection::copyTable | ( | const KDbTableSchema & | tableSchema, |
const KDbObject & | newData ) |
Creates a copy of table schema defined by tableSchema with data. Name, caption and description will be copied from newData.
- Returns
- a table schema object. It is inserted into the KDbConnection structures and is owned by the KDbConnection object. The created table schema object should not be destroyed by hand afterwards. 0 is returned on failure. Table with destination name must not exist.
- See also
- createTable()
Definition at line 1519 of file KDbConnection.cpp.
◆ copyTable() [2/2]
KDbTableSchema * KDbConnection::copyTable | ( | const QString & | tableName, |
const KDbObject & | newData ) |
It is a convenience function, does exactly the same as KDbTableSchema *copyTable(const KDbTableSchema&, const KDbObject&).
Definition at line 1547 of file KDbConnection.cpp.
◆ createDatabase()
bool KDbConnection::createDatabase | ( | const QString & | dbName | ) |
Creates new database with name dbName, using this connection.
If database with dbName already exists, or other error occurred, false is returned. For file-based drivers, dbName should be equal to the database filename (the same as specified for KDbConnectionData).
See docs/kdb_issues.txt document, chapter "Table schema, query schema, etc. storage" for database schema documentation (detailed description of kexi__* 'system' tables).
- See also
- useDatabase()
Definition at line 695 of file KDbConnection.cpp.
◆ createTable()
bool KDbConnection::createTable | ( | KDbTableSchema * | tableSchema, |
CreateTableOptions | options = CreateTableOption::Default ) |
Creates a new table.
Creates a new table defined by tableSchema. true
is returned on success. In this case tableSchema object is added to KDbConnection's structures and becomes owned the KDbConnection object, so should not be destroyed by hand.
If options include the DropDestination value and table schema with the same name as tableSchema exists, it is dropped and the original identifier of the dropped schem is assigned to the tableSchema object.
If options do not include the DropDestination value and table schema with the same name as tableSchema exists, false
is returned.
Table and column definitions are added to "Kexi system" tables.
Prior to dropping the method checks if the table for the schema is in use, and if the new schema defines at least one column.
Note that on error:
- tableSchema is not inserted into KDbConnection's structures, so caller is still owner of the object,
- existing table schema object is not destroyed (e.g. it is still available for KDbConnection::tableSchema(const QString&), even if the table was physically dropped.
- Returns
- true on success.
- Todo
- (js) update any structure (e.g. queries) that depend on this table!
Definition at line 1397 of file KDbConnection.cpp.
◆ currentDatabase()
QString KDbConnection::currentDatabase | ( | ) | const |
Get the name of the current database.
- Returns
- name of currently used database for this connection or empty string if there is no used database
Definition at line 892 of file KDbConnection.cpp.
◆ data()
KDbConnectionData KDbConnection::data | ( | ) | const |
- Returns
- parameters that were used to create this connection.
Definition at line 484 of file KDbConnection.cpp.
◆ databaseExists()
bool KDbConnection::databaseExists | ( | const QString & | dbName, |
bool | ignoreErrors = true ) |
- Returns
- true if database dbName exists. If ignoreErrors is true, error flag of connection won't be modified for any errors (it will quietly return), else (ignoreErrors == false) we can check why the database does not exist using error(), errorNum() and/or errorMsg().
Definition at line 630 of file KDbConnection.cpp.
◆ databaseNames()
QStringList KDbConnection::databaseNames | ( | bool | also_system_db = false | ) |
- Returns
- list of database names for opened connection. If also_system_db is true, the system database names are also returned.
Definition at line 573 of file KDbConnection.cpp.
◆ databaseProperties()
KDbProperties KDbConnection::databaseProperties | ( | ) | const |
- Returns
- KDbProperties object allowing to read and write global database properties for this connection.
Definition at line 1059 of file KDbConnection.cpp.
◆ databaseVersion()
KDbVersionInfo KDbConnection::databaseVersion | ( | ) | const |
- Returns
- version information for this connection. If database is not used (i.e. isDatabaseUsed() is false) null KDbVersionInfo is returned. It can be compared to drivers' and KDb library version to maintain backward/upward compatiblility.
Definition at line 1054 of file KDbConnection.cpp.
◆ defaultTransaction()
KDbTransaction KDbConnection::defaultTransaction | ( | ) | const |
Returns handle of default transaction for this connection.
Null transaction is returned if there is no such a transaction declared. If transactions are supported any operation on database (e.g. inserts) that are started without specifying a transaction context, are be performed in the context of this transaction. Returned null transaction doesn't mean that there are no transactions started at all. Default transaction can be defined automatically for certain drivers, see beginTransaction().
Definition at line 2074 of file KDbConnection.cpp.
◆ deleteAllRecords()
|
protected |
Delete all existing records.
Definition at line 3430 of file KDbConnection.cpp.
◆ deleteCursor()
bool KDbConnection::deleteCursor | ( | KDbCursor * | cursor | ) |
Deletes cursor cursor previously created by functions like executeQuery() for this connection. There is an attempt to close the cursor with KDbCursor::close() if it was opened. Anyway, at last cursor is deleted.
- Returns
- true if cursor is properly closed before deletion.
Definition at line 2185 of file KDbConnection.cpp.
◆ deleteRecord()
|
protected |
Delete an existing record.
- Todo
- allow to delete from a table without pkey
Definition at line 3363 of file KDbConnection.cpp.
◆ destroy()
|
protected |
Method to be called form KDbConnection's subclass destructor.
- See also
- ~KDbConnection()
Definition at line 470 of file KDbConnection.cpp.
◆ disconnect()
bool KDbConnection::disconnect | ( | ) |
Disconnects from driver with given parameters.
The database (if used) is closed, and any active transactions (if supported) are rolled back, so commit these before disconnecting, if you'd like to save your changes.
Definition at line 531 of file KDbConnection.cpp.
◆ driver()
KDbDriver * KDbConnection::driver | ( | ) | const |
- Returns
- the driver used for this connection.
Definition at line 489 of file KDbConnection.cpp.
◆ dropDatabase()
Drops database with name dbName.
if dbName is not specified, currently used database name is used (it is closed before dropping).
Definition at line 920 of file KDbConnection.cpp.
◆ dropQuery() [1/2]
bool KDbConnection::dropQuery | ( | const QString & | queryName | ) |
It is a convenience function, does exactly the same as bool dropQuery( KDbQuerySchema* querySchema )
Definition at line 1833 of file KDbConnection.cpp.
◆ dropQuery() [2/2]
bool KDbConnection::dropQuery | ( | KDbQuerySchema * | querySchema | ) |
Drops a query defined by querySchema. If true is returned, schema information querySchema is destoyed (because it's owned), so don't keep this anymore!
- Todo
- update any structure that depend on this table!
Definition at line 1813 of file KDbConnection.cpp.
◆ dropTable() [1/2]
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. It is a convenience function.
Definition at line 1659 of file KDbConnection.cpp.
◆ dropTable() [2/2]
tristate KDbConnection::dropTable | ( | KDbTableSchema * | tableSchema | ) |
Drops a table defined by tableSchema (both table object as well as physically). If true is returned, schema information tableSchema is destoyed (because it's owned), so don't keep this anymore! No error is raised if the table does not exist physically
- its schema is removed even in this case.
Removes the table and column definitions in kexi__* "system schema" tables. First checks that the table is not a system table.
- Todo
Check that a database is currently in use? (c.f. createTable)
Update any structure (e.g. query) that depends on this table
Definition at line 1590 of file KDbConnection.cpp.
◆ dropTableInternal()
|
protected |
drops table tableSchema physically, but destroys tableSchema object only if alsoRemoveSchema is true. Used (alsoRemoveSchema==false) on table altering: if recreating table can fail we're giving up and keeping the original table schema (even if it is no longer points to any real data).
- Todo
- js: update any structure (e.g. queries) that depend on this table!
Definition at line 1595 of file KDbConnection.cpp.
◆ drv_afterInsert()
|
inlineprotectedvirtual |
Postprocessing (if any) required by drivers before execution of an Insert statement. Reimplement this method in your driver if there are any special processing steps to be executed after an Insert statement.
- See also
- drv_beforeInsert()
Reimplemented in KDbConnectionProxy, and SybaseConnection.
Definition at line 1231 of file KDbConnection.h.
◆ drv_afterUpdate()
|
inlineprotectedvirtual |
Postprocessing required by drivers before execution of an Insert statement. Reimplement this method in your driver if there are any special processing steps to be executed after an Update statement.
- See also
- drv_beforeUpdate()
Reimplemented in KDbConnectionProxy, and SybaseConnection.
Definition at line 1255 of file KDbConnection.h.
◆ drv_alterTableName()
|
protectedvirtual |
Alters table's described tableSchema name to newName. This is the default implementation, using "ALTER TABLE <oldname> RENAME TO <newname>", what's supported by SQLite >= 3.2, PostgreSQL, MySQL. Backends lacking ALTER TABLE, for example SQLite, reimplement this with by an inefficient data copying to a new table. In any case, renaming is performed at the backend. It's good idea to keep the operation within a transaction.
- Returns
- true on success.
Reimplemented in KDbConnectionProxy.
Definition at line 1798 of file KDbConnection.cpp.
◆ drv_beforeInsert()
|
inlineprotectedvirtual |
Preprocessing (if any) required by drivers before execution of an Insert statement. Reimplement this method in your driver if there are any special processing steps to be executed before an Insert statement.
- See also
- drv_afterInsert()
Reimplemented in KDbConnectionProxy, and SybaseConnection.
Definition at line 1219 of file KDbConnection.h.
◆ drv_beforeUpdate()
|
inlineprotectedvirtual |
Preprocessing required by drivers before execution of an Update statement. Reimplement this method in your driver if there are any special processing steps to be executed before an Update statement.
- See also
- drv_afterUpdate()
Reimplemented in KDbConnectionProxy, and SybaseConnection.
Definition at line 1243 of file KDbConnection.h.
◆ drv_beginTransaction()
|
protectedvirtual |
Note for driver developers: begins new transaction and returns handle to it. Default implementation just executes "BEGIN" sql statement and returns just empty data (KDbTransactionData object). Ownership of the returned object is passed to the caller.
Drivers that do not support transactions (see KDbDriver::features()) do never call this method. Reimplement this method if you need to do something more (e.g. if you driver will support multiple transactions per connection). Make subclass of KDbTransactionData (declared in KDbTransaction.h) and return object of this subclass. nullptr
should be returned on error. Do not check anything in connection (isConnected(), etc.) - all is already done.
- Todo
- Add support for nested transactions, e.g. KDbTransactionData* beginTransaction(KDbTransactionData *parent)
Reimplemented in KDbConnectionProxy.
Definition at line 2110 of file KDbConnection.cpp.
◆ drv_changeFieldProperty()
|
inlineprotectedvirtual |
This is a part of alter table interface implementing lower-level operations used to perform table schema altering. Used by KDbAlterTableHandler.
Changes value of field property.
- Returns
- true on success, false on failure, cancelled if the action has been cancelled.
Note for driver developers: implement this if the driver has to supprot the altering.
Reimplemented in SqliteConnection.
Definition at line 1408 of file KDbConnection.h.
◆ drv_closeDatabase()
|
protectedpure virtual |
For implementation: closes previously opened database using connection.
Implemented in KDbConnectionProxy, MysqlConnection, SqliteConnection, SybaseConnection, and xBaseConnection.
◆ drv_commitTransaction()
|
protectedvirtual |
Note for driver developers: begins new transaction and returns handle to it. Default implementation just executes "COMMIT" sql statement and returns true on success.
- See also
- drv_beginTransaction()
Reimplemented in KDbConnectionProxy.
Definition at line 2117 of file KDbConnection.cpp.
◆ drv_connect()
|
protectedpure virtual |
For implementation: connects to database.
- Returns
- true on success.
Implemented in KDbConnectionProxy, MysqlConnection, and SqliteConnection.
◆ drv_containsTable()
|
protectedpure virtual |
LOW LEVEL METHOD. For implementation: returns true if table with name tableName exists in the database.
- Returns
false
if it does not exist orcancelled
if error occurred. The lookup is case insensitive.
Implemented in KDbConnectionProxy, MysqlConnection, SqliteConnection, SybaseConnection, and xBaseConnection.
◆ drv_copyTableData()
|
protectedvirtual |
Copies table data from tableSchema to destinationTableSchema Default implementation executes "INSERT INTO .. SELECT * FROM .."
- Returns
- true on success.
Reimplemented in KDbConnectionProxy.
Definition at line 1559 of file KDbConnection.cpp.
◆ drv_createDatabase()
|
protectedpure virtual |
For implementation: creates new database using connection
Implemented in KDbConnectionProxy, MysqlConnection, SqliteConnection, SybaseConnection, and xBaseConnection.
◆ drv_createTable() [1/2]
|
protectedvirtual |
Creates table using tableSchema information.
- Returns
- true on success.
Default implementation builds a statement using createTableStatement() and calls executeSql(). Note for driver developers: reimplement this only to perform creation in other way.
Reimplemented in KDbConnectionProxy.
Definition at line 1874 of file KDbConnection.cpp.
◆ drv_createTable() [2/2]
|
protectedvirtual |
Creates table named by tableName. Schema object must be on schema tables' list before calling this method (otherwise false if returned). Just uses drv_createTable( const KDbTableSchema& tableSchema ). Used internally, e.g. in createDatabase().
- Returns
- true on success
Reimplemented in KDbConnectionProxy.
Definition at line 1885 of file KDbConnection.cpp.
◆ drv_databaseExists()
|
protectedvirtual |
For optional reimplementation: asks server if database dbName exists. This method is used internally in databaseExists(). The default implementation calls databaseNames and checks if that list contains dbName. If you need to ask the server specifically if a database exists, eg. if you can't retrieve a list of all available database names, please reimplement this method and do all needed checks.
See databaseExists() description for details about ignoreErrors argument. You should use it properly in your implementation.
Note: This method should also work if there is already database used (with useDatabase()); in this situation no changes should be made in current database selection.
Reimplemented in KDbConnectionProxy, and MysqlConnection.
Definition at line 613 of file KDbConnection.cpp.
◆ drv_disconnect()
|
protectedpure virtual |
For implementation: disconnects database
- Returns
- true on success.
Implemented in KDbConnectionProxy, MysqlConnection, SqliteConnection, SybaseConnection, and xBaseConnection.
◆ drv_dropDatabase()
|
protectedpure virtual |
For implementation: drops database from the server using connection. After drop, database shouldn't be accessible anymore.
Implemented in KDbConnectionProxy, MysqlConnection, SqliteConnection, SybaseConnection, and xBaseConnection.
◆ drv_dropTable()
|
protectedvirtual |
Physically drops table named with name. Default impelmentation executes "DROP TABLE.." command, so you rarely want to change this.
Reimplemented in KDbConnectionProxy.
Definition at line 1585 of file KDbConnection.cpp.
◆ drv_executeSql()
|
protectedpure virtual |
Executes query for a raw SQL statement sql without returning resulting records.
It is useful mostly for INSERT queries but it is possible to execute SELECT queries when returned records can be ignored. The @sql should be is valid and properly escaped.
- Note
- Only use this method if you really need.
- See also
- executeSql
Implemented in KDbConnectionProxy, MysqlConnection, SqliteConnection, SybaseConnection, and xBaseConnection.
◆ drv_getDatabasesList()
|
protectedvirtual |
For reimplementation: loads list of databases' names available for this connection and adds these names to list. If your server is not able to offer such a list, consider reimplementing drv_databaseExists() instead. The method should return true only if there was no error on getting database names list from the server. Default implementation puts empty list into list and returns true.
- See also
- databaseNames
Reimplemented in KDbConnectionProxy, MysqlConnection, SqliteConnection, SybaseConnection, and xBaseConnection.
Definition at line 607 of file KDbConnection.cpp.
◆ drv_getServerVersion()
|
protectedpure virtual |
For implementation: Sets version to real server's version. Depending on backend type this method is called after (if KDbDriverBehavior::USING_DATABASE_REQUIRED_TO_CONNECT is true) or before database is used (if KDbDriverBehavior::USING_DATABASE_REQUIRED_TO_CONNECT is false), i.e. for PostgreSQL it is called after. In any case it is called after successful drv_connect().
- Returns
- true on success.
Implemented in KDbConnectionProxy, MysqlConnection, and SqliteConnection.
◆ drv_getTableNames()
|
protectedvirtual |
LOW LEVEL METHOD.
Obtains a list containing names of all physical tables of this connection and returns it.
ok must not be nullptr
.
Default implementation covers functionality of SQL backends. It executes low-level SQL defined by KDbDriverBehavior::GET_TABLE_NAMES_SQL string. On failure of execution or if KDbDriverBehavior::GET_TABLE_NAMES_SQL is empty, ok is set to false
. On success ok is set to true
. Returning empty list is not an error.
If the database driver is not able to offer such a list, do not reimplement this method, it will just always return false and users of KDb will need to take this into account.
To reimplement the method, set ok to true
only on successfull obtaining of table names, and to false
otherwise.
This method is used by tableNames() to filter out tables names that have been found in project's metadata but lack related physical tables.
- Since
- 3.2
- See also
- tableNames()
Reimplemented in KDbConnectionProxy.
Definition at line 1845 of file KDbConnection.cpp.
◆ drv_isDatabaseUsed()
|
inlineprotectedvirtual |
- Returns
- true if internal driver's structure is still in opened/connected state and database is used. Note for driver developers: Put here every test that you can do using your internal engine's database API, eg (a bit schematic): my_connection_struct->isConnected()==true. Do not check things like KDbConnection::isDatabaseUsed() here or other things that "KDb already knows" at its level. If you cannot test anything, just leave default implementation (that returns true).
Result of this method is used as an additional chance to check for isDatabaseUsed(). Do not call this method from your driver's code, it should be used at KDb level only.
Reimplemented in KDbConnectionProxy.
Definition at line 1159 of file KDbConnection.h.
◆ drv_prepareSql()
|
protectedpure virtual |
Prepares query for a raw SQL statement sql with possibility of returning records.
It is useful mostly for SELECT queries. While INSERT queries do not return records, the KDbSqlResult object offers KDbSqlResult::lastInsertRecordId(). The @sql should be is valid and properly escaped. Only use this method if you really need. For low-level access to the results (without cursors). The result may be not stored (not buffered) yet. Use KDbSqlResult::fetchRecord() to fetch each record.
- Returns
- Null pointer if there is no proper result or error. Ownership of the returned object is passed to the caller.
- See also
- prepareSql
Implemented in KDbConnectionProxy, MysqlConnection, and SqliteConnection.
◆ drv_rollbackTransaction()
|
protectedvirtual |
Note for driver developers: begins new transaction and returns handle to it. Default implementation just executes "ROLLBACK" sql statement and returns true on success.
- See also
- drv_beginTransaction()
Reimplemented in KDbConnectionProxy.
Definition at line 2122 of file KDbConnection.cpp.
◆ drv_setAutoCommit()
|
protectedvirtual |
Changes autocommiting option for established connection.
- Returns
- true on success.
Note for driver developers: reimplement this only if your engine allows to set special auto commit option (like "SET AUTOCOMMIT=.." in MySQL). If not, auto commit behavior will be simulated if at least single transactions per connection are supported by the engine. Do not set any internal flags for autocommiting – it is already done inside setAutoCommit().
Default implementation does nothing with connection, just returns true.
Reimplemented in KDbConnectionProxy.
Definition at line 2127 of file KDbConnection.cpp.
◆ drv_useDatabase()
|
protectedpure virtual |
For implementation: opens existing database using connection
- Returns
- true on success, false on failure; sets cancelled to true if this action has been cancelled.
Implemented in KDbConnectionProxy, MysqlConnection, SqliteConnection, SybaseConnection, and xBaseConnection.
◆ escapeIdentifier()
Identifier escaping function in the associated KDbDriver.
Calls the identifier escaping function in this connection to escape table and column names. This should be used when explicitly constructing SQL strings (e.g. "FROM " + escapeIdentifier(tablename)). It should not be used for other functions (e.g. don't do useDatabase(escapeIdentifier(database))), because the identifier will be escaped when the called function generates, for example, "USE " + escapeIdentifier(database).
For efficiency, KDb "system" tables (prefixed with kexi__) and columns therein are not escaped - we assume these are valid identifiers for all drivers.
Use KDbEscapedString::isValid() to check if escaping has been performed successfully. Invalid strings are set to null in addition, that is KDbEscapedString::isNull() is true, not just isEmpty().
Reimplemented in KDbConnectionProxy.
Definition at line 3044 of file KDbConnection.cpp.
◆ escapeString()
|
virtual |
Connection-specific string escaping. Default implementation uses driver's escaping. Use KDbEscapedString::isValid() to check if escaping has been performed successfully. Invalid strings are set to null in addition, that is KDbEscapedString::isNull() is true, not just isEmpty().
Reimplemented in KDbConnectionProxy.
Definition at line 3535 of file KDbConnection.cpp.
◆ executeQuery() [1/4]
KDbCursor * KDbConnection::executeQuery | ( | const KDbEscapedString & | sql, |
KDbCursor::Options | options = KDbCursor::Option::None ) |
Executes SELECT query described by a raw SQL statement sql.
- Returns
- opened cursor created for results of this query or 0 if there was any error on the cursor creation or opening. Ownership of the returned object is passed to the caller. KDbCursor can have optionally applied options. Identifiers in sql that are the same as keywords in KDbSQL dialect or the backend's SQL have to be escaped.
Definition at line 2132 of file KDbConnection.cpp.
◆ executeQuery() [2/4]
KDbCursor * KDbConnection::executeQuery | ( | KDbQuerySchema * | query, |
const QList< QVariant > & | params, | ||
KDbCursor::Options | options = KDbCursor::Option::None ) |
Definition at line 2147 of file KDbConnection.cpp.
◆ executeQuery() [3/4]
KDbCursor * KDbConnection::executeQuery | ( | KDbQuerySchema * | query, |
KDbCursor::Options | options = KDbCursor::Option::None ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 2161 of file KDbConnection.cpp.
◆ executeQuery() [4/4]
KDbCursor * KDbConnection::executeQuery | ( | KDbTableSchema * | table, |
KDbCursor::Options | options = KDbCursor::Option::None ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Executes query described by query schema without parameters. Statement is build from data provided by table schema, it is like "select * from table_name".
Definition at line 2166 of file KDbConnection.cpp.
◆ executeQueryInternal()
|
protected |
used by *Internal() methods. Executes query based on a raw SQL statement sql or query with optional params. Ownership of the returned object is passed to the caller.
Definition at line 2335 of file KDbConnection.cpp.
◆ executeSql()
bool KDbConnection::executeSql | ( | const KDbEscapedString & | sql | ) |
Executes a new native (raw, backend-specific) SQL query.
The query is described by a raw statement sql which should be is valid and properly escaped. This method is a convenience version of prepareSql() that immediately starts and finalizes execution of a raw query in one step and provides a result. Use it for queries that do not return records, i.e. for queries that manipulate data (INSERT, UPDATE, DELETE, ...) or if the caller is not interested in the returned records.
- Note
- Only use this method if a non-portable raw query is required. In other cases use prepareQuery() or executeQuery() and the KDbCursor object.
Definition at line 1286 of file KDbConnection.cpp.
◆ findSystemFieldName()
KDbField * KDbConnection::findSystemFieldName | ( | const KDbFieldList & | fieldlist | ) |
- Returns
- first field from fieldlist that has system name,
nullptr
if there are no such field. For checking, KDbDriver::isSystemFieldName() is used, so this check can be driver-dependent.
Definition at line 1303 of file KDbConnection.cpp.
◆ insertRecord() [1/3]
QSharedPointer< KDbSqlResult > KDbConnection::insertRecord | ( | KDbFieldList * | fields, |
const QList< QVariant > & | values ) |
Definition at line 1232 of file KDbConnection.cpp.
◆ insertRecord() [2/3]
|
protected |
◆ insertRecord() [3/3]
C_INS_REC_ALL QSharedPointer< KDbSqlResult > KDbConnection::insertRecord | ( | KDbTableSchema * | tableSchema, |
const QList< QVariant > & | values ) |
Definition at line 1199 of file KDbConnection.cpp.
◆ insertRecordInternal()
|
protected |
used by insertRecord() methods.
Definition at line 1119 of file KDbConnection.cpp.
◆ isConnected()
bool KDbConnection::isConnected | ( | ) | const |
- Returns
- true, if connection is properly established.
Definition at line 546 of file KDbConnection.cpp.
◆ isDatabaseUsed()
bool KDbConnection::isDatabaseUsed | ( | ) | const |
- Returns
- true, both if connection is properly established and any database within this connection is properly used with useDatabase().
Definition at line 521 of file KDbConnection.cpp.
◆ isEmpty()
tristate KDbConnection::isEmpty | ( | KDbTableSchema * | table | ) |
- Returns
- true if there is at least one record in table.
Definition at line 2587 of file KDbConnection.cpp.
◆ isInternalTableSchema()
bool KDbConnection::isInternalTableSchema | ( | const QString & | tableName | ) |
Definition at line 3049 of file KDbConnection.cpp.
◆ kdbSystemTableNames()
|
static |
- Returns
- list of internal KDb system table names (kexi__*). This does not mean that these tables can be found in currently opened database. Just static list of table names is returned.
The list contents may depend on KDb library version; opened database can contain fewer 'system' tables than in current KDb implementation, if the current one is newer than the one used to build the database.
Definition at line 1044 of file KDbConnection.cpp.
◆ loadDataBlock()
tristate KDbConnection::loadDataBlock | ( | int | objectID, |
QString * | dataString, | ||
const QString & | dataID = QString() ) |
Loads (potentially large) data block (e.g. xml form's representation), referenced by objectID and puts it to dataString. The can be block indexed with optional dataID.
- Returns
- true on success, false on failure and cancelled when there is no such data block
- See also
- storeDataBlock().
Definition at line 2933 of file KDbConnection.cpp.
◆ loadExtendedTableSchemaData()
|
protected |
Loads extended schema information for table tableSchema, if present (see ExtendedTableSchemaInformation in Kexi Wiki).
- Returns
- true on success
- Todo
- look at the current format version (KDB_EXTENDED_TABLE_SCHEMA_VERSION)
- Todo
- more properties
- Todo
- more properties...
Definition at line 2751 of file KDbConnection.cpp.
◆ loadObjectData() [1/2]
Finds object data for object of type type and name name. If the object is found, resulted schema is stored in object and true is returned, otherwise false is returned.
Definition at line 2247 of file KDbConnection.cpp.
◆ loadObjectData() [2/2]
Finds object data for object of type type and identifier id. Added for convenience. If type is KDb::AnyObjectType, object type is ignored during the find.
- See also
- setupObjectData(const KDbRecordData*, KDbObject*).
- Returns
- true on success, false on failure and cancelled when such object couldn't be found.
- Since
- 3.1
Definition at line 2224 of file KDbConnection.cpp.
◆ objectIds()
QList< int > KDbConnection::objectIds | ( | int | objectType, |
bool * | ok = nullptr ) |
- Returns
- names of all schemas of object with objectType type that are stored in currently used database. If ok is not 0 then variable pointed by it will be set to the result.
- Note
- The fact that given id is on the returned list does not mean that the definition of the object is valid, so you have to double check this.
Only IDs of objects with names that are identifiers (checked using KDb::isIdentifier()) are returned.
- See also
- tableIds() queryIds()
Definition at line 1074 of file KDbConnection.cpp.
◆ objectNames()
QStringList KDbConnection::objectNames | ( | int | objectType = KDb::AnyObjectType, |
bool * | ok = nullptr ) |
- Returns
- names of all the objectType (see ObjectType in KDbGlobal.h) schemas stored in currently used database. KDb::AnyObjectType can be passed as objectType to get names of objects of any type. The list ordered is based on object identifiers. Only names that are identifiers (checked using KDb::isIdentifier()) are returned. If ok is not 0 then variable pointed by it will be set to the result. On error, the function returns empty list.
- See also
- kdbSystemTableNames() tableNames(int,bool*)
Definition at line 981 of file KDbConnection.cpp.
◆ options()
KDbConnectionOptions * KDbConnection::options | ( | ) |
- Returns
- generic options for a single connection. The options are accessible using key/value pairs. This enables extensibility depending on driver's type and version.
Definition at line 3504 of file KDbConnection.cpp.
◆ prepareQuery() [1/4]
|
pure virtual |
Prepares SELECT query described by a raw statement sql.
- Returns
- opened cursor created for results of this query or
nullptr
if there was any error. Ownership of the returned object is passed to the caller. KDbCursor can have optionally applied options (one of more selected from KDbCursor::Options). Preparation means that returned cursor is created but not opened. Open this when you would like to do it with KDbCursor::open().
Note for driver developers: you should initialize cursor engine-specific resources and return KDbCursor subclass' object (passing sql and options to its constructor).
Implemented in KDbConnectionProxy, MysqlConnection, and SqliteConnection.
◆ prepareQuery() [2/4]
KDbCursor * KDbConnection::prepareQuery | ( | KDbQuerySchema * | query, |
const QList< QVariant > & | params, | ||
KDbCursor::Options | options = KDbCursor::Option::None ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Prepares query described by query schema. params are values of parameters that will be inserted into places marked with [] before execution of the query.
Note for driver developers: you should initialize cursor engine-specific resources and return KDbCursor subclass' object (passing query and options to it's constructor). Kexi SQL and driver-specific escaping is performed on table names.
Definition at line 2176 of file KDbConnection.cpp.
◆ prepareQuery() [3/4]
|
pure virtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Prepares query described by query schema without parameters.
Implemented in KDbConnectionProxy, MysqlConnection, and SqliteConnection.
◆ prepareQuery() [4/4]
KDbCursor * KDbConnection::prepareQuery | ( | KDbTableSchema * | table, |
KDbCursor::Options | options = KDbCursor::Option::None ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Statement is build from data provided by table schema, it is like "select * from table_name".
Definition at line 2171 of file KDbConnection.cpp.
◆ prepareSql()
QSharedPointer< KDbSqlResult > KDbConnection::prepareSql | ( | const KDbEscapedString & | sql | ) |
Prepares execution of a new native (raw, backend-specific) SQL query.
The query is described by a raw statement sql which should be is valid and properly escaped. Access to results can be obtained using the returned KDbSqlResult object. The object is guarded with a shared pointer to facilitate transfer of ownership and memory management. A null pointer is returned if preparation of the query fails. Use KDbConnection::result() immediately after calling prepareSql() to obtain detailed result information about the preparation.
The returned object should be deleted before the database connection is closed. Connection object does not deletes the KDbSqlResult objects. It is also possible and recommended that caller deletes the KDbSqlResult object as soon as the result is not needed.
The returned object can be ignored if the query is not supposed to return records (e.g. manipulates data through INSERT, UPDATE, DELETE, ...) or the caller is not interested in the records. Thanks to the use of the shared pointer the object will be immediately deleted and execution will be finalized prior to that. However to execute queries that return no results, executeSql() is a better choice because of performance and easier reporting to results.
- Note
- Only use this method if a non-portable raw query is required. In other cases use prepareQuery() or executeQuery() and the KDbCursor object.
Definition at line 1280 of file KDbConnection.cpp.
◆ prepareStatement()
KDbPreparedStatement KDbConnection::prepareStatement | ( | KDbPreparedStatement::Type | type, |
KDbFieldList * | fields, | ||
const QStringList & | whereFieldNames = QStringList() ) |
Prepare an SQL statement and return a KDbPreparedStatement instance.
- Todo
- move to ConnectionInterface just like we moved execute() and prepare() to KDbPreparedStatementInterface...
Definition at line 3521 of file KDbConnection.cpp.
◆ prepareStatementInternal()
|
protectedpure virtual |
Prepare an SQL statement and return a KDbPreparedStatementInterface-derived object. Ownership of the returned object is passed to the caller.
Implemented in KDbConnectionProxy, MysqlConnection, SqliteConnection, and xBaseConnection.
◆ queryIds()
QList< int > KDbConnection::queryIds | ( | bool * | ok = nullptr | ) |
- Returns
- ids of all database query schemas stored in currently used database. These ids can be later used as argument for querySchema(). This is a shortcut for objectIds(KDb::QueryObjectType). If ok is not 0 then variable pointed by it will be set to the result.
- Note
- The fact that given id is on the returned list does not mean that querySchema( id ) returns anything. The query definition can be broken, so you have to double check this.
Only IDs of objects with names that are identifiers (checked using KDb::isIdentifier()) are returned.
- See also
- tableIds()
Definition at line 1069 of file KDbConnection.cpp.
◆ querySchema() [1/2]
KDbQuerySchema * KDbConnection::querySchema | ( | const QString & | queryName | ) |
- Returns
- schema of a query pointed by queryName, retrieved from currently used database.
- See also
- querySchema( int queryId )
Definition at line 3005 of file KDbConnection.cpp.
◆ querySchema() [2/2]
KDbQuerySchema * KDbConnection::querySchema | ( | int | queryId | ) |
- Returns
- schema of a query pointed by queryId, retrieved from currently used database. The schema is cached inside connection, so retrieval is performed only once, on demand.
Definition at line 3021 of file KDbConnection.cpp.
◆ querySingleNumber() [1/3]
tristate KDbConnection::querySingleNumber | ( | const KDbEscapedString & | sql, |
int * | number, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Convenience function: executes query for a raw SQL statement sql and stores first record's field's (number column) value inside number.
- See also
- querySingleString(). If options includes AddLimitTo1 value, "LIMIT 1" clause is added to the query (this is the default). Caller should make sure it is not there in the sql already.
- Returns
- true if query was successfully executed and first record has been found, false on data retrieving failure, and cancelled if there's no single record available.
Definition at line 2477 of file KDbConnection.cpp.
◆ querySingleNumber() [2/3]
tristate KDbConnection::querySingleNumber | ( | KDbQuerySchema * | query, |
int * | number, | ||
const QList< QVariant > & | params, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Accepts params as parameters that will be inserted into places marked with "[]" before query execution.
Definition at line 2489 of file KDbConnection.cpp.
◆ querySingleNumber() [3/3]
tristate KDbConnection::querySingleNumber | ( | KDbQuerySchema * | query, |
int * | number, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Uses a KDbQuerySchema object.
Definition at line 2483 of file KDbConnection.cpp.
◆ querySingleNumberInternal()
|
protected |
used by queryNumberString() methods.
Definition at line 2458 of file KDbConnection.cpp.
◆ querySingleRecord() [1/3]
tristate KDbConnection::querySingleRecord | ( | const KDbEscapedString & | sql, |
KDbRecordData * | data, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Executes query for a raw statement sql and stores first record's data inside data. This is a convenient method when we need only first record from query result, or when we know that query result has only one record. If options includes AddLimitTo1 value, "LIMIT 1" clause is added to the query (this is the default). Caller should make sure it is not there in the sql already.
- Returns
true
if query was successfully executed and first record has been found,false
on data retrieving failure, andcancelled
if there's no single record available.
Definition at line 2380 of file KDbConnection.cpp.
◆ querySingleRecord() [2/3]
tristate KDbConnection::querySingleRecord | ( | KDbQuerySchema * | query, |
KDbRecordData * | data, | ||
const QList< QVariant > & | params, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Accepts params as parameters that will be inserted into places marked with "[]" before query execution.
Definition at line 2392 of file KDbConnection.cpp.
◆ querySingleRecord() [3/3]
tristate KDbConnection::querySingleRecord | ( | KDbQuerySchema * | query, |
KDbRecordData * | data, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Uses a KDbQuerySchema object.
Definition at line 2386 of file KDbConnection.cpp.
◆ querySingleRecordInternal()
|
protected |
used by querySingleRecord() methods.
- Todo
- does not work with non-SQL data sources
Definition at line 2353 of file KDbConnection.cpp.
◆ querySingleString() [1/3]
tristate KDbConnection::querySingleString | ( | const KDbEscapedString & | sql, |
QString * | value, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Executes query for a raw statement sql and stores first record's field's (number column) string value inside value. For efficiency it's recommended that a query defined by sql should have just one field (SELECT one_field FROM ....). If options includes AddLimitTo1 value, "LIMIT 1" clause is added to the query (this is the default). Caller should make sure it is not there in the sql already.
- Returns
true
if query was successfully executed and first record has been found,false
on data retrieving failure, andcancelled
if there's no single record available.
- See also
- queryStringList()
Definition at line 2439 of file KDbConnection.cpp.
◆ querySingleString() [2/3]
tristate KDbConnection::querySingleString | ( | KDbQuerySchema * | query, |
QString * | value, | ||
const QList< QVariant > & | params, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Accepts params as parameters that will be inserted into places marked with [] before query execution.
Definition at line 2451 of file KDbConnection.cpp.
◆ querySingleString() [3/3]
tristate KDbConnection::querySingleString | ( | KDbQuerySchema * | query, |
QString * | value, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Uses a KDbQuerySchema object.
Definition at line 2445 of file KDbConnection.cpp.
◆ querySingleStringInternal()
|
protected |
used by querySingleString() methods.
- Todo
- does not work with non-SQL data sources
Definition at line 2408 of file KDbConnection.cpp.
◆ queryStringList() [1/3]
bool KDbConnection::queryStringList | ( | const KDbEscapedString & | sql, |
QStringList * | list, | ||
int | column = 0 ) |
Executes query for a raw SQL statement sql and stores Nth field's string value of every record inside list, where N is equal to column. The list is initially cleared. For efficiency it's recommended that a query defined by sql should have just one field (SELECT one_field FROM ....).
- Returns
- true if all values were fetched successfuly, false on data retrieving failure. Returning empty list can be still a valid result. On errors, the list is not cleared, it may contain a few retrieved values.
Definition at line 2539 of file KDbConnection.cpp.
◆ queryStringList() [2/3]
bool KDbConnection::queryStringList | ( | KDbQuerySchema * | query, |
QStringList * | list, | ||
const QList< QVariant > & | params, | ||
int | column = 0 ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Accepts params as parameters that will be inserted into places marked with "[]" before query execution.
Definition at line 2550 of file KDbConnection.cpp.
◆ queryStringList() [3/3]
bool KDbConnection::queryStringList | ( | KDbQuerySchema * | query, |
QStringList * | list, | ||
int | column = 0 ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Uses a QuerySchema object.
Definition at line 2545 of file KDbConnection.cpp.
◆ queryStringListInternal()
|
protected |
used by queryStringList() methods.
Definition at line 2496 of file KDbConnection.cpp.
◆ recentSqlString()
|
virtual |
Return recently used SQL string.
If there was a successful query execution it is equal to result().sql(), otherwise it is equal to result().errorSql().
Reimplemented in KDbConnectionProxy.
Definition at line 3531 of file KDbConnection.cpp.
◆ recordCount() [1/4]
int KDbConnection::recordCount | ( | const KDbEscapedString & | sql | ) |
Returns number of records returned by given SQL statement.
- Returns
- number of records that can be retrieved after executing sql statement within a connection conn. The statement should be of type SELECT. For SQL data sources it does not fetch any records, only "COUNT(*)" SQL aggregation is used at the backed. -1 is returned if any error occurred or if conn is
nullptr
.
- Since
- 3.1
- Todo
- perhaps use quint64 here?
Definition at line 3453 of file KDbConnection.cpp.
◆ recordCount() [2/4]
int KDbConnection::recordCount | ( | const KDbTableSchema & | tableSchema | ) |
Returns number of records that contains given table.
- Returns
- number of records that can be retrieved from tableSchema. To obtain the result the table must be created or retrieved using a KDbConnection object, i.e. tableSchema.connection() must not return
nullptr
. For SQL data sources only "COUNT(*)" SQL aggregation is used at the backed. -1 is returned if error occurred or if tableSchema.connection() isnullptr
.
- Since
- 3.1
- Todo
perhaps use quint64 here?
does not work with non-SQL data sources
- Todo
- does not work with non-SQL data sources
Definition at line 3464 of file KDbConnection.cpp.
◆ recordCount() [3/4]
int KDbConnection::recordCount | ( | KDbQuerySchema * | querySchema, |
const QList< QVariant > & | params = QList<QVariant>() ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.Operates on a query schema.
params are optional values of parameters that will be inserted into [] placeholders before execution of query that counts the records. To obtain the result the query must be created or retrieved using a KDbConnection object, i.e. querySchema->connection() must not return nullptr
. For SQL data sources only "COUNT(*)" SQL aggregation is used at the backed. -1 is returned if error occurred or if querySchema->connection() is nullptr
.
- Since
- 3.1
- Todo
- perhaps use quint64 here?
- Todo
- does not work with non-SQL data sources
Definition at line 3476 of file KDbConnection.cpp.
◆ recordCount() [4/4]
int KDbConnection::recordCount | ( | KDbTableOrQuerySchema * | tableOrQuery, |
const QList< QVariant > & | params = QList<QVariant>() ) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.Operates on a table or query schema.
params is a list of optional parameters that will be inserted into [] placeholders before execution of query that counts the records.
If tableOrQuery is nullptr
or provides neither table nor query, -1 is returned.
- Since
- 3.1
- Todo
- perhaps use quint64 here?
Definition at line 3493 of file KDbConnection.cpp.
◆ removeDataBlock()
Removes (potentially large) string data (e.g. xml form's representation), referenced by objectID, and pointed by optional dataID.
- Returns
- true on success. Does not fail if the block does not exist. Note that if dataID is not specified, all data blocks for the objectID will be removed.
Definition at line 2992 of file KDbConnection.cpp.
◆ removeMe()
|
protected |
Called by KDbTableSchema – signals destruction to KDbConnection object To avoid having deleted table object on its list.
Definition at line 3058 of file KDbConnection.cpp.
◆ removeObject()
bool KDbConnection::removeObject | ( | int | objId | ) |
Removes information about object with objId from internal "kexi__object" and "kexi__objectdata" tables.
- Returns
- true on success.
Definition at line 1568 of file KDbConnection.cpp.
◆ resultExists()
tristate KDbConnection::resultExists | ( | const KDbEscapedString & | sql, |
QueryRecordOptions | options = QueryRecordOption::Default ) |
- Returns
true
if there is at least one record has been returned by executing query for a raw SQL statement sql orfalse
if no such record exists. If options includes AddLimitTo1 value, the query is optimized into "SELECT 1 FROM (sql) LIMIT 1" (this is the default). Caller should make sure "SELECT 1" and "LIMIT 1" is not there in the sql already. This method does not fetch any records. On error returnscancelled
.
Definition at line 2556 of file KDbConnection.cpp.
◆ rollbackAutoCommitTransaction()
|
protected |
Internal, for handling autocommited transactions: Rolls back transaction prevoiusly started with beginAutoCommitTransaction().
- Returns
- true on success or when no transactions are supported at all by the driver.
Special case when used database driver has only single transaction support (KDbDriver::SingleTransactions): trans will not be rolled back if it has been started outside this KDbConnection object.
Definition at line 1937 of file KDbConnection.cpp.
◆ rollbackTransaction()
bool KDbConnection::rollbackTransaction | ( | KDbTransaction | trans = KDbTransaction(), |
KDbTransaction::CommitOptions | options = KDbTransaction::CommitOptions() ) |
Rolls back specified transaction for this connection.
If transaction is not active and default transaction (obtained from defaultTransaction()) exists, the default one will be rolled back. If neither default one is present true
is returned if IgnoreInactive is set for options or false
if IgnoreInactive is not set in options.
- Returns
false
on any error.
On successful rollback, transaction object will point to a null transaction. After rollong back a default transaction, there is no default transaction anymore.
Definition at line 2029 of file KDbConnection.cpp.
◆ serverVersion()
KDbServerVersionInfo KDbConnection::serverVersion | ( | ) | const |
- Returns
- server version information for this connection. If database is not connected (i.e. isConnected() is false) null KDbServerVersionInfo is returned.
Definition at line 1049 of file KDbConnection.cpp.
◆ setAutoCommit()
bool KDbConnection::setAutoCommit | ( | bool | on | ) |
Changes auto commit option. This does not affect currently started transactions. This option can be changed even when connection is not established.
- See also
- autoCommit()
Definition at line 2100 of file KDbConnection.cpp.
◆ setAvailableDatabaseName()
void KDbConnection::setAvailableDatabaseName | ( | const QString & | dbName | ) |
Sets dbName as name of a database that can be accessible. This is option that e.g. application that make use of KDb library can set to tune connection's behavior when it needs to temporary connect to any database in the server to do some work. You can pass empty dbName - then anyAvailableDatabaseName() will try return KDbDriverBehavior::ALWAYS_AVAILABLE_DATABASE_NAME (the default) value instead of the one previously set with setAvailableDatabaseName().
- See also
- anyAvailableDatabaseName()
Definition at line 3073 of file KDbConnection.cpp.
◆ setDefaultTransaction()
void KDbConnection::setDefaultTransaction | ( | const KDbTransaction & | trans | ) |
Sets default transaction.
Default transaction is used as a context for data modifications for this connection when no specific transaction is provided.
Definition at line 2079 of file KDbConnection.cpp.
◆ setQuerySchemaObsolete()
bool KDbConnection::setQuerySchemaObsolete | ( | const QString & | queryName | ) |
Sets queryName query obsolete by moving it out of the query sets, so it will not be accessible by querySchema( const QString& queryName ). The existing query object is not destroyed, to avoid problems when it's referenced. In this case, a new query schema will be retrieved directly from the backend.
For now it's used in KexiQueryDesignerGuiEditor::storeLayout(). This solves the problem when user has changed a query schema but already form still uses previously instantiated query schema.
- Returns
- true if there is such query. Otherwise the method does nothing.
Definition at line 3035 of file KDbConnection.cpp.
◆ setupField()
|
protected |
- Returns
- a new field table schema for a table retrieved from data. Ownership of the returned object is passed to the caller. Used internally by tableSchema().
- Todo
- load maxLengthStrategy info to see if the maxLength is the default
Definition at line 2855 of file KDbConnection.cpp.
◆ setupObjectData()
|
protected |
Setups data for object that owns object (e.g. table, query) opened on 'kexi__objects' table, pointing to a record corresponding to given object.
- Todo
- IMPORTANT: fix KDbConnection::setupObjectData() after refactoring
Definition at line 2199 of file KDbConnection.cpp.
◆ storeDataBlock()
bool KDbConnection::storeDataBlock | ( | int | objectID, |
const QString & | dataString, | ||
const QString & | dataID = QString() ) |
Stores (potentially large) data block dataString (e.g. xml form's representation), referenced by objectID. Block will be stored in "kexi__objectdata" table and an optional dataID identifier. If there is already such record in the table, it's simply overwritten.
- Returns
- true on success
Definition at line 2946 of file KDbConnection.cpp.
◆ storeExtendedTableSchemaData()
|
protected |
Stores extended schema information for table tableSchema, (see ExtendedTableSchemaInformation in Kexi Wiki). The action is performed within the current transaction, so it's up to you to commit. Used, e.g. by createTable(), within its transaction.
- Returns
- true on success
- Todo
- future: save in older versions if neeed
Definition at line 2680 of file KDbConnection.cpp.
◆ storeMainFieldSchema()
|
protected |
Stores main field's schema information for field field. Used in table altering code when information in kexi__fields has to be updated.
- Returns
- true on success and false on failure.
Definition at line 1363 of file KDbConnection.cpp.
◆ storeNewObjectData()
bool KDbConnection::storeNewObjectData | ( | KDbObject * | object | ) |
Stores new entry for object (id, name, caption, description) described by object on the backend. If object.id() was less than 0, new, unique object identifier is obtained and assigned to object (see KDbObject::id()).
- Returns
- true on success.
Definition at line 2324 of file KDbConnection.cpp.
◆ storeObjectData()
bool KDbConnection::storeObjectData | ( | KDbObject * | object | ) |
Stores object (id, name, caption, description) described by object on the backend. It is expected that entry on the backend already exists, so it's updated. Changes to identifier attribute are not allowed.
- Returns
- true on success.
Definition at line 2319 of file KDbConnection.cpp.
◆ tableIds()
QList< int > KDbConnection::tableIds | ( | bool * | ok = nullptr | ) |
- Returns
- ids of all table schema names stored in currently used database. These ids can be later used as argument for tableSchema(). This is a shortcut for objectIds(KDb::TableObjectType). Internal KDb system tables (kexi__*) are not available here because these have no identifiers assigned (more formally: id=-1). If ok is not 0 then variable pointed by it will be set to the result.
- Note
- The fact that given id is on the returned list does not mean that tableSchema( id ) returns anything. The table definition can be broken, so you have to double check this.
Only IDs of objects with names that are identifiers (checked using KDb::isIdentifier()) are returned.
- See also
- queryIds()
Definition at line 1064 of file KDbConnection.cpp.
◆ tableNames()
QStringList KDbConnection::tableNames | ( | bool | alsoSystemTables = false, |
bool * | ok = nullptr ) |
- Returns
- names of all table schemas stored in currently used database. If alsoSystemTables is true, internal KDb system table names (kexi__*) are also returned. The list ordered is based on object identifiers. Only names that are identifiers (checked using KDb::isIdentifier()) are returned. If ok is not 0 then variable pointed by it will be set to the result. On error, the function returns empty list.
Definition at line 1007 of file KDbConnection.cpp.
◆ tableSchema() [1/2]
KDbTableSchema * KDbConnection::tableSchema | ( | const QString & | tableName | ) |
- Returns
- schema of a table pointed by tableName, retrieved from currently used database. KDb system table schema can be also retrieved.
- See also
- tableSchema( int tableId )
Definition at line 2904 of file KDbConnection.cpp.
◆ tableSchema() [2/2]
KDbTableSchema * KDbConnection::tableSchema | ( | int | tableId | ) |
- Returns
- schema of a table pointed by tableId, retrieved from currently used database. The schema is cached inside connection, so retrieval is performed only once, on demand.
Definition at line 2919 of file KDbConnection.cpp.
◆ takeCursor()
|
protected |
Used by KDbCursor class.
Definition at line 3514 of file KDbConnection.cpp.
◆ transactions()
QList< KDbTransaction > KDbConnection::transactions | ( | ) |
Returns set of handles of currently active transactions.
- Note
- In multithreading environment some of these transactions can be already inactive after calling this method. Use KDbTransaction::isActive() to check that. Inactive transaction handle is useless and can be safely ignored.
Definition at line 2090 of file KDbConnection.cpp.
◆ updateRecord()
|
protected |
Update a record.
- Todo
- perhaps we can try to update without using PKEY?
Definition at line 3098 of file KDbConnection.cpp.
◆ useDatabase()
bool KDbConnection::useDatabase | ( | const QString & | dbName = QString(), |
bool | kexiCompatible = true, | ||
bool * | cancelled = nullptr, | ||
KDbMessageHandler * | msgHandler = nullptr ) |
Opens an existing database specified by dbName.
If kexiCompatible is true (the default) initial checks will be performed to recognize database Kexi-specific format. Set kexiCompatible to false if you're using native database (one that have no Kexi System tables). For file-based drivers, dbName can be skipped, so the same as specified for KDbConnectionData is used.
- Returns
- true on success, false on failure. If user has cancelled this action and cancelled is not 0, *cancelled is set to true.
Definition at line 787 of file KDbConnection.cpp.
◆ useTemporaryDatabaseIfNeeded()
bool KDbConnection::useTemporaryDatabaseIfNeeded | ( | QString * | name | ) |
Because some engines need to have opened any database before executing administrative SQL statements like "create database" or "drop database", this method is used to use appropriate, existing database for this connection. For file-based db drivers this always return true and does not set name to any value. For other db drivers: this sets name to db name computed using anyAvailableDatabaseName(), and if the name computed is empty, false is returned; if it is not empty, useDatabase() is called. False is returned also when useDatabase() fails. You can call this method from your application's level if you really want to perform tasks that require any used database. In such a case don't forget to closeDatabase() if returned name is not empty.
Note: This method has nothing to do with creating or using temporary databases in such meaning that these database are not persistent
Definition at line 897 of file KDbConnection.cpp.
Member Data Documentation
◆ H_INS_REC_ALL
KDbConnection::H_INS_REC_ALL |
Definition at line 607 of file KDbConnection.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.