SqliteConnection
#include <SqliteConnection.h>
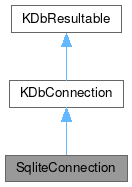
Protected Attributes | |
SqliteConnectionInternal * | d |
![]() | |
Private *const | d |
KDbResult | m_result |
Additional Inherited Members | |
![]() | |
enum class | AlterTableNameOption { Default = 0 , DropDestination = 1 } |
typedef QFlags< AlterTableNameOption > | AlterTableNameOptions |
enum class | CreateTableOption { Default = 0 , DropDestination = 1 } |
typedef QFlags< CreateTableOption > | CreateTableOptions |
enum class | QueryRecordOption { AddLimitTo1 = 1 , Default = AddLimitTo1 } |
typedef QFlags< QueryRecordOption > | QueryRecordOptions |
![]() | |
static QStringList | kdbSystemTableNames () |
![]() | |
H_INS_REC_ALL | |
Detailed Description
SQLite-specific connection Following connection options are supported (see KDbConnectionOptions):
- extraSqliteExtensionPaths (read/write, QStringList): adds extra seach paths for SQLite extensions. Set them before KDbConnection::useDatabase() is called. Absolute paths are recommended.
Definition at line 36 of file SqliteConnection.h.
Constructor & Destructor Documentation
◆ ~SqliteConnection()
|
override |
Definition at line 52 of file SqliteConnection.cpp.
◆ SqliteConnection()
|
protected |
Used by driver
Definition at line 39 of file SqliteConnection.cpp.
Member Function Documentation
◆ changeFieldType()
|
protected |
From https://sqlite.org/datatype3.html : Version 3 enhances provides the ability to store integer and real numbers in a more compact format and the capability to store BLOB data.
Each value stored in an SQLite database (or manipulated by the database engine) has one of the following storage classes: NULL. The value is a NULL value. INTEGER. The value is a signed integer, stored in 1, 2, 3, 4, 6, or 8 bytes depending on the magnitude of the value. REAL. The value is a floating point value, stored as an 8-byte IEEE floating point number. TEXT. The value is a text string, stored using the database encoding (UTF-8, UTF-16BE or UTF-16-LE). BLOB. The value is a blob of data, stored exactly as it was input.
Column Affinity In SQLite version 3, the type of a value is associated with the value itself, not with the column or variable in which the value is stored. .The type affinity of a column is the recommended type for data stored in that column.
See alter_table_type_conversions.ods for details.
Definition at line 98 of file SqliteAlter.cpp.
◆ drv_changeFieldProperty()
|
overrideprotectedvirtual |
This is a part of alter table interface implementing lower-level operations used to perform table schema altering. Used by KDbAlterTableHandler.
Changes value of field property.
- Returns
- true on success, false on failure, cancelled if the action has been cancelled.
Note for driver developers: implement this if the driver has to supprot the altering.
- Todo
- msg
Reimplemented from KDbConnection.
Definition at line 61 of file SqliteAlter.cpp.
◆ drv_closeDatabase()
|
overrideprotectedvirtual |
For implementation: closes previously opened database using connection.
Implements KDbConnection.
Definition at line 247 of file SqliteConnection.cpp.
◆ drv_connect()
|
overrideprotectedvirtual |
For implementation: connects to database.
- Returns
- true on success.
Implements KDbConnection.
Definition at line 63 of file SqliteConnection.cpp.
◆ drv_containsTable()
- Todo
- move this somewhere to low level class (MIGRATION?)
Implements KDbConnection.
Definition at line 93 of file SqliteConnection.cpp.
◆ drv_createDatabase()
|
overrideprotectedvirtual |
Creates new database using connection. Note: Do not pass dbName arg because for file-based engine (that has one database per connection) it is defined during connection.
Implements KDbConnection.
Definition at line 121 of file SqliteConnection.cpp.
◆ drv_disconnect()
|
overrideprotectedvirtual |
For implementation: disconnects database
- Returns
- true on success.
Implements KDbConnection.
Definition at line 81 of file SqliteConnection.cpp.
◆ drv_dropDatabase()
|
overrideprotectedvirtual |
Drops database from the server using connection. After drop, database shouldn't be accessible anymore, so database file is just removed. See note from drv_useDatabase().
Implements KDbConnection.
Definition at line 267 of file SqliteConnection.cpp.
◆ drv_executeSql()
|
overrideprotectedvirtual |
Executes query for a raw SQL statement sql without returning resulting records.
It is useful mostly for INSERT queries but it is possible to execute SELECT queries when returned records can be ignored. The @sql should be is valid and properly escaped.
- Note
- Only use this method if you really need.
- See also
- executeSql
Implements KDbConnection.
Definition at line 321 of file SqliteConnection.cpp.
◆ drv_getDatabasesList()
|
overrideprotectedvirtual |
For reimplementation: loads list of databases' names available for this connection and adds these names to list. If your server is not able to offer such a list, consider reimplementing drv_databaseExists() instead. The method should return true only if there was no error on getting database names list from the server. Default implementation puts empty list into list and returns true.
- See also
- databaseNames
Reimplemented from KDbConnection.
Definition at line 86 of file SqliteConnection.cpp.
◆ drv_getServerVersion()
|
overrideprotectedvirtual |
For implementation: Sets version to real server's version. Depending on backend type this method is called after (if KDbDriverBehavior::USING_DATABASE_REQUIRED_TO_CONNECT is true) or before database is used (if KDbDriverBehavior::USING_DATABASE_REQUIRED_TO_CONNECT is false), i.e. for PostgreSQL it is called after. In any case it is called after successful drv_connect().
- Returns
- true on success.
Implements KDbConnection.
Definition at line 68 of file SqliteConnection.cpp.
◆ drv_prepareSql()
|
overrideprotectedvirtual |
Prepares query for a raw SQL statement sql with possibility of returning records.
It is useful mostly for SELECT queries. While INSERT queries do not return records, the KDbSqlResult object offers KDbSqlResult::lastInsertRecordId(). The @sql should be is valid and properly escaped. Only use this method if you really need. For low-level access to the results (without cursors). The result may be not stored (not buffered) yet. Use KDbSqlResult::fetchRecord() to fetch each record.
- Returns
- Null pointer if there is no proper result or error. Ownership of the returned object is passed to the caller.
- See also
- prepareSql
Implements KDbConnection.
Definition at line 292 of file SqliteConnection.cpp.
◆ drv_useDatabase()
|
overrideprotectedvirtual |
Opens existing database using connection. Do not pass dbName arg because for file-based engine (that has one database per connection) it is defined during connection. If you pass it, database file name will be changed.
Implements KDbConnection.
Definition at line 127 of file SqliteConnection.cpp.
◆ prepareQuery() [1/2]
|
overridevirtual |
Prepares SELECT query described by a raw statement sql.
- Returns
- opened cursor created for results of this query or
nullptr
if there was any error. Ownership of the returned object is passed to the caller. KDbCursor can have optionally applied options (one of more selected from KDbCursor::Options). Preparation means that returned cursor is created but not opened. Open this when you would like to do it with KDbCursor::open().
Note for driver developers: you should initialize cursor engine-specific resources and return KDbCursor subclass' object (passing sql and options to its constructor).
Implements KDbConnection.
Definition at line 282 of file SqliteConnection.cpp.
◆ prepareQuery() [2/2]
|
overridevirtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Prepares query described by query schema without parameters.
Implements KDbConnection.
Definition at line 287 of file SqliteConnection.cpp.
◆ prepareStatementInternal()
|
overridevirtual |
Prepare an SQL statement and return a KDbPreparedStatementInterface-derived object. Ownership of the returned object is passed to the caller.
Implements KDbConnection.
Definition at line 356 of file SqliteConnection.cpp.
◆ serverResultName()
|
overrideprotectedvirtual |
Implemented for KDbResultable.
Reimplemented from KDbResultable.
Definition at line 351 of file SqliteConnection.cpp.
◆ storeResult()
|
protected |
Definition at line 58 of file SqliteConnection.cpp.
Member Data Documentation
◆ d
|
protected |
Definition at line 103 of file SqliteConnection.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.