KDbPreparedStatement
#include <KDbPreparedStatement.h>
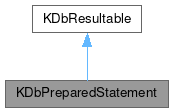
Classes | |
class | Data |
Public Types | |
enum | Type { InvalidStatement , SelectStatement , InsertStatement } |
Public Member Functions | |
KDbPreparedStatement () | |
bool | execute (const KDbPreparedStatementParameters ¶meters) |
const KDbFieldList * | fields () const |
bool | isValid () const |
quint64 | lastInsertRecordId () const |
void | setFields (KDbFieldList *fields) |
void | setType (KDbPreparedStatement::Type type) |
void | setWhereFieldNames (const QStringList &whereFieldNames) |
KDbPreparedStatement::Type | type () const |
QStringList | whereFieldNames () const |
![]() | |
KDbResultable (const KDbResultable &other) | |
void | clearResult () |
KDbMessageHandler * | messageHandler () const |
KDbResultable & | operator= (const KDbResultable &other) |
KDbResult | result () const |
virtual QString | serverResultName () const |
void | setMessageHandler (KDbMessageHandler *handler) |
void | showMessage () |
Protected Member Functions | |
KDbPreparedStatement (KDbPreparedStatementInterface *iface, Type type, KDbFieldList *fields, const QStringList &whereFieldNames=QStringList()) | |
Additional Inherited Members | |
![]() | |
Private *const | d |
KDbResult | m_result |
Detailed Description
Prepared database command for optimizing sequences of multiple database actions.
Currently INSERT and SELECT statements are supported. For example when using KDbPreparedStatement for INSERTs, you can gain about 30% speedup compared to using multiple connection.insertRecord(*tabelSchema, dbRecordBuffer).
To use KDbPreparedStatement, create is using KDbConnection:prepareStatement(), providing table schema; set up parameters using operator << ( const QVariant& value ); and call execute() when ready. KDbPreparedStatement objects are accessed using KDE shared pointers, i.e KDbPreparedStatement, so you do not need to remember about destroying them. However, when underlying KDbConnection object is destroyed, KDbPreparedStatement should not be used.
Let's assume tableSchema contains two columns NUMBER integer and TEXT text. Following code inserts 10000 records with random numbers and text strings obtained elsewhere using getText(i).
If you do not call clearParameters() after every insert, you can insert the same value multiple times using execute() what increases efficiency even more.
Another use case is inserting large objects (BLOBs or CLOBs). Depending on database backend, you can avoid escaping BLOBs. See KexiFormView::storeData() for example use.
Definition at line 75 of file KDbPreparedStatement.h.
Member Enumeration Documentation
◆ Type
Defines type of the prepared statement.
Enumerator | |
---|---|
InvalidStatement | Used only in invalid statements. |
SelectStatement | SELECT statement will be prepared end executed. |
InsertStatement | INSERT statement will be prepared end executed. |
Definition at line 80 of file KDbPreparedStatement.h.
Constructor & Destructor Documentation
◆ KDbPreparedStatement() [1/2]
KDbPreparedStatement::KDbPreparedStatement | ( | ) |
Creates an invalid prepared statement.
Definition at line 46 of file KDbPreparedStatement.cpp.
◆ ~KDbPreparedStatement()
|
override |
Definition at line 58 of file KDbPreparedStatement.cpp.
◆ KDbPreparedStatement() [2/2]
|
protected |
Creates a new prepared statement.
In your code use Users call KDbConnection:prepareStatement() instead.
Definition at line 51 of file KDbPreparedStatement.cpp.
Member Function Documentation
◆ execute()
bool KDbPreparedStatement::execute | ( | const KDbPreparedStatementParameters & | parameters | ) |
Executes the prepared statement using parameters parameters. A number parameters set up for the statement must be the same as a number of fields defined in the underlying database table.
- Returns
- false on failure. Detailed error status can be obtained from KDbConnection object that was used to create this statement object.
- Todo
- error message?
Definition at line 62 of file KDbPreparedStatement.cpp.
◆ fields()
const KDbFieldList * KDbPreparedStatement::fields | ( | ) | const |
Definition at line 189 of file KDbPreparedStatement.cpp.
◆ isValid()
bool KDbPreparedStatement::isValid | ( | ) | const |
Definition at line 173 of file KDbPreparedStatement.cpp.
◆ lastInsertRecordId()
quint64 KDbPreparedStatement::lastInsertRecordId | ( | ) | const |
- Returns
- unique identifier of the most recently inserted record. Typically this is just primary key value. This identifier could be reused when we want to reference just inserted record. If there was no insertion recently performed, std::numeric_limits<quint64>::max() is returned.
Definition at line 213 of file KDbPreparedStatement.cpp.
◆ setFields()
void KDbPreparedStatement::setFields | ( | KDbFieldList * | fields | ) |
Sets fields for the statement. Does nothing if fields is nullptr
.
Definition at line 194 of file KDbPreparedStatement.cpp.
◆ setType()
void KDbPreparedStatement::setType | ( | KDbPreparedStatement::Type | type | ) |
Definition at line 183 of file KDbPreparedStatement.cpp.
◆ setWhereFieldNames()
void KDbPreparedStatement::setWhereFieldNames | ( | const QStringList & | whereFieldNames | ) |
Definition at line 207 of file KDbPreparedStatement.cpp.
◆ type()
KDbPreparedStatement::Type KDbPreparedStatement::type | ( | ) | const |
Definition at line 178 of file KDbPreparedStatement.cpp.
◆ whereFieldNames()
QStringList KDbPreparedStatement::whereFieldNames | ( | ) | const |
Definition at line 202 of file KDbPreparedStatement.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:54:34 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.