KDbConnectionProxy
#include <KDbConnectionProxy.h>
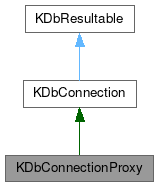
Public Attributes | |
H_INS_REC_ALL | |
Detailed Description
The KDbConnectionProxy class gives access to protected (low-level) API of KDbConnection.
The connection object specified in constructor of the proxy is called a parent connection. All inherited methods of the KDbConnection API call equivalent methods of the parent connection. The KDbConnectionProxy class also provides non-virtual methods that are equivalent to KDbConnection ones. These KDbConnection's equivalent methods are called by the proxy too.
Example use of this class is Kexi's database database migration plugins when the source database is only accessibly using low-level routines.
Definition at line 36 of file KDbConnectionProxy.h.
Constructor & Destructor Documentation
◆ KDbConnectionProxy()
|
explicit |
Creates a proxy object for parent connection.
connection must not be nullptr
. It is owned by this proxy unless setConnectionIsOwned(false) is called.
Definition at line 44 of file KDbConnectionProxy.cpp.
◆ ~KDbConnectionProxy()
|
override |
Deletes this proxy. Owned connection is closed and destroyed.
Definition at line 52 of file KDbConnectionProxy.cpp.
Member Function Documentation
◆ alterTable()
tristate KDbConnectionProxy::alterTable | ( | KDbTableSchema * | tableSchema, |
KDbTableSchema * | newTableSchema ) |
Definition at line 466 of file KDbConnectionProxy.cpp.
◆ alterTableName()
bool KDbConnectionProxy::alterTableName | ( | KDbTableSchema * | tableSchema, |
const QString & | newName, | ||
AlterTableNameOptions | options = AlterTableNameOption::Default ) |
Definition at line 471 of file KDbConnectionProxy.cpp.
◆ anyAvailableDatabaseName()
|
overridevirtual |
- Returns
- name of any (e.g. first found) database for this connection. This method does not close or open this connection. The method can be used (it is also internally used, e.g. for database dropping) when we need a database name before we can connect and execute any SQL statement (e.g. DROP DATABASE).
The method can return nul lstring, but in this situation no automatic (implicit) connections could be made, what is useful by e.g. dropDatabase().
Note for driver developers: return here a name of database which you are sure is existing. Default implementation returns:
- value that previously had been set using setAvailableDatabaseName() for this connection, if it is not empty
- else (2nd priority): value of KDbDriverBehavior::ALWAYS_AVAILABLE_DATABASE_NAME if it is not empty.
See description of KDbDriverBehavior::ALWAYS_AVAILABLE_DATABASE_NAME member. You may want to reimplement this method only when you need to depend on this connection specifics (e.g. you need to check something remotely).
Reimplemented from KDbConnection.
Definition at line 497 of file KDbConnectionProxy.cpp.
◆ autoCommit()
bool KDbConnectionProxy::autoCommit | ( | ) | const |
Definition at line 235 of file KDbConnectionProxy.cpp.
◆ beginAutoCommitTransaction()
bool KDbConnectionProxy::beginAutoCommitTransaction | ( | KDbTransactionGuard * | tg | ) |
Definition at line 737 of file KDbConnectionProxy.cpp.
◆ beginTransaction()
KDbTransaction KDbConnectionProxy::beginTransaction | ( | ) |
Definition at line 203 of file KDbConnectionProxy.cpp.
◆ checkConnected()
bool KDbConnectionProxy::checkConnected | ( | ) |
Definition at line 752 of file KDbConnectionProxy.cpp.
◆ checkIfColumnExists()
bool KDbConnectionProxy::checkIfColumnExists | ( | KDbCursor * | cursor, |
int | column ) |
Definition at line 782 of file KDbConnectionProxy.cpp.
◆ checkIsDatabaseUsed()
bool KDbConnectionProxy::checkIsDatabaseUsed | ( | ) |
Definition at line 757 of file KDbConnectionProxy.cpp.
◆ clearResult()
void KDbConnectionProxy::clearResult | ( | ) |
Definition at line 102 of file KDbConnectionProxy.cpp.
◆ closeDatabase()
bool KDbConnectionProxy::closeDatabase | ( | ) |
Definition at line 143 of file KDbConnectionProxy.cpp.
◆ commitAutoCommitTransaction()
bool KDbConnectionProxy::commitAutoCommitTransaction | ( | const KDbTransaction & | trans | ) |
Definition at line 742 of file KDbConnectionProxy.cpp.
◆ commitTransaction()
bool KDbConnectionProxy::commitTransaction | ( | KDbTransaction | trans = KDbTransaction(), |
KDbTransaction::CommitOptions | options = KDbTransaction::CommitOptions() ) |
Definition at line 208 of file KDbConnectionProxy.cpp.
◆ connect()
bool KDbConnectionProxy::connect | ( | ) |
Definition at line 82 of file KDbConnectionProxy.cpp.
◆ containsTable()
Definition at line 168 of file KDbConnectionProxy.cpp.
◆ copyDataBlock()
bool KDbConnectionProxy::copyDataBlock | ( | int | sourceObjectID, |
int | destObjectID, | ||
const QString & | dataID = QString() ) |
Definition at line 553 of file KDbConnectionProxy.cpp.
◆ copyTable() [1/2]
KDbTableSchema * KDbConnectionProxy::copyTable | ( | const KDbTableSchema & | tableSchema, |
const KDbObject & | newData ) |
Definition at line 446 of file KDbConnectionProxy.cpp.
◆ copyTable() [2/2]
KDbTableSchema * KDbConnectionProxy::copyTable | ( | const QString & | tableName, |
const KDbObject & | newData ) |
Definition at line 451 of file KDbConnectionProxy.cpp.
◆ createDatabase()
bool KDbConnectionProxy::createDatabase | ( | const QString & | dbName | ) |
Definition at line 132 of file KDbConnectionProxy.cpp.
◆ createTable()
bool KDbConnectionProxy::createTable | ( | KDbTableSchema * | tableSchema, |
CreateTableOptions | options = CreateTableOption::Default ) |
Definition at line 441 of file KDbConnectionProxy.cpp.
◆ currentDatabase()
QString KDbConnectionProxy::currentDatabase | ( | ) | const |
Definition at line 148 of file KDbConnectionProxy.cpp.
◆ data()
KDbConnectionData KDbConnectionProxy::data | ( | ) | const |
Definition at line 72 of file KDbConnectionProxy.cpp.
◆ databaseExists()
bool KDbConnectionProxy::databaseExists | ( | const QString & | dbName, |
bool | ignoreErrors = true ) |
Definition at line 127 of file KDbConnectionProxy.cpp.
◆ databaseNames()
QStringList KDbConnectionProxy::databaseNames | ( | bool | also_system_db = false | ) |
Definition at line 122 of file KDbConnectionProxy.cpp.
◆ databaseProperties()
KDbProperties KDbConnectionProxy::databaseProperties | ( | ) | const |
Definition at line 183 of file KDbConnectionProxy.cpp.
◆ databaseVersion()
KDbVersionInfo KDbConnectionProxy::databaseVersion | ( | ) | const |
Definition at line 178 of file KDbConnectionProxy.cpp.
◆ defaultTransaction()
KDbTransaction KDbConnectionProxy::defaultTransaction | ( | ) | const |
Definition at line 220 of file KDbConnectionProxy.cpp.
◆ deleteAllRecords()
bool KDbConnectionProxy::deleteAllRecords | ( | KDbQuerySchema * | query | ) |
Definition at line 777 of file KDbConnectionProxy.cpp.
◆ deleteCursor()
bool KDbConnectionProxy::deleteCursor | ( | KDbCursor * | cursor | ) |
Definition at line 286 of file KDbConnectionProxy.cpp.
◆ deleteRecord()
bool KDbConnectionProxy::deleteRecord | ( | KDbQuerySchema * | query, |
KDbRecordData * | data, | ||
bool | useRecordId = false ) |
Definition at line 772 of file KDbConnectionProxy.cpp.
◆ disconnect()
bool KDbConnectionProxy::disconnect | ( | ) |
Definition at line 117 of file KDbConnectionProxy.cpp.
◆ driver()
KDbDriver * KDbConnectionProxy::driver | ( | ) | const |
Definition at line 77 of file KDbConnectionProxy.cpp.
◆ dropDatabase()
Definition at line 153 of file KDbConnectionProxy.cpp.
◆ dropQuery() [1/2]
bool KDbConnectionProxy::dropQuery | ( | const QString & | queryName | ) |
Definition at line 482 of file KDbConnectionProxy.cpp.
◆ dropQuery() [2/2]
bool KDbConnectionProxy::dropQuery | ( | KDbQuerySchema * | querySchema | ) |
Definition at line 477 of file KDbConnectionProxy.cpp.
◆ dropTable() [1/2]
Definition at line 461 of file KDbConnectionProxy.cpp.
◆ dropTable() [2/2]
tristate KDbConnectionProxy::dropTable | ( | KDbTableSchema * | tableSchema | ) |
Definition at line 456 of file KDbConnectionProxy.cpp.
◆ dropTableInternal()
tristate KDbConnectionProxy::dropTableInternal | ( | KDbTableSchema * | tableSchema, |
bool | alsoRemoveSchema ) |
Definition at line 626 of file KDbConnectionProxy.cpp.
◆ drv_afterInsert()
|
overridevirtual |
Postprocessing (if any) required by drivers before execution of an Insert statement. Reimplement this method in your driver if there are any special processing steps to be executed after an Insert statement.
- See also
- drv_beforeInsert()
Reimplemented from KDbConnection.
Definition at line 712 of file KDbConnectionProxy.cpp.
◆ drv_afterUpdate()
|
overridevirtual |
Postprocessing required by drivers before execution of an Insert statement. Reimplement this method in your driver if there are any special processing steps to be executed after an Update statement.
- See also
- drv_beforeUpdate()
Reimplemented from KDbConnection.
Definition at line 722 of file KDbConnectionProxy.cpp.
◆ drv_alterTableName()
|
overridevirtual |
Alters table's described tableSchema name to newName. This is the default implementation, using "ALTER TABLE <oldname> RENAME TO <newname>", what's supported by SQLite >= 3.2, PostgreSQL, MySQL. Backends lacking ALTER TABLE, for example SQLite, reimplement this with by an inefficient data copying to a new table. In any case, renaming is performed at the backend. It's good idea to keep the operation within a transaction.
- Returns
- true on success.
Reimplemented from KDbConnection.
Definition at line 610 of file KDbConnectionProxy.cpp.
◆ drv_beforeInsert()
|
overridevirtual |
Preprocessing (if any) required by drivers before execution of an Insert statement. Reimplement this method in your driver if there are any special processing steps to be executed before an Insert statement.
- See also
- drv_afterInsert()
Reimplemented from KDbConnection.
Definition at line 707 of file KDbConnectionProxy.cpp.
◆ drv_beforeUpdate()
|
overridevirtual |
Preprocessing required by drivers before execution of an Update statement. Reimplement this method in your driver if there are any special processing steps to be executed before an Update statement.
- See also
- drv_afterUpdate()
Reimplemented from KDbConnection.
Definition at line 717 of file KDbConnectionProxy.cpp.
◆ drv_beginTransaction()
|
overridevirtual |
Note for driver developers: begins new transaction and returns handle to it. Default implementation just executes "BEGIN" sql statement and returns just empty data (KDbTransactionData object). Ownership of the returned object is passed to the caller.
Drivers that do not support transactions (see KDbDriver::features()) do never call this method. Reimplement this method if you need to do something more (e.g. if you driver will support multiple transactions per connection). Make subclass of KDbTransactionData (declared in KDbTransaction.h) and return object of this subclass. nullptr
should be returned on error. Do not check anything in connection (isConnected(), etc.) - all is already done.
- Todo
- Add support for nested transactions, e.g. KDbTransactionData* beginTransaction(KDbTransactionData *parent)
Reimplemented from KDbConnection.
Definition at line 692 of file KDbConnectionProxy.cpp.
◆ drv_closeDatabase()
|
overridevirtual |
For implementation: closes previously opened database using connection.
Implements KDbConnection.
Definition at line 672 of file KDbConnectionProxy.cpp.
◆ drv_commitTransaction()
|
overridevirtual |
Note for driver developers: begins new transaction and returns handle to it. Default implementation just executes "COMMIT" sql statement and returns true on success.
- See also
- drv_beginTransaction()
Reimplemented from KDbConnection.
Definition at line 697 of file KDbConnectionProxy.cpp.
◆ drv_connect()
|
overridevirtual |
For implementation: connects to database.
- Returns
- true on success.
Implements KDbConnection.
Definition at line 579 of file KDbConnectionProxy.cpp.
◆ drv_containsTable()
LOW LEVEL METHOD. For implementation: returns true if table with name tableName exists in the database.
- Returns
false
if it does not exist orcancelled
if error occurred. The lookup is case insensitive.
Implements KDbConnection.
Definition at line 600 of file KDbConnectionProxy.cpp.
◆ drv_copyTableData()
|
overridevirtual |
Copies table data from tableSchema to destinationTableSchema Default implementation executes "INSERT INTO .. SELECT * FROM .."
- Returns
- true on success.
Reimplemented from KDbConnection.
Definition at line 615 of file KDbConnectionProxy.cpp.
◆ drv_createDatabase()
For implementation: creates new database using connection
Implements KDbConnection.
Definition at line 661 of file KDbConnectionProxy.cpp.
◆ drv_createTable() [1/2]
|
overridevirtual |
Creates table using tableSchema information.
- Returns
- true on success.
Default implementation builds a statement using createTableStatement() and calls executeSql(). Note for driver developers: reimplement this only to perform creation in other way.
Reimplemented from KDbConnection.
Definition at line 605 of file KDbConnectionProxy.cpp.
◆ drv_createTable() [2/2]
|
overridevirtual |
Creates table named by tableName. Schema object must be on schema tables' list before calling this method (otherwise false if returned). Just uses drv_createTable( const KDbTableSchema& tableSchema ). Used internally, e.g. in createDatabase().
- Returns
- true on success
Reimplemented from KDbConnection.
Definition at line 687 of file KDbConnectionProxy.cpp.
◆ drv_databaseExists()
|
overridevirtual |
For optional reimplementation: asks server if database dbName exists. This method is used internally in databaseExists(). The default implementation calls databaseNames and checks if that list contains dbName. If you need to ask the server specifically if a database exists, eg. if you can't retrieve a list of all available database names, please reimplement this method and do all needed checks.
See databaseExists() description for details about ignoreErrors argument. You should use it properly in your implementation.
Note: This method should also work if there is already database used (with useDatabase()); in this situation no changes should be made in current database selection.
Reimplemented from KDbConnection.
Definition at line 656 of file KDbConnectionProxy.cpp.
◆ drv_disconnect()
|
overridevirtual |
For implementation: disconnects database
- Returns
- true on success.
Implements KDbConnection.
Definition at line 584 of file KDbConnectionProxy.cpp.
◆ drv_dropDatabase()
For implementation: drops database from the server using connection. After drop, database shouldn't be accessible anymore.
Implements KDbConnection.
Definition at line 682 of file KDbConnectionProxy.cpp.
◆ drv_dropTable()
|
overridevirtual |
Physically drops table named with name. Default impelmentation executes "DROP TABLE.." command, so you rarely want to change this.
Reimplemented from KDbConnection.
Definition at line 621 of file KDbConnectionProxy.cpp.
◆ drv_executeSql()
|
overridevirtual |
Executes query for a raw SQL statement sql without returning resulting records.
It is useful mostly for INSERT queries but it is possible to execute SELECT queries when returned records can be ignored. The @sql should be is valid and properly escaped.
- Note
- Only use this method if you really need.
- See also
- executeSql
Implements KDbConnection.
Definition at line 646 of file KDbConnectionProxy.cpp.
◆ drv_getDatabasesList()
|
overridevirtual |
For reimplementation: loads list of databases' names available for this connection and adds these names to list. If your server is not able to offer such a list, consider reimplementing drv_databaseExists() instead. The method should return true only if there was no error on getting database names list from the server. Default implementation puts empty list into list and returns true.
- See also
- databaseNames
Reimplemented from KDbConnection.
Definition at line 651 of file KDbConnectionProxy.cpp.
◆ drv_getServerVersion()
|
overridevirtual |
For implementation: Sets version to real server's version. Depending on backend type this method is called after (if KDbDriverBehavior::USING_DATABASE_REQUIRED_TO_CONNECT is true) or before database is used (if KDbDriverBehavior::USING_DATABASE_REQUIRED_TO_CONNECT is false), i.e. for PostgreSQL it is called after. In any case it is called after successful drv_connect().
- Returns
- true on success.
Implements KDbConnection.
Definition at line 589 of file KDbConnectionProxy.cpp.
◆ drv_getTableNames()
|
overridevirtual |
◆ drv_isDatabaseUsed()
|
overridevirtual |
- Returns
- true if internal driver's structure is still in opened/connected state and database is used. Note for driver developers: Put here every test that you can do using your internal engine's database API, eg (a bit schematic): my_connection_struct->isConnected()==true. Do not check things like KDbConnection::isDatabaseUsed() here or other things that "KDb already knows" at its level. If you cannot test anything, just leave default implementation (that returns true).
Result of this method is used as an additional chance to check for isDatabaseUsed(). Do not call this method from your driver's code, it should be used at KDb level only.
Reimplemented from KDbConnection.
Definition at line 677 of file KDbConnectionProxy.cpp.
◆ drv_prepareSql()
|
overridevirtual |
Prepares query for a raw SQL statement sql with possibility of returning records.
It is useful mostly for SELECT queries. While INSERT queries do not return records, the KDbSqlResult object offers KDbSqlResult::lastInsertRecordId(). The @sql should be is valid and properly escaped. Only use this method if you really need. For low-level access to the results (without cursors). The result may be not stored (not buffered) yet. Use KDbSqlResult::fetchRecord() to fetch each record.
- Returns
- Null pointer if there is no proper result or error. Ownership of the returned object is passed to the caller.
- See also
- prepareSql
Implements KDbConnection.
Definition at line 641 of file KDbConnectionProxy.cpp.
◆ drv_rollbackTransaction()
|
overridevirtual |
Note for driver developers: begins new transaction and returns handle to it. Default implementation just executes "ROLLBACK" sql statement and returns true on success.
- See also
- drv_beginTransaction()
Reimplemented from KDbConnection.
Definition at line 702 of file KDbConnectionProxy.cpp.
◆ drv_setAutoCommit()
|
overridevirtual |
Changes autocommiting option for established connection.
- Returns
- true on success.
Note for driver developers: reimplement this only if your engine allows to set special auto commit option (like "SET AUTOCOMMIT=.." in MySQL). If not, auto commit behavior will be simulated if at least single transactions per connection are supported by the engine. Do not set any internal flags for autocommiting – it is already done inside setAutoCommit().
Default implementation does nothing with connection, just returns true.
- See also
- drv_beginTransaction(), autoCommit(), setAutoCommit()
Reimplemented from KDbConnection.
Definition at line 727 of file KDbConnectionProxy.cpp.
◆ drv_useDatabase()
|
overridevirtual |
For implementation: opens existing database using connection
- Returns
- true on success, false on failure; sets cancelled to true if this action has been cancelled.
Implements KDbConnection.
Definition at line 666 of file KDbConnectionProxy.cpp.
◆ escapeIdentifier()
Identifier escaping function in the associated KDbDriver.
Calls the identifier escaping function in this connection to escape table and column names. This should be used when explicitly constructing SQL strings (e.g. "FROM " + escapeIdentifier(tablename)). It should not be used for other functions (e.g. don't do useDatabase(escapeIdentifier(database))), because the identifier will be escaped when the called function generates, for example, "USE " + escapeIdentifier(database).
For efficiency, KDb "system" tables (prefixed with kexi__) and columns therein are not escaped - we assume these are valid identifiers for all drivers.
Use KDbEscapedString::isValid() to check if escaping has been performed successfully. Invalid strings are set to null in addition, that is KDbEscapedString::isNull() is true, not just isEmpty().
Reimplemented from KDbConnection.
Definition at line 574 of file KDbConnectionProxy.cpp.
◆ escapeString()
|
overridevirtual |
Connection-specific string escaping. Default implementation uses driver's escaping. Use KDbEscapedString::isValid() to check if escaping has been performed successfully. Invalid strings are set to null in addition, that is KDbEscapedString::isNull() is true, not just isEmpty().
Reimplemented from KDbConnection.
Definition at line 245 of file KDbConnectionProxy.cpp.
◆ executeQuery() [1/4]
KDbCursor * KDbConnectionProxy::executeQuery | ( | const KDbEscapedString & | sql, |
KDbCursor::Options | options = KDbCursor::Option::None ) |
Definition at line 265 of file KDbConnectionProxy.cpp.
◆ executeQuery() [2/4]
KDbCursor * KDbConnectionProxy::executeQuery | ( | KDbQuerySchema * | query, |
const QList< QVariant > & | params, | ||
KDbCursor::Options | options = KDbCursor::Option::None ) |
Definition at line 270 of file KDbConnectionProxy.cpp.
◆ executeQuery() [3/4]
KDbCursor * KDbConnectionProxy::executeQuery | ( | KDbQuerySchema * | query, |
KDbCursor::Options | options = KDbCursor::Option::None ) |
Definition at line 276 of file KDbConnectionProxy.cpp.
◆ executeQuery() [4/4]
KDbCursor * KDbConnectionProxy::executeQuery | ( | KDbTableSchema * | table, |
KDbCursor::Options | options = KDbCursor::Option::None ) |
Definition at line 281 of file KDbConnectionProxy.cpp.
◆ executeQueryInternal()
KDbCursor * KDbConnectionProxy::executeQueryInternal | ( | const KDbEscapedString & | sql, |
KDbQuerySchema * | query, | ||
const QList< QVariant > * | params ) |
Definition at line 815 of file KDbConnectionProxy.cpp.
◆ executeSql()
bool KDbConnectionProxy::executeSql | ( | const KDbEscapedString & | sql | ) |
Definition at line 517 of file KDbConnectionProxy.cpp.
◆ findSystemFieldName()
KDbField * KDbConnectionProxy::findSystemFieldName | ( | const KDbFieldList & | fieldlist | ) |
Definition at line 492 of file KDbConnectionProxy.cpp.
◆ insertRecord() [1/3]
bool KDbConnectionProxy::insertRecord | ( | KDbFieldList * | fields, |
const QList< QVariant > & | values ) |
Definition at line 436 of file KDbConnectionProxy.cpp.
◆ insertRecord() [2/3]
bool KDbConnectionProxy::insertRecord | ( | KDbQuerySchema * | query, |
KDbRecordData * | data, | ||
KDbRecordEditBuffer * | buf, | ||
bool | getRecordId = false ) |
Definition at line 767 of file KDbConnectionProxy.cpp.
◆ insertRecord() [3/3]
H_INS_REC_ALL bool KDbConnectionProxy::insertRecord | ( | KDbTableSchema * | tableSchema, |
const QList< QVariant > & | values ) |
Definition at line 431 of file KDbConnectionProxy.cpp.
◆ isConnected()
bool KDbConnectionProxy::isConnected | ( | ) | const |
Definition at line 87 of file KDbConnectionProxy.cpp.
◆ isDatabaseUsed()
bool KDbConnectionProxy::isDatabaseUsed | ( | ) | const |
Definition at line 92 of file KDbConnectionProxy.cpp.
◆ isEmpty()
tristate KDbConnectionProxy::isEmpty | ( | KDbTableSchema * | table | ) |
Definition at line 393 of file KDbConnectionProxy.cpp.
◆ isInternalTableSchema()
bool KDbConnectionProxy::isInternalTableSchema | ( | const QString & | tableName | ) |
Definition at line 569 of file KDbConnectionProxy.cpp.
◆ loadDataBlock()
tristate KDbConnectionProxy::loadDataBlock | ( | int | objectID, |
QString * | dataString, | ||
const QString & | dataID = QString() ) |
Definition at line 542 of file KDbConnectionProxy.cpp.
◆ loadExtendedTableSchemaData()
bool KDbConnectionProxy::loadExtendedTableSchemaData | ( | KDbTableSchema * | tableSchema | ) |
Definition at line 821 of file KDbConnectionProxy.cpp.
◆ loadObjectData() [1/2]
Definition at line 537 of file KDbConnectionProxy.cpp.
◆ loadObjectData() [2/2]
- Since
- 3.1
Definition at line 532 of file KDbConnectionProxy.cpp.
◆ objectIds()
QList< int > KDbConnectionProxy::objectIds | ( | int | objectType, |
bool * | ok = nullptr ) |
Definition at line 198 of file KDbConnectionProxy.cpp.
◆ objectNames()
QStringList KDbConnectionProxy::objectNames | ( | int | objectType = KDb::AnyObjectType, |
bool * | ok = nullptr ) |
Definition at line 158 of file KDbConnectionProxy.cpp.
◆ options()
KDbConnectionOptions * KDbConnectionProxy::options | ( | ) |
Definition at line 97 of file KDbConnectionProxy.cpp.
◆ parentConnection() [1/2]
KDbConnection * KDbConnectionProxy::parentConnection | ( | ) |
- Returns
- parent connection for this proxy
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 57 of file KDbConnectionProxy.cpp.
◆ parentConnection() [2/2]
const KDbConnection * KDbConnectionProxy::parentConnection | ( | ) | const |
Definition at line 62 of file KDbConnectionProxy.cpp.
◆ prepareQuery() [1/3]
|
overridevirtual |
Prepares SELECT query described by a raw statement sql.
- Returns
- opened cursor created for results of this query or
nullptr
if there was any error. Ownership of the returned object is passed to the caller. KDbCursor can have optionally applied options (one of more selected from KDbCursor::Options). Preparation means that returned cursor is created but not opened. Open this when you would like to do it with KDbCursor::open().
Note for driver developers: you should initialize cursor engine-specific resources and return KDbCursor subclass' object (passing sql and options to its constructor).
Implements KDbConnection.
Definition at line 250 of file KDbConnectionProxy.cpp.
◆ prepareQuery() [2/3]
|
overridevirtual |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Prepares query described by query schema without parameters.
Implements KDbConnection.
Definition at line 255 of file KDbConnectionProxy.cpp.
◆ prepareQuery() [3/3]
KDbCursor * KDbConnectionProxy::prepareQuery | ( | KDbTableSchema * | table, |
KDbCursor::Options | options = KDbCursor::Option::None ) |
Definition at line 260 of file KDbConnectionProxy.cpp.
◆ prepareSql()
QSharedPointer< KDbSqlResult > KDbConnectionProxy::prepareSql | ( | const KDbEscapedString & | sql | ) |
Definition at line 512 of file KDbConnectionProxy.cpp.
◆ prepareStatement()
KDbPreparedStatement KDbConnectionProxy::prepareStatement | ( | KDbPreparedStatement::Type | type, |
KDbFieldList * | fields, | ||
const QStringList & | whereFieldNames = QStringList() ) |
Definition at line 563 of file KDbConnectionProxy.cpp.
◆ prepareStatementInternal()
|
overridevirtual |
Prepare an SQL statement and return a KDbPreparedStatementInterface-derived object. Ownership of the returned object is passed to the caller.
Implements KDbConnection.
Definition at line 732 of file KDbConnectionProxy.cpp.
◆ queryIds()
QList< int > KDbConnectionProxy::queryIds | ( | bool * | ok = nullptr | ) |
Definition at line 193 of file KDbConnectionProxy.cpp.
◆ querySchema() [1/2]
KDbQuerySchema * KDbConnectionProxy::querySchema | ( | const QString & | queryName | ) |
Definition at line 306 of file KDbConnectionProxy.cpp.
◆ querySchema() [2/2]
KDbQuerySchema * KDbConnectionProxy::querySchema | ( | int | queryId | ) |
Definition at line 301 of file KDbConnectionProxy.cpp.
◆ querySingleNumber() [1/3]
tristate KDbConnectionProxy::querySingleNumber | ( | const KDbEscapedString & | sql, |
int * | number, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 353 of file KDbConnectionProxy.cpp.
◆ querySingleNumber() [2/3]
tristate KDbConnectionProxy::querySingleNumber | ( | KDbQuerySchema * | query, |
int * | number, | ||
const QList< QVariant > & | params, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 365 of file KDbConnectionProxy.cpp.
◆ querySingleNumber() [3/3]
tristate KDbConnectionProxy::querySingleNumber | ( | KDbQuerySchema * | query, |
int * | number, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 359 of file KDbConnectionProxy.cpp.
◆ querySingleNumberInternal()
tristate KDbConnectionProxy::querySingleNumberInternal | ( | const KDbEscapedString * | sql, |
int * | number, | ||
KDbQuerySchema * | query, | ||
const QList< QVariant > * | params, | ||
int | column, | ||
QueryRecordOptions | options ) |
Definition at line 801 of file KDbConnectionProxy.cpp.
◆ querySingleRecord() [1/3]
tristate KDbConnectionProxy::querySingleRecord | ( | const KDbEscapedString & | sql, |
KDbRecordData * | data, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 316 of file KDbConnectionProxy.cpp.
◆ querySingleRecord() [2/3]
tristate KDbConnectionProxy::querySingleRecord | ( | KDbQuerySchema * | query, |
KDbRecordData * | data, | ||
const QList< QVariant > & | params, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 328 of file KDbConnectionProxy.cpp.
◆ querySingleRecord() [3/3]
tristate KDbConnectionProxy::querySingleRecord | ( | KDbQuerySchema * | query, |
KDbRecordData * | data, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 322 of file KDbConnectionProxy.cpp.
◆ querySingleRecordInternal()
tristate KDbConnectionProxy::querySingleRecordInternal | ( | KDbRecordData * | data, |
const KDbEscapedString * | sql, | ||
KDbQuerySchema * | query, | ||
const QList< QVariant > * | params, | ||
QueryRecordOptions | options ) |
Definition at line 787 of file KDbConnectionProxy.cpp.
◆ querySingleString() [1/3]
tristate KDbConnectionProxy::querySingleString | ( | const KDbEscapedString & | sql, |
QString * | value, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 334 of file KDbConnectionProxy.cpp.
◆ querySingleString() [2/3]
tristate KDbConnectionProxy::querySingleString | ( | KDbQuerySchema * | query, |
QString * | value, | ||
const QList< QVariant > & | params, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 346 of file KDbConnectionProxy.cpp.
◆ querySingleString() [3/3]
tristate KDbConnectionProxy::querySingleString | ( | KDbQuerySchema * | query, |
QString * | value, | ||
int | column = 0, | ||
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 340 of file KDbConnectionProxy.cpp.
◆ querySingleStringInternal()
tristate KDbConnectionProxy::querySingleStringInternal | ( | const KDbEscapedString * | sql, |
QString * | value, | ||
KDbQuerySchema * | query, | ||
const QList< QVariant > * | params, | ||
int | column, | ||
QueryRecordOptions | options ) |
Definition at line 794 of file KDbConnectionProxy.cpp.
◆ queryStringList() [1/3]
bool KDbConnectionProxy::queryStringList | ( | const KDbEscapedString & | sql, |
QStringList * | list, | ||
int | column = 0 ) |
Definition at line 372 of file KDbConnectionProxy.cpp.
◆ queryStringList() [2/3]
bool KDbConnectionProxy::queryStringList | ( | KDbQuerySchema * | query, |
QStringList * | list, | ||
const QList< QVariant > & | params, | ||
int | column = 0 ) |
Definition at line 382 of file KDbConnectionProxy.cpp.
◆ queryStringList() [3/3]
bool KDbConnectionProxy::queryStringList | ( | KDbQuerySchema * | query, |
QStringList * | list, | ||
int | column = 0 ) |
Definition at line 377 of file KDbConnectionProxy.cpp.
◆ queryStringListInternal()
bool KDbConnectionProxy::queryStringListInternal | ( | const KDbEscapedString * | sql, |
QStringList * | list, | ||
KDbQuerySchema * | query, | ||
const QList< QVariant > * | params, | ||
int | column, | ||
bool(* | filterFunction )(const QString &) ) |
Definition at line 808 of file KDbConnectionProxy.cpp.
◆ recentSqlString()
|
overridevirtual |
Return recently used SQL string.
If there was a successful query execution it is equal to result().sql(), otherwise it is equal to result().errorSql().
Reimplemented from KDbConnection.
Definition at line 398 of file KDbConnectionProxy.cpp.
◆ removeDataBlock()
Definition at line 558 of file KDbConnectionProxy.cpp.
◆ removeObject()
bool KDbConnectionProxy::removeObject | ( | int | objId | ) |
Definition at line 487 of file KDbConnectionProxy.cpp.
◆ result()
KDbResult KDbConnectionProxy::result | ( | ) | const |
Definition at line 107 of file KDbConnectionProxy.cpp.
◆ resultable()
KDbResultable KDbConnectionProxy::resultable | ( | ) | const |
Definition at line 112 of file KDbConnectionProxy.cpp.
◆ resultExists()
tristate KDbConnectionProxy::resultExists | ( | const KDbEscapedString & | sql, |
QueryRecordOptions | options = QueryRecordOption::Default ) |
Definition at line 388 of file KDbConnectionProxy.cpp.
◆ rollbackAutoCommitTransaction()
bool KDbConnectionProxy::rollbackAutoCommitTransaction | ( | const KDbTransaction & | trans | ) |
Definition at line 747 of file KDbConnectionProxy.cpp.
◆ rollbackTransaction()
bool KDbConnectionProxy::rollbackTransaction | ( | KDbTransaction | trans = KDbTransaction(), |
KDbTransaction::CommitOptions | options = KDbTransaction::CommitOptions() ) |
Definition at line 214 of file KDbConnectionProxy.cpp.
◆ serverVersion()
KDbServerVersionInfo KDbConnectionProxy::serverVersion | ( | ) | const |
Definition at line 173 of file KDbConnectionProxy.cpp.
◆ setAutoCommit()
bool KDbConnectionProxy::setAutoCommit | ( | bool | on | ) |
Definition at line 240 of file KDbConnectionProxy.cpp.
◆ setAvailableDatabaseName()
void KDbConnectionProxy::setAvailableDatabaseName | ( | const QString & | dbName | ) |
Definition at line 502 of file KDbConnectionProxy.cpp.
◆ setDefaultTransaction()
void KDbConnectionProxy::setDefaultTransaction | ( | const KDbTransaction & | trans | ) |
Definition at line 225 of file KDbConnectionProxy.cpp.
◆ setParentConnectionIsOwned()
void KDbConnectionProxy::setParentConnectionIsOwned | ( | bool | set | ) |
Control owhership of parent connection that is assigned to this proxy.
Owned connection is closed and destroyed upon destruction of the KDbConnectionProxy object.
Definition at line 67 of file KDbConnectionProxy.cpp.
◆ setQuerySchemaObsolete()
bool KDbConnectionProxy::setQuerySchemaObsolete | ( | const QString & | queryName | ) |
Definition at line 311 of file KDbConnectionProxy.cpp.
◆ setupField()
KDbField * KDbConnectionProxy::setupField | ( | const KDbRecordData & | data | ) |
Definition at line 636 of file KDbConnectionProxy.cpp.
◆ setupObjectData()
bool KDbConnectionProxy::setupObjectData | ( | const KDbRecordData & | data, |
KDbObject * | object ) |
Definition at line 631 of file KDbConnectionProxy.cpp.
◆ storeDataBlock()
bool KDbConnectionProxy::storeDataBlock | ( | int | objectID, |
const QString & | dataString, | ||
const QString & | dataID = QString() ) |
Definition at line 547 of file KDbConnectionProxy.cpp.
◆ storeExtendedTableSchemaData()
bool KDbConnectionProxy::storeExtendedTableSchemaData | ( | KDbTableSchema * | tableSchema | ) |
Definition at line 826 of file KDbConnectionProxy.cpp.
◆ storeMainFieldSchema()
bool KDbConnectionProxy::storeMainFieldSchema | ( | KDbField * | field | ) |
Definition at line 831 of file KDbConnectionProxy.cpp.
◆ storeNewObjectData()
bool KDbConnectionProxy::storeNewObjectData | ( | KDbObject * | object | ) |
Definition at line 527 of file KDbConnectionProxy.cpp.
◆ storeObjectData()
bool KDbConnectionProxy::storeObjectData | ( | KDbObject * | object | ) |
Definition at line 522 of file KDbConnectionProxy.cpp.
◆ tableIds()
QList< int > KDbConnectionProxy::tableIds | ( | bool * | ok = nullptr | ) |
Definition at line 188 of file KDbConnectionProxy.cpp.
◆ tableNames()
QStringList KDbConnectionProxy::tableNames | ( | bool | alsoSystemTables = false, |
bool * | ok = nullptr ) |
Definition at line 163 of file KDbConnectionProxy.cpp.
◆ tableSchema() [1/2]
KDbTableSchema * KDbConnectionProxy::tableSchema | ( | const QString & | tableName | ) |
Definition at line 296 of file KDbConnectionProxy.cpp.
◆ tableSchema() [2/2]
KDbTableSchema * KDbConnectionProxy::tableSchema | ( | int | tableId | ) |
Definition at line 291 of file KDbConnectionProxy.cpp.
◆ transactions()
QList< KDbTransaction > KDbConnectionProxy::transactions | ( | ) |
Definition at line 230 of file KDbConnectionProxy.cpp.
◆ updateRecord()
bool KDbConnectionProxy::updateRecord | ( | KDbQuerySchema * | query, |
KDbRecordData * | data, | ||
KDbRecordEditBuffer * | buf, | ||
bool | useRecordId = false ) |
Definition at line 762 of file KDbConnectionProxy.cpp.
◆ useDatabase()
bool KDbConnectionProxy::useDatabase | ( | const QString & | dbName = QString(), |
bool | kexiCompatible = true, | ||
bool * | cancelled = nullptr, | ||
KDbMessageHandler * | msgHandler = nullptr ) |
Definition at line 137 of file KDbConnectionProxy.cpp.
◆ useTemporaryDatabaseIfNeeded()
bool KDbConnectionProxy::useTemporaryDatabaseIfNeeded | ( | QString * | name | ) |
Definition at line 507 of file KDbConnectionProxy.cpp.
Member Data Documentation
◆ H_INS_REC_ALL
KDbConnectionProxy::H_INS_REC_ALL |
Definition at line 218 of file KDbConnectionProxy.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Feb 21 2025 11:51:50 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.