KDbIndexSchema
#include <KDbIndexSchema.h>
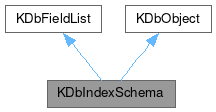
Public Member Functions | |
KDbIndexSchema () | |
~KDbIndexSchema () override | |
virtual bool | addField (KDbField *field) |
void | attachRelationship (KDbRelationship *rel) |
void | detachRelationship (KDbRelationship *rel) |
QList< const KDbRelationship * > | detailsRelationships () const |
bool | isAutoGenerated () const |
bool | isForeignKey () const |
bool | isPrimaryKey () const |
bool | isUnique () const |
QList< const KDbRelationship * > | masterRelationships () const |
void | setPrimaryKey (bool set) |
void | setUnique (bool set) |
KDbTableSchema * | table () |
const KDbTableSchema * | table () const |
![]() | |
KDbFieldList (bool owner=false) | |
KDbFieldList (const KDbFieldList &fl, bool deepCopyFields=true) | |
virtual | ~KDbFieldList () |
bool | addField (KDbField *field) |
KDbField::List * | autoIncrementFields () const |
virtual void | clear () |
virtual KDbField * | field (const QString &name) |
virtual const KDbField * | field (const QString &name) const |
virtual KDbField * | field (int id) |
virtual const KDbField * | field (int id) const |
int | fieldCount () const |
KDbField::List * | fields () |
const KDbField::List * | fields () const |
KDbField::ListIterator | fieldsIterator () const |
KDbField::ListIterator | fieldsIteratorConstEnd () const |
bool | hasField (const KDbField &field) const |
int | indexOf (const KDbField &field) const |
virtual bool | insertField (int index, KDbField *field) |
bool | isEmpty () const |
bool | isOwner () const |
virtual bool | moveField (KDbField *field, int newIndex) |
QStringList | names () const |
virtual bool | removeField (KDbField *field) |
bool | renameField (const QString &oldName, const QString &newName) |
bool | renameField (KDbField *field, const QString &newName) |
KDbEscapedString | sqlFieldsList (KDbConnection *conn, const QString &separator=QLatin1String(","), const QString &tableOrAlias=QString(), KDb::IdentifierEscapingType escapingType=KDb::DriverEscaping) const |
KDbFieldList * | subList (const QList< int > &list) |
KDbFieldList * | subList (const QList< QByteArray > &list) |
KDbFieldList * | subList (const QString &n1, const QString &n2=QString(), const QString &n3=QString(), const QString &n4=QString(), const QString &n5=QString(), const QString &n6=QString(), const QString &n7=QString(), const QString &n8=QString(), const QString &n9=QString(), const QString &n10=QString(), const QString &n11=QString(), const QString &n12=QString(), const QString &n13=QString(), const QString &n14=QString(), const QString &n15=QString(), const QString &n16=QString(), const QString &n17=QString(), const QString &n18=QString()) |
KDbFieldList * | subList (const QStringList &list) |
![]() | |
KDbObject (int type) | |
QString | captionOrName () const |
Protected Member Functions | |
KDbIndexSchema (const KDbIndexSchema &index, KDbTableSchema *parentTable) | |
void | attachRelationship (KDbRelationship *rel, bool ownedByMaster) |
void | setAutoGenerated (bool set) |
void | setForeignKey (bool set) |
void | setTable (KDbTableSchema *table) |
![]() | |
virtual void | clear () |
Additional Inherited Members | |
![]() | |
static KDbEscapedString | sqlFieldsList (const KDbField::List &list, KDbConnection *conn, const QString &separator=QLatin1String(","), const QString &tableOrAlias=QString(), KDb::IdentifierEscapingType escapingType=KDb::DriverEscaping) |
![]() | |
QString | caption |
QString | description |
int | id |
QString | name |
int | type |
Detailed Description
Provides information about database index that can be created for a database table.
KDbIndexSchema object stores information about table fields that defines this index and additional properties like: whether index is unique or primary key (requires unique). Single-field index can be also auto generated.
Definition at line 39 of file KDbIndexSchema.h.
Constructor & Destructor Documentation
◆ KDbIndexSchema() [1/2]
KDbIndexSchema::KDbIndexSchema | ( | ) |
Constructs empty index schema object that not assigned to any table. KDbTableSchema::addIndex() should be called afterwards, before adding any fields or attaching relationships. Any fields added with addField() will not be owned by index but by their table.
Definition at line 74 of file KDbIndexSchema.cpp.
◆ ~KDbIndexSchema()
|
override |
Deletes the index. Referenced KDbField objects are not deleted. All KDbRelationship objects listed by masterRelationships() are detached from detail-side indices and then deleted. KDbRelationship objects listed by detailsRelationships() are not deleted.
Definition at line 108 of file KDbIndexSchema.cpp.
◆ KDbIndexSchema() [2/2]
|
protected |
Used by KDbTableSchema::copyIndex(const KDbIndexSchema&)
- Todo
- copy relationships!
Definition at line 81 of file KDbIndexSchema.cpp.
Member Function Documentation
◆ addField()
|
virtual |
Adds field at the end of field list. KDbField will not be owned by index. KDbField must belong to a table specified by a KDbTableSchema::addIndex() call, otherwise field couldn't be added.
- Note
- Do not forget to add the field to a table, because adding it only to the KDbIndexSchema is not enough.
Definition at line 124 of file KDbIndexSchema.cpp.
◆ attachRelationship() [1/2]
void KDbIndexSchema::attachRelationship | ( | KDbRelationship * | rel | ) |
Attaches relationship definition rel to this KDbIndexSchema object. If rel relationship has this KDbIndexSchema defined at the master-side, rel is added to the list of master relationships (available with masterRelationships()). If rel relationship has this KDbIndexSchema defined at the details-side, rel is added to the list of details relationships (available with detailsRelationships()). For the former case, attached rel object is now owned by this KDbIndexSchema object.
Note: call detachRelationship() for KDbIndexSchema object that rel was previously attached to, if any.
- Note
- Before using attachRelationship() the index KDbField must already belong to a table specified by a KDbTableSchema::addIndex() call.
Definition at line 218 of file KDbIndexSchema.cpp.
◆ attachRelationship() [2/2]
|
protected |
Internal version of attachRelationship(). If ownedByMaster is true, attached rel object will be owned by this index.
Definition at line 223 of file KDbIndexSchema.cpp.
◆ detachRelationship()
void KDbIndexSchema::detachRelationship | ( | KDbRelationship * | rel | ) |
Detaches relationship definition rel for this KDbIndexSchema object from the list of master relationships (available with masterRelationships()), or from details relationships list, depending for which side of the relationship is this IndexSchem object assigned.
Note: If rel was detached from masterRelationships() list, this object now has no parent, so it must be attached to other index or deleted.
Definition at line 244 of file KDbIndexSchema.cpp.
◆ detailsRelationships()
QList< const KDbRelationship * > KDbIndexSchema::detailsRelationships | ( | ) | const |
- Returns
- list of relationships to the table (of this index), i.e. any such relationship in which this table is at 'details' side. See KDbRelationship class documentation for more information.
Definition at line 151 of file KDbIndexSchema.cpp.
◆ isAutoGenerated()
bool KDbIndexSchema::isAutoGenerated | ( | ) | const |
- Returns
- true if index is auto-generated. Auto-generated index is one-field index that was automatically generated for CREATE TABLE statement when the field has UNIQUE or PRIMARY KEY constraint enabled.
Any newly created KDbIndexSchema object has this flag set to false.
This flag is handled internally by KDbTableSchema. It can be usable for GUI application if we do not want display implicity/auto generated indices on the indices list or we if want to show these indices to the user in a special way.
Definition at line 156 of file KDbIndexSchema.cpp.
◆ isForeignKey()
bool KDbIndexSchema::isForeignKey | ( | ) | const |
- Returns
- true if the index defines a foreign key, Created implicity for KDbRelationship object.
Definition at line 190 of file KDbIndexSchema.cpp.
◆ isPrimaryKey()
bool KDbIndexSchema::isPrimaryKey | ( | ) | const |
- Returns
- true if this index is primary key of its table. This can be one or multifield.
Definition at line 166 of file KDbIndexSchema.cpp.
◆ isUnique()
bool KDbIndexSchema::isUnique | ( | ) | const |
- Returns
- true if this is unique index. This can be one or multifield.
Definition at line 178 of file KDbIndexSchema.cpp.
◆ masterRelationships()
QList< const KDbRelationship * > KDbIndexSchema::masterRelationships | ( | ) | const |
- Returns
- list of relationships from the table (of this index), i.e. any such relationship in which this table is at 'master' side. See KDbRelationship class documentation for more information. All objects on this list will be automatically deleted when this KDbIndexSchema object is deleted.
Definition at line 146 of file KDbIndexSchema.cpp.
◆ setAutoGenerated()
|
protected |
Sets auto-generated flag. This method should be called only from KDbTableSchema code
- See also
- isAutoGenerated().
Definition at line 161 of file KDbIndexSchema.cpp.
◆ setForeignKey()
|
protected |
If set is true, declares that the index defines a foreign key, created implicity for KDbRelationship object. Setting this to true, implies clearing 'primary key', 'unique' and 'auto generated' flags. If this index contains just single field, it's 'foreign field' flag will be set to true as well.
Definition at line 195 of file KDbIndexSchema.cpp.
◆ setPrimaryKey()
void KDbIndexSchema::setPrimaryKey | ( | bool | set | ) |
Sets PRIMARY KEY flag.
- See also
- isPrimary(). Note: Setting PRIMARY KEY on (true), UNIQUE flag will be also implicity set.
Definition at line 171 of file KDbIndexSchema.cpp.
◆ setTable()
|
protected |
Assigns this index to table table() must be nullptr
and table must be not be nullptr.
- Since
- 3.1
Definition at line 113 of file KDbIndexSchema.cpp.
◆ setUnique()
void KDbIndexSchema::setUnique | ( | bool | set | ) |
Sets UNIQUE flag.
- See also
- isUnique(). Note: Setting UNIQUE off (false), PRIMARY KEY flag will be also implicity set off, because this UNIQUE is the requirement for PRIMARY KEYS.
Definition at line 183 of file KDbIndexSchema.cpp.
◆ table() [1/2]
KDbTableSchema * KDbIndexSchema::table | ( | ) |
- Returns
- table that index belongs to Index should be assigned to a table using KDbTableSchema::addIndex(). If it is not, table() returns
nullptr
.
Definition at line 141 of file KDbIndexSchema.cpp.
◆ table() [2/2]
const KDbTableSchema * KDbIndexSchema::table | ( | ) | const |
- Returns
- table that index is defined for, const version.
Definition at line 136 of file KDbIndexSchema.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.