KDbNArgExpression
#include <KDbExpression.h>
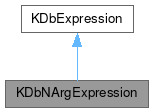
Additional Inherited Members | |
![]() | |
static KDb::ExpressionClass | classForToken (KDbToken token) |
![]() | |
ExplicitlySharedExpressionDataPointer | d |
Detailed Description
The KDbNArgExpression class represents a base class N-argument expression.
Definition at line 212 of file KDbExpression.h.
Constructor & Destructor Documentation
◆ KDbNArgExpression() [1/5]
KDbNArgExpression::KDbNArgExpression | ( | ) |
Constructs a null N-argument expression.
- See also
- KDbExpression::isNull()
Definition at line 181 of file KDbNArgExpression.cpp.
◆ KDbNArgExpression() [2/5]
KDbNArgExpression::KDbNArgExpression | ( | KDb::ExpressionClass | aClass, |
KDbToken | token ) |
Constructs an N-argument expression of class aClass and token token.
Definition at line 193 of file KDbNArgExpression.cpp.
◆ KDbNArgExpression() [3/5]
KDbNArgExpression::KDbNArgExpression | ( | const KDbNArgExpression & | expr | ) |
Constructs a copy of other N-argument expression expr. Resulting object is not a deep copy but rather a reference to the object expr.
Definition at line 199 of file KDbNArgExpression.cpp.
◆ ~KDbNArgExpression()
|
override |
Destroys the expression.
Definition at line 209 of file KDbNArgExpression.cpp.
◆ KDbNArgExpression() [4/5]
|
explicitprotected |
Definition at line 187 of file KDbNArgExpression.cpp.
◆ KDbNArgExpression() [5/5]
|
explicitprotected |
Definition at line 204 of file KDbNArgExpression.cpp.
Member Function Documentation
◆ append()
void KDbNArgExpression::append | ( | const KDbExpression & | expr | ) |
Inserts expression argument expr at the end of this expression.
Definition at line 213 of file KDbNArgExpression.cpp.
◆ arg()
KDbExpression KDbNArgExpression::arg | ( | int | i | ) | const |
- Returns
- expression index i in the list of arguments. If the index i is out of bounds, the function returns null expression.
Definition at line 223 of file KDbNArgExpression.cpp.
◆ argCount()
int KDbNArgExpression::argCount | ( | ) | const |
- Returns
- the number of expression arguments in this expression.
Definition at line 269 of file KDbNArgExpression.cpp.
◆ containsInvalidArgument()
bool KDbNArgExpression::containsInvalidArgument | ( | ) | const |
- Returns
- true if any argument is invalid (!KDbExpressionisValid()).
Definition at line 279 of file KDbNArgExpression.cpp.
◆ containsNullArgument()
bool KDbNArgExpression::containsNullArgument | ( | ) | const |
- Returns
- true if any argument is NULL (type KDbField::Null).
Definition at line 284 of file KDbNArgExpression.cpp.
◆ indexOf()
int KDbNArgExpression::indexOf | ( | const KDbExpression & | expr, |
int | from = 0 ) const |
- Returns
- the index position of the first occurrence of expression argument expr in this expression, searching forward from index position from.
- -1 if no argument matched.
- See also
- lastIndexOf()
Definition at line 259 of file KDbNArgExpression.cpp.
◆ insert()
void KDbNArgExpression::insert | ( | int | i, |
const KDbExpression & | expr ) |
Inserts expression argument expr at index position i in this expression. If i is 0, the expression is prepended to the list of arguments. If i is argCount(), the value is appended to the list of arguments. i must be a valid index position in the list (i.e., 0 <= i < argCount()).
Definition at line 228 of file KDbNArgExpression.cpp.
◆ isEmpty()
bool KDbNArgExpression::isEmpty | ( | ) | const |
- Returns
- true if the expression contains no arguments; otherwise returns false.
Definition at line 274 of file KDbNArgExpression.cpp.
◆ lastIndexOf()
int KDbNArgExpression::lastIndexOf | ( | const KDbExpression & | expr, |
int | from = -1 ) const |
- Returns
- the index position of the last occurrence of expression argument expr in this expression, searching backward from index position from. If from is -1 (the default), the search starts at the last item. Returns -1 if no argument matched.
- See also
- indexOf()
Definition at line 264 of file KDbNArgExpression.cpp.
◆ prepend()
void KDbNArgExpression::prepend | ( | const KDbExpression & | expr | ) |
Inserts expression argument expr at the beginning of this expression.
Definition at line 218 of file KDbNArgExpression.cpp.
◆ remove()
bool KDbNArgExpression::remove | ( | const KDbExpression & | expr | ) |
Removes the expression argument expr and returns true on success; otherwise returns false.
Definition at line 244 of file KDbNArgExpression.cpp.
◆ removeAt()
void KDbNArgExpression::removeAt | ( | int | i | ) |
Removes the expression at index position i. i must be a valid index position in the list (i.e., 0 <= i < argCount()).
Definition at line 249 of file KDbNArgExpression.cpp.
◆ replace()
void KDbNArgExpression::replace | ( | int | i, |
const KDbExpression & | expr ) |
Replaces expression argument at index i with expression expr.
i must be a valid index position in the list (i.e., 0 <= i < argCount()). */
Definition at line 233 of file KDbNArgExpression.cpp.
◆ takeAt()
KDbExpression KDbNArgExpression::takeAt | ( | int | i | ) |
Removes the expression at index position i and returns it. i must be a valid index position in the list (i.e., 0 <= i < argCount()). If you don't use the return value, removeAt() is more efficient.
Definition at line 254 of file KDbNArgExpression.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.