KDbExpressionData
#include <KDbExpressionData.h>
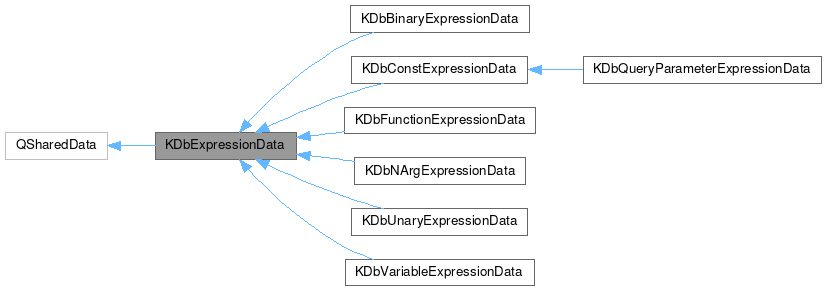
Public Member Functions | |
virtual KDbExpressionData * | clone () |
template<typename T > | |
T * | convert () |
template<typename T > | |
const T * | convertConst () const |
QDebug | debug (QDebug dbg, KDb::ExpressionCallStack *callStack) const |
virtual void | getQueryParameters (QList< KDbQuerySchemaParameter > *params) |
bool | isDateTimeType () const |
bool | isFPNumericType () const |
bool | isIntegerType () const |
bool | isNumericType () const |
bool | isTextType () const |
bool | isValid () const |
KDbEscapedString | toString (const KDbDriver *driver, KDbQuerySchemaParameterValueListIterator *params=nullptr, KDb::ExpressionCallStack *callStack=nullptr) const |
KDbField::Type | type () const |
KDbField::Type | type (KDb::ExpressionCallStack *callStack) const |
bool | validate (KDbParseInfo *parseInfo) |
bool | validate (KDbParseInfo *parseInfo, KDb::ExpressionCallStack *callStack) |
![]() | |
QSharedData (const QSharedData &) | |
Public Attributes | |
QList< ExplicitlySharedExpressionDataPointer > | children |
KDb::ExpressionClass | expressionClass |
ExplicitlySharedExpressionDataPointer | parent |
KDbToken | token |
Protected Member Functions | |
bool | addToCallStack (QDebug *dbg, KDb::ExpressionCallStack *callStack) const |
virtual void | debugInternal (QDebug dbg, KDb::ExpressionCallStack *callStack) const |
virtual KDbEscapedString | toStringInternal (const KDbDriver *driver, KDbQuerySchemaParameterValueListIterator *params, KDb::ExpressionCallStack *callStack) const |
virtual KDbField::Type | typeInternal (KDb::ExpressionCallStack *callStack) const |
virtual bool | validateInternal (KDbParseInfo *parseInfo, KDb::ExpressionCallStack *callStack) |
Detailed Description
Internal data class used to implement implicitly shared class KDbExpression.
Provides thread-safe reference counting.
Definition at line 67 of file KDbExpressionData.h.
Constructor & Destructor Documentation
◆ KDbExpressionData()
KDbExpressionData::KDbExpressionData | ( | ) |
Definition at line 75 of file KDbExpression.cpp.
◆ ~KDbExpressionData()
|
virtual |
Definition at line 91 of file KDbExpression.cpp.
Member Function Documentation
◆ addToCallStack()
|
protected |
Definition at line 216 of file KDbExpression.cpp.
◆ clone()
|
virtual |
Definition at line 96 of file KDbExpression.cpp.
◆ convert()
|
inline |
Definition at line 108 of file KDbExpressionData.h.
◆ convertConst()
|
inline |
Definition at line 105 of file KDbExpressionData.h.
◆ debug()
QDebug KDbExpressionData::debug | ( | QDebug | dbg, |
KDb::ExpressionCallStack * | callStack ) const |
Sends information about this expression to debug output dbg.
Definition at line 238 of file KDbExpression.cpp.
◆ debugInternal()
|
protectedvirtual |
Sends information about this expression to debug output dbg (internal).
Reimplemented in KDbNArgExpressionData, KDbUnaryExpressionData, KDbBinaryExpressionData, KDbConstExpressionData, KDbQueryParameterExpressionData, KDbVariableExpressionData, and KDbFunctionExpressionData.
Definition at line 254 of file KDbExpression.cpp.
◆ getQueryParameters()
|
virtual |
Definition at line 211 of file KDbExpression.cpp.
◆ isDateTimeType()
bool KDbExpressionData::isDateTimeType | ( | ) | const |
Definition at line 149 of file KDbExpression.cpp.
◆ isFPNumericType()
bool KDbExpressionData::isFPNumericType | ( | ) | const |
Definition at line 144 of file KDbExpression.cpp.
◆ isIntegerType()
bool KDbExpressionData::isIntegerType | ( | ) | const |
Definition at line 134 of file KDbExpression.cpp.
◆ isNumericType()
bool KDbExpressionData::isNumericType | ( | ) | const |
Definition at line 139 of file KDbExpression.cpp.
◆ isTextType()
bool KDbExpressionData::isTextType | ( | ) | const |
Definition at line 129 of file KDbExpression.cpp.
◆ isValid()
bool KDbExpressionData::isValid | ( | ) | const |
Definition at line 124 of file KDbExpression.cpp.
◆ toString()
KDbEscapedString KDbExpressionData::toString | ( | const KDbDriver * | driver, |
KDbQuerySchemaParameterValueListIterator * | params = nullptr, | ||
KDb::ExpressionCallStack * | callStack = nullptr ) const |
Definition at line 177 of file KDbExpression.cpp.
◆ toStringInternal()
|
protectedvirtual |
Reimplemented in KDbConstExpressionData.
Definition at line 200 of file KDbExpression.cpp.
◆ type() [1/2]
KDbField::Type KDbExpressionData::type | ( | ) | const |
- Returns
- type of this expression;
Definition at line 118 of file KDbExpression.cpp.
◆ type() [2/2]
KDbField::Type KDbExpressionData::type | ( | KDb::ExpressionCallStack * | callStack | ) | const |
Definition at line 108 of file KDbExpression.cpp.
◆ typeInternal()
|
protectedvirtual |
Reimplemented in KDbUnaryExpressionData, KDbBinaryExpressionData, KDbConstExpressionData, KDbVariableExpressionData, and KDbFunctionExpressionData.
Definition at line 102 of file KDbExpression.cpp.
◆ validate() [1/2]
bool KDbExpressionData::validate | ( | KDbParseInfo * | parseInfo | ) |
Definition at line 154 of file KDbExpression.cpp.
◆ validate() [2/2]
bool KDbExpressionData::validate | ( | KDbParseInfo * | parseInfo, |
KDb::ExpressionCallStack * | callStack ) |
Definition at line 160 of file KDbExpression.cpp.
◆ validateInternal()
|
protectedvirtual |
Reimplemented in KDbUnaryExpressionData, KDbBinaryExpressionData, KDbVariableExpressionData, and KDbFunctionExpressionData.
Definition at line 170 of file KDbExpression.cpp.
Member Data Documentation
◆ children
QList<ExplicitlySharedExpressionDataPointer> KDbExpressionData::children |
Definition at line 89 of file KDbExpressionData.h.
◆ expressionClass
KDb::ExpressionClass KDbExpressionData::expressionClass |
- See also
- KDbExpression::expressionClass()
Definition at line 87 of file KDbExpressionData.h.
◆ parent
ExplicitlySharedExpressionDataPointer KDbExpressionData::parent |
Definition at line 88 of file KDbExpressionData.h.
◆ token
KDbToken KDbExpressionData::token |
- See also
- KDbExpression::token()
Definition at line 85 of file KDbExpressionData.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2024 The KDE developers.
Generated on Tue Mar 26 2024 11:21:01 by doxygen 1.10.0 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.