KDbFunctionExpression
#include <KDbExpression.h>
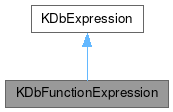
Static Public Member Functions | |
static QStringList | builtInAggregates () |
static KDbEscapedString | greatestOrLeastFunctionUsingCaseToString (const QString &name, const KDbDriver *driver, const KDbNArgExpression &args, KDbQuerySchemaParameterValueListIterator *params, KDb::ExpressionCallStack *callStack) |
static bool | isBuiltInAggregate (const QString &function) |
static KDbEscapedString | toString (const QString &name, const KDbDriver *driver, const KDbNArgExpression &args, KDbQuerySchemaParameterValueListIterator *params, KDb::ExpressionCallStack *callStack) |
![]() | |
static KDb::ExpressionClass | classForToken (KDbToken token) |
Additional Inherited Members | |
![]() | |
ExplicitlySharedExpressionDataPointer | d |
Detailed Description
The KDbFunctionExpression class represents expression that use functional notation F(x, ...)
The functions list include:
- aggregation functions like SUM, COUNT, MAX, ...
- builtin functions like CURRENT_TIME()
- user defined functions
Definition at line 502 of file KDbExpression.h.
Constructor & Destructor Documentation
◆ KDbFunctionExpression() [1/6]
KDbFunctionExpression::KDbFunctionExpression | ( | ) |
Constructs a null function expression.
- See also
- KDbExpression::isNull()
Definition at line 1309 of file KDbFunctionExpression.cpp.
◆ KDbFunctionExpression() [2/6]
|
explicit |
Constructs function expression with name name, without arguments.
Definition at line 1315 of file KDbFunctionExpression.cpp.
◆ KDbFunctionExpression() [3/6]
KDbFunctionExpression::KDbFunctionExpression | ( | const QString & | name, |
const KDbNArgExpression & | arguments ) |
Constructs function expression with name name and arguments arguments.
Definition at line 1321 of file KDbFunctionExpression.cpp.
◆ KDbFunctionExpression() [4/6]
KDbFunctionExpression::KDbFunctionExpression | ( | const KDbFunctionExpression & | expr | ) |
Constructs a copy of other function expression expr. Resulting object is not a deep copy but rather a reference to the object expr.
Definition at line 1328 of file KDbFunctionExpression.cpp.
◆ ~KDbFunctionExpression()
|
override |
Definition at line 1344 of file KDbFunctionExpression.cpp.
◆ KDbFunctionExpression() [5/6]
|
explicitprotected |
Definition at line 1333 of file KDbFunctionExpression.cpp.
◆ KDbFunctionExpression() [6/6]
|
explicitprotected |
Definition at line 1339 of file KDbFunctionExpression.cpp.
Member Function Documentation
◆ arguments()
KDbNArgExpression KDbFunctionExpression::arguments | ( | ) |
- Returns
- list of arguments of the function.
Definition at line 1382 of file KDbFunctionExpression.cpp.
◆ builtInAggregates()
|
static |
Definition at line 1355 of file KDbFunctionExpression.cpp.
◆ greatestOrLeastFunctionUsingCaseToString()
|
static |
- Returns
- a native (driver-specific) GREATEST() and LEAST() function calls generated to string using CASE WHEN... keywords. This is a workaround for cases when LEAST()/GREATEST() function ignores NULL values and only returns NULL if all the expressions evaluate to NULL. Instead of using F(v0,..,vN), this is used: (CASE WHEN (v0) IS NULL OR .. OR (vN) IS NULL THEN NULL ELSE F(v0,..,vN) END) where F == GREATEST or LEAST. Actually it is needed by MySQL < 5.0.13 and PostgreSQL.
Definition at line 1393 of file KDbFunctionExpression.cpp.
◆ isBuiltInAggregate()
|
static |
Definition at line 1349 of file KDbFunctionExpression.cpp.
◆ name()
QString KDbFunctionExpression::name | ( | ) | const |
- Returns
- name of the function.
Definition at line 1372 of file KDbFunctionExpression.cpp.
◆ setArguments()
void KDbFunctionExpression::setArguments | ( | const KDbNArgExpression & | arguments | ) |
Sets the list of arguments to arguments.
Definition at line 1387 of file KDbFunctionExpression.cpp.
◆ setName()
void KDbFunctionExpression::setName | ( | const QString & | name | ) |
Sets name of the function to name.
Definition at line 1377 of file KDbFunctionExpression.cpp.
◆ toString() [1/2]
KDbEscapedString KDbExpression::toString | ( | const KDbDriver * | driver, |
KDbQuerySchemaParameterValueListIterator * | params = nullptr, | ||
KDb::ExpressionCallStack * | callStack = nullptr ) const |
- Returns
- string as a representation of this expression element by running recursive calls. param, if not 0, points to a list item containing value of a query parameter (used in QueryParameterExpr). The result may depend on the optional driver parameter. If driver is 0, representation for portable KDbSQL dialect is returned.
Definition at line 124 of file KDbExpression.cpp.
◆ toString() [2/2]
|
static |
Constructs function expression with name name and args args.
Definition at line 1361 of file KDbFunctionExpression.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri May 2 2025 12:05:18 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.