KDbMultiValidator
#include <KDbValidator.h>
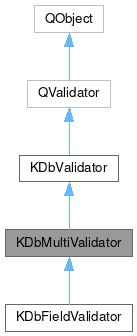
Public Member Functions | |
KDbMultiValidator (QObject *parent=nullptr) | |
KDbMultiValidator (QValidator *validator, QObject *parent=nullptr) | |
void | addSubvalidator (QValidator *validator, bool owned=true) |
void | fixup (QString &input) const override |
QValidator::State | validate (QString &input, int &pos) const override |
![]() | |
KDbValidator (QObject *parent=nullptr) | |
bool | acceptsEmptyValue () const |
void | addChildValidator (KDbValidator *v) |
Result | check (const QString &valueName, const QVariant &v, QString *message, QString *details) |
void | setAcceptsEmptyValue (bool set) |
QValidator::State | validate (QString &input, int &pos) const override |
![]() | |
QValidator (QObject *parent) | |
void | changed () |
QLocale | locale () const const |
void | setLocale (const QLocale &locale) |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Protected Member Functions | |
KDbValidator::Result | internalCheck (const QString &valueName, const QVariant &value, QString *message, QString *details) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const QMetaMethod &signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
enum | Result { Error = 0 , Ok = 1 , Warning = 2 } |
![]() | |
enum | State |
![]() | |
objectName | |
![]() | |
static const QString | messageColumnNotEmpty () |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
![]() | |
Acceptable | |
Intermediate | |
Invalid | |
![]() | |
typedef | QObjectList |
Detailed Description
A validator groupping multiple QValidators.
KDbMultiValidator behaves like normal KDbValidator, but it allows to add define more than one different validator. Given validation is successful if every subvalidator accepted given value.
- acceptsEmptyValue() is used globally here (no matter what is defined in subvalidators).
- result of calling check() depends on value of check() returned by subvalidators:
- Error is returned if at least one subvalidator returned Error;
- Warning is returned if at least one subvalidator returned Warning and no validator returned error;
- Ok is returned only if exactly all subvalidators returned Ok.
- If there is no subvalidators defined, Error is always returned.
- If a given subvalidator is not of class KDbValidator but ust QValidator, it's assumed it's check() method returned Ok.
- result of calling validate() (a method implemented for QValidator) depends on value of validate() returned by subvalidators:
- Invalid is returned if at least one subvalidator returned Invalid
- Intermediate is returned if at least one subvalidator returned Intermediate
- Acceptable is returned if exactly all subvalidators returned Acceptable.
- If there is no subvalidators defined, Invalid is always returned.
If there are no subvalidators, the multi validator always accepts the input.
Definition at line 119 of file KDbValidator.h.
Constructor & Destructor Documentation
◆ KDbMultiValidator() [1/2]
|
explicit |
Constructs multivalidator with no subvalidators defined. You can add more validators with addSubvalidator().
Definition at line 107 of file KDbValidator.cpp.
◆ KDbMultiValidator() [2/2]
|
explicit |
Constructs multivalidator with one validator validator. It will be owned if has no parent defined. You can add more validators with addSubvalidator().
Definition at line 113 of file KDbValidator.cpp.
◆ ~KDbMultiValidator()
|
override |
Definition at line 120 of file KDbValidator.cpp.
Member Function Documentation
◆ addSubvalidator()
void KDbMultiValidator::addSubvalidator | ( | QValidator * | validator, |
bool | owned = true ) |
Adds validator validator as another subvalidator. Subvalidator will be owned by this multivalidator if owned is true and its parent is nullptr
.
Definition at line 125 of file KDbValidator.cpp.
◆ fixup()
|
overridevirtual |
Calls QValidator::fixup() on every subvalidator. This may be senseless to use this methog in certain cases (can return weir results), so think twice before..
Reimplemented from QValidator.
Definition at line 145 of file KDbValidator.cpp.
◆ internalCheck()
|
overrideprotectedvirtual |
Reimplemented from KDbValidator.
Definition at line 152 of file KDbValidator.cpp.
◆ validate()
|
overridevirtual |
Reimplemented to call validate() on subvalidators.
Implements QValidator.
Definition at line 134 of file KDbValidator.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Mar 28 2025 11:54:34 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.