KirigamiActionCollection
#include <KirigamiActionCollection>
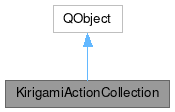
Properties | |
QString | configGroup |
bool | configIsGlobal |
![]() | |
objectName | |
Signals | |
void | actionHovered (QAction *action) |
void | actionTriggered (QAction *action) |
void | changed () |
void | inserted (QAction *action) |
Public Member Functions | |
KirigamiActionCollection (QObject *parent, const QString &cName=QString()) | |
~KirigamiActionCollection () override | |
Q_INVOKABLE QAction * | action (const QString &name) const |
QAction * | action (int index) const |
const QList< QActionGroup * > | actionGroups () const |
QList< QAction * > | actions () const |
const QList< QAction * > | actionsWithoutGroup () const |
template<class ActionType> | |
ActionType * | add (const QString &name, const Receiver *receiver, Func slot) |
QAction * | addAction (const QString &name, const Receiver *receiver, Func slot) |
Q_INVOKABLE QAction * | addAction (const QString &name, QAction *action) |
QAction * | addAction (KStandardActions::StandardAction actionType, const QString &name, const Receiver *receiver, Func slot) |
void | addActions (const QList< QAction * > &actions) |
void | clear () |
QString | componentDisplayName () const |
QString | componentName () const |
QString | configGroup () const |
bool | configIsGlobal () const |
int | count () const |
bool | isEmpty () const |
void | readSettings (KConfigGroup *config=nullptr) |
void | removeAction (QAction *action) |
void | setComponentDisplayName (const QString &displayName) |
void | setComponentName (const QString &componentName) |
void | setConfigGlobal (bool global) |
void | setConfigGroup (const QString &group) |
QAction * | takeAction (QAction *action) |
void | writeSettings (KConfigGroup *config=nullptr, bool writeDefaults=false, QAction *oneAction=nullptr) const |
![]() | |
QObject (QObject *parent) | |
QBindable< QString > | bindableObjectName () |
bool | blockSignals (bool block) |
const QObjectList & | children () const const |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) const const |
bool | disconnect (const QObject *receiver, const char *method) const const |
void | dumpObjectInfo () const const |
void | dumpObjectTree () const const |
QList< QByteArray > | dynamicPropertyNames () const const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QRegularExpression &re, Qt::FindChildOptions options) const const |
QList< T > | findChildren (const QString &name, Qt::FindChildOptions options) const const |
QList< T > | findChildren (Qt::FindChildOptions options) const const |
bool | inherits (const char *className) const const |
void | installEventFilter (QObject *filterObj) |
bool | isQuickItemType () const const |
bool | isWidgetType () const const |
bool | isWindowType () const const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const const |
void | moveToThread (QThread *targetThread) |
QString | objectName () const const |
void | objectNameChanged (const QString &objectName) |
QObject * | parent () const const |
QVariant | property (const char *name) const const |
Q_CLASSINFO (Name, Value) | |
Q_EMIT Q_EMIT | |
Q_ENUM (...) | |
Q_ENUM_NS (...) | |
Q_ENUMS (...) | |
Q_FLAG (...) | |
Q_FLAG_NS (...) | |
Q_FLAGS (...) | |
Q_GADGET Q_GADGET | |
Q_GADGET_EXPORT (EXPORT_MACRO) | |
Q_INTERFACES (...) | |
Q_INVOKABLE Q_INVOKABLE | |
Q_MOC_INCLUDE Q_MOC_INCLUDE | |
Q_NAMESPACE Q_NAMESPACE | |
Q_NAMESPACE_EXPORT (EXPORT_MACRO) | |
Q_OBJECT Q_OBJECT | |
Q_PROPERTY (...) | |
Q_REVISION Q_REVISION | |
Q_SET_OBJECT_NAME (Object) | |
Q_SIGNAL Q_SIGNAL | |
Q_SIGNALS Q_SIGNALS | |
Q_SLOT Q_SLOT | |
Q_SLOTS Q_SLOTS | |
T | qobject_cast (const QObject *object) |
T | qobject_cast (QObject *object) |
QT_NO_NARROWING_CONVERSIONS_IN_CONNECT QT_NO_NARROWING_CONVERSIONS_IN_CONNECT | |
void | removeEventFilter (QObject *obj) |
void | setObjectName (const QString &name) |
void | setObjectName (QAnyStringView name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | setProperty (const char *name, QVariant &&value) |
bool | signalsBlocked () const const |
int | startTimer (int interval, Qt::TimerType timerType) |
int | startTimer (std::chrono::milliseconds interval, Qt::TimerType timerType) |
QThread * | thread () const const |
Static Public Member Functions | |
static const QList< KirigamiActionCollection * > & | allCollections () |
static QKeySequence | defaultShortcut (QAction *action) |
static QList< QKeySequence > | defaultShortcuts (QAction *action) |
static bool | isShortcutsConfigurable (QAction *action) |
static void | setDefaultShortcut (QAction *action, const QKeySequence &shortcut) |
static Q_INVOKABLE void | setDefaultShortcuts (QAction *action, const QList< QKeySequence > &shortcuts) |
static void | setShortcutsConfigurable (QAction *action, bool configurable) |
![]() | |
QMetaObject::Connection | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *context, Functor functor, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method, Qt::ConnectionType type) |
QMetaObject::Connection | connect (const QObject *sender, PointerToMemberFunction signal, Functor functor) |
bool | disconnect (const QMetaObject::Connection &connection) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
bool | disconnect (const QObject *sender, PointerToMemberFunction signal, const QObject *receiver, PointerToMemberFunction method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
Protected Slots | |
virtual void | slotActionTriggered () |
Protected Member Functions | |
void | connectNotify (const QMetaMethod &signal) override |
![]() | |
virtual void | childEvent (QChildEvent *event) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const QMetaMethod &signal) |
bool | isSignalConnected (const QMetaMethod &signal) const const |
int | receivers (const char *signal) const const |
QObject * | sender () const const |
int | senderSignalIndex () const const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
typedef | QObjectList |
Detailed Description
A container for a set of QAction objects.
KirigamiActionCollection manages a set of QAction objects. It allows them to be grouped for organized presentation of configuration to the user, saving + loading of configuration, and optionally for automatic plugging into specified widget(s).
Additionally, KirigamiActionCollection provides several convenience functions for locating named actions, and actions grouped by QActionGroup.
- Since
- KirigamiAddons 1.4.0
Definition at line 43 of file kirigamiactioncollection.h.
Property Documentation
◆ configGroup
|
readwrite |
Definition at line 47 of file kirigamiactioncollection.h.
◆ configIsGlobal
|
readwrite |
Definition at line 48 of file kirigamiactioncollection.h.
Constructor & Destructor Documentation
◆ KirigamiActionCollection()
|
explicit |
Constructor.
Allows specification of a component name other than the default application name, where needed (remember to call setComponentDisplayName() too).
Definition at line 69 of file kirigamiactioncollection.cpp.
◆ ~KirigamiActionCollection()
|
override |
Destructor.
Definition at line 77 of file kirigamiactioncollection.cpp.
Member Function Documentation
◆ action() [1/2]
Get the action with the given name
from the action collection.
This won't return the action for the menus defined using a "<Menu>" tag in XMLGUI files (e.g. "<Menu name="menuId">" in "applicationNameui.rc"). To access menu actions defined like this, use e.g.
after having called setupGUI() or createGUI().
- Parameters
-
name Name of the QAction
- Returns
- A pointer to the QAction in the collection which matches the parameters or null if nothing matches.
Definition at line 89 of file kirigamiactioncollection.cpp.
◆ action() [2/2]
QAction * KirigamiActionCollection::action | ( | int | index | ) | const |
Return the QAction* at position index
in the action collection.
This is equivalent to actions().value(index);
Definition at line 100 of file kirigamiactioncollection.cpp.
◆ actionGroups()
const QList< QActionGroup * > KirigamiActionCollection::actionGroups | ( | ) | const |
Returns the list of all QActionGroups associated with actions in this action collection.
Definition at line 162 of file kirigamiactioncollection.cpp.
◆ actionHovered
|
signal |
Indicates that action
was hovered.
◆ actions()
Returns the list of QActions which belong to this action collection.
The list is guaranteed to be in the same order the action were put into the collection.
Definition at line 146 of file kirigamiactioncollection.cpp.
◆ actionsWithoutGroup()
Returns the list of QActions without an QAction::actionGroup() which belong to this action collection.
Definition at line 151 of file kirigamiactioncollection.cpp.
◆ actionTriggered
|
signal |
Indicates that action
was triggered.
◆ add()
|
inline |
Creates a new action under the given name, adds it to the collection and connects the action's triggered(bool) signal to the specified receiver/member.
The type of the action is specified by the template parameter ActionType.
The KirigamiActionCollection takes ownership of the action object.
- Parameters
-
name The internal name of the action (e.g. "file-open"). receiver The QObject to connect the triggered(bool) signal to. slot The slot or lambda to connect the triggered(bool) signal to.
- Returns
- new action of the given type ActionType.
- See also
- add(const QString &, const QObject *, const char *)
Definition at line 334 of file kirigamiactioncollection.h.
◆ addAction() [1/3]
|
inline |
Creates a new action under the given name to the collection and connects the action's triggered(bool) signal to the specified receiver/member.
The newly created action is returned.
- Parameters
-
name The internal name of the action (e.g. "file-open"). receiver The QObject to connect the triggered(bool) signal to. slot The slot or lambda to connect the triggered(bool) signal to.
- Returns
- new action of the given type ActionType.
Definition at line 358 of file kirigamiactioncollection.h.
◆ addAction() [2/3]
Add an action under the given name to the collection.
Inserting an action that was previously inserted under a different name will replace the old entry, i.e. the action will not be available under the old name anymore but only under the new one.
Inserting an action under a name that is already used for another action will replace the other action in the collection (but will not delete it).
If KAuthorized::authorizeAction() reports that the action is not authorized, it will be disabled and hidden.
The ownership of the action object is not transferred. If the action is destroyed it will be removed automatically from the KirigamiActionCollection.
- Parameters
-
name The name by which the action be retrieved again from the collection. action The action to add.
- Returns
- the same as the action given as parameter. This is just for convenience (chaining calls) and consistency with the other addAction methods, you can also simply ignore the return value.
Definition at line 173 of file kirigamiactioncollection.cpp.
◆ addAction() [3/3]
|
inline |
Creates a new standard action, adds it to the collection and connects the action's triggered(bool) signal to the specified receiver/member.
The newly created action is also returned.
The action can be retrieved later from the collection by its standard name as per KStandardActions::stdName.
The KirigamiActionCollection takes ownership of the action object.
- Parameters
-
actionType The standard action type of the action to create. name The name by which the action be retrieved again from the collection. receiver The QObject to connect the triggered(bool) signal to. slot The slot or lambda to connect the triggered(bool) signal to.
- Returns
- new action of the given type ActionType.
Definition at line 303 of file kirigamiactioncollection.h.
◆ addActions()
Adds a list of actions to the collection.
The objectName of the actions is used as their internal name in the collection.
The ownership of the action objects is not transferred. If the action is destroyed it will be removed automatically from the KirigamiActionCollection.
Uses addAction(const QString&, QAction*).
- Parameters
-
actions the list of the actions to add.
- See also
- addAction()
Definition at line 260 of file kirigamiactioncollection.cpp.
◆ allCollections()
|
static |
Access the list of all action collections in existence for this app.
Definition at line 493 of file kirigamiactioncollection.cpp.
◆ changed
|
signal |
Emitted when an action has been inserted into, or removed from, this action collection.
◆ clear()
void KirigamiActionCollection::clear | ( | ) |
Clears the entire action collection, deleting all actions.
Definition at line 82 of file kirigamiactioncollection.cpp.
◆ componentDisplayName()
QString KirigamiActionCollection::componentDisplayName | ( | ) | const |
The display name for the associated component.
Definition at line 135 of file kirigamiactioncollection.cpp.
◆ componentName()
QString KirigamiActionCollection::componentName | ( | ) | const |
The component name with which this class is associated.
Definition at line 125 of file kirigamiactioncollection.cpp.
◆ configGroup()
QString KirigamiActionCollection::configGroup | ( | ) | const |
Returns the KConfig group with which settings will be loaded and saved.
Definition at line 318 of file kirigamiactioncollection.cpp.
◆ configIsGlobal()
bool KirigamiActionCollection::configIsGlobal | ( | ) | const |
Returns whether this action collection's configuration should be global to KDE ( true
), or specific to the application ( false
).
Definition at line 328 of file kirigamiactioncollection.cpp.
◆ connectNotify()
|
overrideprotectedvirtual |
Overridden to perform connections when someone wants to know whether an action was highlighted or triggered.
Reimplemented from QObject.
Definition at line 467 of file kirigamiactioncollection.cpp.
◆ count()
int KirigamiActionCollection::count | ( | ) | const |
Returns the number of actions in the collection.
This is equivalent to actions().count().
Definition at line 106 of file kirigamiactioncollection.cpp.
◆ defaultShortcut()
|
static |
Get the default primary shortcut for the given action.
- Parameters
-
action the action for which the default primary shortcut should be returned.
- Returns
- the default primary shortcut of the given action
Definition at line 284 of file kirigamiactioncollection.cpp.
◆ defaultShortcuts()
|
static |
Get the default shortcuts for the given action.
- Parameters
-
action the action for which the default shortcuts should be returned.
- Returns
- the default shortcuts of the given action
Definition at line 290 of file kirigamiactioncollection.cpp.
◆ inserted
|
signal |
Indicates that action
was inserted into this action collection.
◆ isEmpty()
bool KirigamiActionCollection::isEmpty | ( | ) | const |
Returns whether the action collection is empty or not.
Definition at line 111 of file kirigamiactioncollection.cpp.
◆ isShortcutsConfigurable()
|
static |
Returns true if the given action's shortcuts may be configured by the user.
- Parameters
-
action the action for the hint should be verified.
Definition at line 306 of file kirigamiactioncollection.cpp.
◆ readSettings()
void KirigamiActionCollection::readSettings | ( | KConfigGroup * | config = nullptr | ) |
Read all key associations from config
.
If config
is zero, read all key associations from the application's configuration file KSharedConfig::openConfig(), in the group set by setConfigGroup().
Definition at line 338 of file kirigamiactioncollection.cpp.
◆ removeAction()
void KirigamiActionCollection::removeAction | ( | QAction * | action | ) |
Removes an action from the collection and deletes it.
- Parameters
-
action The action to remove.
Definition at line 267 of file kirigamiactioncollection.cpp.
◆ setComponentDisplayName()
void KirigamiActionCollection::setComponentDisplayName | ( | const QString & | displayName | ) |
Set the component display name associated with this action collection.
(e.g. for the toolbar editor) KXMLGUIClient::setComponentName takes care of calling this.
Definition at line 130 of file kirigamiactioncollection.cpp.
◆ setComponentName()
void KirigamiActionCollection::setComponentName | ( | const QString & | componentName | ) |
Set the componentName
associated with this action collection.
- Warning
- Don't call this method on a KirigamiActionCollection that contains actions. This is not supported.
- Parameters
-
componentData the name which is to be associated with this action collection, or QString() to indicate the app name. This is used to load/save settings into XML files. KXMLGUIClient::setComponentName takes care of calling this.
Definition at line 116 of file kirigamiactioncollection.cpp.
◆ setConfigGlobal()
void KirigamiActionCollection::setConfigGlobal | ( | bool | global | ) |
Set whether this action collection's configuration should be global to KDE ( true
), or specific to the application ( false
).
Definition at line 333 of file kirigamiactioncollection.cpp.
◆ setConfigGroup()
void KirigamiActionCollection::setConfigGroup | ( | const QString & | group | ) |
Sets group
as the KConfig group with which settings will be loaded and saved.
Definition at line 323 of file kirigamiactioncollection.cpp.
◆ setDefaultShortcut()
|
static |
Set the default shortcut for the given action.
- Parameters
-
action the action for which the default shortcut should be set. shortcut the shortcut to use for the given action in its specified shortcutContext()
Definition at line 295 of file kirigamiactioncollection.cpp.
◆ setDefaultShortcuts()
|
static |
Set the default shortcuts for the given action.
- Parameters
-
action the action for which the default shortcut should be set. shortcuts the shortcuts to use for the given action in its specified shortcutContext()
Definition at line 300 of file kirigamiactioncollection.cpp.
◆ setShortcutsConfigurable()
|
static |
Indicate whether the user may configure the action's shortcuts.
- Parameters
-
action the action for the hint should be verified. configurable set to true if the shortcuts of the given action may be configured by the user, otherwise false.
Definition at line 313 of file kirigamiactioncollection.cpp.
◆ slotActionTriggered
|
protectedvirtualslot |
Definition at line 432 of file kirigamiactioncollection.cpp.
◆ takeAction()
Removes an action from the collection.
The ownership of the action object is not changed.
- Parameters
-
action the action to remove.
Definition at line 272 of file kirigamiactioncollection.cpp.
◆ writeSettings()
void KirigamiActionCollection::writeSettings | ( | KConfigGroup * | config = nullptr, |
bool | writeDefaults = false, | ||
QAction * | oneAction = nullptr ) const |
Write the current configurable key associations to config
.
What the function does if config
is zero depends. If this action collection belongs to a KXMLGUIClient the setting are saved to the kxmlgui definition file. If not the settings are written to the applications config file.
- Note
oneAction
andwriteDefaults
have no meaning for the kxmlgui configuration file.
- Parameters
-
config Config object to save to, or null (see above) writeDefaults set to true to write settings which are already at defaults. oneAction pass an action here if you just want to save the values for one action, eg. if you know that action is the only one which has changed.
Definition at line 369 of file kirigamiactioncollection.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2025 The KDE developers.
Generated on Fri Jan 24 2025 11:49:11 by doxygen 1.13.2 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.